Using the Sugar Market API to Get Contacts in Python
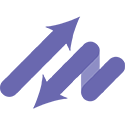
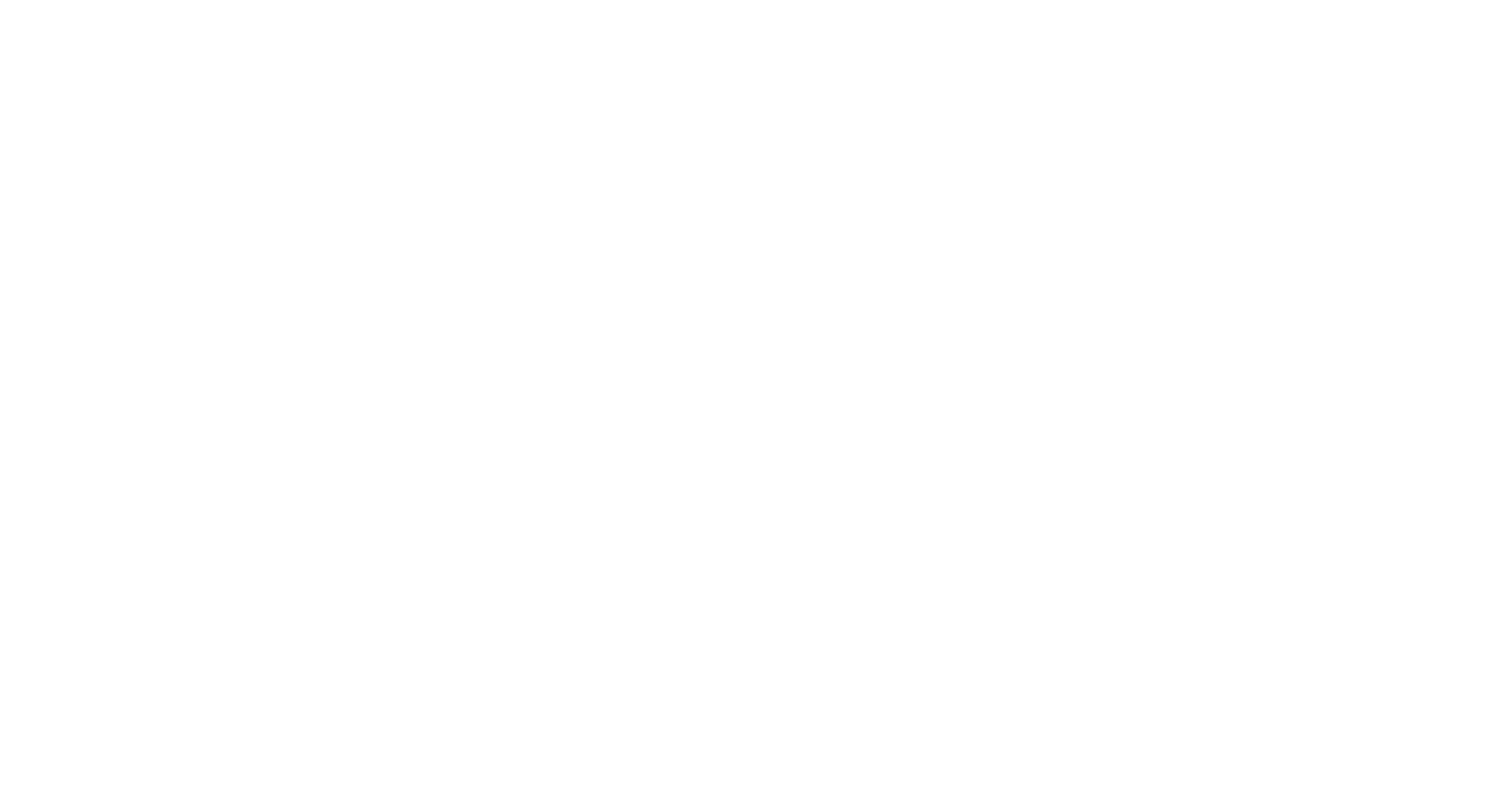
Introduction to Sugar Market API
Sugar Market is a robust marketing automation platform designed to enhance the marketing efforts of businesses by providing tools for campaign management, lead nurturing, and analytics. It offers a comprehensive suite of features that help businesses streamline their marketing processes and improve customer engagement.
Integrating with the Sugar Market API allows developers to access and manage marketing data, such as contacts, to optimize marketing strategies. For example, a developer might use the Sugar Market API to retrieve contact information and analyze engagement metrics, enabling more personalized and effective marketing campaigns.
Setting Up Your Sugar Market Test Account
Before you can start interacting with the Sugar Market API, you'll need to set up a test or sandbox account. This allows you to safely experiment with API calls without affecting live data.
Creating a Sugar Market Account
If you don't already have a Sugar Market account, you can sign up for a free trial or request access to a sandbox environment through the Sugar Market website. Follow the instructions provided to complete the registration process.
Generating API Credentials for Sugar Market
Once your account is set up, you'll need to generate API credentials to authenticate your requests. Sugar Market uses a custom authentication method, so follow these steps to obtain your credentials:
- Log in to your Sugar Market account.
- Navigate to the API settings section, typically found under account settings or integrations.
- Create a new API application by providing necessary details such as application name and description.
- Once created, you'll receive a client ID and client secret. Keep these credentials secure as they will be used to authenticate your API requests.
Configuring OAuth Authentication
Sugar Market's API requires OAuth-based authentication. Here's how to set it up:
- Use the client ID and client secret obtained earlier to request an access token.
- Send a POST request to the token endpoint with the required parameters, including client ID, client secret, and grant type.
- Upon successful authentication, you'll receive an access token. This token must be included in the header of your API requests to authenticate them.
For detailed instructions, refer to the Sugar Market API documentation.
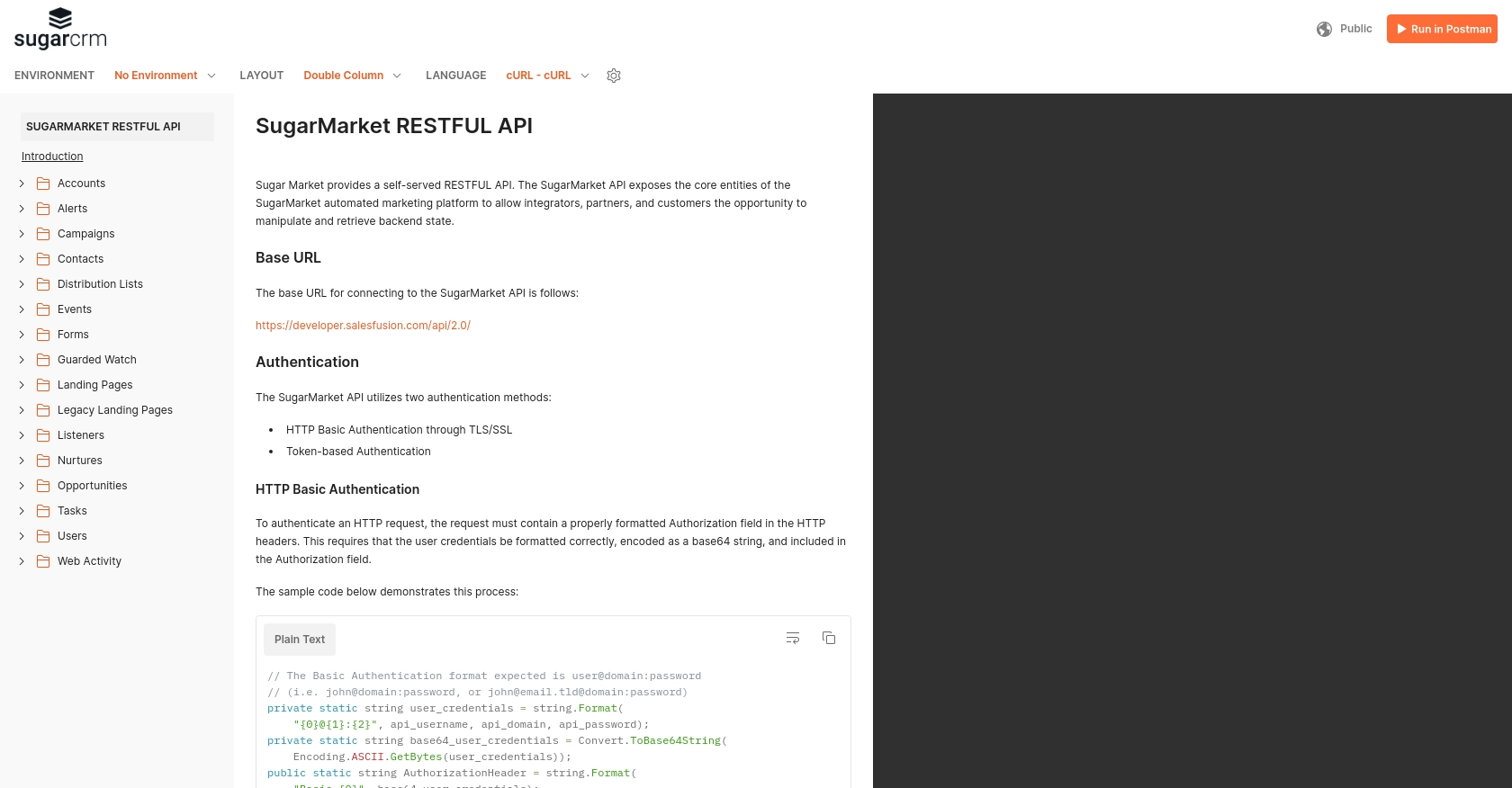
sbb-itb-96038d7
Making API Calls to Retrieve Contacts from Sugar Market Using Python
To interact with the Sugar Market API and retrieve contact data, you'll need to set up your Python environment and make the necessary API calls. This section will guide you through the process, ensuring you have the right tools and code to successfully access your contact information.
Setting Up Your Python Environment for Sugar Market API
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests.
- Verify your Python installation by running
python --version
in your terminal. - Install the
requests
library using pip:
pip install requests
Writing Python Code to Fetch Contacts from Sugar Market API
With your environment ready, you can now write the Python code to fetch contacts. Follow these steps to create a script that interacts with the Sugar Market API:
- Create a new Python file named
get_sugar_market_contacts.py
. - Add the following code to the file:
import requests
# Set the API endpoint and headers
endpoint = "https://api.sugarmarket.com/v1/contacts"
headers = {
"Authorization": "Bearer Your_Access_Token",
"Content-Type": "application/json"
}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
data = response.json()
# Loop through the contacts and print their information
for contact in data["contacts"]:
print(contact)
else:
print("Failed to retrieve contacts:", response.status_code, response.text)
Replace Your_Access_Token
with the access token you obtained during the authentication setup.
Running the Python Script and Verifying Results
To execute the script and retrieve contacts, run the following command in your terminal:
python get_sugar_market_contacts.py
If successful, the script will output the contact information retrieved from Sugar Market. Verify the results by checking the contact data against your test account.
Handling Errors and Troubleshooting API Requests
When making API calls, it's crucial to handle potential errors gracefully. The example code checks for a successful response (HTTP status code 200) and prints an error message if the request fails. Common error codes include:
- 401 Unauthorized: Check your access token and ensure it's valid.
- 403 Forbidden: Verify your API permissions and scopes.
- 500 Internal Server Error: Retry the request or contact Sugar Market support.
For more details on error handling, refer to the Sugar Market API documentation.
Conclusion and Best Practices for Using Sugar Market API in Python
Integrating with the Sugar Market API using Python can significantly enhance your marketing automation efforts by providing seamless access to contact data and other marketing resources. By following the steps outlined in this guide, you can efficiently retrieve and manage contacts, enabling more personalized and effective marketing strategies.
Best Practices for Secure and Efficient API Integration
- Secure Storage of Credentials: Always store your client ID, client secret, and access tokens securely. Consider using environment variables or a secure vault service to manage sensitive information.
- Handling Rate Limits: Be mindful of any rate limits imposed by the Sugar Market API. Implement retry logic with exponential backoff to handle rate limit errors gracefully. Check the Sugar Market API documentation for specific rate limit details.
- Data Transformation and Standardization: Ensure that the data retrieved from the API is transformed and standardized to fit your application's requirements. This may involve mapping fields or converting data types.
Streamlining Integrations with Endgrate
Building and maintaining multiple integrations can be time-consuming and complex. Endgrate offers a unified API solution that simplifies the integration process, allowing you to focus on your core product. By using Endgrate, you can:
- Save time and resources by outsourcing integration development.
- Build once for each use case instead of multiple times for different platforms.
- Provide an intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate's website.
Read More
Ready to get started?