Using the Outreach API to Create or Update Prospects (with Javascript examples)
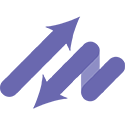
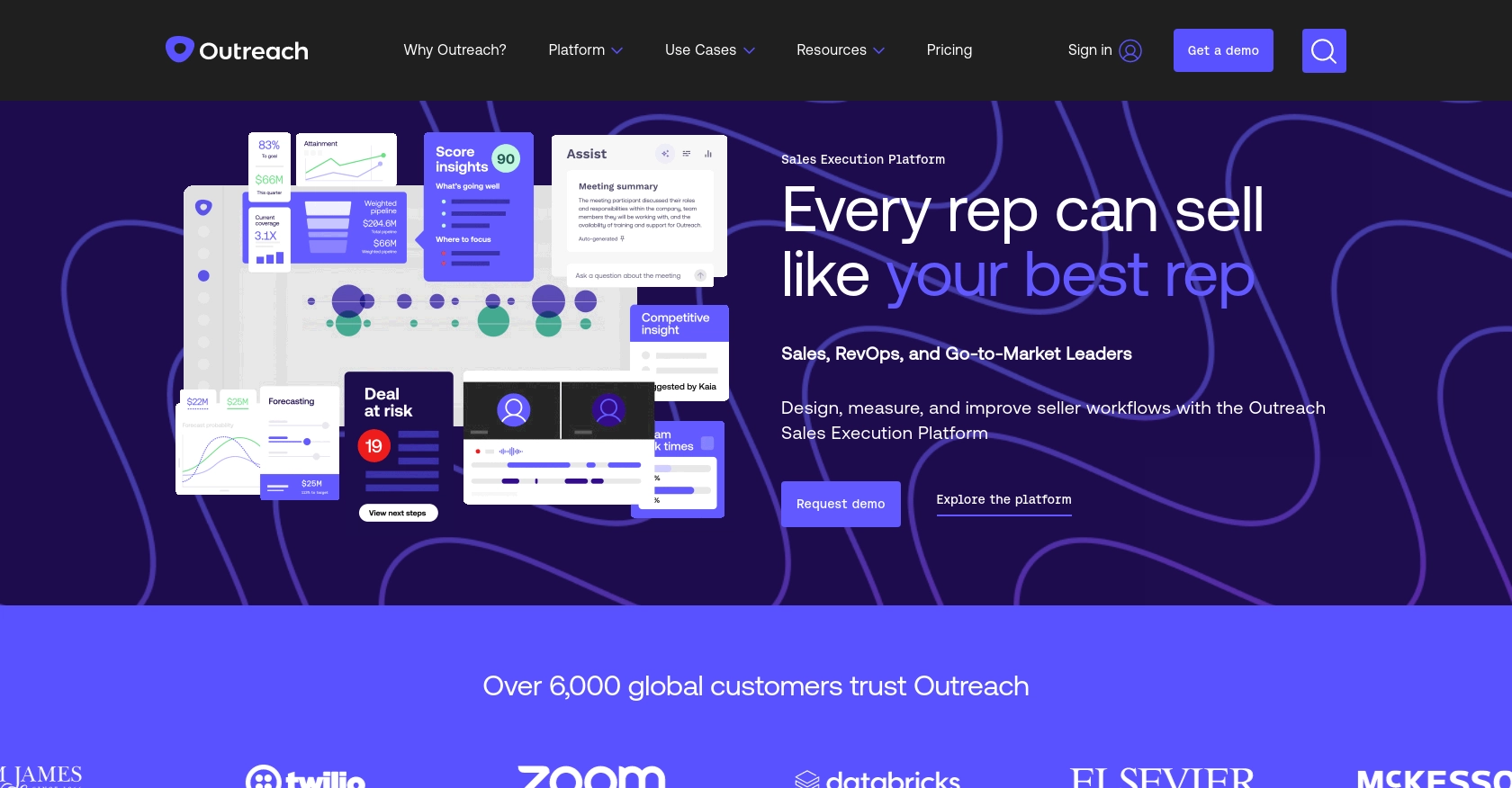
Introduction to Outreach API for Prospect Management
Outreach is a powerful sales engagement platform that helps businesses streamline their sales processes and improve communication with prospects. It offers a suite of tools designed to enhance productivity and drive better sales outcomes through automation and data-driven insights.
Integrating with the Outreach API allows developers to efficiently manage prospect data, enabling seamless updates and creation of prospect records. For example, a developer might use the Outreach API to automatically update prospect information based on interactions from other platforms, ensuring that sales teams have the most current data at their fingertips.
Setting Up Your Outreach Test or Sandbox Account
Before you can start integrating with the Outreach API, you need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting live data. Outreach provides a development environment where you can create and manage your applications.
Creating an Outreach Developer Account
If you don't already have an Outreach account, you can sign up for a developer account on the Outreach website. This account will give you access to the necessary tools and resources to begin your integration journey.
- Visit the Outreach Developer Portal.
- Follow the instructions to create your account.
- Once your account is set up, log in to access the developer dashboard.
Configuring OAuth for Outreach API Access
Outreach uses OAuth 2.0 for authentication, which requires you to create an application in the Outreach platform. This will provide you with the client ID and client secret needed for API access.
- Navigate to the "API Access" section in your Outreach developer dashboard.
- Click on "Create a New App" to start the process.
- Fill in the required details, including the app name and description.
- Specify one or more redirect URIs where users will be redirected after authorization.
- Select the necessary OAuth scopes that your application will require. Ensure you select scopes related to prospect management.
- Save your application settings to generate your client ID and client secret.
Note: Keep your client secret secure as it will only be displayed once. If needed, you can regenerate it.
Obtaining Access and Refresh Tokens
After setting up your OAuth application, you can obtain access and refresh tokens to authenticate API requests.
// Example of obtaining an access token using OAuth
const axios = require('axios');
const getToken = async () => {
try {
const response = await axios.post('https://api.outreach.io/oauth/token', {
client_id: 'Your_Client_ID',
client_secret: 'Your_Client_Secret',
redirect_uri: 'Your_Redirect_URI',
grant_type: 'authorization_code',
code: 'Authorization_Grant_Code'
});
console.log(response.data);
} catch (error) {
console.error(error);
}
};
getToken();
Replace Your_Client_ID
, Your_Client_Secret
, Your_Redirect_URI
, and Authorization_Grant_Code
with your actual credentials and authorization code.
For more details on setting up OAuth, refer to the Outreach OAuth Documentation.
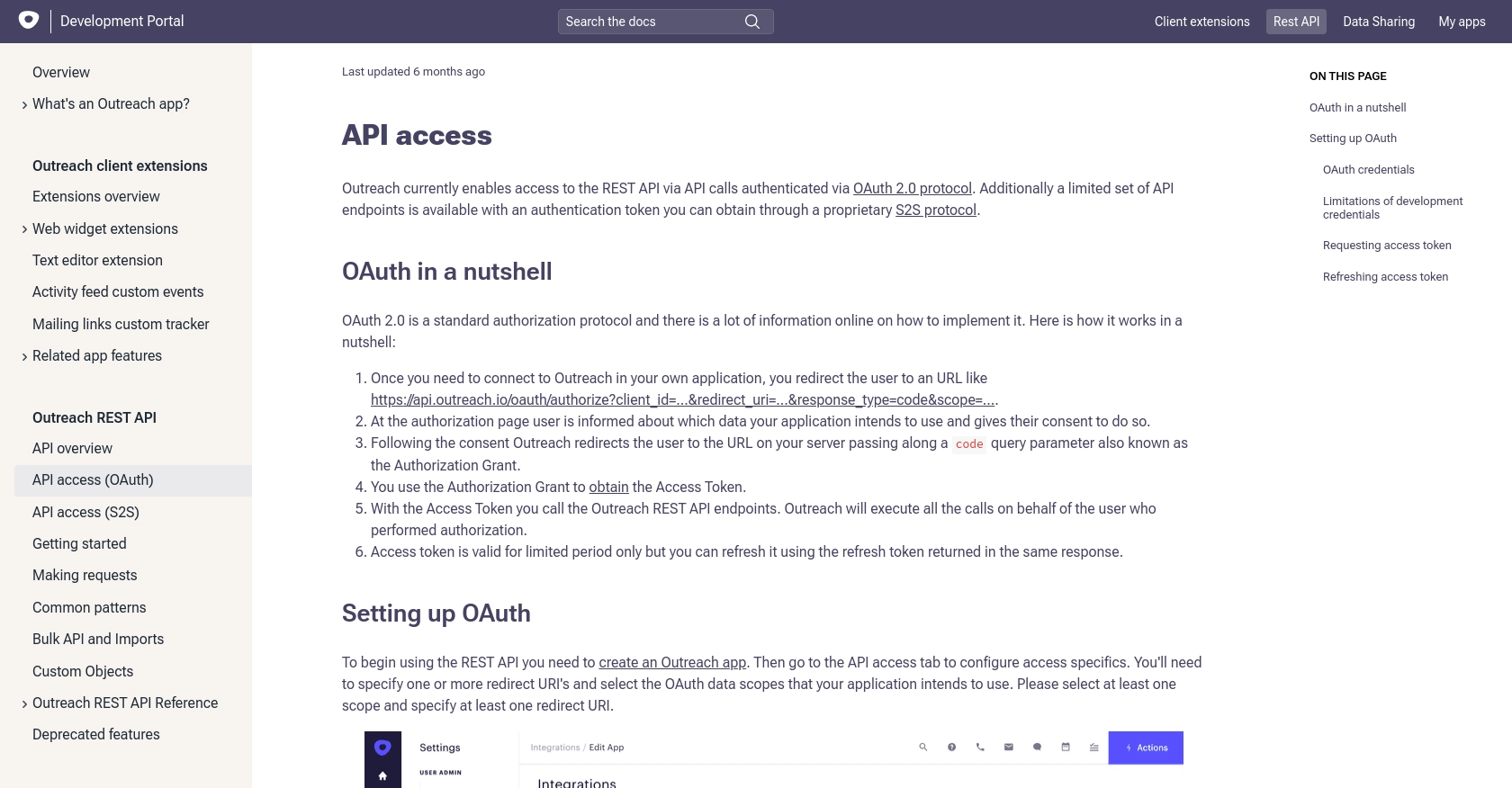
sbb-itb-96038d7
Making API Calls to Create or Update Prospects Using Outreach API with JavaScript
To interact with the Outreach API for creating or updating prospects, you'll need to use JavaScript to make HTTP requests. This section will guide you through the process of setting up your environment, writing the necessary code, and handling responses effectively.
Setting Up Your JavaScript Environment for Outreach API Integration
Before making API calls, ensure you have Node.js installed on your machine. You'll also need the Axios library to handle HTTP requests. Install Axios using npm:
npm install axios
Creating a New Prospect with Outreach API
To create a new prospect, you'll need to send a POST request to the Outreach API endpoint. Here's how you can do it using JavaScript:
const axios = require('axios');
const createProspect = async () => {
try {
const response = await axios.post('https://api.outreach.io/api/v2/prospects', {
data: {
attributes: {
firstName: 'John',
lastName: 'Doe',
email: 'john.doe@example.com'
}
}
}, {
headers: {
'Authorization': 'Bearer Your_Access_Token',
'Content-Type': 'application/vnd.api+json'
}
});
console.log('Prospect Created:', response.data);
} catch (error) {
console.error('Error Creating Prospect:', error.response.data);
}
};
createProspect();
Replace Your_Access_Token
with your actual access token. This code snippet sends a POST request to create a new prospect with specified attributes.
Updating an Existing Prospect with Outreach API
To update an existing prospect, you'll need to send a PATCH request. Here's an example:
const updateProspect = async (prospectId) => {
try {
const response = await axios.patch(`https://api.outreach.io/api/v2/prospects/${prospectId}`, {
data: {
attributes: {
firstName: 'Jane',
lastName: 'Smith'
}
}
}, {
headers: {
'Authorization': 'Bearer Your_Access_Token',
'Content-Type': 'application/vnd.api+json'
}
});
console.log('Prospect Updated:', response.data);
} catch (error) {
console.error('Error Updating Prospect:', error.response.data);
}
};
updateProspect('Prospect_ID');
Replace Prospect_ID
with the ID of the prospect you wish to update. This code updates the prospect's first and last name.
Handling API Responses and Errors
When making API calls, it's crucial to handle responses and potential errors. Successful requests will return a 200 or 201 status code, while errors might return codes like 422 for validation errors or 429 if rate limits are exceeded. Always check the response status and handle errors gracefully:
- Log error details for debugging.
- Implement retry logic for transient errors.
- Respect rate limits by monitoring the
X-RateLimit-Remaining
header.
For more information on error handling, refer to the Outreach API Documentation.
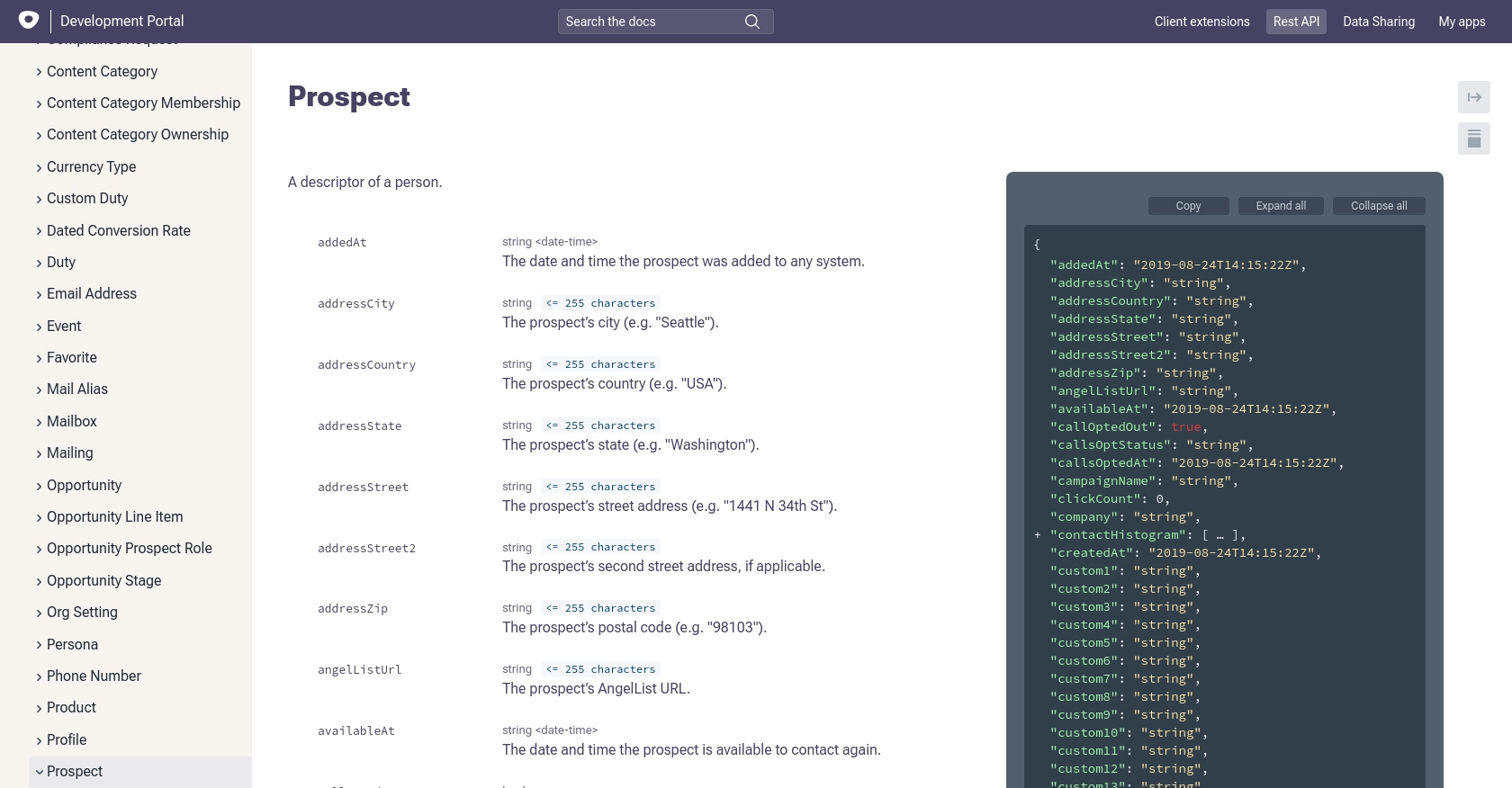
Conclusion and Best Practices for Using Outreach API with JavaScript
Integrating with the Outreach API to manage prospects can significantly enhance your sales processes by ensuring that your team has access to the most up-to-date information. By following the steps outlined in this guide, you can efficiently create and update prospect records using JavaScript, leveraging the power of automation to streamline your workflow.
Best Practices for Secure and Efficient API Integration
- Secure Storage of Credentials: Always store your client ID, client secret, and access tokens securely. Consider using environment variables or a secure vault to manage sensitive information.
- Handle Rate Limiting: The Outreach API has a rate limit of 10,000 requests per hour. Monitor the
X-RateLimit-Remaining
header and implement logic to handle 429 errors gracefully. - Use Refresh Tokens Wisely: Refresh tokens are valid for 14 days. Always use the latest refresh token to obtain new access tokens and avoid unnecessary token requests.
- Data Standardization: Ensure that data fields are standardized across platforms to maintain consistency and accuracy in prospect information.
Enhance Your Integration Strategy with Endgrate
While integrating with the Outreach API can be straightforward, managing multiple integrations across different platforms can become complex. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Outreach. This allows you to build once for each use case, saving time and resources.
With Endgrate, you can focus on your core product while outsourcing the intricacies of integration management. Explore how Endgrate can enhance your integration strategy by visiting Endgrate today.
Read More
Ready to get started?