How to Create Opportunities with the Sugar Market API in Javascript
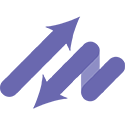
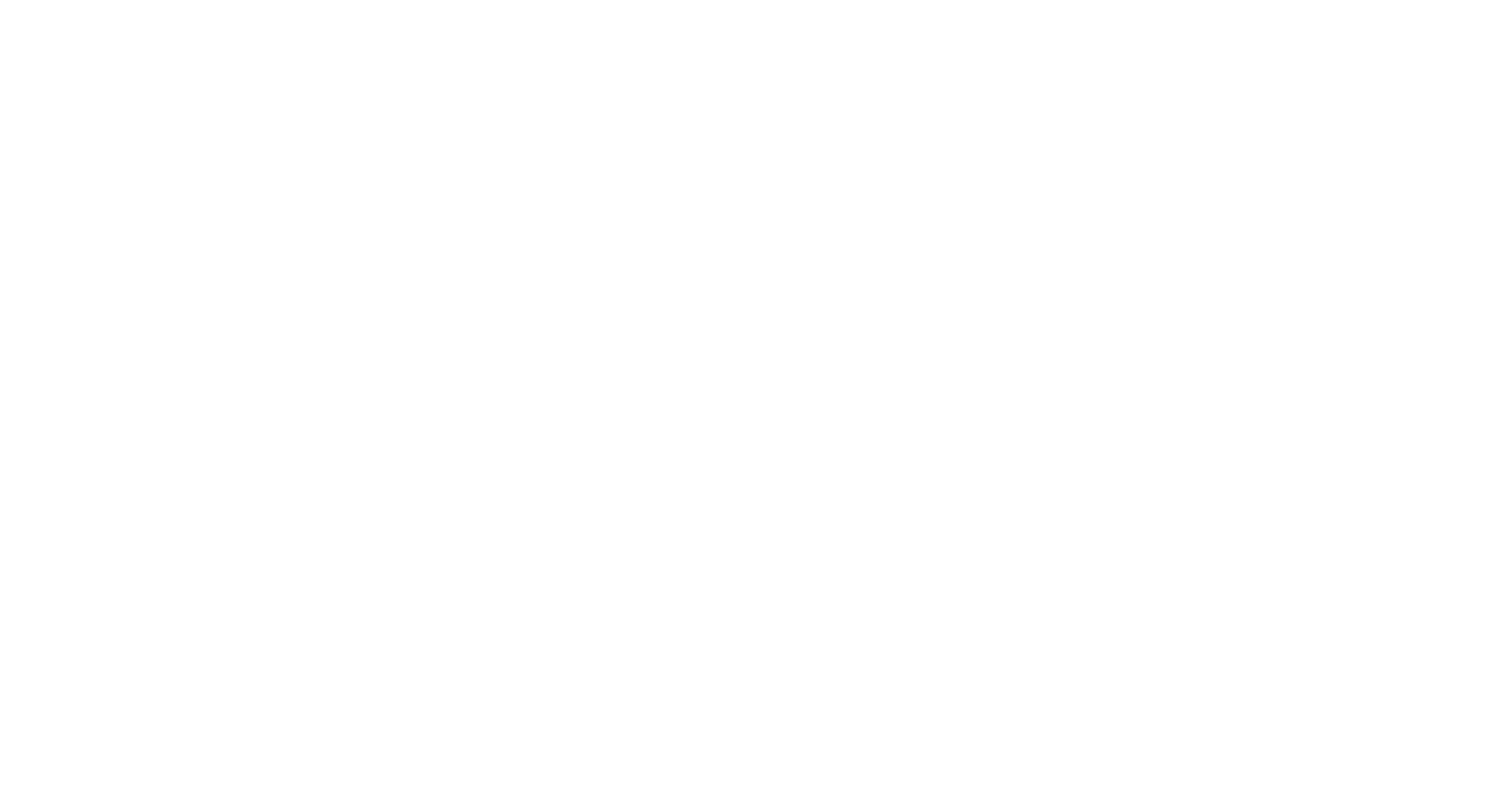
Introduction to Sugar Market API
Sugar Market is a robust marketing automation platform designed to enhance the marketing efforts of B2B businesses. It offers a comprehensive suite of tools that enable marketers to create, execute, and analyze campaigns effectively. With features like email marketing, lead nurturing, and advanced analytics, Sugar Market empowers businesses to drive growth and improve customer engagement.
Integrating with the Sugar Market API allows developers to automate and streamline various marketing processes. For example, developers can create opportunities within the Sugar Market platform using JavaScript, enabling seamless integration with other business systems and enhancing the efficiency of sales and marketing teams.
Setting Up Your Sugar Market Test Account for API Integration
Before you can start creating opportunities with the Sugar Market API using JavaScript, you'll need to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting live data.
Creating a Sugar Market Sandbox Account
To begin, you'll need to sign up for a Sugar Market account. If you don't already have one, visit the Sugar Market website and register for a free trial or demo account. Follow the on-screen instructions to complete the registration process.
Generating API Credentials for Sugar Market
Once your account is set up, you'll need to generate API credentials to authenticate your API requests. Sugar Market uses a custom authentication method, so follow these steps to obtain your credentials:
- Log in to your Sugar Market account.
- Navigate to the API settings section, typically found under the "Integrations" or "Developer" tab.
- Create a new API application by providing the necessary details such as application name and description.
- Once the application is created, you will receive a client ID and client secret. Make sure to store these securely as you'll need them for authentication.
Configuring OAuth for Sugar Market API
To authenticate your API requests, you'll need to implement OAuth 2.0 using the client ID and client secret obtained earlier. Here's a basic outline of the process:
- Use the client ID and client secret to request an access token from the Sugar Market authorization server.
- Include the access token in the header of your API requests to authenticate them.
With your sandbox account and API credentials ready, you're now set to start integrating with the Sugar Market API using JavaScript. In the next section, we'll explore how to make API calls to create opportunities.
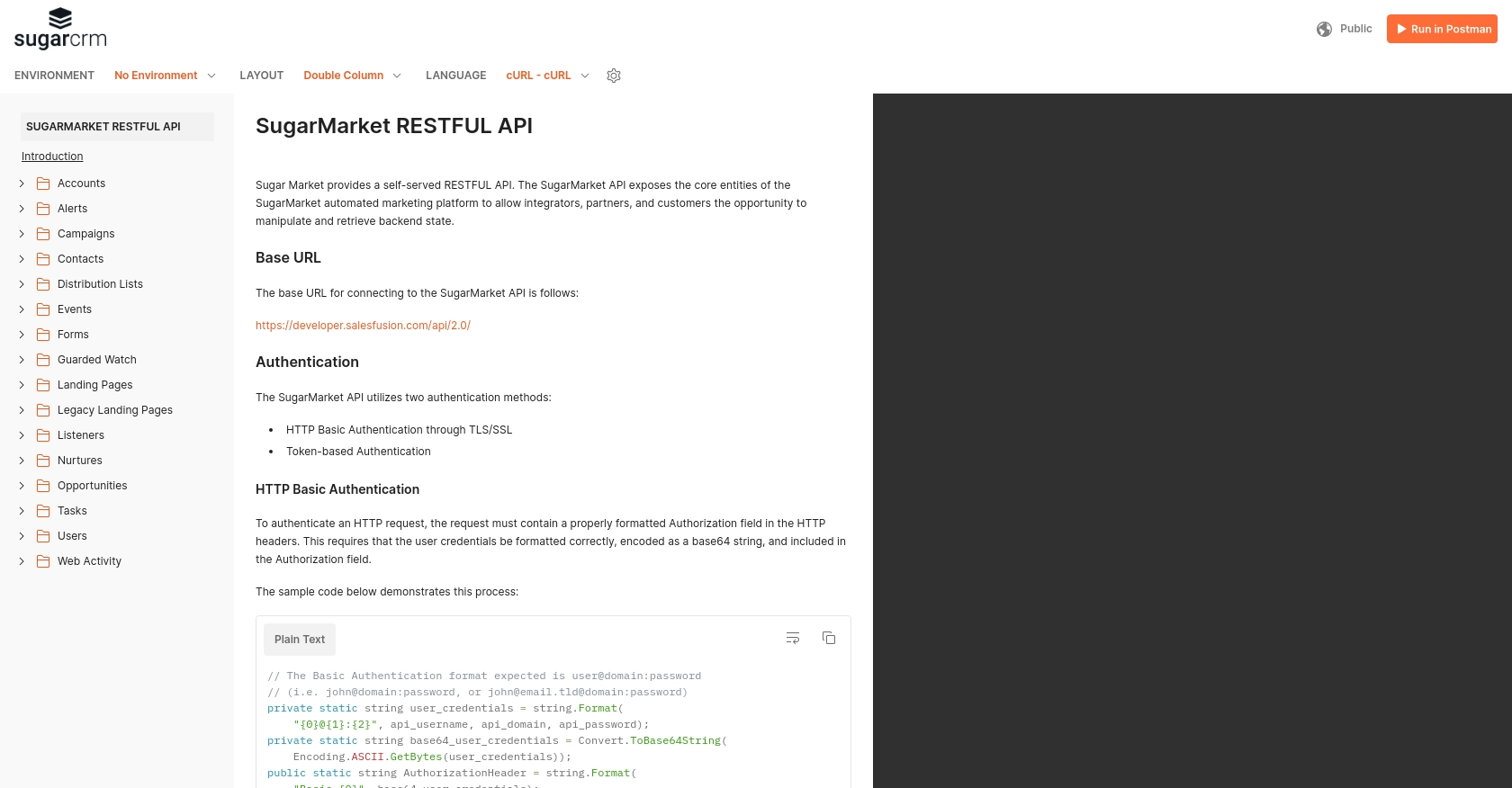
sbb-itb-96038d7
Making API Calls to Create Opportunities with Sugar Market API in JavaScript
With your Sugar Market sandbox account and API credentials ready, you can now proceed to make API calls to create opportunities using JavaScript. This section will guide you through the necessary steps, including setting up your environment and writing the code to interact with the Sugar Market API.
Setting Up Your JavaScript Environment for Sugar Market API Integration
Before making API calls, ensure that your development environment is properly configured. You'll need Node.js and npm (Node Package Manager) installed on your machine. If you haven't already, download and install them from the official Node.js website.
Once Node.js and npm are installed, create a new project directory and navigate to it in your terminal. Initialize a new Node.js project by running:
npm init -y
Next, install the Axios library, which will be used to make HTTP requests:
npm install axios
Writing JavaScript Code to Create Opportunities in Sugar Market
Now that your environment is set up, you can write the JavaScript code to create opportunities in Sugar Market. Create a new file named createOpportunity.js
and add the following code:
const axios = require('axios');
// Replace with your actual client ID and client secret
const clientId = 'YOUR_CLIENT_ID';
const clientSecret = 'YOUR_CLIENT_SECRET';
// Function to get access token
async function getAccessToken() {
try {
const response = await axios.post('https://api.sugarmarket.com/oauth/token', {
client_id: clientId,
client_secret: clientSecret,
grant_type: 'client_credentials'
});
return response.data.access_token;
} catch (error) {
console.error('Error obtaining access token:', error);
}
}
// Function to create an opportunity
async function createOpportunity() {
const accessToken = await getAccessToken();
if (!accessToken) return;
const opportunityData = {
name: 'New Business Opportunity',
amount: 5000,
close_date: '2023-12-31',
stage: 'Prospecting'
};
try {
const response = await axios.post('https://api.sugarmarket.com/opportunities', opportunityData, {
headers: {
Authorization: `Bearer ${accessToken}`,
'Content-Type': 'application/json'
}
});
console.log('Opportunity created successfully:', response.data);
} catch (error) {
console.error('Error creating opportunity:', error.response.data);
}
}
createOpportunity();
In this code, you first obtain an access token using the client ID and client secret. Then, you use this token to authenticate a POST request to the Sugar Market API, creating a new opportunity with specified details such as name, amount, close date, and stage.
Running Your JavaScript Code and Verifying the API Call
To execute the code, run the following command in your terminal:
node createOpportunity.js
If successful, you should see a confirmation message in the terminal indicating that the opportunity was created. You can verify this by checking the opportunities section in your Sugar Market sandbox account.
Handling Errors and Troubleshooting API Calls
When making API calls, it's important to handle potential errors gracefully. The code above includes basic error handling, logging any issues encountered during the token retrieval or opportunity creation process. Ensure that your client ID and client secret are correct, and check the API documentation for any specific error codes and their meanings.
For more detailed information on error handling and API usage, refer to the Sugar Market API documentation.
Conclusion and Best Practices for Sugar Market API Integration
Integrating with the Sugar Market API using JavaScript can significantly enhance your marketing automation processes by allowing seamless interaction with opportunities and other resources. By following the steps outlined in this guide, you can efficiently create opportunities and integrate them with your existing systems.
Best Practices for Secure and Efficient Sugar Market API Usage
- Secure Storage of Credentials: Always store your client ID and client secret securely. Consider using environment variables or secure vaults to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of the API rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Standardization: Ensure that the data you send and receive is standardized to maintain consistency across different systems and integrations.
- Error Handling: Implement robust error handling to manage API call failures. Log errors for troubleshooting and refer to the Sugar Market API documentation for specific error codes and solutions.
Streamlining Integrations with Endgrate
While integrating with individual APIs like Sugar Market can be beneficial, managing multiple integrations can become complex and time-consuming. This is where Endgrate can simplify your integration efforts. By providing a unified API endpoint, Endgrate allows you to connect with multiple platforms seamlessly, saving time and resources.
With Endgrate, you can focus on your core product development while outsourcing the complexities of integration management. Take advantage of an intuitive integration experience and build once for each use case instead of multiple times for different platforms.
Explore how Endgrate can transform your integration strategy by visiting Endgrate and discover the benefits of a streamlined integration process.
Read More
Ready to get started?