Using the Constant Contact API to Create or Update Contacts in Javascript
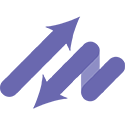
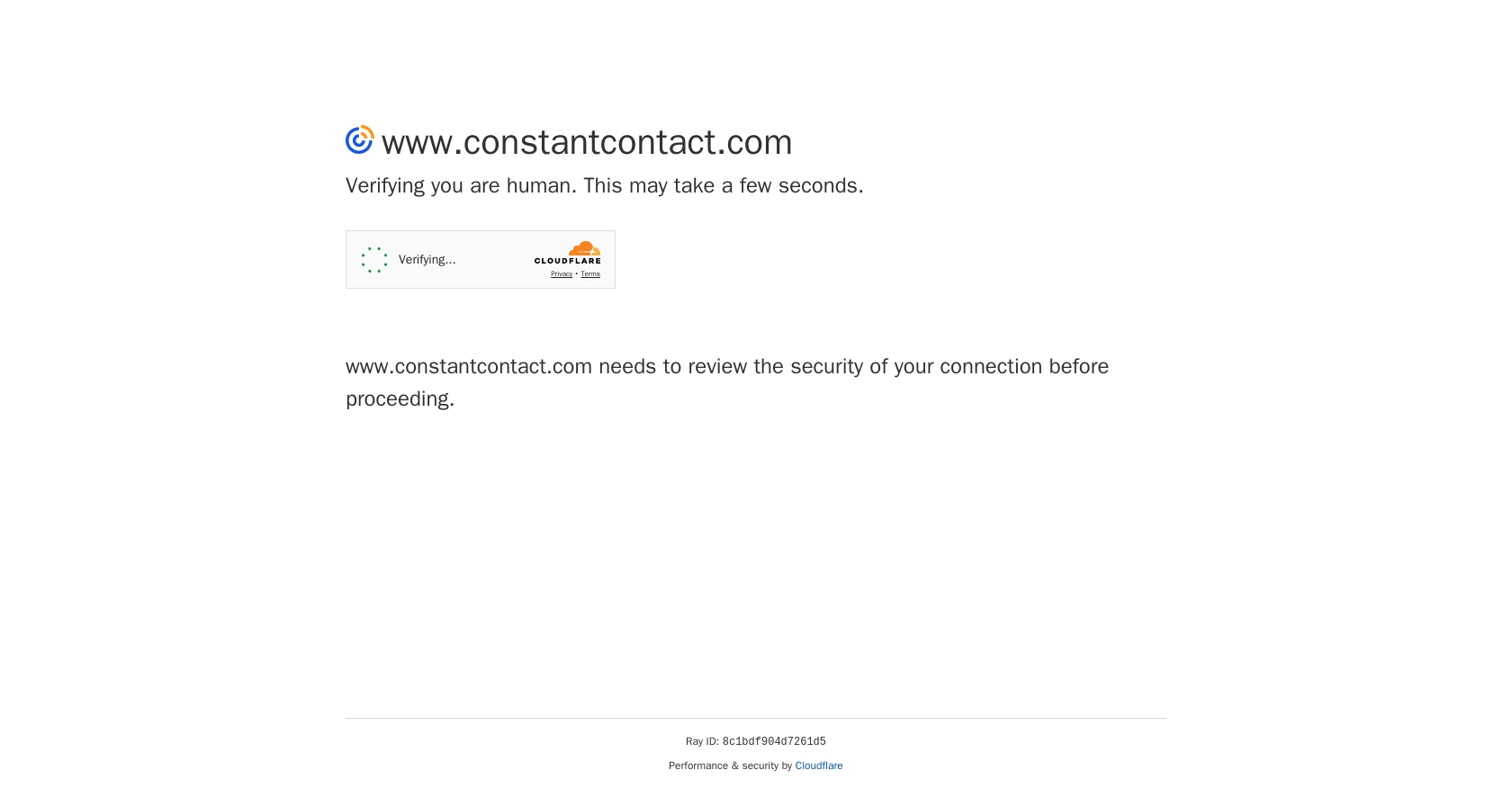
Introduction to Constant Contact API
Constant Contact is a powerful email marketing platform that enables businesses to create and manage effective email campaigns. With its robust set of tools, businesses can engage with their audience, track campaign performance, and grow their customer base.
Integrating with the Constant Contact API allows developers to automate and streamline marketing processes. For example, you can use the API to create or update contact information directly from your application, ensuring that your email lists are always up-to-date and accurate.
This article will guide you through using JavaScript to interact with the Constant Contact API, focusing on creating or updating contacts efficiently.
Setting Up Your Constant Contact Developer Account and OAuth2 Authentication
Before you can start using the Constant Contact API, you need to set up a developer account and configure OAuth2 authentication. This process will allow you to securely access and manage contact data within your application.
Create a Constant Contact Developer Account
- Visit the Constant Contact Developer Portal and sign up for a developer account.
- Once registered, log in to your account and navigate to the "My Applications" section.
- Click on "Create Application" to start setting up your new app.
- Fill in the required details such as application name, description, and redirect URI.
- Save your application to generate your client ID and client secret.
Configure OAuth2 Authentication for Constant Contact API
The Constant Contact API uses OAuth2 for authentication, which involves obtaining an access token to make API requests. Follow these steps to configure OAuth2:
- Choose the OAuth2 flow that suits your application type. For web applications, the Authorization Code Flow is recommended.
- Create an authorization request by making a GET call to the authorization endpoint:
- Direct users to the authorization URL to log in and authorize your application.
- Once authorized, the user will be redirected to your specified redirect URI with an authorization code.
- Exchange the authorization code for an access token by making a POST request to the token endpoint:
- Use the access token to authenticate your API requests by including it in the Authorization header:
const authUrl = `https://authz.constantcontact.com/oauth2/default/v1/authorize?client_id=${your_client_id}&redirect_uri=${your_redirect_uri}&response_type=code&scope=contact_data`;
const tokenUrl = 'https://authz.constantcontact.com/oauth2/default/v1/token';
const response = await fetch(tokenUrl, {
method: 'POST',
headers: {
'Content-Type': 'application/x-www-form-urlencoded'
},
body: `client_id=${your_client_id}&client_secret=${your_client_secret}&code=${authorization_code}&redirect_uri=${your_redirect_uri}&grant_type=authorization_code`
});
const data = await response.json();
const accessToken = data.access_token;
const apiUrl = 'https://api.cc.email/v3/contacts';
const apiResponse = await fetch(apiUrl, {
method: 'GET',
headers: {
'Authorization': `Bearer ${accessToken}`,
'Content-Type': 'application/json'
}
});
const contacts = await apiResponse.json();
By following these steps, you will have successfully set up your Constant Contact developer account and configured OAuth2 authentication, enabling you to interact with the API securely.
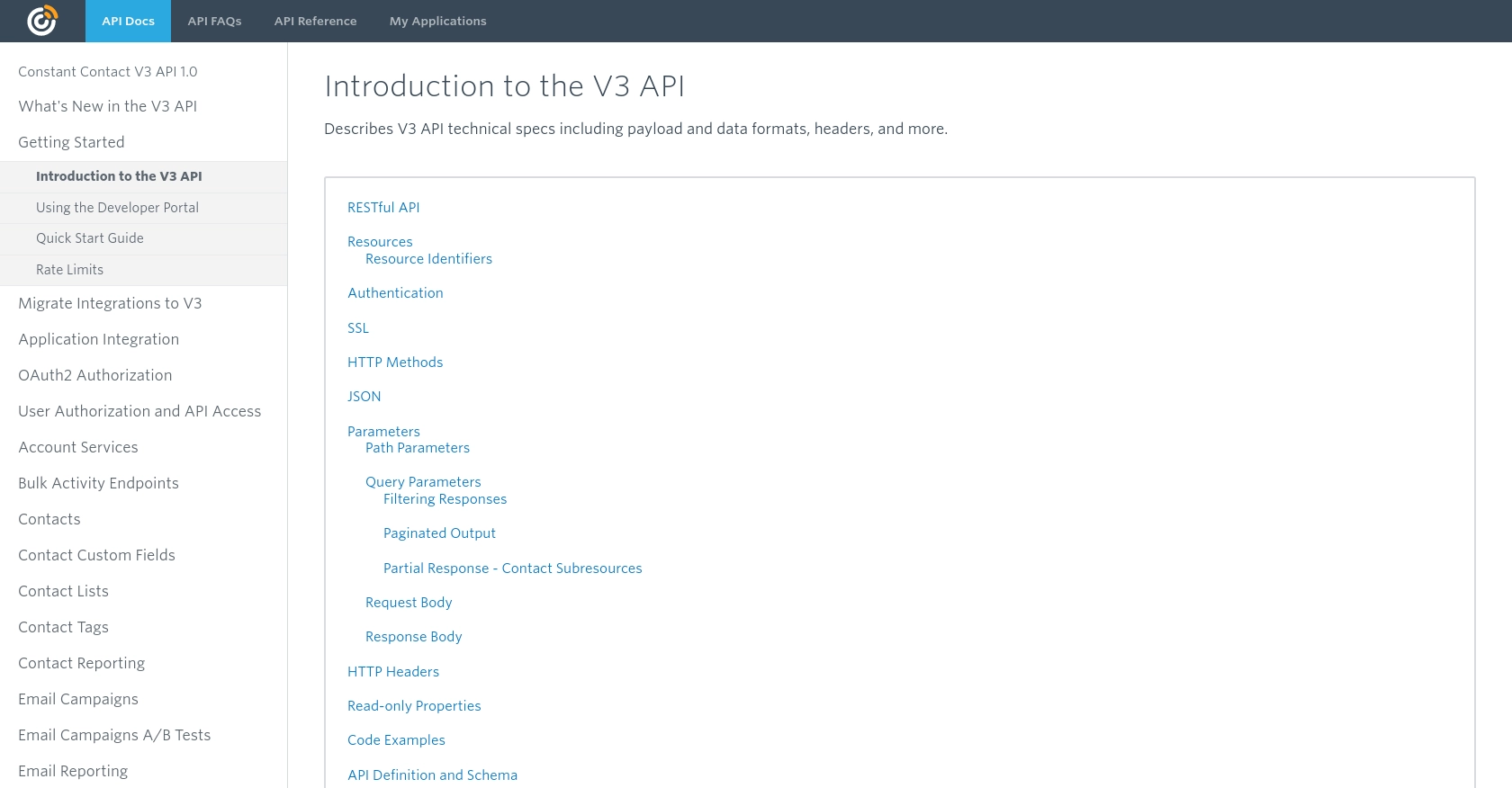
sbb-itb-96038d7
Making API Calls to Constant Contact for Creating or Updating Contacts Using JavaScript
Once you have set up your Constant Contact developer account and configured OAuth2 authentication, you can proceed to make API calls to create or update contacts. This section will guide you through the necessary steps and provide example code to help you interact with the Constant Contact API using JavaScript.
Prerequisites for Using JavaScript with Constant Contact API
Before making API calls, ensure you have the following prerequisites:
- Node.js installed on your machine.
- A valid access token obtained through the OAuth2 authentication process.
- Familiarity with JavaScript and asynchronous programming.
Installing Required Dependencies
To make HTTP requests in JavaScript, you can use the node-fetch
library. Install it using npm:
npm install node-fetch
Creating or Updating Contacts with Constant Contact API
To create or update contacts, you will need to make a POST request to the Constant Contact API. Here's a step-by-step guide with example code:
- Import the
node-fetch
library: - Define the API endpoint and headers:
- Create the contact data you want to send:
- Make the POST request to create or update the contact:
const fetch = require('node-fetch');
const apiUrl = 'https://api.cc.email/v3/contacts';
const headers = {
'Authorization': `Bearer ${accessToken}`,
'Content-Type': 'application/json'
};
const contactData = {
email_address: 'example@example.com',
first_name: 'John',
last_name: 'Doe',
create_source: 'Contact'
};
async function createOrUpdateContact() {
try {
const response = await fetch(apiUrl, {
method: 'POST',
headers: headers,
body: JSON.stringify(contactData)
});
if (response.ok) {
const data = await response.json();
console.log('Contact created or updated successfully:', data);
} else {
console.error('Failed to create or update contact:', response.status, response.statusText);
}
} catch (error) {
console.error('Error:', error);
}
}
createOrUpdateContact();
Handling API Response and Errors
After making the API call, you should handle the response to ensure the contact was created or updated successfully. Check the response status code to determine the outcome:
- 201 Created: The contact was successfully created.
- 200 OK: The contact was successfully updated.
- 400 Bad Request: There was an issue with the request, such as malformed JSON.
- 401 Unauthorized: The access token is invalid or expired.
- 429 Too Many Requests: You have exceeded the rate limit. Wait before retrying.
Refer to the Constant Contact API documentation for more details on error codes and handling.
By following these steps, you can efficiently create or update contacts in Constant Contact using JavaScript, ensuring your email lists remain accurate and up-to-date.
Conclusion and Best Practices for Using Constant Contact API with JavaScript
Integrating with the Constant Contact API using JavaScript allows developers to automate and enhance their email marketing processes. By following the steps outlined in this article, you can efficiently create or update contacts, ensuring your email lists are always current and accurate.
Best Practices for Secure and Efficient API Integration
- Securely Store Credentials: Always store your client ID, client secret, and access tokens securely. Avoid hardcoding them in your source code. Use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Be mindful of the API's rate limits, which are 10,000 requests per day and 4 requests per second. Implement retry logic with exponential backoff to handle 429 Too Many Requests errors gracefully.
- Data Standardization: Ensure that the data you send to the API is standardized and validated to prevent errors. This includes formatting email addresses and names correctly.
- Monitor API Usage: Regularly monitor your API usage and error logs to identify and resolve issues promptly. This will help maintain the reliability of your integration.
Enhancing Your Integration Experience with Endgrate
While integrating with Constant Contact API directly can be powerful, it can also be complex and time-consuming. Endgrate offers a streamlined solution by providing a unified API endpoint that connects to multiple platforms, including Constant Contact. This allows you to build integrations once and reuse them across different platforms, saving time and resources.
By leveraging Endgrate, you can focus on your core product while outsourcing the complexities of integration management. Explore how Endgrate can enhance your integration experience by visiting Endgrate.
Read More
- https://endgrate.com/provider/constantcontact
- https://developer.constantcontact.com/api_guide/v3_technical_overview.html
- https://developer.constantcontact.com/api_guide/auth_overview.html
- https://developer.constantcontact.com/api_guide/scopes.html
- https://developer.constantcontact.com/api_guide/glossary_responses.html
Ready to get started?