How to Get Contacts with the FreeAgent API in Python
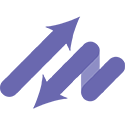
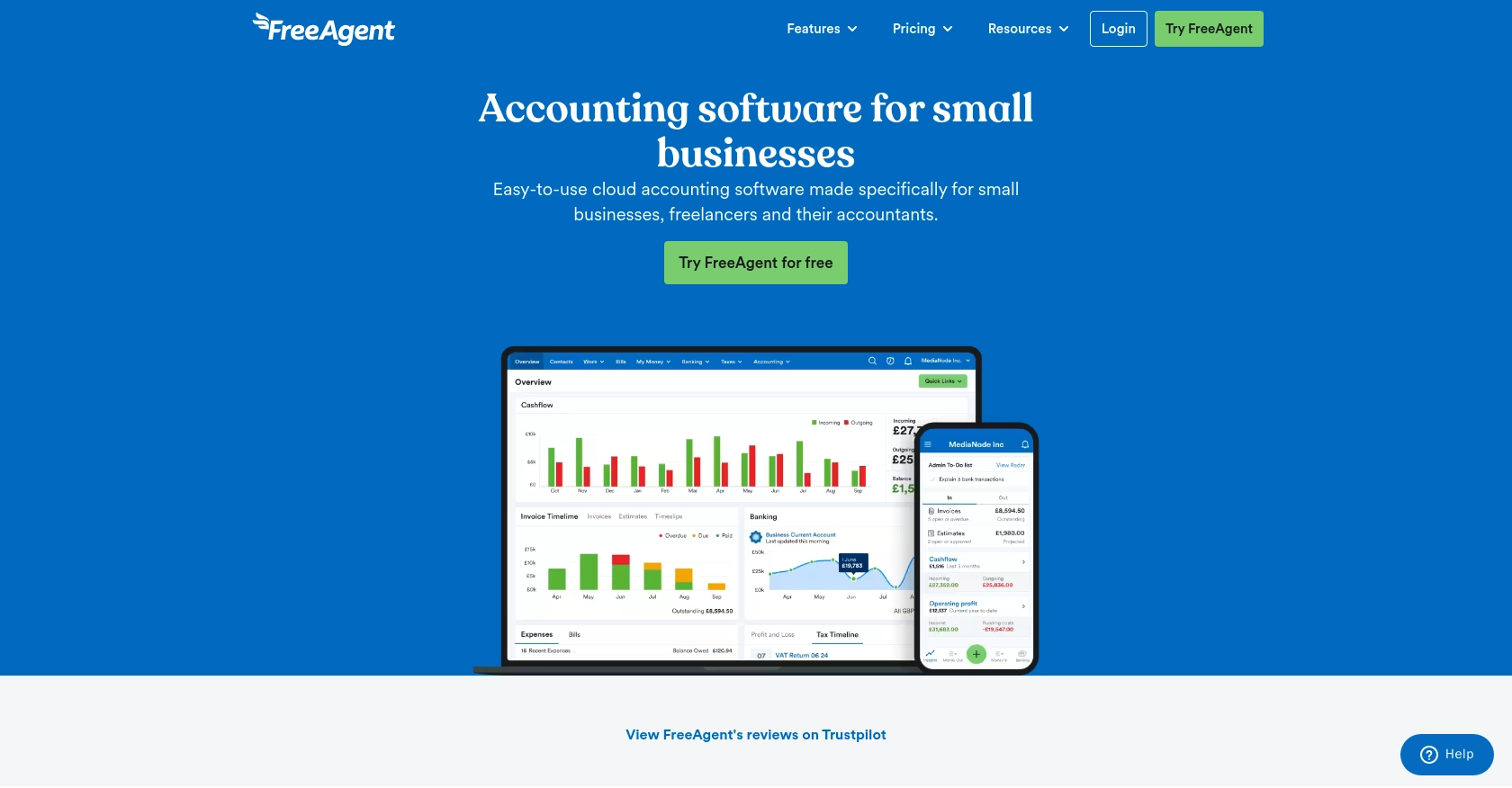
Introduction to FreeAgent API Integration
FreeAgent is a comprehensive accounting software designed to simplify financial management for small businesses and freelancers. It offers a wide range of features, including invoicing, expense tracking, and project management, all within an intuitive platform.
Developers may want to integrate with FreeAgent's API to streamline access to financial data and automate accounting tasks. For example, using the FreeAgent API, a developer can retrieve contact information to synchronize client data across multiple systems, ensuring consistency and reducing manual data entry.
Setting Up Your FreeAgent Sandbox Account for API Integration
Before you can start using the FreeAgent API, you need to set up a sandbox account. This allows you to test API interactions without affecting real data. Follow these steps to create your FreeAgent sandbox account and configure OAuth authentication.
Create a FreeAgent Sandbox Account
- Visit the FreeAgent Sandbox page.
- Sign up for a free temporary FreeAgent user account.
- Log in to your sandbox account and complete the setup stages to avoid unexpected errors when using the API.
Create an App in the FreeAgent Developer Dashboard
- Navigate to the FreeAgent Developer Dashboard.
- Create a new app and note down the OAuth Client ID and Client Secret.
Configure OAuth Authentication
To interact with the FreeAgent API, you need to obtain an Access Token using OAuth 2.0. Follow these steps:
- Go to the Google OAuth 2.0 Playground.
- Enter the OAuth Client ID and Client Secret from your FreeAgent app.
- Set the OAuth Authorization Endpoint to
https://api.sandbox.freeagent.com/v2/approve_app
. - Set the OAuth Token Endpoint to
https://api.sandbox.freeagent.com/v2/token_endpoint
. - Specify a scope name and click the "Authorize APIs" button.
- Log in to your FreeAgent sandbox account and approve the app.
- Click "Exchange Authorization Code for Tokens" to generate Access and Refresh Tokens.
With these tokens, you can now access the FreeAgent API for your sandbox account. You are ready to proceed with making API calls using Python.
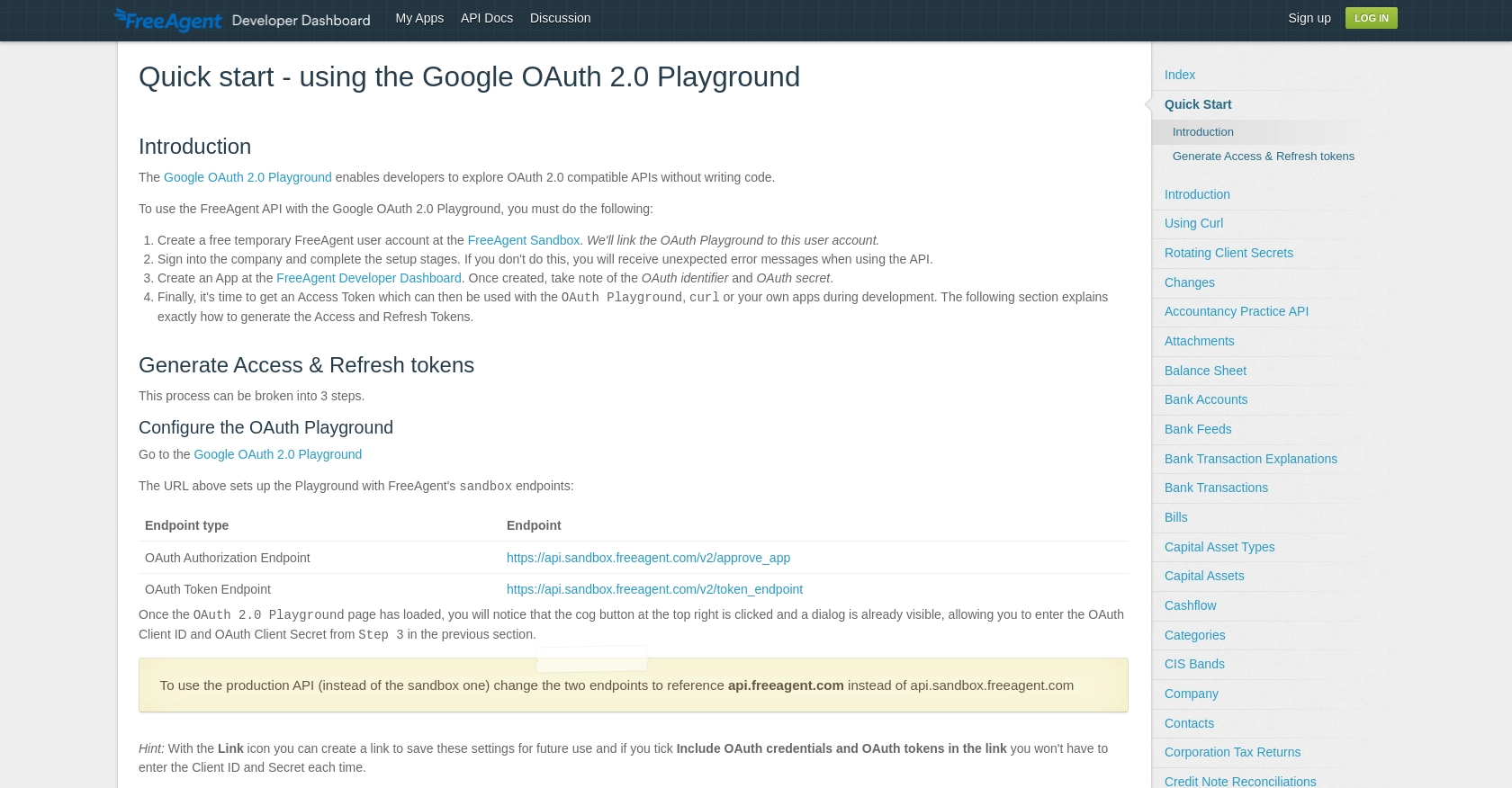
sbb-itb-96038d7
Making API Calls to Retrieve Contacts from FreeAgent Using Python
Now that you have set up your FreeAgent sandbox account and obtained the necessary OAuth tokens, you can proceed to make API calls to retrieve contact information. This section will guide you through the process of using Python to interact with the FreeAgent API.
Prerequisites for Using Python with FreeAgent API
Before you begin, ensure you have the following installed on your machine:
- Python 3.11.1 or later
- The Python package installer
pip
Once you have these installed, open your terminal or command prompt and install the requests
library using the following command:
pip install requests
Example Code to Fetch Contacts from FreeAgent API
Create a new Python file named get_freeagent_contacts.py
and add the following code:
import requests
# Set the API endpoint and headers
endpoint = "https://api.sandbox.freeagent.com/v2/contacts"
headers = {
"Authorization": "Bearer Your_Access_Token",
"Accept": "application/json"
}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
# Parse the JSON data from the response
contacts = response.json()["contacts"]
# Loop through the contacts and print their information
for contact in contacts:
print(f"Name: {contact.get('first_name', '')} {contact.get('last_name', '')}")
print(f"Email: {contact.get('email', 'N/A')}")
print(f"Organisation: {contact.get('organisation_name', 'N/A')}\n")
else:
print(f"Failed to retrieve contacts: {response.status_code} - {response.text}")
Replace Your_Access_Token
with the access token you obtained earlier. This script sends a GET request to the FreeAgent API to fetch contact information and prints out the details of each contact.
Running the Python Script and Verifying Results
Run the script from your terminal or command line using the following command:
python get_freeagent_contacts.py
If successful, you should see a list of contacts printed in your terminal. Verify the results by checking the contact information in your FreeAgent sandbox account.
Handling Errors and Understanding Response Codes
When making API calls, it's crucial to handle potential errors. The FreeAgent API may return various HTTP status codes, such as:
- 200 OK: The request was successful.
- 401 Unauthorized: Authentication failed. Check your access token.
- 429 Too Many Requests: Rate limit exceeded. Implement a back-off strategy.
For more details on handling errors, refer to the FreeAgent API documentation.
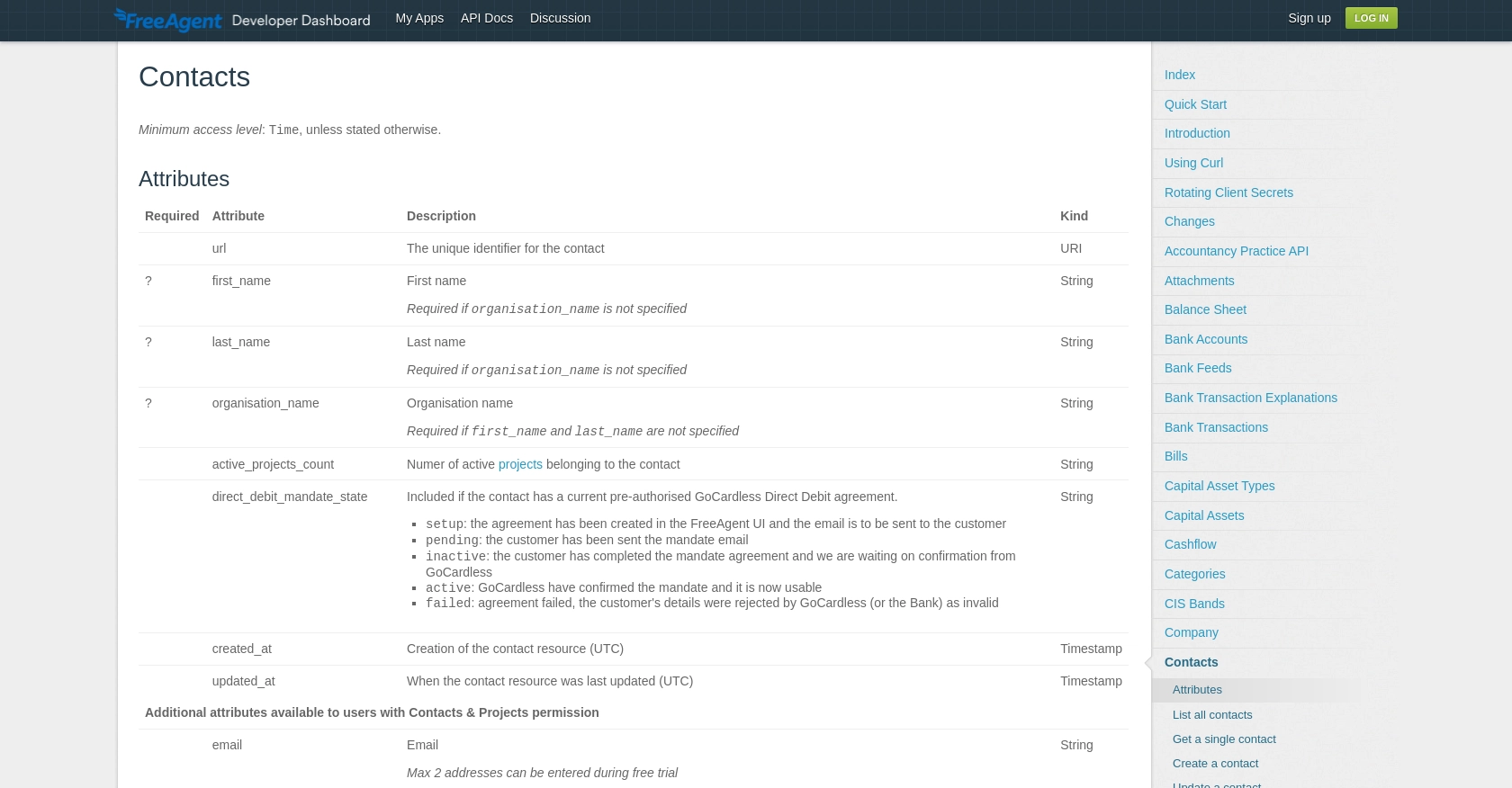
Conclusion and Best Practices for Integrating FreeAgent API with Python
Integrating with the FreeAgent API using Python can significantly streamline your accounting processes by automating data retrieval and synchronization. By following the steps outlined in this guide, you can efficiently access contact information and ensure data consistency across your systems.
Best Practices for Secure and Efficient FreeAgent API Integration
- Securely Store Credentials: Always store your OAuth tokens securely, using environment variables or a secure vault, to prevent unauthorized access.
- Implement Rate Limiting: Respect FreeAgent's rate limits of 120 requests per minute and 3600 per hour. Implement a back-off strategy to handle the 429 status code effectively. For more details, refer to the FreeAgent API documentation.
- Data Transformation: Standardize and transform data fields as needed to ensure compatibility with your existing systems.
Enhance Your Integration Strategy with Endgrate
While building integrations with FreeAgent and other platforms, consider leveraging Endgrate to simplify and accelerate your development process. Endgrate offers a unified API endpoint that connects to multiple platforms, allowing you to focus on your core product while outsourcing integration complexities.
By using Endgrate, you can build once for each use case instead of multiple times for different integrations, providing an easy and intuitive experience for your customers. Visit Endgrate to learn more about how it can enhance your integration strategy.
Read More
Ready to get started?