Using the Endear API to Get Tasks (with Python examples)
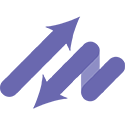
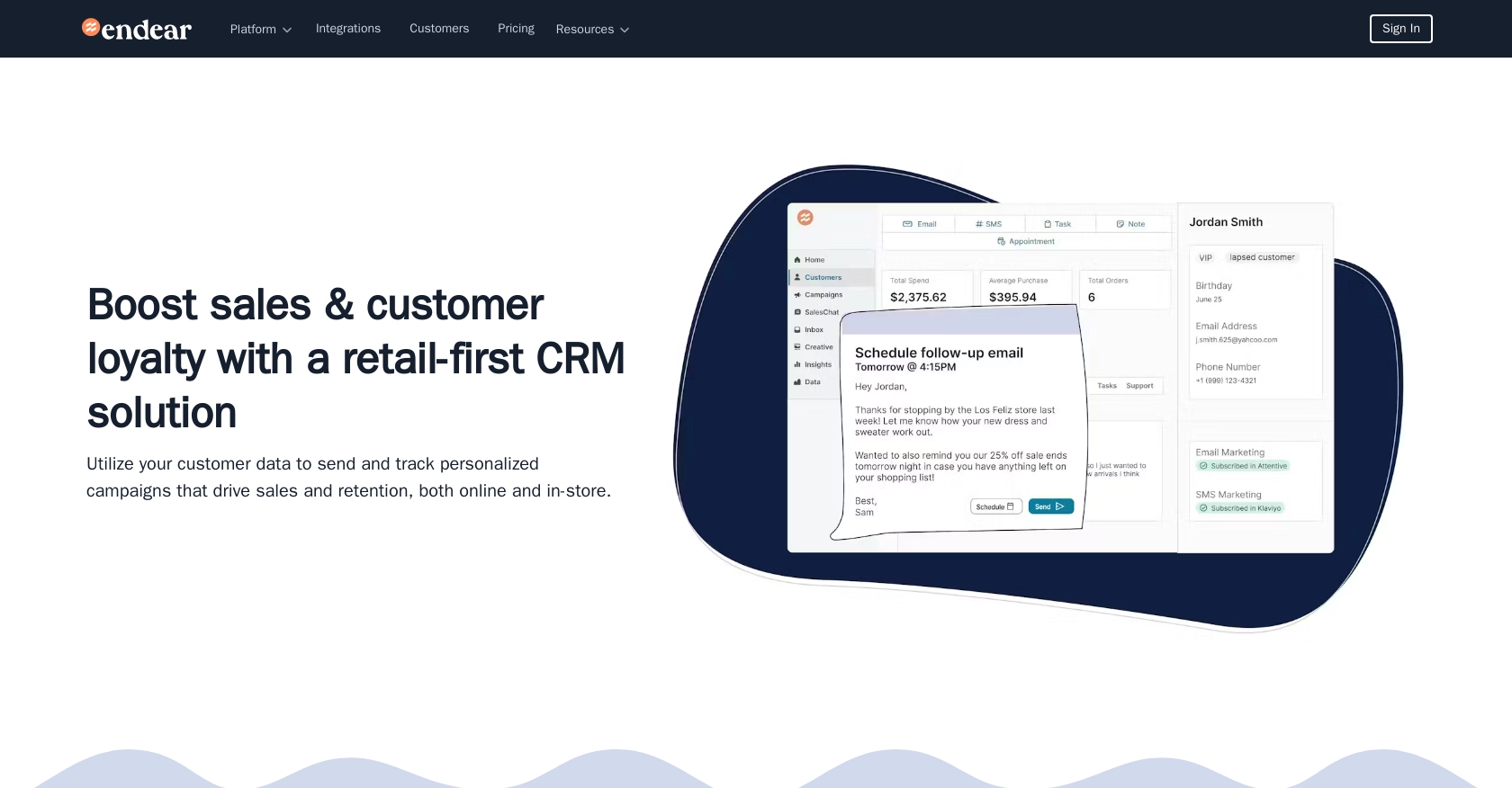
Introduction to Endear API Integration
Endear is a powerful CRM platform designed to enhance customer relationships through personalized communication and task management. It offers a robust suite of tools that help businesses streamline their customer engagement processes, making it a popular choice for companies looking to improve their customer interactions.
Developers may want to integrate with Endear's API to automate and manage tasks efficiently. For example, by using the Endear API, a developer can retrieve and organize tasks, allowing for seamless task management and improved productivity within their applications.
This article will guide you through using Python to interact with the Endear API, specifically focusing on retrieving tasks. You'll learn how to set up your environment, authenticate requests, and handle API responses effectively.
Setting Up Your Endear Test Account and API Key
Before you can start interacting with the Endear API, you'll need to set up a test account and generate an API key. This key will allow you to authenticate your requests and access the API endpoints securely.
Creating an Endear Account
If you don't already have an Endear account, you can sign up for a free trial or demo account on the Endear website. Follow the instructions to create your account and log in.
Generating an API Key for Endear Integration
Once your account is set up, you'll need to generate an API key to authenticate your API requests. Follow these steps to create an API key:
- Navigate to your Endear account settings.
- Click on Integrations in the menu.
- Select Add Integration and choose API from the options.
- Fill in the required details and submit the form to generate your API key.
Make sure to copy and store your API key securely, as you'll need it to authenticate your requests to the Endear API.
Authenticating API Requests with Your API Key
With your API key ready, you can now authenticate your requests to the Endear API. You'll need to include the API key in the headers of your requests. Here's an example of how to structure your request headers:
headers = {
"Content-Type": "application/json",
"X-Endear-Api-Key": "Your_API_Key"
}
Replace Your_API_Key
with the API key you generated earlier. This header will be used in all your API requests to ensure secure communication with the Endear platform.
For more detailed information on authentication, you can refer to the Endear Authentication Documentation.
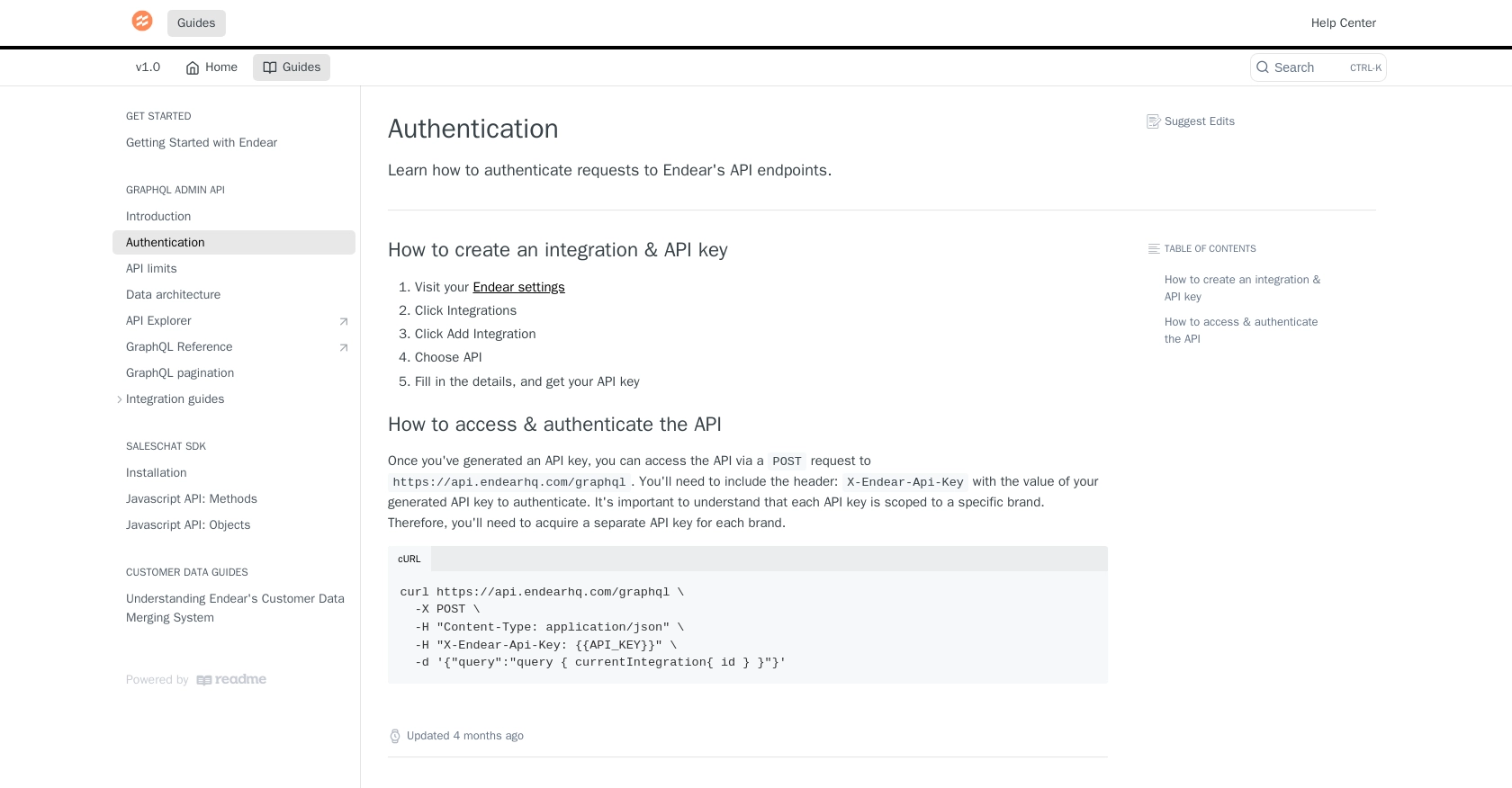
sbb-itb-96038d7
Making API Calls to Retrieve Tasks from Endear Using Python
To interact with the Endear API and retrieve tasks, you'll need to set up your Python environment and make HTTP requests to the API endpoint. This section will guide you through the necessary steps, including setting up your environment, writing the code to make API calls, and handling responses effectively.
Setting Up Your Python Environment for Endear API Integration
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests. Install it using the following command:
pip install requests
Writing Python Code to Retrieve Tasks from Endear API
With your environment set up, you can now write the Python code to interact with the Endear API. Create a file named get_endear_tasks.py
and add the following code:
import requests
# Define the API endpoint and headers
url = "https://api.endearhq.com/graphql"
headers = {
"Content-Type": "application/json",
"X-Endear-Api-Key": "Your_API_Key"
}
# Define the GraphQL query to retrieve tasks
query = """
query {
searchTasks {
edges {
node {
recurring
recurrence_rule
note {
title
description
}
deadline
timezone
id
}
}
pageInfo {
endCursor
hasNextPage
}
}
}
"""
# Make a POST request to the API
response = requests.post(url, headers=headers, json={"query": query})
# Check if the request was successful
if response.status_code == 200:
data = response.json()
# Loop through the tasks and print their details
for task in data["data"]["searchTasks"]["edges"]:
print(task["node"])
else:
print(f"Failed to retrieve tasks: {response.status_code}")
Replace Your_API_Key
with the API key you generated earlier. This script sends a POST request to the Endear API, retrieves tasks, and prints their details.
Running the Python Script and Verifying API Responses
Run the script from your terminal using the following command:
python get_endear_tasks.py
If successful, the script will output the tasks retrieved from the Endear API. Verify the results by checking the task details in your Endear account.
Handling Errors and Understanding Endear API Rate Limits
When making API calls, it's crucial to handle potential errors. The Endear API may return various HTTP status codes, such as 429 for rate limit errors. Endear's rate limit is 120 requests per minute. If you exceed this limit, you'll receive a 429 error. Implement error handling to manage these scenarios:
if response.status_code == 429:
print("Rate limit exceeded. Please try again later.")
elif response.status_code != 200:
print(f"Error: {response.status_code} - {response.text}")
For more information on rate limits and error codes, refer to the Endear Rate Limits and Error Codes Documentation.
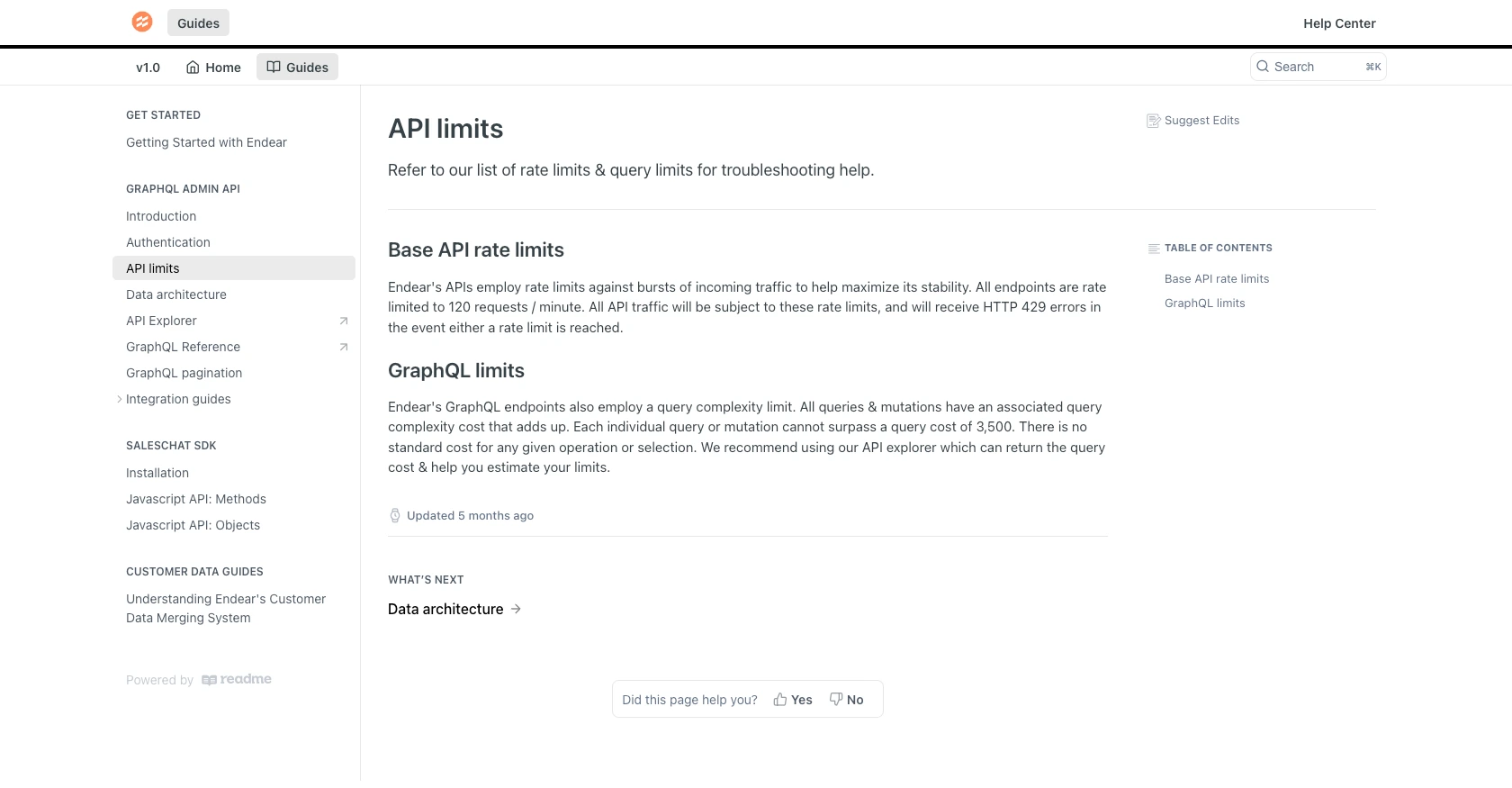
Best Practices for Using Endear API in Python
When integrating with the Endear API, it's essential to follow best practices to ensure secure and efficient interactions. Here are some recommendations:
- Securely Store API Keys: Always store your API keys securely and avoid hardcoding them in your source code. Consider using environment variables or a secure vault to manage sensitive information.
- Handle Rate Limits: Be mindful of Endear's rate limit of 120 requests per minute. Implement logic to handle HTTP 429 errors gracefully, such as retrying requests after a delay.
- Optimize Data Handling: When processing data from the API, ensure that you handle it efficiently. Consider using pagination if dealing with large datasets to avoid overwhelming your application.
- Standardize Data Fields: Transform and standardize data fields to match your application's requirements. This can help maintain consistency and improve data integration across platforms.
Leverage Endgrate for Seamless Endear API Integrations
While integrating with the Endear API can enhance your application's capabilities, managing multiple integrations can be time-consuming and complex. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Endear.
With Endgrate, you can:
- Save Time and Resources: Focus on your core product development while outsourcing integrations to Endgrate.
- Build Once, Use Everywhere: Develop a single integration for each use case and apply it across multiple platforms effortlessly.
- Enhance User Experience: Offer your customers a seamless and intuitive integration experience with minimal effort.
Explore how Endgrate can streamline your integration processes by visiting Endgrate today.
Read More
- https://endgrate.com/provider/endear
- https://docs.endearhq.com/docs/authentication
- https://docs.endearhq.com/docs/rate-limits-status-codes-and-errors
- https://docs.endearhq.com/docs/disclaimers
- https://developers.endearhq.com/docs/graphql/objects/Task
- https://docs.endearhq.com/docs/send-your-first-record
Ready to get started?