Using the Teamwork CRM API to Get Contacts in Javascript
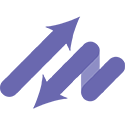
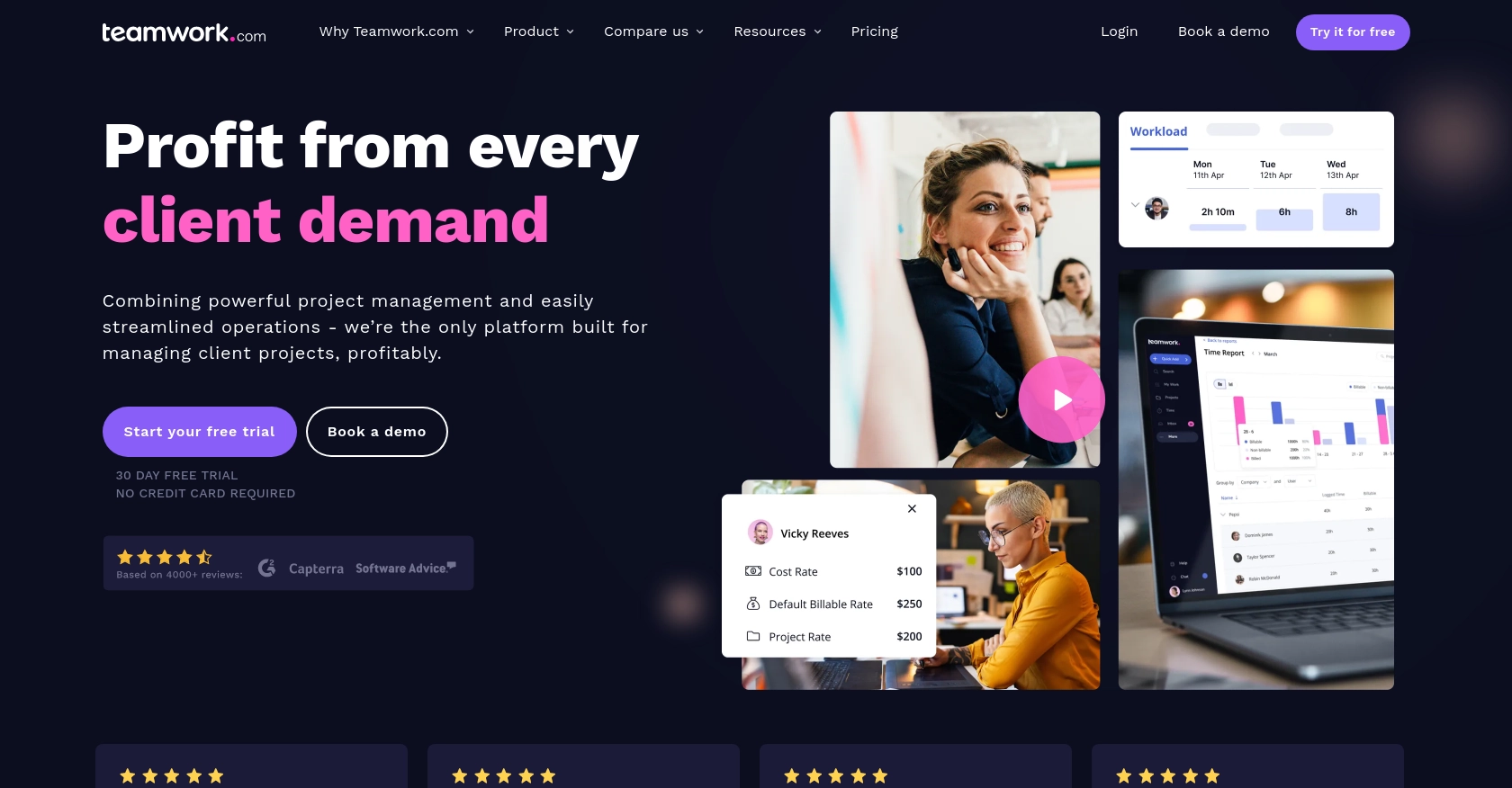
Introduction to Teamwork CRM API
Teamwork CRM is a powerful customer relationship management tool designed to help businesses manage their sales pipelines and customer interactions more effectively. It offers a comprehensive suite of features that streamline sales processes, enhance team collaboration, and improve customer engagement.
Integrating with the Teamwork CRM API allows developers to automate and enhance various CRM functionalities. For example, developers can use the API to retrieve contact information, enabling seamless integration of customer data into other applications or systems. This can be particularly useful for businesses looking to synchronize their sales data across multiple platforms, ensuring that all team members have access to up-to-date customer information.
Setting Up Your Teamwork CRM Test Account
Before you can start interacting with the Teamwork CRM API, you'll need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting live data. Follow these steps to get started:
Create a Teamwork CRM Account
- Visit the Teamwork CRM website and sign up for a free trial or demo account.
- Follow the on-screen instructions to complete the registration process.
- Once your account is created, log in to access the Teamwork CRM dashboard.
Generate API Credentials for Teamwork CRM
To authenticate your API requests, you'll need to generate API credentials. Teamwork CRM supports OAuth 2.0 for secure authentication. Here's how to set it up:
- Navigate to the Developer Portal within your Teamwork CRM account.
- Register a new application to obtain your client ID and client secret.
- Follow the OAuth 2.0 setup instructions to implement the App Login Flow.
- Once configured, you'll receive a bearer token to include in your API requests.
For more details on authentication, refer to the Teamwork CRM Authentication Guide.
Understanding Teamwork CRM API Authentication
Teamwork CRM offers two main authentication methods: Basic Authentication and OAuth 2.0. For enhanced security, OAuth 2.0 is recommended. Here's a brief overview:
- Basic Authentication: Use a base64-encoded string of your API key or username and password. This method is less secure and should be used with HTTPS.
- OAuth 2.0: Register your app to generate a client ID and secret. Use these to obtain a bearer token for API requests.
Ensure you include the token in the Authorization header of your requests, formatted as Authorization: Bearer {token}
.
With your Teamwork CRM test account and API credentials set up, you're ready to start making API calls to retrieve contact information and more.
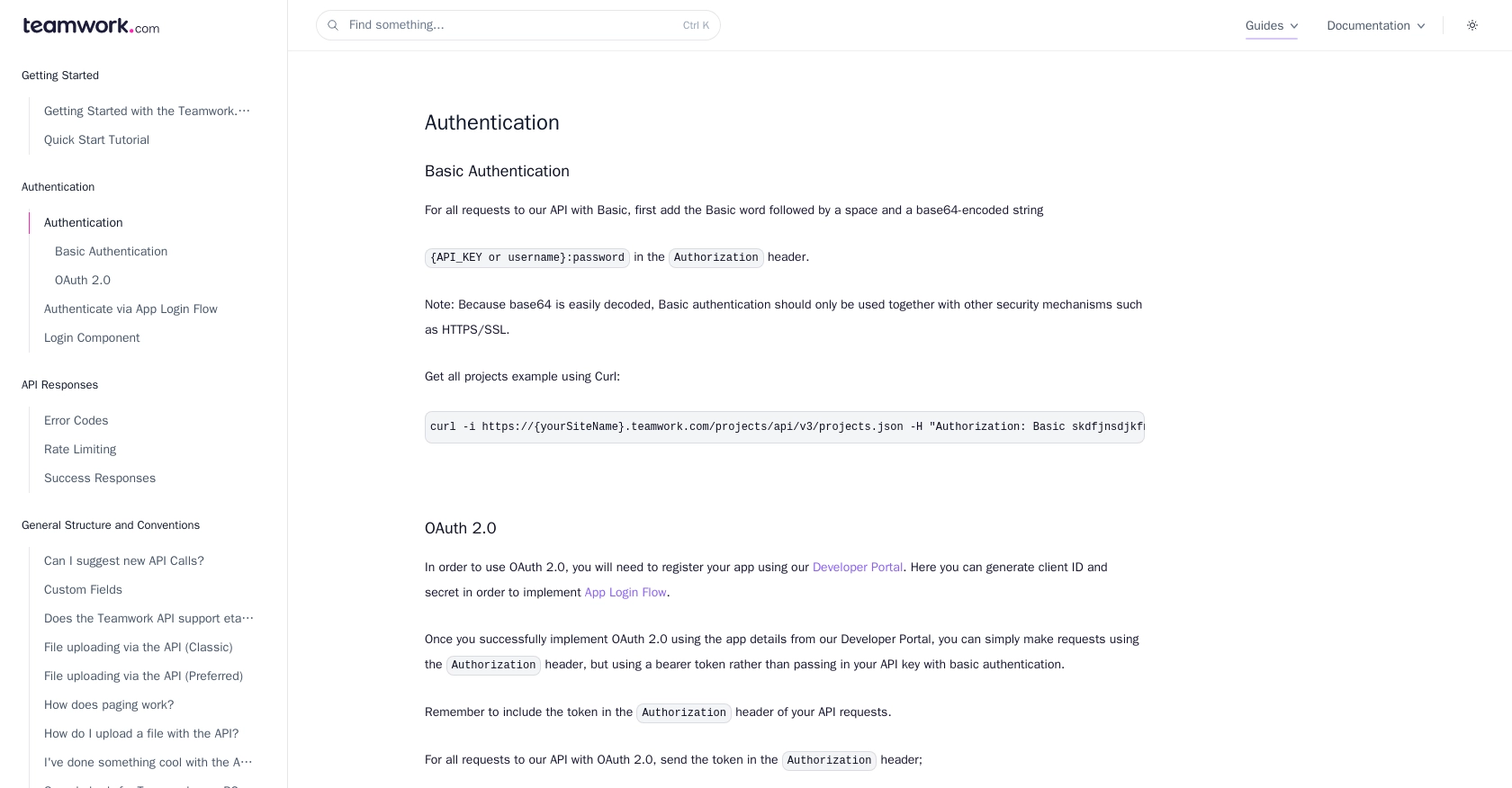
sbb-itb-96038d7
Making API Calls to Retrieve Contacts from Teamwork CRM Using JavaScript
To interact with the Teamwork CRM API and retrieve contact information, you'll need to use JavaScript to make HTTP requests. This section will guide you through the process of setting up your environment, writing the code, and handling responses effectively.
Setting Up Your JavaScript Environment for Teamwork CRM API
Before you begin, ensure you have the following prerequisites:
- A modern web browser with developer tools enabled.
- A text editor or integrated development environment (IDE) for writing JavaScript code.
- Access to your Teamwork CRM API credentials, including the bearer token obtained during the OAuth 2.0 setup.
Writing JavaScript Code to Fetch Contacts from Teamwork CRM
Once your environment is ready, you can write the JavaScript code to make an API call to Teamwork CRM. The following example demonstrates how to retrieve contacts:
// Define the API endpoint and headers
const endpoint = 'https://{yourSiteName}.teamwork.com/crm/v2/contacts';
const headers = {
'Authorization': 'Bearer Your_Token',
'Content-Type': 'application/json'
};
// Function to fetch contacts
async function fetchContacts() {
try {
const response = await fetch(endpoint, { headers });
if (!response.ok) {
throw new Error(`HTTP error! Status: ${response.status}`);
}
const data = await response.json();
console.log('Contacts:', data);
} catch (error) {
console.error('Error fetching contacts:', error);
}
}
// Call the function to fetch contacts
fetchContacts();
Replace Your_Token
with the bearer token you obtained from the Teamwork CRM Developer Portal.
Understanding the JavaScript Code for Teamwork CRM API
In the code above, we define the API endpoint for retrieving contacts and set up the necessary headers, including the authorization token. The fetchContacts
function uses the Fetch API to make an asynchronous request to the Teamwork CRM API.
We handle the response by checking if it was successful and parsing the JSON data. If an error occurs, it is caught and logged to the console.
Verifying Successful API Requests and Handling Errors
To verify that your request was successful, check the console output for the list of contacts. If the request fails, the error message will provide insight into what went wrong. Common error codes include:
- 401 Unauthorized: Check your API token and ensure it is correctly set up.
- 404 Not Found: Verify the API endpoint URL.
- 429 Rate Limit Exceeded: Teamwork CRM enforces a rate limit of 150 requests per minute. If you exceed this, you will receive a 429 error. Refer to the Teamwork CRM Rate Limiting Guide for more details.
By following these steps, you can effectively retrieve contact information from Teamwork CRM using JavaScript, enabling seamless integration with your applications.
Conclusion and Best Practices for Using Teamwork CRM API with JavaScript
Integrating with the Teamwork CRM API using JavaScript provides a robust solution for automating CRM functionalities and synchronizing customer data across platforms. By following the steps outlined in this guide, developers can efficiently retrieve and manage contact information, enhancing their applications' capabilities.
Best Practices for Secure and Efficient API Integration with Teamwork CRM
- Secure Storage of Credentials: Always store your API credentials securely. Avoid hardcoding them in your source code. Use environment variables or secure vaults to manage sensitive information.
- Handling Rate Limits: Be mindful of Teamwork CRM's rate limit of 150 requests per minute. Implement logic to handle HTTP 429 errors gracefully and consider using exponential backoff strategies to retry requests.
- Data Transformation and Standardization: Ensure that the data retrieved from Teamwork CRM is transformed and standardized to fit your application's data model. This will help maintain consistency across different systems.
Leverage Endgrate for Streamlined Integration Solutions
While integrating with Teamwork CRM API can be straightforward, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process, allowing developers to focus on core product development. By using Endgrate, you can build once for each use case and seamlessly connect with various platforms, including Teamwork CRM.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate's website and discover how it can save you time and resources.
Read More
Ready to get started?