How to Create Calendar Events with the Google Calendar API in PHP
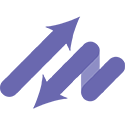
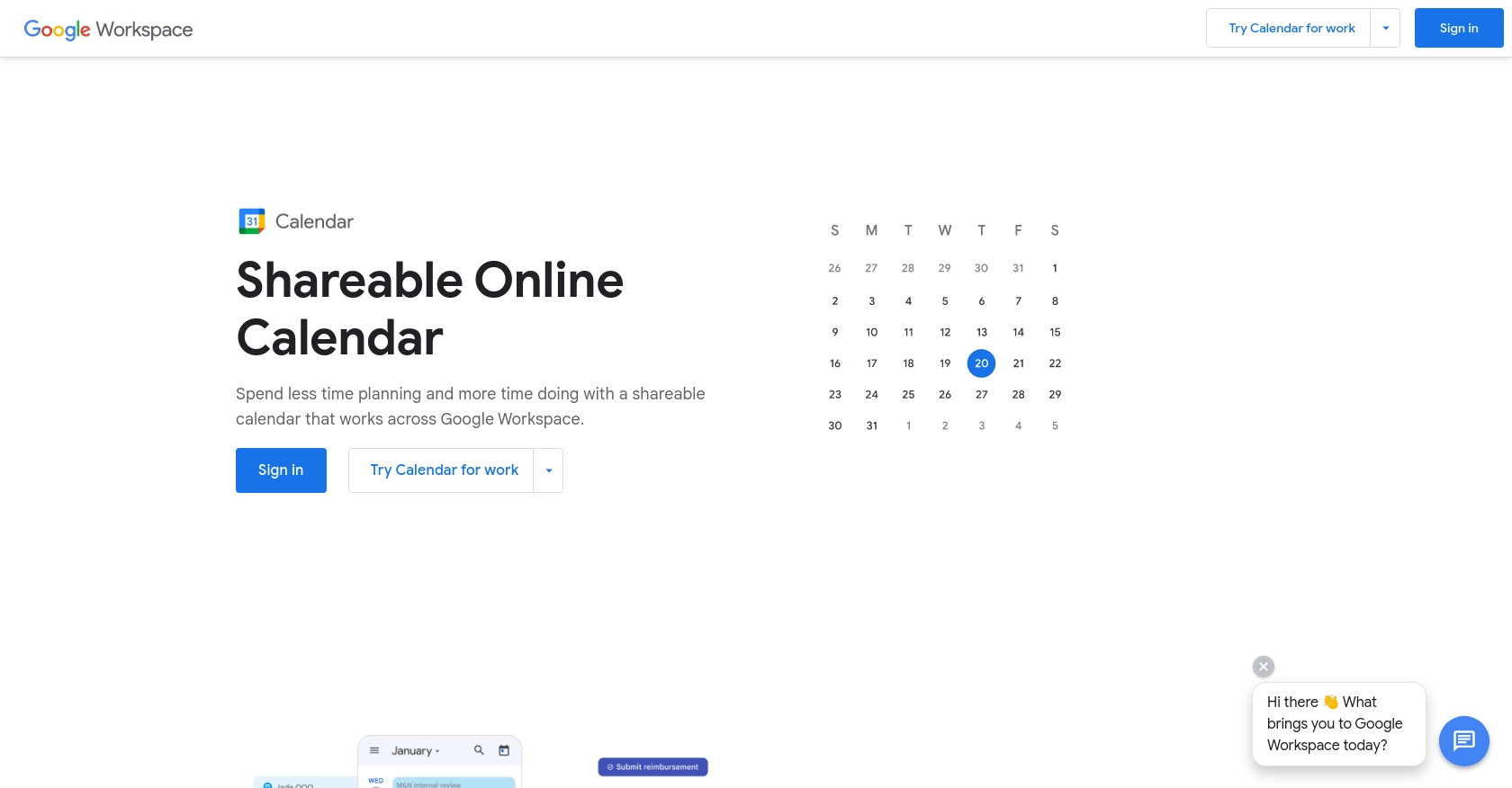
Introduction to Google Calendar API
Google Calendar is a widely used time-management and scheduling tool that allows users to create and edit events. It integrates seamlessly with other Google services, providing a comprehensive solution for organizing personal and professional schedules.
Developers often integrate with the Google Calendar API to automate event management, such as creating, updating, or deleting events programmatically. For example, a developer might use the API to automatically schedule meetings based on user availability, ensuring efficient time management and reducing manual scheduling tasks.
Setting Up Your Google Calendar API Test Environment
Before you can start creating calendar events using the Google Calendar API in PHP, you need to set up a Google Cloud project and configure OAuth 2.0 authentication. This setup will allow you to access the API securely and manage calendar events programmatically.
Create a Google Cloud Project
- Go to the Google Cloud Console.
- Click on the Menu icon, then navigate to IAM & Admin > Create a Project.
- Enter a descriptive name for your project and click Create.
Enable Google Calendar API
- In the Google Cloud Console, navigate to APIs & Services > Library.
- Search for "Google Calendar API" and click on it.
- Click Enable to activate the API for your project.
Configure OAuth Consent Screen
- Go to APIs & Services > OAuth consent screen.
- Select the user type for your app and click Create.
- Fill in the required fields, such as app name and support email, then click Save and Continue.
Create OAuth 2.0 Credentials
- Navigate to APIs & Services > Credentials.
- Click on Create Credentials and select OAuth client ID.
- Choose Web application as the application type.
- Enter a name for your client ID and specify the authorized redirect URIs.
- Click Create to generate your client ID and client secret.
Make sure to securely store your client ID and client secret, as you will need them to authenticate API requests.
For more detailed instructions, refer to the official Google documentation on creating a Google Cloud project and configuring OAuth consent.
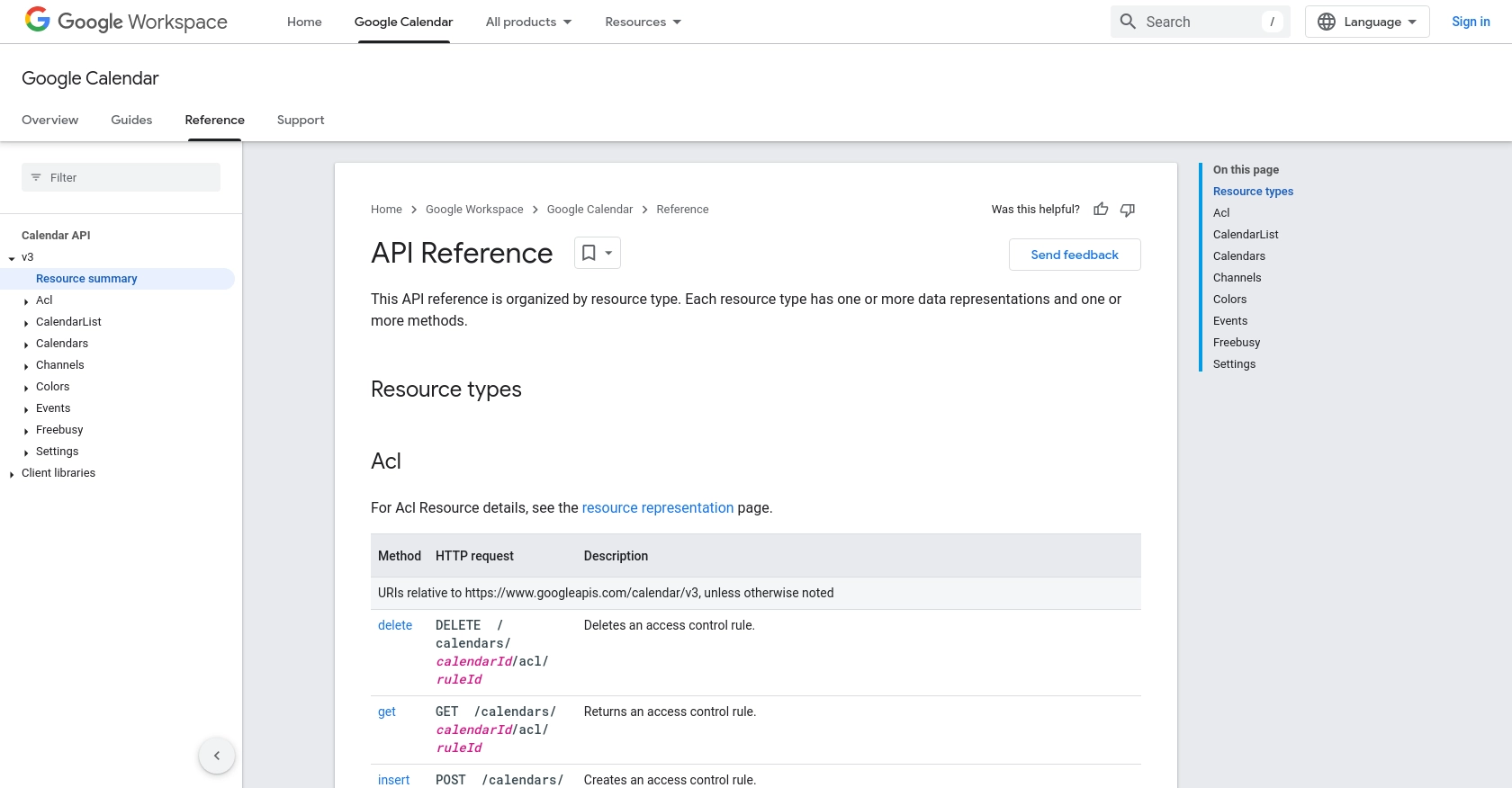
sbb-itb-96038d7
Making API Calls to Google Calendar with PHP
To interact with the Google Calendar API using PHP, you'll need to set up your environment and write code to create calendar events. This section will guide you through the necessary steps, including setting up PHP, installing dependencies, and writing the code to make API calls.
Setting Up PHP Environment for Google Calendar API
Ensure you have PHP installed on your machine. You can download it from the official PHP website. Additionally, you'll need Composer, a dependency manager for PHP, to install the required libraries.
- Install Composer by following the instructions on the Composer download page.
- Open your terminal and navigate to your project directory.
- Run the following command to install the Google Client Library for PHP:
composer require google/apiclient:^2.0
Creating a Calendar Event with Google Calendar API in PHP
Once your environment is set up, you can proceed to write the PHP code to create a calendar event. The following example demonstrates how to use the Google Calendar API to insert an event into a calendar.
require 'vendor/autoload.php';
session_start();
// Set up the Google Client
$client = new Google_Client();
$client->setAuthConfig('path/to/your/client_credentials.json');
$client->addScope(Google_Service_Calendar::CALENDAR);
// Redirect to Google's OAuth 2.0 server
if (!isset($_SESSION['access_token']) && !isset($_GET['code'])) {
$authUrl = $client->createAuthUrl();
header('Location: ' . filter_var($authUrl, FILTER_SANITIZE_URL));
exit();
}
// Exchange authorization code for an access token
if (isset($_GET['code'])) {
$token = $client->fetchAccessTokenWithAuthCode($_GET['code']);
$_SESSION['access_token'] = $token;
}
// Set the access token
$client->setAccessToken($_SESSION['access_token']);
// Create a new Google Calendar service
$service = new Google_Service_Calendar($client);
// Define the event details
$event = new Google_Service_Calendar_Event(array(
'summary' => 'Meeting with Client',
'location' => '123 Business St, Business City, BC',
'description' => 'Discuss project requirements and timelines.',
'start' => array(
'dateTime' => '2023-10-10T10:00:00-07:00',
'timeZone' => 'America/Los_Angeles',
),
'end' => array(
'dateTime' => '2023-10-10T11:00:00-07:00',
'timeZone' => 'America/Los_Angeles',
),
'attendees' => array(
array('email' => 'client@example.com'),
),
'reminders' => array(
'useDefault' => FALSE,
'overrides' => array(
array('method' => 'email', 'minutes' => 24 * 60),
array('method' => 'popup', 'minutes' => 10),
),
),
));
// Insert the event into the calendar
$calendarId = 'primary';
$event = $service->events->insert($calendarId, $event);
printf('Event created: %s\n', $event->htmlLink);
Verifying API Call Success and Handling Errors
After running the script, check your Google Calendar to verify that the event has been created. If the event appears, the API call was successful. If not, ensure that your OAuth credentials are correct and that you have the necessary permissions.
To handle errors, you can use try-catch blocks around your API calls. The Google Client Library will throw exceptions for any errors encountered during the request.
try {
$event = $service->events->insert($calendarId, $event);
printf('Event created: %s\n', $event->htmlLink);
} catch (Exception $e) {
echo 'Error: ' . $e->getMessage();
}
For more information on error codes and handling, refer to the Google Calendar API documentation.
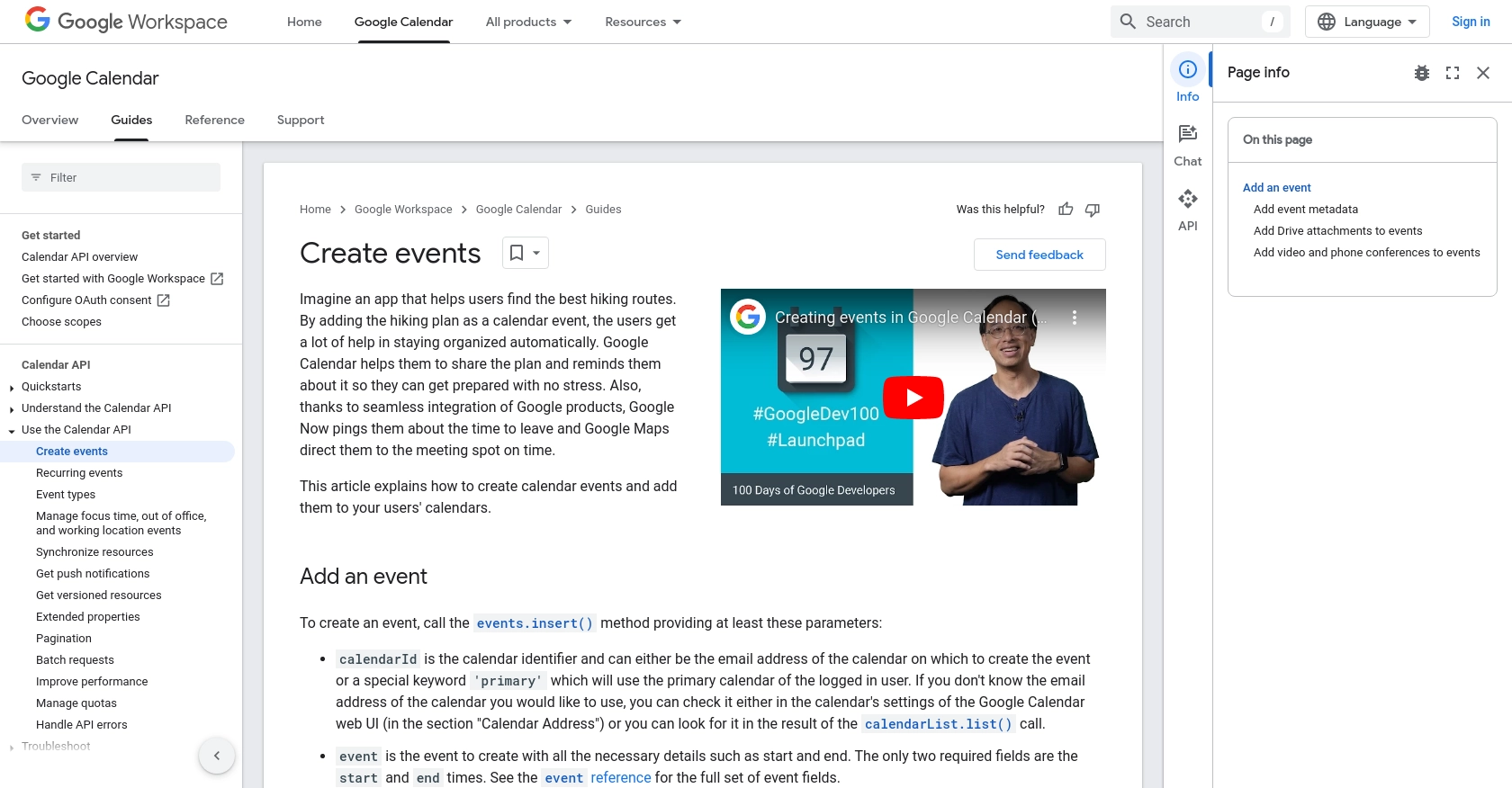
Conclusion and Best Practices for Using Google Calendar API in PHP
Integrating with the Google Calendar API in PHP offers developers a powerful way to automate and manage calendar events programmatically. By following the steps outlined in this guide, you can efficiently create, update, and manage events, enhancing productivity and ensuring seamless scheduling for users.
Best Practices for Secure and Efficient Google Calendar API Integration
- Securely Store Credentials: Always store your OAuth client ID and client secret securely. Consider using environment variables or secure vaults to protect sensitive information.
- Handle Rate Limiting: Be aware of Google Calendar API's rate limits. Implement exponential backoff strategies to handle rate limit errors gracefully and ensure your application remains responsive.
- Validate User Permissions: Ensure that your application requests only the necessary permissions and scopes to maintain user trust and comply with privacy standards.
- Standardize Data Formats: Consistently format date and time fields to match the user's locale and time zone settings, providing a seamless experience across different regions.
Enhance Your Integration Strategy with Endgrate
While integrating with Google Calendar API directly offers flexibility, managing multiple integrations can become complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Google Calendar.
By leveraging Endgrate, you can streamline your integration efforts, reduce development time, and focus on your core product. Whether you're building once for multiple use cases or providing an intuitive integration experience for your customers, Endgrate can help you achieve your goals efficiently.
Explore how Endgrate can transform your integration strategy by visiting Endgrate's website and discover the benefits of a unified integration solution.
Read More
- https://endgrate.com/provider/googlecalendar
- https://developers.google.com/calendar/api/v3/reference
- https://developers.google.com/workspace/guides/create-project
- https://developers.google.com/workspace/guides/enable-apis
- https://developers.google.com/workspace/guides/configure-oauth-consent
- https://developers.google.com/workspace/guides/create-credentials
- https://developers.google.com/calendar/api/guides/create-events
Ready to get started?