Using the Zoho CRM API to Get Custom Objects (with PHP examples)
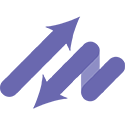
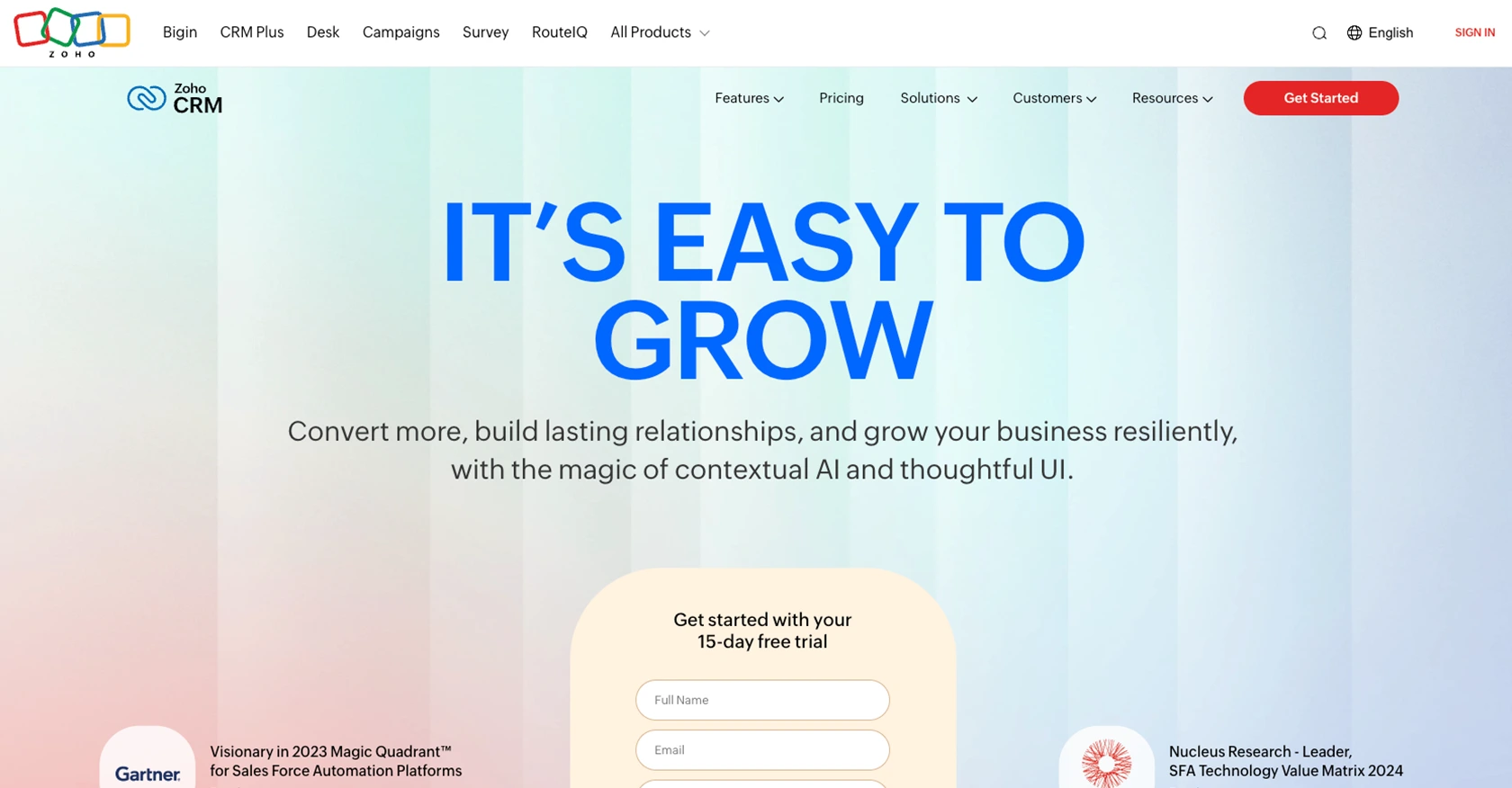
Introduction to Zoho CRM API Integration
Zoho CRM is a comprehensive customer relationship management platform that empowers businesses to manage their sales, marketing, and support in a unified manner. Known for its flexibility and extensive features, Zoho CRM is a popular choice for businesses looking to enhance their customer interactions and streamline operations.
Developers often seek to integrate with Zoho CRM to access and manipulate customer data, such as custom objects, to tailor solutions that meet specific business needs. For example, a developer might use the Zoho CRM API to retrieve custom object data and integrate it with other business applications, enabling seamless data flow and enhanced functionality.
This article will guide you through using PHP to interact with Zoho CRM's API, specifically focusing on retrieving custom objects. By following this tutorial, you will learn how to efficiently access and manage custom data within the Zoho CRM platform using PHP.
Setting Up Your Zoho CRM Test/Sandbox Account
Before diving into the Zoho CRM API integration, it's essential to set up a test or sandbox account. This environment allows you to experiment with API calls without affecting your live data, ensuring a safe and controlled testing space.
Creating a Zoho CRM Account
- Visit the Zoho CRM website and sign up for a free trial or demo account.
- Follow the on-screen instructions to complete the registration process. Once registered, log in to your Zoho CRM account.
Registering a Client for OAuth Authentication
Zoho CRM uses OAuth 2.0 for authentication, which requires registering your application to obtain the necessary credentials.
- Navigate to the Zoho Developer Console.
- Select the appropriate client type for your application (e.g., Web Based, Self Client).
- Enter the required details:
- Client Name: Name of your application.
- Homepage URL: The URL of your application.
- Authorized Redirect URIs: A valid URL where Zoho will redirect with a grant token after authentication.
- Click Create to register your application.
Upon successful registration, you will receive a Client ID and Client Secret. Keep these credentials secure as they are essential for making authorized API requests.
Generating Access and Refresh Tokens
With your client registered, you can now generate access and refresh tokens to authenticate API requests.
- Direct the user to Zoho's authorization URL, including your client ID and redirect URI.
- Upon user consent, Zoho will redirect to your specified URI with an authorization code.
- Exchange this authorization code for access and refresh tokens by making a POST request to Zoho's token endpoint.
// Example PHP code to exchange authorization code for tokens
$client_id = 'YOUR_CLIENT_ID';
$client_secret = 'YOUR_CLIENT_SECRET';
$redirect_uri = 'YOUR_REDIRECT_URI';
$authorization_code = 'AUTHORIZATION_CODE_RECEIVED';
$url = 'https://accounts.zoho.com/oauth/v2/token';
$data = [
'grant_type' => 'authorization_code',
'client_id' => $client_id,
'client_secret' => $client_secret,
'redirect_uri' => $redirect_uri,
'code' => $authorization_code
];
$options = [
'http' => [
'header' => "Content-type: application/x-www-form-urlencoded\r\n",
'method' => 'POST',
'content' => http_build_query($data),
],
];
$context = stream_context_create($options);
$result = file_get_contents($url, false, $context);
$response = json_decode($result, true);
$access_token = $response['access_token'];
$refresh_token = $response['refresh_token'];
Store the access and refresh tokens securely, as they are required for authenticating API requests. The access token is valid for a limited time, while the refresh token can be used to obtain new access tokens.
For more detailed information, refer to the official Zoho CRM OAuth documentation: OAuth Overview.
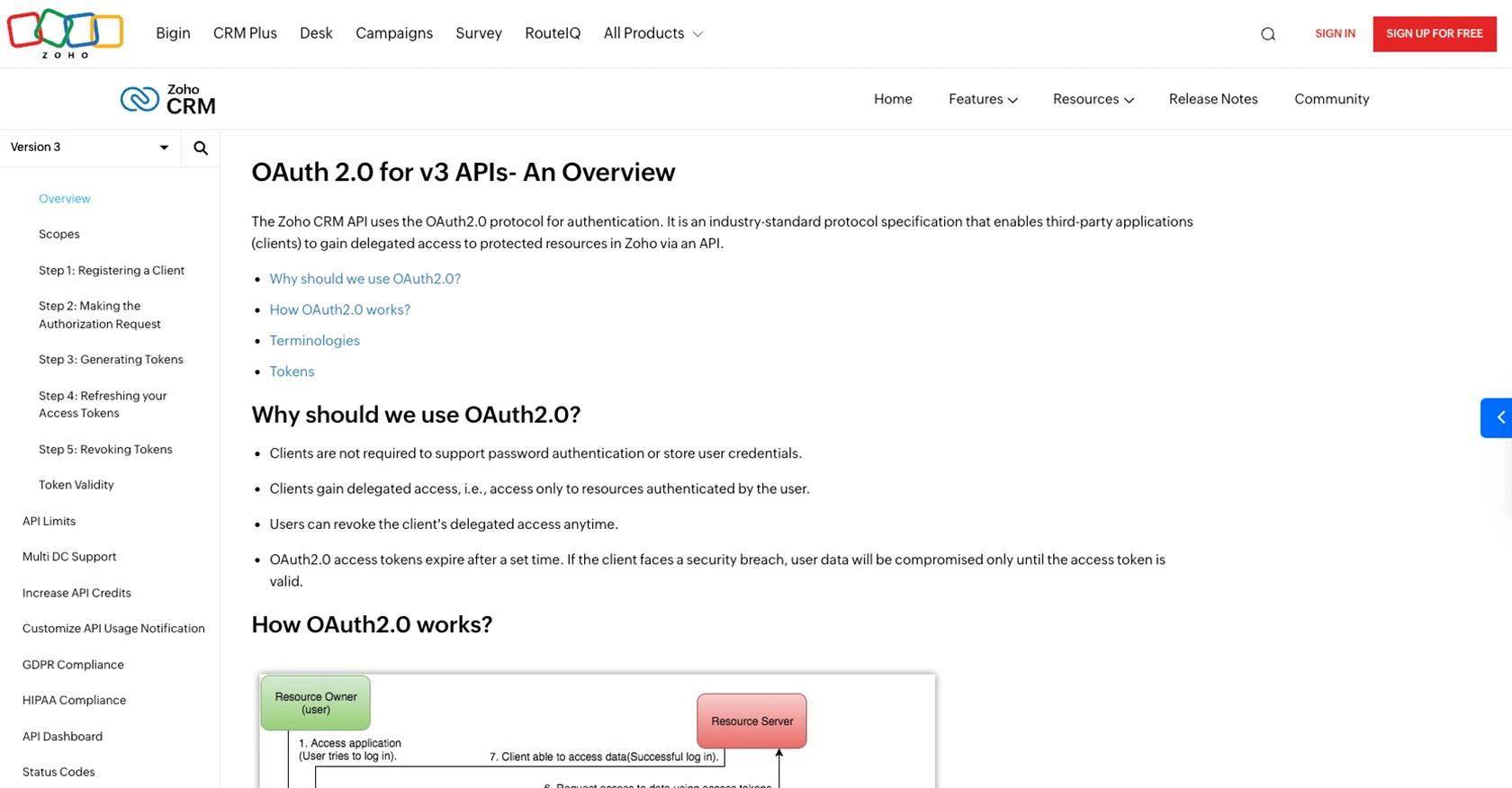
sbb-itb-96038d7
Making API Calls to Retrieve Custom Objects from Zoho CRM Using PHP
Once you have set up your Zoho CRM account and obtained the necessary authentication tokens, you can proceed to make API calls to retrieve custom objects. This section will guide you through the process of using PHP to interact with the Zoho CRM API and access custom object data.
Prerequisites for Zoho CRM API Integration with PHP
Before making API calls, ensure you have the following prerequisites:
- PHP 7.4 or higher installed on your machine.
- Composer for managing PHP dependencies.
- Access and refresh tokens obtained from the OAuth authentication process.
Install the necessary PHP library for making HTTP requests:
composer require guzzlehttp/guzzle
Retrieving Custom Objects from Zoho CRM
To retrieve custom objects from Zoho CRM, you need to make a GET request to the appropriate API endpoint. The endpoint URL format is https://www.zohoapis.com/crm/v3/MODULE_HERE
, where MODULE_HERE
is the API name of your custom object module.
// Example PHP code to retrieve custom objects from Zoho CRM
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$accessToken = 'YOUR_ACCESS_TOKEN';
$moduleName = 'CustomModule'; // Replace with your custom module API name
$response = $client->request('GET', "https://www.zohoapis.com/crm/v3/$moduleName", [
'headers' => [
'Authorization' => "Zoho-oauthtoken $accessToken",
'Content-Type' => 'application/json'
]
]);
$data = json_decode($response->getBody(), true);
foreach ($data['data'] as $record) {
echo "Record ID: " . $record['id'] . "\n";
// Access other fields as needed
}
Verifying API Call Success and Handling Errors
After executing the API call, verify the success of the request by checking the response status code. A successful request will return a status code of 200. If the request fails, handle errors appropriately by checking the status code and response message.
// Error handling example
if ($response->getStatusCode() === 200) {
echo "API call successful!";
} else {
echo "Error: " . $response->getReasonPhrase();
}
For more information on error codes and handling, refer to the official Zoho CRM API documentation: Status Codes.
Checking Retrieved Data in Zoho CRM Sandbox
To ensure the data retrieved matches the records in your Zoho CRM sandbox, log in to your Zoho CRM account and navigate to the custom module. Verify that the records displayed in the CRM match the data returned by your API call.
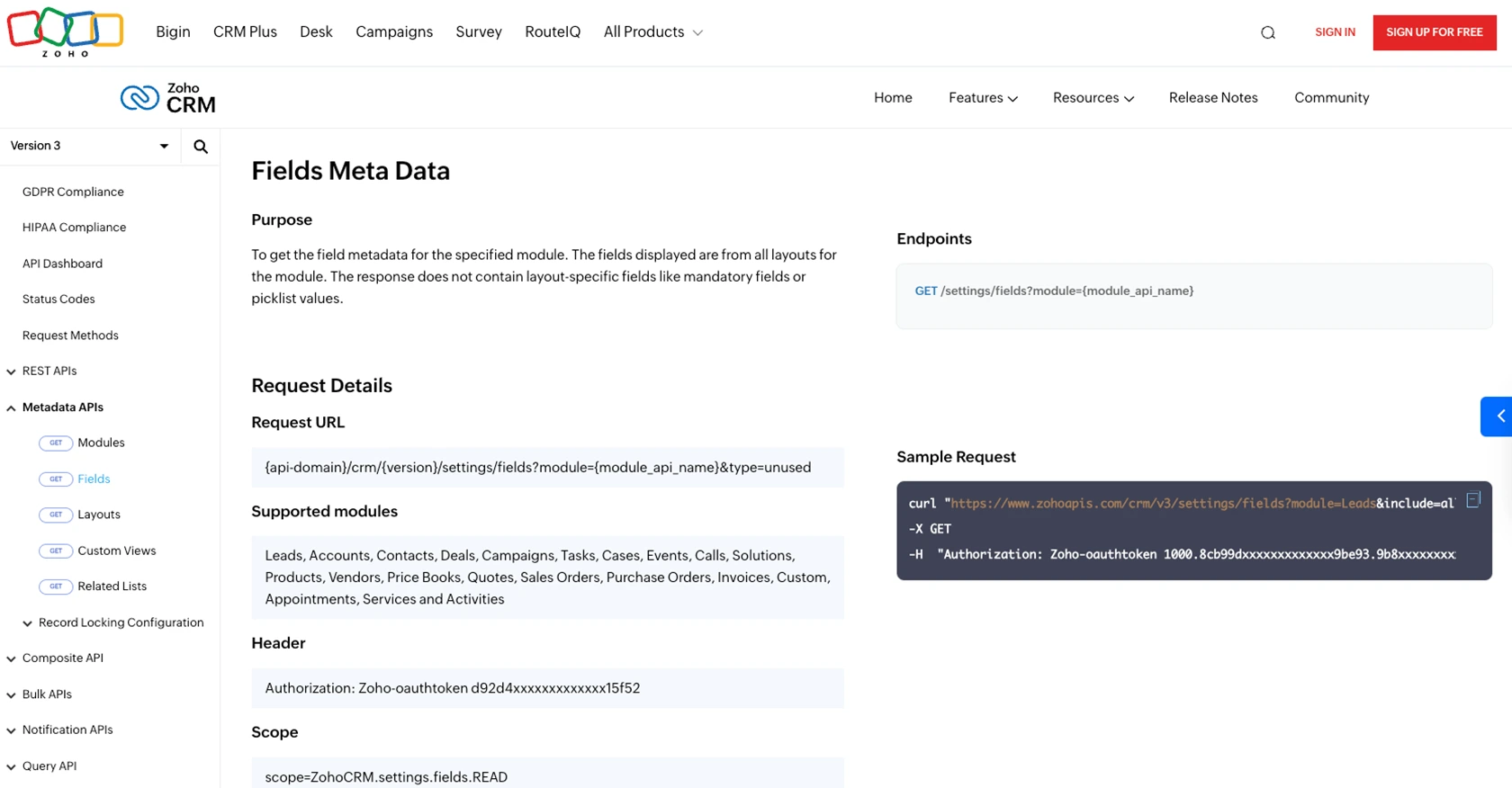
Conclusion and Best Practices for Zoho CRM API Integration Using PHP
Integrating with the Zoho CRM API to retrieve custom objects using PHP can significantly enhance your application's capabilities by providing seamless access to customer data. By following the steps outlined in this guide, you can efficiently set up authentication, make API calls, and handle responses to manage custom objects within Zoho CRM.
Best Practices for Secure and Efficient Zoho CRM API Integration
- Secure Token Storage: Always store your access and refresh tokens securely, using environment variables or secure vaults, to prevent unauthorized access.
- Handle Rate Limits: Be mindful of Zoho CRM's API rate limits. Implement retry logic and exponential backoff strategies to handle rate limit errors gracefully. For more details, refer to the API Limits documentation.
- Data Transformation: Standardize and transform data fields as needed to ensure consistency across different systems and applications.
- Error Handling: Implement robust error handling to manage different HTTP status codes and response messages effectively. This ensures your application can recover from API errors smoothly.
Streamline Your Integration Process with Endgrate
While integrating with Zoho CRM's API can be highly beneficial, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process, allowing you to focus on your core product development. With Endgrate, you can build once for each use case and leverage an intuitive integration experience for your customers.
Explore how Endgrate can help streamline your integration efforts by visiting Endgrate's website and discover the benefits of outsourcing your integration needs.
Read More
- https://endgrate.com/provider/zohocrm
- https://www.zoho.com/crm/developer/docs/api/v3/oauth-overview.html
- https://www.zoho.com/crm/developer/docs/api/v3/scopes.html
- https://www.zoho.com/crm/developer/docs/api/v3/register-client.html
- https://www.zoho.com/crm/developer/docs/api/v3/api-limits.html
- https://www.zoho.com/crm/developer/docs/api/v3/status-codes.html
- https://www.zoho.com/crm/developer/docs/api/v3/module-meta.html
- https://www.zoho.com/crm/developer/docs/api/v3/field-meta.html
- https://www.zoho.com/crm/developer/docs/api/v3/get-records.html
Ready to get started?