Using the Xero API to Create or Update Sales Orders in Javascript
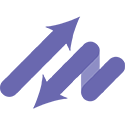
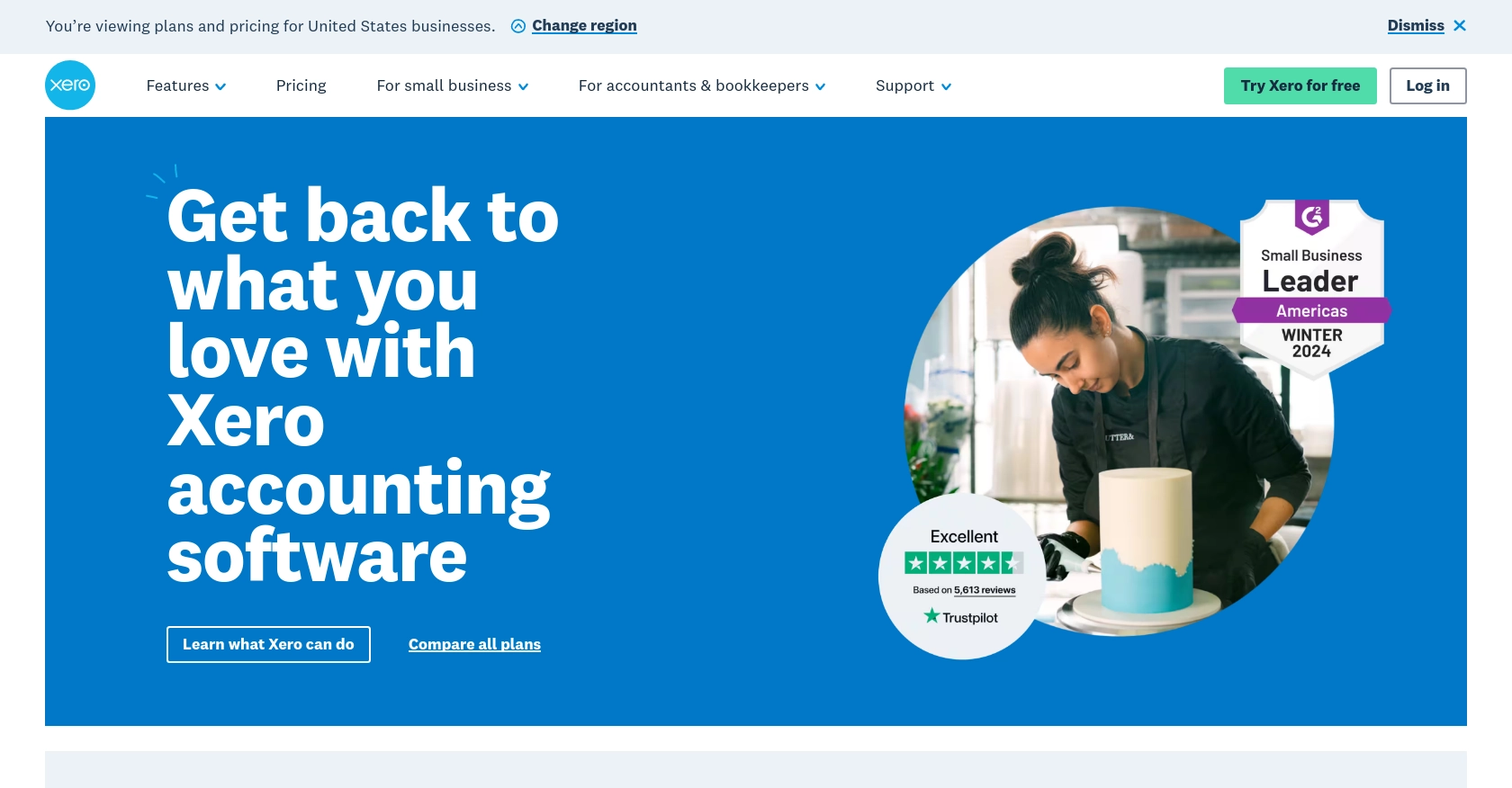
Introduction to Xero API for Sales Orders
Xero is a powerful cloud-based accounting software platform designed to simplify financial management for businesses of all sizes. It offers a wide range of features, including invoicing, payroll, and expense tracking, making it an essential tool for modern businesses.
Developers may want to integrate with Xero's API to automate and streamline accounting processes, such as creating or updating sales orders. For example, a developer could use the Xero API to automatically generate sales orders from an e-commerce platform, ensuring that financial records are always up-to-date and accurate.
Setting Up a Xero Test or Sandbox Account for API Integration
Before you can start integrating with the Xero API to create or update sales orders, you'll need to set up a test or sandbox account. This allows you to safely experiment with API calls without affecting real data.
Creating a Xero Developer Account
To begin, you'll need a Xero developer account. Follow these steps to create one:
- Visit the Xero Developer Portal and sign up for an account.
- Once registered, log in to your developer account.
Setting Up a Xero Sandbox Organization
After creating your developer account, you can set up a sandbox organization:
- Navigate to the "My Apps" section in the Xero Developer Portal.
- Select "Add a new app" and fill in the required details, such as the app name and company URL.
- Choose the "OAuth 2.0" authentication method, as Xero uses OAuth for secure API access.
- Once your app is created, you'll receive a client ID and client secret, which are essential for authentication.
Configuring OAuth 2.0 Authentication
To authenticate API requests, you'll need to configure OAuth 2.0:
- In your app settings, define the redirect URI that Xero will use to send authorization codes.
- Use the client ID and client secret to request an access token from Xero's authorization server.
- Ensure you have the necessary scopes for accessing sales orders. Refer to the Xero OAuth 2.0 Scopes documentation for more information.
For detailed guidance on the OAuth 2.0 flow, consult the Xero OAuth 2.0 Authorization Flow documentation.
Testing Your Xero API Integration
With your sandbox account and OAuth 2.0 configuration in place, you can now test your API integration:
- Use the access token to make API calls to the Xero sandbox environment.
- Verify that your sales orders are created or updated as expected in the sandbox.
By following these steps, you'll be well-prepared to integrate with the Xero API and automate your sales order processes.
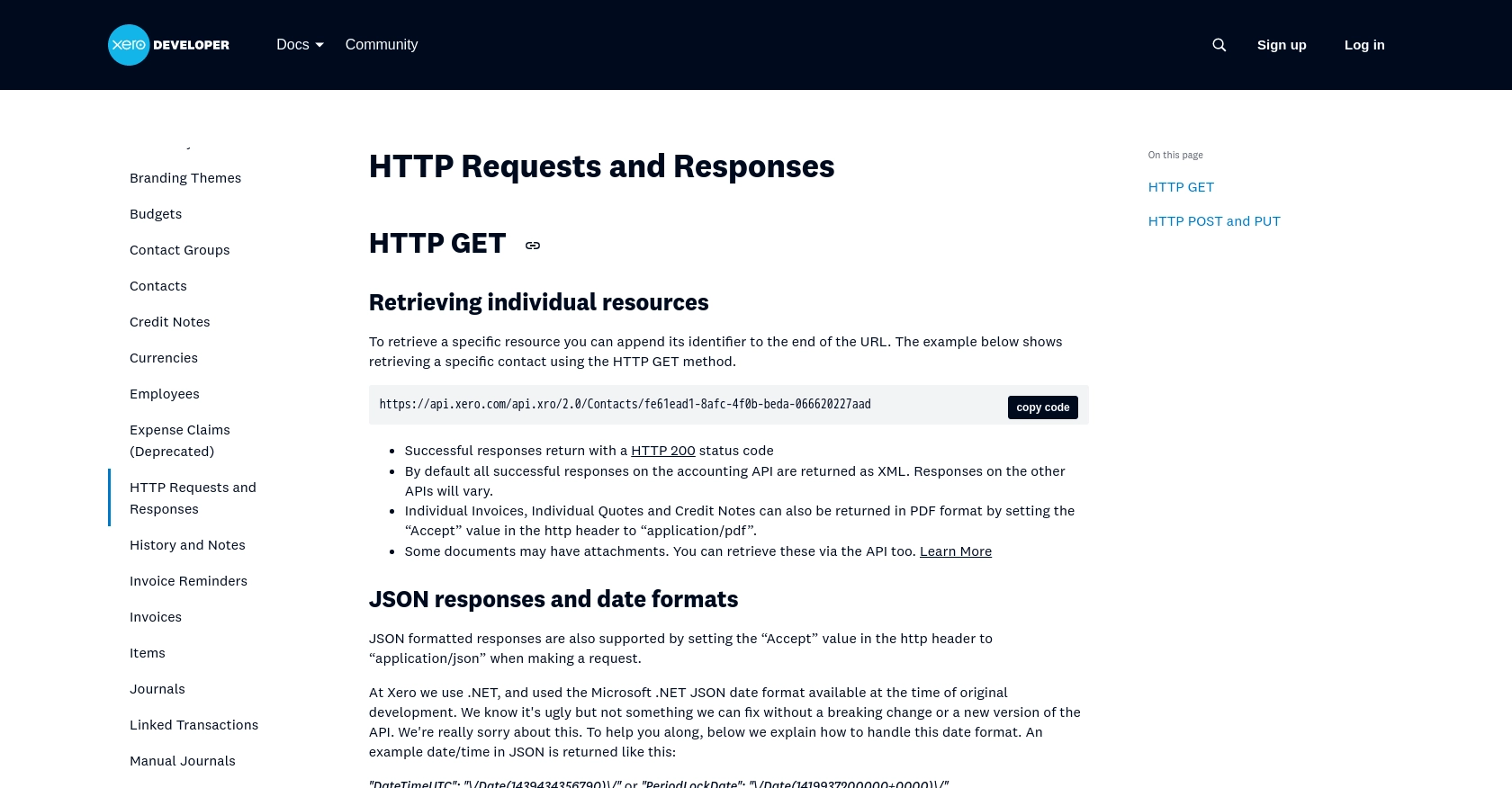
sbb-itb-96038d7
Making API Calls to Xero for Creating or Updating Sales Orders Using JavaScript
To interact with the Xero API for creating or updating sales orders, you'll need to use JavaScript to make HTTP requests. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling responses and errors.
Setting Up Your JavaScript Environment for Xero API Integration
Before you begin coding, ensure you have the following prerequisites:
- Node.js installed on your machine.
- A package manager like npm or yarn.
Install the Axios library to handle HTTP requests:
npm install axios
Writing JavaScript Code to Create or Update Sales Orders in Xero
Create a file named salesOrders.js
and add the following code:
const axios = require('axios');
// Set the API endpoint for sales orders
const endpoint = 'https://api.xero.com/api.xro/2.0/Orders';
// Set the access token obtained from OAuth 2.0 authentication
const accessToken = 'Your_Access_Token';
// Define the sales order data
const salesOrderData = {
"Orders": [
{
"OrderNumber": "12345",
"Contact": {
"ContactID": "contact-id"
},
"LineItems": [
{
"Description": "Product Description",
"Quantity": 1,
"UnitAmount": 100.00
}
]
}
]
};
// Configure the request headers
const headers = {
'Authorization': `Bearer ${accessToken}`,
'Content-Type': 'application/json'
};
// Function to create or update a sales order
async function createOrUpdateSalesOrder() {
try {
const response = await axios.post(endpoint, salesOrderData, { headers });
console.log('Sales Order Created/Updated:', response.data);
} catch (error) {
console.error('Error creating/updating sales order:', error.response.data);
}
}
// Execute the function
createOrUpdateSalesOrder();
Replace Your_Access_Token
with the access token obtained during the OAuth 2.0 authentication process.
Verifying Successful API Requests in Xero Sandbox
After running the code, check your Xero sandbox account to verify that the sales order has been created or updated as expected. You should see the new or modified sales order in the sandbox environment.
Handling Errors and Understanding Xero API Error Codes
When making API calls, it's crucial to handle potential errors. The Xero API may return various error codes, such as:
- 400 Bad Request: The request was invalid or cannot be served.
- 401 Unauthorized: Authentication failed or user does not have permissions.
- 403 Forbidden: The request is understood, but it has been refused.
- 404 Not Found: The requested resource could not be found.
Refer to the Xero API Requests and Responses documentation for more details on handling errors.
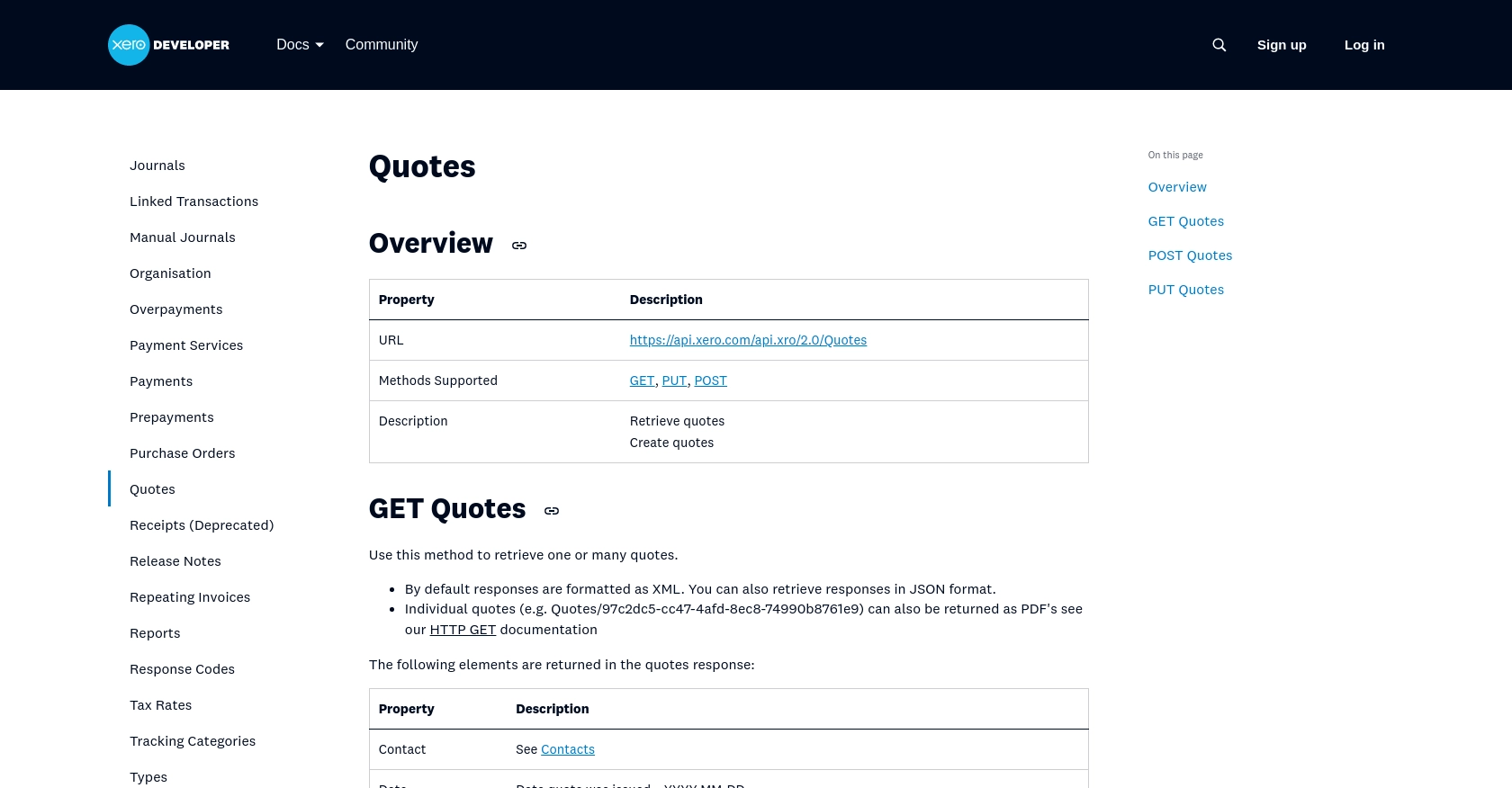
Conclusion: Best Practices for Xero API Integration and Next Steps
Integrating with the Xero API to create or update sales orders using JavaScript can significantly enhance your business's accounting processes. By automating these tasks, you ensure accuracy and efficiency, freeing up valuable time for other critical operations.
Best Practices for Secure and Efficient Xero API Integration
- Securely Store Credentials: Always store your OAuth 2.0 credentials, such as client ID and client secret, securely. Avoid hardcoding them in your source code.
- Handle Rate Limiting: Xero's API has rate limits in place. Ensure your application gracefully handles these limits by implementing retry logic and monitoring API usage. Refer to the Xero OAuth 2.0 API Limits documentation for more details.
- Standardize Data Fields: Consistently format and validate data fields to match Xero's requirements, reducing the risk of errors during API calls.
Streamline Your Integration Process with Endgrate
While integrating with Xero's API can be rewarding, it may also be complex and time-consuming. Endgrate offers a solution by providing a unified API endpoint that connects to multiple platforms, including Xero. This allows you to build once for each use case, saving time and resources.
With Endgrate, you can focus on your core product while enjoying an intuitive integration experience for your customers. Explore how Endgrate can simplify your integration needs by visiting Endgrate today.
Read More
- https://endgrate.com/provider/xero
- https://developer.xero.com/documentation/api/accounting/requests-and-responses
- https://developer.xero.com/documentation/guides/oauth2/limits/
- https://developer.xero.com/documentation/guides/oauth2/overview
- https://developer.xero.com/documentation/guides/oauth2/scopes
- https://developer.xero.com/documentation/guides/oauth2/auth-flow
- https://developer.xero.com/documentation/guides/oauth2/tenants
- https://developer.xero.com/documentation/api/accounting/quotes
Ready to get started?