Using the Keap API to Create or Update Contacts (with Javascript examples)
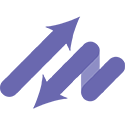
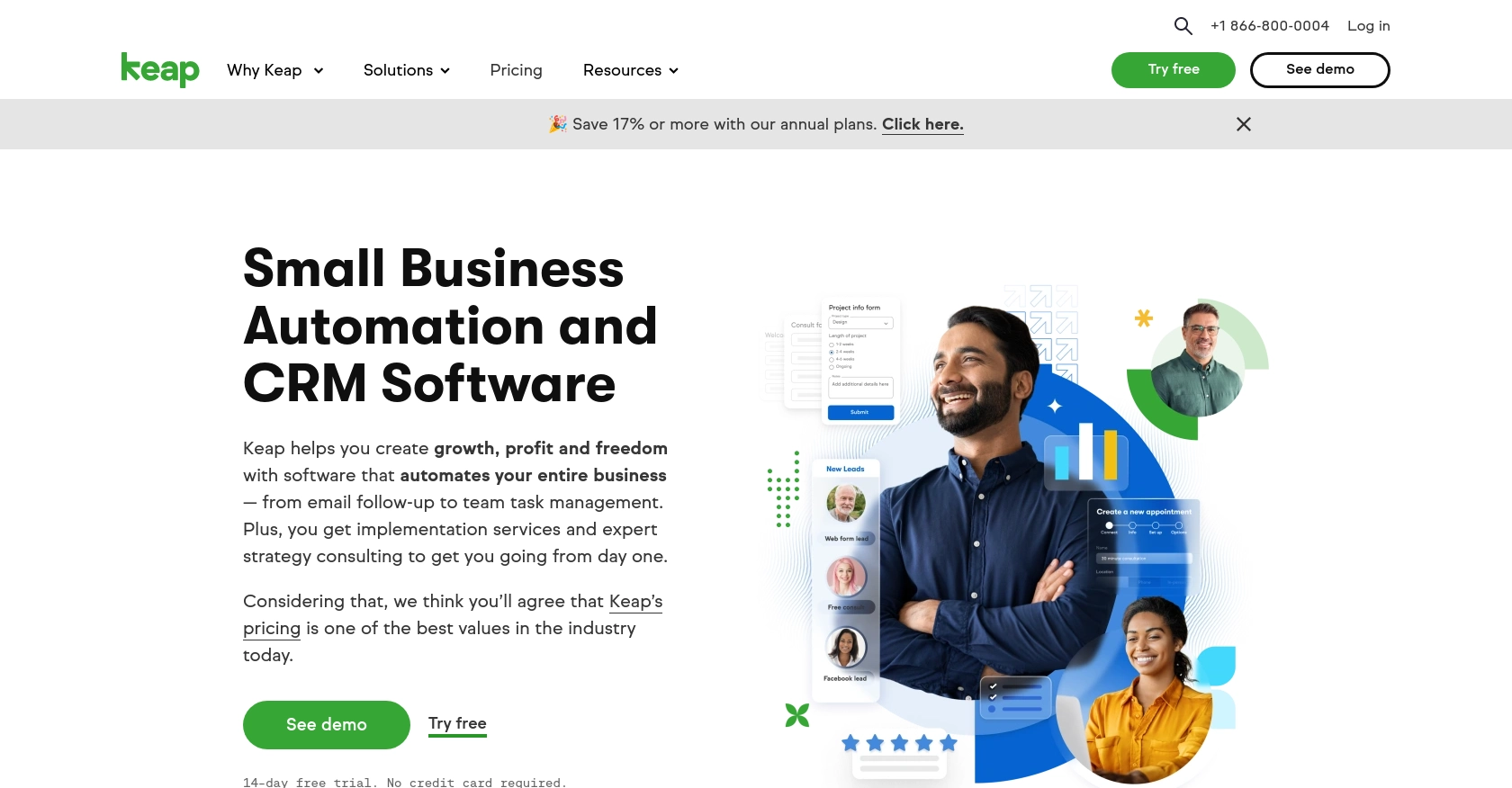
Introduction to Keap API Integration
Keap, formerly known as Infusionsoft, is a powerful CRM and marketing automation platform designed to help small businesses streamline their sales and marketing efforts. With features like contact management, email marketing, and e-commerce, Keap provides a comprehensive solution for businesses looking to grow and manage their customer relationships effectively.
Integrating with the Keap API allows developers to automate and enhance various business processes by interacting directly with Keap's robust features. For example, developers can use the Keap API to create or update contact information programmatically, ensuring that customer data is always up-to-date and synchronized across different platforms.
This article will guide you through the process of using JavaScript to interact with the Keap API, focusing on creating or updating contacts. By following this tutorial, you'll learn how to leverage Keap's API to automate contact management, saving time and reducing manual data entry errors.
Setting Up Your Keap Developer Account and Sandbox Environment
Before you can start integrating with the Keap API, you'll need to set up a developer account and a sandbox environment. This will allow you to test your API interactions without affecting live data. Follow these steps to get started:
Create a Keap Developer Account
To begin, you need to create a Keap developer account. This account will give you access to the necessary tools and resources to build and test your integrations.
- Visit the Keap Developer Portal.
- Click on the "Register" button to sign up for a developer account.
- Fill in the required information and submit the form to create your account.
Set Up a Keap Sandbox App
Once your developer account is ready, the next step is to create a sandbox app. This environment will allow you to safely test your API calls.
- Log in to your Keap developer account.
- Navigate to the "Sandbox" section and click on "Create Sandbox App."
- Follow the prompts to set up your sandbox app, which will provide you with a Client ID and Client Secret.
Configure OAuth Authentication for Keap API
The Keap API uses OAuth 2.0 for authentication. You'll need to create an app to obtain the necessary credentials for authorization.
- In your sandbox app, go to the "OAuth" section.
- Click on "Create App" and fill in the required details, including the redirect URI.
- Once the app is created, note down the Client ID and Client Secret, as you'll need these for API requests.
For more detailed information on setting up OAuth, refer to the Keap REST API documentation.
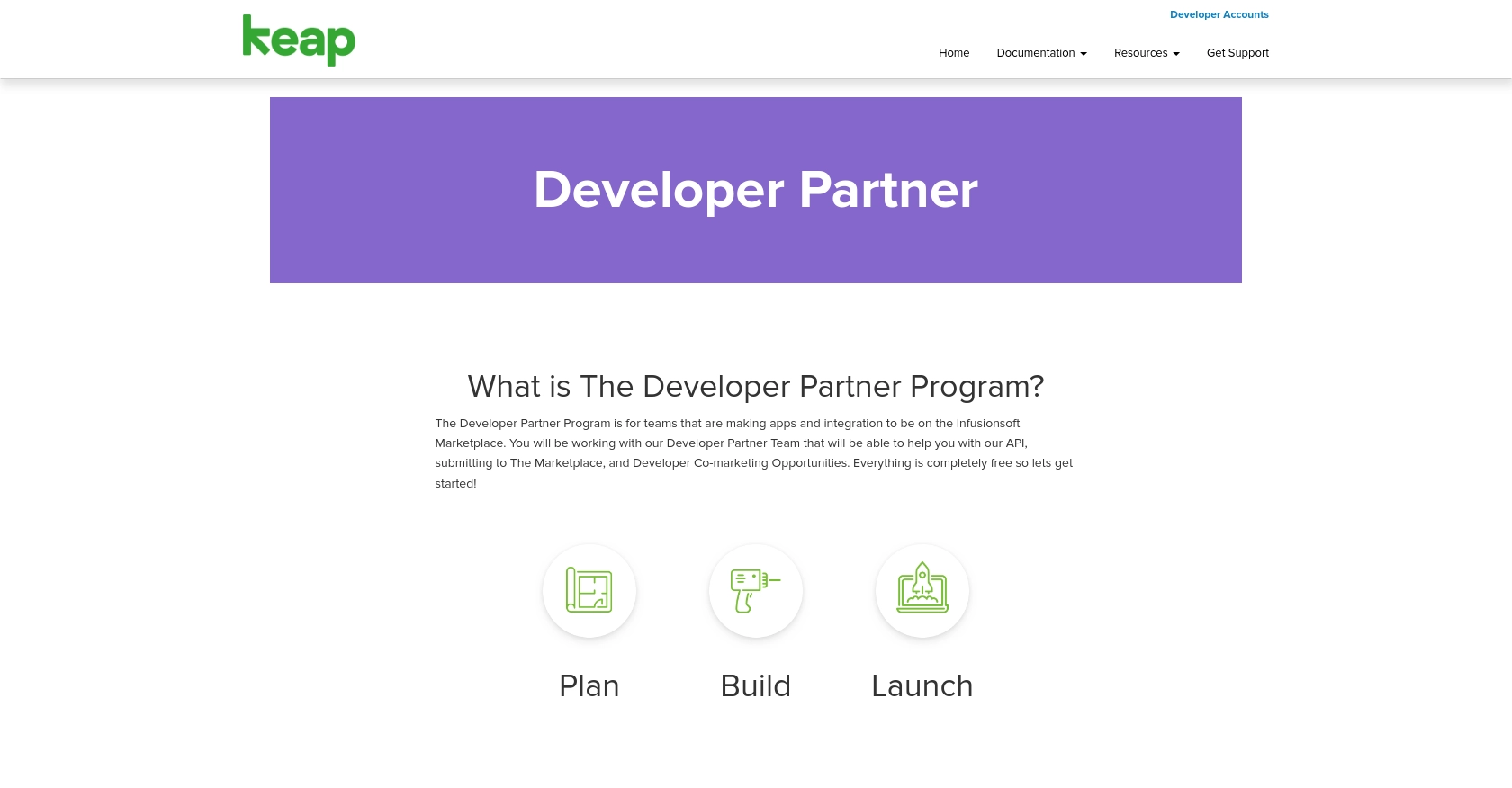
sbb-itb-96038d7
Making API Calls to Keap for Contact Management Using JavaScript
With your Keap developer account and sandbox environment set up, you can now proceed to make API calls to create or update contacts. This section will guide you through the process using JavaScript, ensuring you have the right setup and code to interact with the Keap API effectively.
Prerequisites for JavaScript and Keap API Integration
Before diving into the code, ensure you have the following prerequisites:
- Node.js installed on your machine. You can download it from the official Node.js website.
- A code editor like Visual Studio Code.
- Basic knowledge of JavaScript and RESTful APIs.
Installing Required Dependencies
To interact with the Keap API, you'll need to install the Axios library, which simplifies making HTTP requests. Run the following command in your terminal:
npm install axios
Creating or Updating Contacts with Keap API Using JavaScript
Now, let's write the JavaScript code to create or update contacts in Keap. Start by creating a new file named keapContacts.js
and add the following code:
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://api.infusionsoft.com/crm/rest/v1/contacts';
const headers = {
'Content-Type': 'application/json',
'Authorization': 'Bearer YOUR_ACCESS_TOKEN'
};
// Function to create or update a contact
async function createOrUpdateContact(contactData) {
try {
const response = await axios.post(endpoint, contactData, { headers });
console.log('Contact created or updated successfully:', response.data);
} catch (error) {
console.error('Error creating or updating contact:', error.response ? error.response.data : error.message);
}
}
// Sample contact data
const contactData = {
given_name: 'John',
family_name: 'Doe',
email_addresses: [{ email: 'john.doe@example.com', field: 'EMAIL1' }]
};
// Call the function
createOrUpdateContact(contactData);
Replace YOUR_ACCESS_TOKEN
with the access token obtained from your OAuth setup. The code above uses Axios to send a POST request to the Keap API, creating or updating a contact with the provided data.
Verifying API Call Success in Keap Sandbox
After running the script, you can verify the success of your API call by checking the contacts in your Keap sandbox environment. If the contact was created or updated successfully, it should appear in the sandbox with the specified details.
Handling Errors and Common Error Codes
When making API calls, it's crucial to handle potential errors gracefully. The code above includes a try-catch
block to catch and log errors. Common error codes you might encounter include:
- 400 Bad Request: The request was malformed. Check your request data and headers.
- 401 Unauthorized: The access token is missing or invalid. Ensure your token is correct and not expired.
- 404 Not Found: The endpoint URL is incorrect. Verify the API endpoint.
For more detailed error handling, refer to the Keap REST API documentation.
Conclusion and Best Practices for Keap API Integration
Integrating with the Keap API using JavaScript allows developers to automate contact management processes, ensuring that customer data is consistently updated and synchronized across platforms. By following the steps outlined in this article, you can efficiently create or update contacts within Keap, leveraging its powerful CRM capabilities to enhance your business operations.
Best Practices for Secure and Efficient Keap API Usage
- Securely Store Credentials: Always store your Client ID, Client Secret, and access tokens securely. Consider using environment variables or a secure vault to manage sensitive information.
- Handle Rate Limiting: Be mindful of Keap's API rate limits to avoid throttling. Implement retry logic with exponential backoff to manage requests efficiently.
- Standardize Data Fields: Ensure that data fields are consistent and standardized across your applications to prevent data mismatches and errors.
Enhance Your Integration Strategy with Endgrate
While integrating with Keap is a powerful way to manage contacts, handling multiple integrations can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint for various platforms, including Keap. By using Endgrate, you can streamline your integration efforts, focus on your core product, and deliver a seamless experience to your customers.
Explore how Endgrate can transform your integration strategy by visiting Endgrate's website and discover how you can save time and resources by outsourcing integrations.
Read More
Ready to get started?