Using the Stripe API to Get Customers in PHP
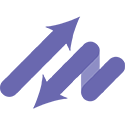
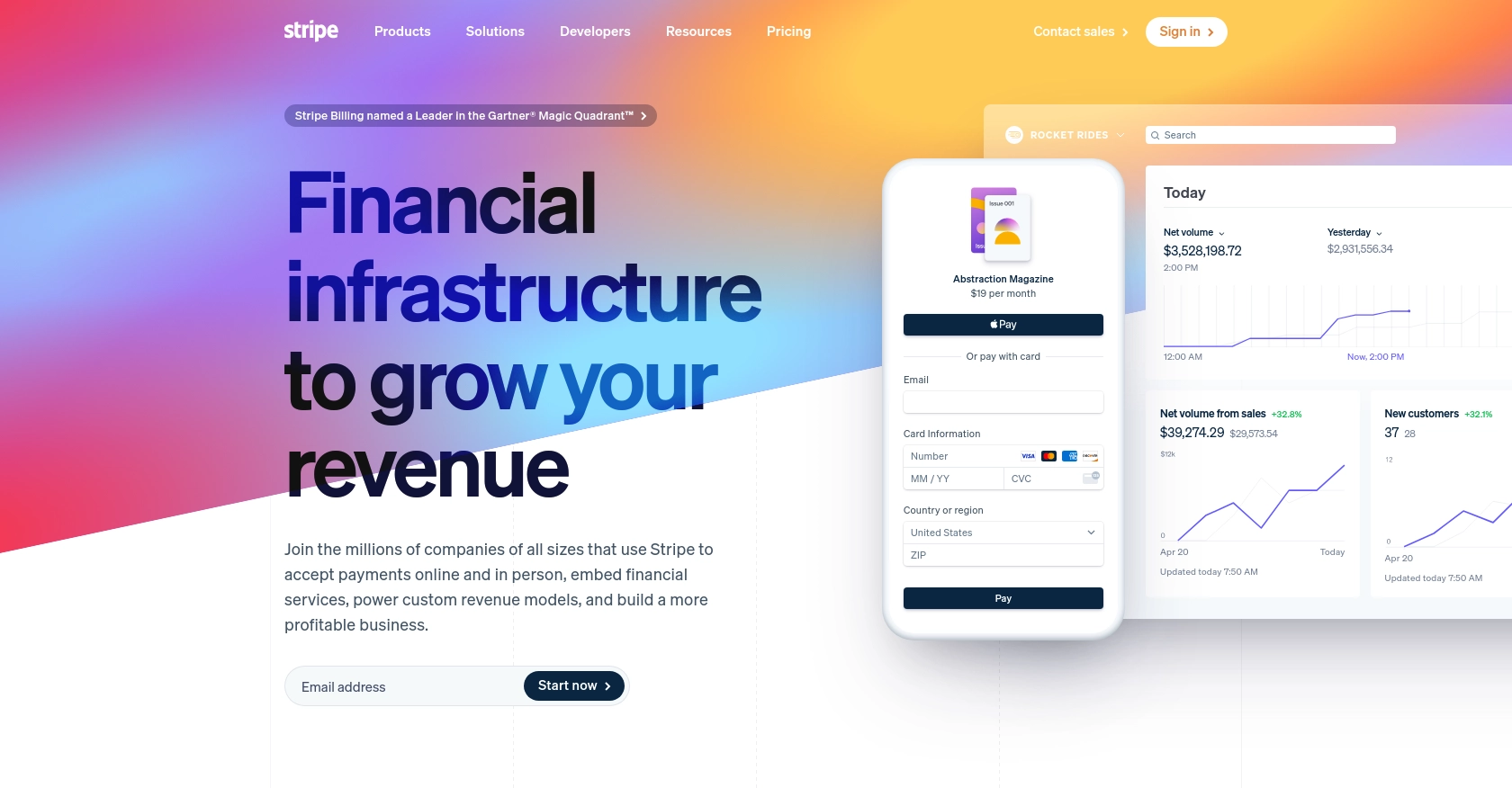
Introduction to Stripe API Integration
Stripe is a powerful payment processing platform that enables businesses to accept payments online with ease. It offers a wide range of tools and APIs that allow developers to build custom payment solutions tailored to their specific needs. With Stripe, businesses can manage transactions, subscriptions, and customer data efficiently.
Integrating with the Stripe API can be incredibly beneficial for developers looking to streamline payment processes and manage customer information. For example, using the Stripe API, a developer can retrieve customer data to analyze purchasing patterns or manage subscriptions, enhancing the overall customer experience.
This article will guide you through the process of using PHP to interact with the Stripe API, specifically focusing on retrieving customer information. By the end of this tutorial, you'll be equipped with the knowledge to seamlessly integrate Stripe's capabilities into your PHP applications.
Setting Up Your Stripe Test Account for API Integration
Before you can start integrating with the Stripe API using PHP, you need to set up a Stripe test account. This will allow you to safely test your API calls without affecting any live data. Stripe provides a sandbox environment where you can experiment with API requests and responses.
Creating a Stripe Account
If you don't already have a Stripe account, you can sign up for free on the Stripe website. Once you've created your account, you'll have access to the Stripe Dashboard, where you can manage your API keys, view test data, and configure your account settings.
Accessing the Stripe Dashboard
Log in to your Stripe account and navigate to the Dashboard. Here, you can switch between live and test modes. Ensure that you are in test mode to avoid any unintended charges or data changes.
Generating Stripe API Keys
Stripe uses API keys to authenticate requests. In test mode, you will use test API keys, which usually start with sk_test_
for secret keys. Follow these steps to obtain your API keys:
- In the Stripe Dashboard, click on Developers in the left-hand menu.
- Select API keys.
- Locate the Secret key and Publishable key under the Test data section.
- Copy these keys and store them securely, as you'll need them to authenticate your API requests.
Configuring API Key Security
Your API keys carry significant privileges, so it's crucial to keep them secure. Avoid sharing your secret keys in publicly accessible areas, such as GitHub repositories or client-side code. For more information on securing your API keys, refer to the Stripe API documentation.
Testing API Calls in the Sandbox Environment
With your test API keys, you can now make API calls in the Stripe sandbox environment. This allows you to simulate transactions, create test customers, and explore the API's capabilities without impacting live data. Use the test mode to verify that your integration works as expected before moving to production.
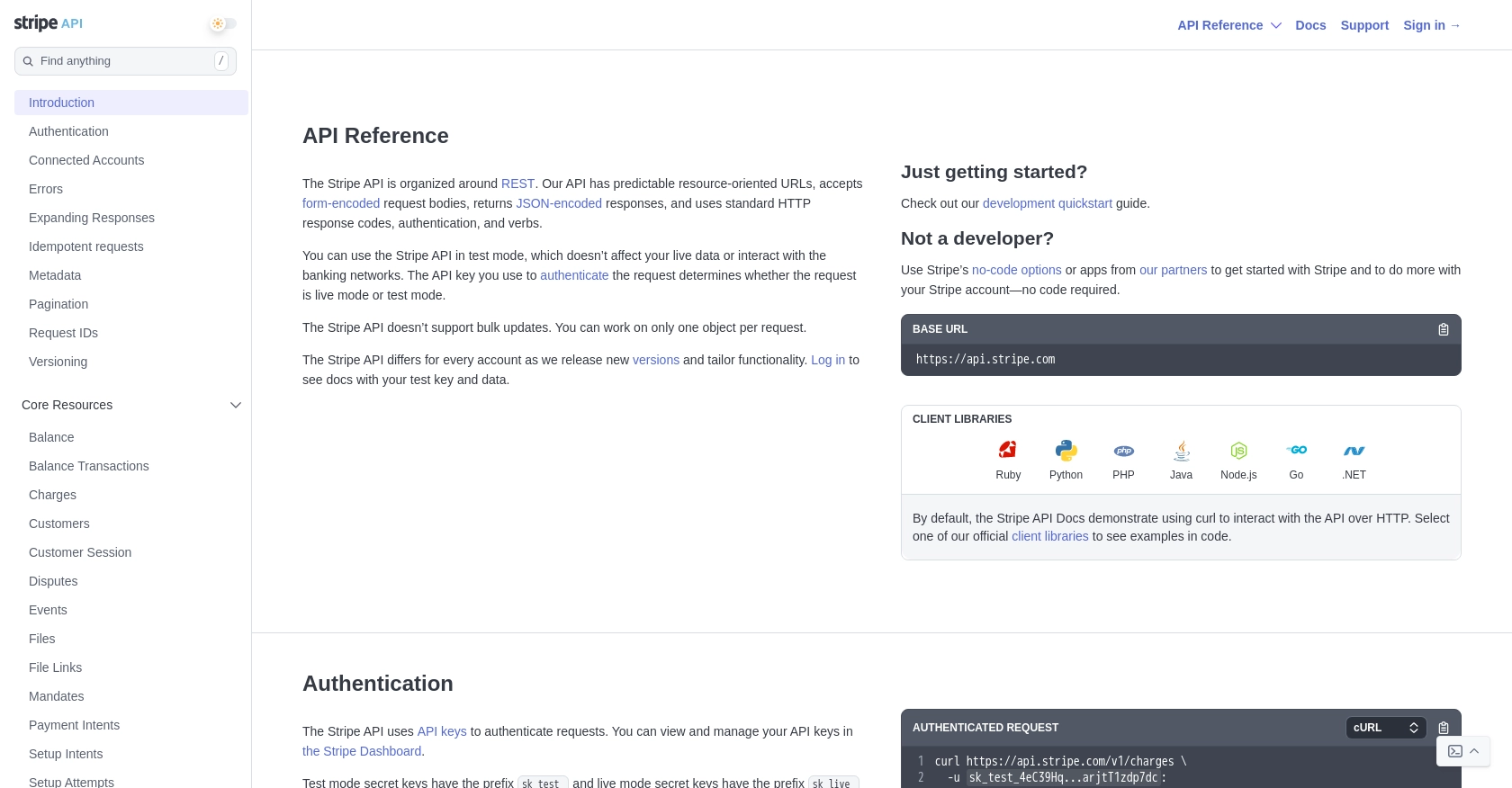
sbb-itb-96038d7
How to Make API Calls to Retrieve Customers Using Stripe API in PHP
To interact with the Stripe API using PHP, you'll need to set up your environment and write code to make API calls. This section will guide you through the process of retrieving customer information from Stripe using PHP.
Setting Up Your PHP Environment for Stripe API Integration
Before making API calls, ensure your PHP environment is correctly configured. You'll need PHP version 7.2 or higher and the Stripe PHP library. Follow these steps to set up your environment:
- Ensure PHP is installed on your server. You can check your PHP version by running
php -v
in your terminal. - Install Composer, a dependency manager for PHP, if you haven't already. You can download it from the Composer website.
- Use Composer to install the Stripe PHP library by running the following command:
composer require stripe/stripe-php
Writing PHP Code to Retrieve Customers from Stripe
With your environment set up, you can now write PHP code to retrieve customer data from Stripe. Follow these steps to create a script that fetches customer information:
require 'vendor/autoload.php';
// Set your secret API key
\Stripe\Stripe::setApiKey('sk_test_your_secret_key');
// Retrieve a list of customers
try {
$customers = \Stripe\Customer::all(['limit' => 10]);
foreach ($customers->data as $customer) {
echo 'Customer ID: ' . $customer->id . '<br>';
echo 'Email: ' . $customer->email . '<br>';
}
} catch (\Stripe\Exception\ApiErrorException $e) {
echo 'Error: ' . $e->getMessage();
}
Replace 'sk_test_your_secret_key'
with your actual test secret API key. This script uses the Stripe PHP library to authenticate requests and retrieve a list of customers. It handles errors gracefully by catching exceptions and displaying error messages.
Verifying Successful API Requests in Stripe Dashboard
After running your PHP script, verify that the API requests were successful by checking the Stripe Dashboard. Navigate to the Developers section and select Logs to view the details of your API requests. You should see entries corresponding to the customer retrieval requests made by your script.
Handling Errors and Understanding Stripe API Response Codes
Stripe uses standard HTTP response codes to indicate the success or failure of an API request. Here are some common response codes you might encounter:
- 200 OK: The request was successful.
- 400 Bad Request: The request was unacceptable, often due to missing parameters.
- 401 Unauthorized: No valid API key provided.
- 402 Request Failed: The parameters were valid but the request failed.
- 429 Too Many Requests: Too many requests hit the API too quickly. Implement exponential backoff to handle rate limiting.
For more detailed information on handling errors, refer to the Stripe API documentation.
Conclusion and Best Practices for Using Stripe API in PHP
Integrating the Stripe API into your PHP applications can significantly enhance your ability to manage customer data and streamline payment processes. By following the steps outlined in this guide, you can efficiently retrieve customer information and handle transactions securely.
Best Practices for Secure and Efficient Stripe API Integration
- Secure API Keys: Always keep your API keys secure and never expose them in public repositories or client-side code. Use environment variables or secure vaults to store them.
- Handle Rate Limiting: Stripe imposes rate limits on API requests. Implement exponential backoff strategies to handle
429 Too Many Requests
errors gracefully. - Data Standardization: Ensure that customer data retrieved from Stripe is standardized and transformed as needed to fit your application's requirements.
- Error Handling: Implement robust error handling to manage different API response codes and exceptions, ensuring a smooth user experience.
Streamline Your Integrations with Endgrate
While integrating with Stripe is powerful, managing multiple integrations can be complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process across various platforms, including Stripe. By using Endgrate, you can save time and resources, allowing you to focus on your core product development.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate and discover a more efficient way to manage your API integrations.
Read More
Ready to get started?