How to Get Notes with the Zoho CRM API in Python
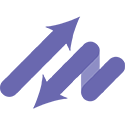
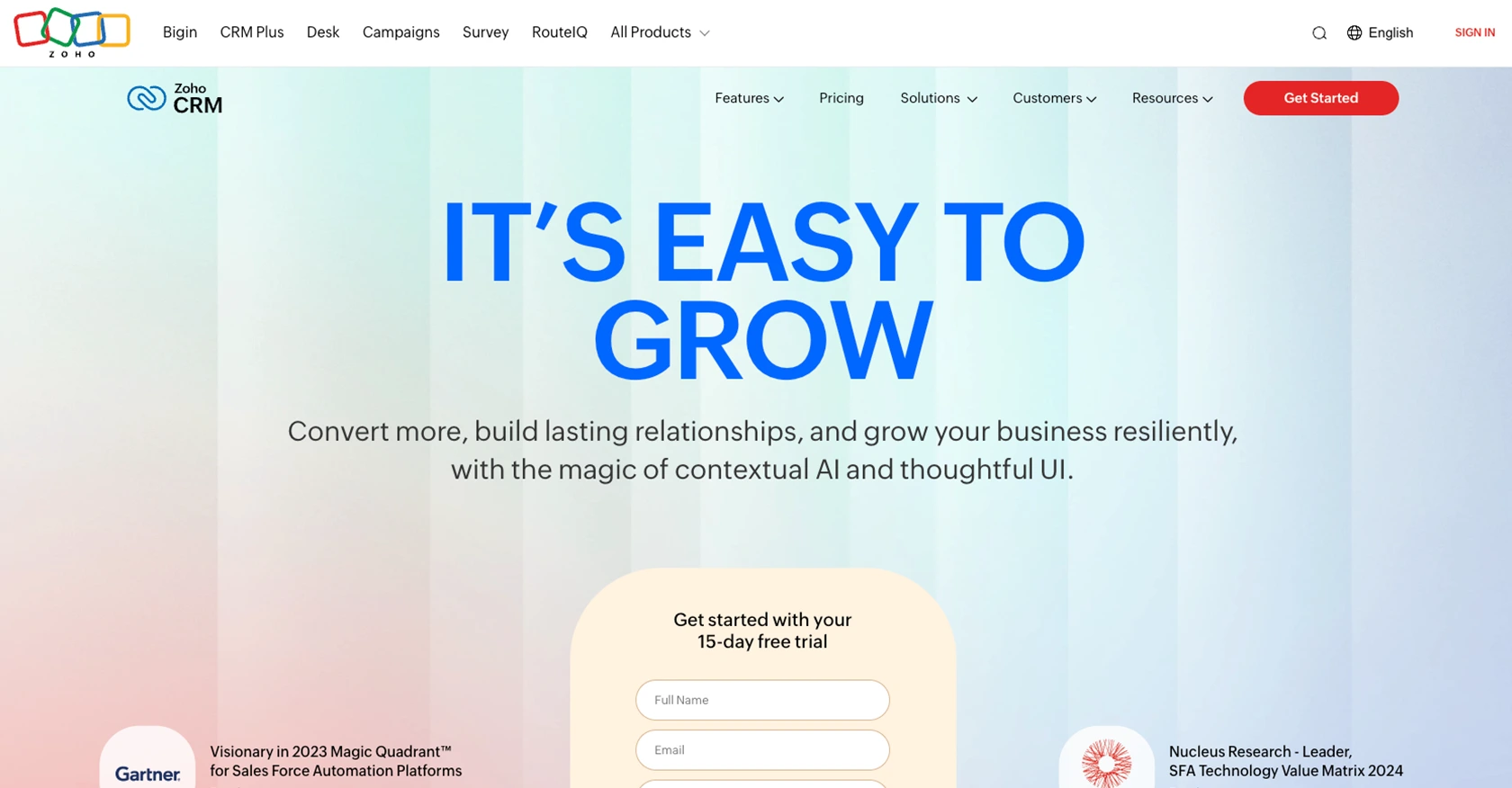
Introduction to Zoho CRM API
Zoho CRM is a comprehensive customer relationship management platform that empowers businesses to manage their sales, marketing, and support in a unified interface. Known for its flexibility and scalability, Zoho CRM is a popular choice for organizations looking to enhance their customer interactions and streamline operations.
Developers often integrate with the Zoho CRM API to automate and enhance their workflows. One common use case is retrieving notes associated with various CRM records. For example, a developer might want to extract notes from Zoho CRM to analyze customer interactions or to synchronize them with another application for a more cohesive data management strategy.
Setting Up Your Zoho CRM Sandbox Account
Before you can start interacting with the Zoho CRM API, you need to set up a sandbox account. This allows you to test API calls without affecting your live data. Zoho CRM provides a robust sandbox environment that mirrors your production setup, making it ideal for development and testing.
Creating a Zoho CRM Sandbox Account
If you don't have a Zoho CRM account, you can sign up for a free trial on the Zoho CRM website. Once your account is created, you can access the sandbox environment from the Zoho CRM dashboard.
Registering a Client Application for OAuth Authentication
Zoho CRM uses OAuth 2.0 for authentication, which requires you to register your application to obtain the necessary credentials. Follow these steps to register your application:
- Go to the Zoho Developer Console.
- Select "Add Client" and choose "Server-based Applications" as the client type.
- Fill in the required details, including the Client Name, Homepage URL, and Authorized Redirect URIs.
- Click "Create" to generate your Client ID and Client Secret.
Ensure you save the Client ID and Client Secret securely, as you will need them for generating access tokens.
Generating Access Tokens for Zoho CRM API
With your client application registered, you can now generate access tokens to authenticate API requests. Follow these steps:
- Direct users to the Zoho authorization URL to obtain an authorization code:
- Exchange the authorization code for an access token by making a POST request to the token endpoint:
- Store the access token securely for use in API requests.
https://accounts.zoho.com/oauth/v2/auth?scope=ZohoCRM.modules.ALL&client_id=YOUR_CLIENT_ID&response_type=code&access_type=offline&redirect_uri=YOUR_REDIRECT_URI
import requests
url = "https://accounts.zoho.com/oauth/v2/token"
payload = {
"grant_type": "authorization_code",
"client_id": "YOUR_CLIENT_ID",
"client_secret": "YOUR_CLIENT_SECRET",
"redirect_uri": "YOUR_REDIRECT_URI",
"code": "AUTHORIZATION_CODE"
}
response = requests.post(url, data=payload)
access_token = response.json().get("access_token")
print(access_token)
Remember that access tokens are valid for a limited time, and you may need to refresh them periodically. Refer to the Zoho CRM OAuth documentation for more details.
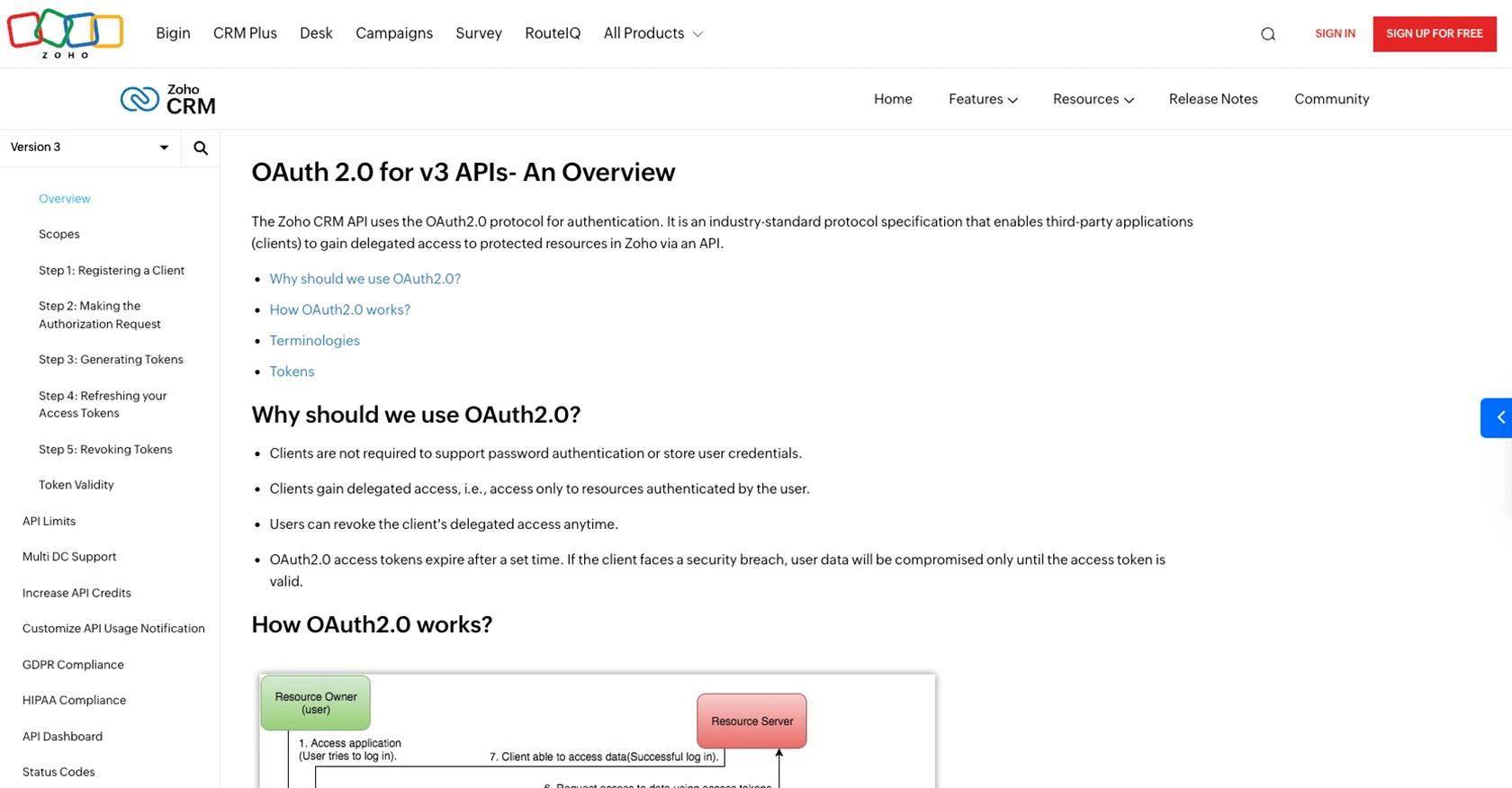
sbb-itb-96038d7
Making API Calls to Retrieve Notes from Zoho CRM Using Python
To interact with the Zoho CRM API and retrieve notes, you need to ensure your Python environment is properly set up. This section will guide you through the necessary steps to make successful API calls using Python.
Setting Up Your Python Environment for Zoho CRM API Integration
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you will need the requests
library to handle HTTP requests. Install it using the following command:
pip install requests
Writing Python Code to Retrieve Notes from Zoho CRM
Once your environment is ready, you can write a Python script to fetch notes from Zoho CRM. Create a file named get_zoho_notes.py
and add the following code:
import requests
# Define the API endpoint and headers
endpoint = "https://www.zohoapis.com/crm/v3/Notes"
headers = {
"Authorization": "Zoho-oauthtoken YOUR_ACCESS_TOKEN"
}
# Make a GET request to the Zoho CRM API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
notes_data = response.json().get("data", [])
for note in notes_data:
print(f"Note ID: {note['id']}, Content: {note['Note_Content']}")
else:
print(f"Failed to retrieve notes: {response.status_code} - {response.text}")
Replace YOUR_ACCESS_TOKEN
with the access token you obtained earlier. This script sends a GET request to the Zoho CRM API to fetch notes and prints the note ID and content if the request is successful.
Verifying Successful API Calls and Handling Errors
After running your script, verify the output to ensure notes are retrieved correctly. If the request fails, the script will print an error message with the status code and response text. Common error codes include:
- 401 Unauthorized: Check if your access token is valid and not expired.
- 403 Forbidden: Ensure your API credentials have the necessary permissions to access the Notes module.
- 404 Not Found: Verify the API endpoint URL is correct.
Refer to the Zoho CRM status codes documentation for more details on handling errors.
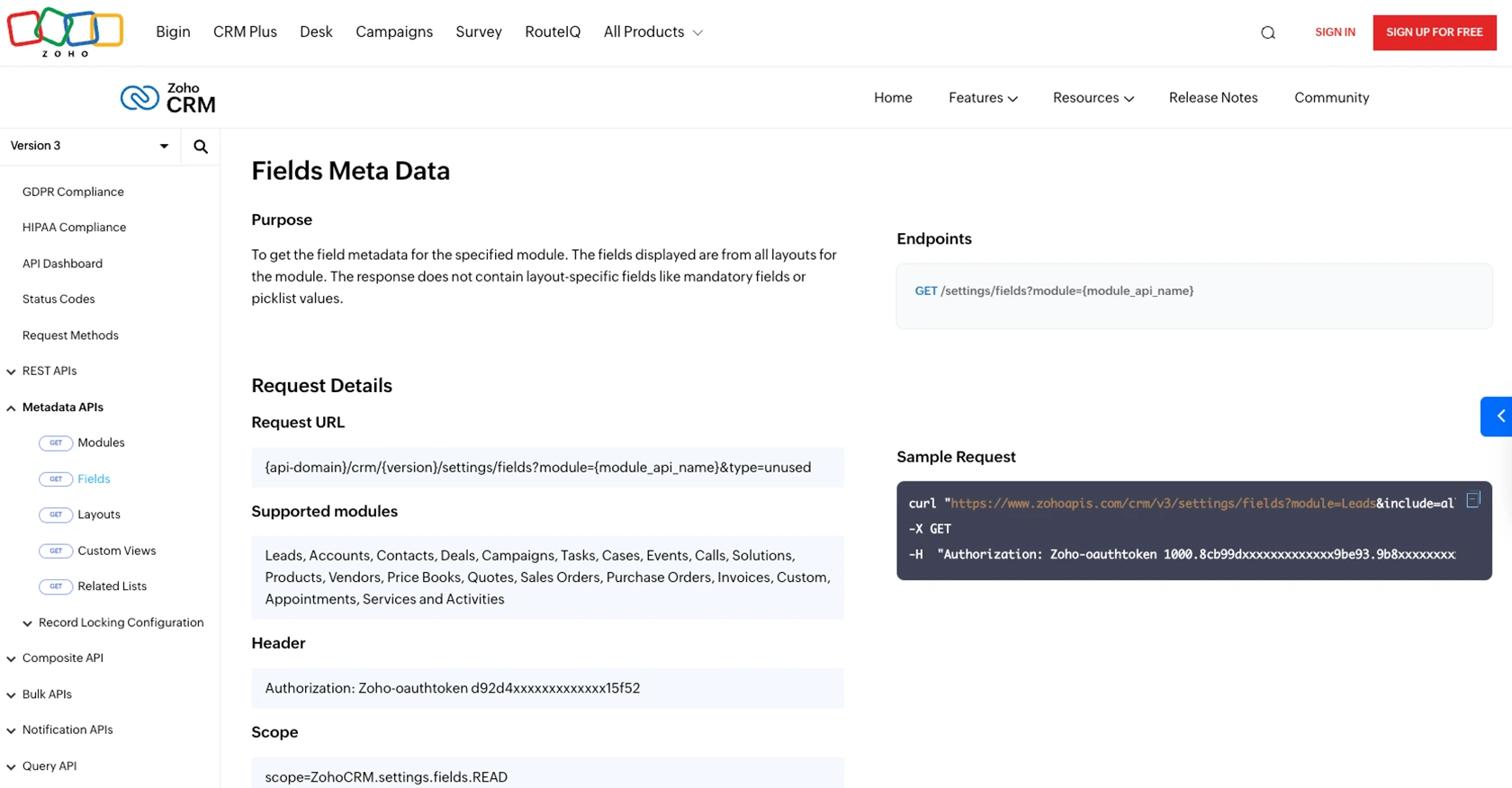
Conclusion and Best Practices for Zoho CRM API Integration
Integrating with the Zoho CRM API to retrieve notes can significantly enhance your data management and analysis capabilities. By following the steps outlined in this guide, you can efficiently set up your environment, authenticate using OAuth 2.0, and make API calls to access valuable CRM data.
Best Practices for Secure and Efficient Zoho CRM API Usage
- Secure Storage of Credentials: Always store your Client ID, Client Secret, and access tokens securely. Avoid hardcoding them in your scripts or exposing them in public repositories.
- Handling Rate Limits: Be mindful of Zoho CRM's API rate limits. As per the documentation, ensure your application handles rate limiting gracefully by implementing retry logic or backoff strategies.
- Data Transformation and Standardization: When retrieving notes or other data, consider transforming and standardizing fields to match your application's data model for seamless integration.
- Error Handling: Implement robust error handling to manage API response errors effectively. Refer to the status codes documentation for guidance on resolving common issues.
Streamlining Integrations with Endgrate
Building and maintaining multiple integrations can be time-consuming and complex. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Zoho CRM. By leveraging Endgrate, you can focus on your core product while outsourcing integration tasks, ensuring a seamless experience for your customers.
Explore how Endgrate can help you save time and resources by visiting Endgrate's website and discover the benefits of a streamlined integration strategy.
Read More
- https://endgrate.com/provider/zohocrm
- https://www.zoho.com/crm/developer/docs/api/v3/oauth-overview.html
- https://www.zoho.com/crm/developer/docs/api/v3/scopes.html
- https://www.zoho.com/crm/developer/docs/api/v3/register-client.html
- https://www.zoho.com/crm/developer/docs/api/v3/api-limits.html
- https://www.zoho.com/crm/developer/docs/api/v3/status-codes.html
- https://www.zoho.com/crm/developer/docs/api/v3/field-meta.html
- https://www.zoho.com/crm/developer/docs/api/v3/get-records.html
Ready to get started?