Using the FreshService API to Create or Update Departments (with PHP examples)
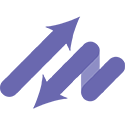
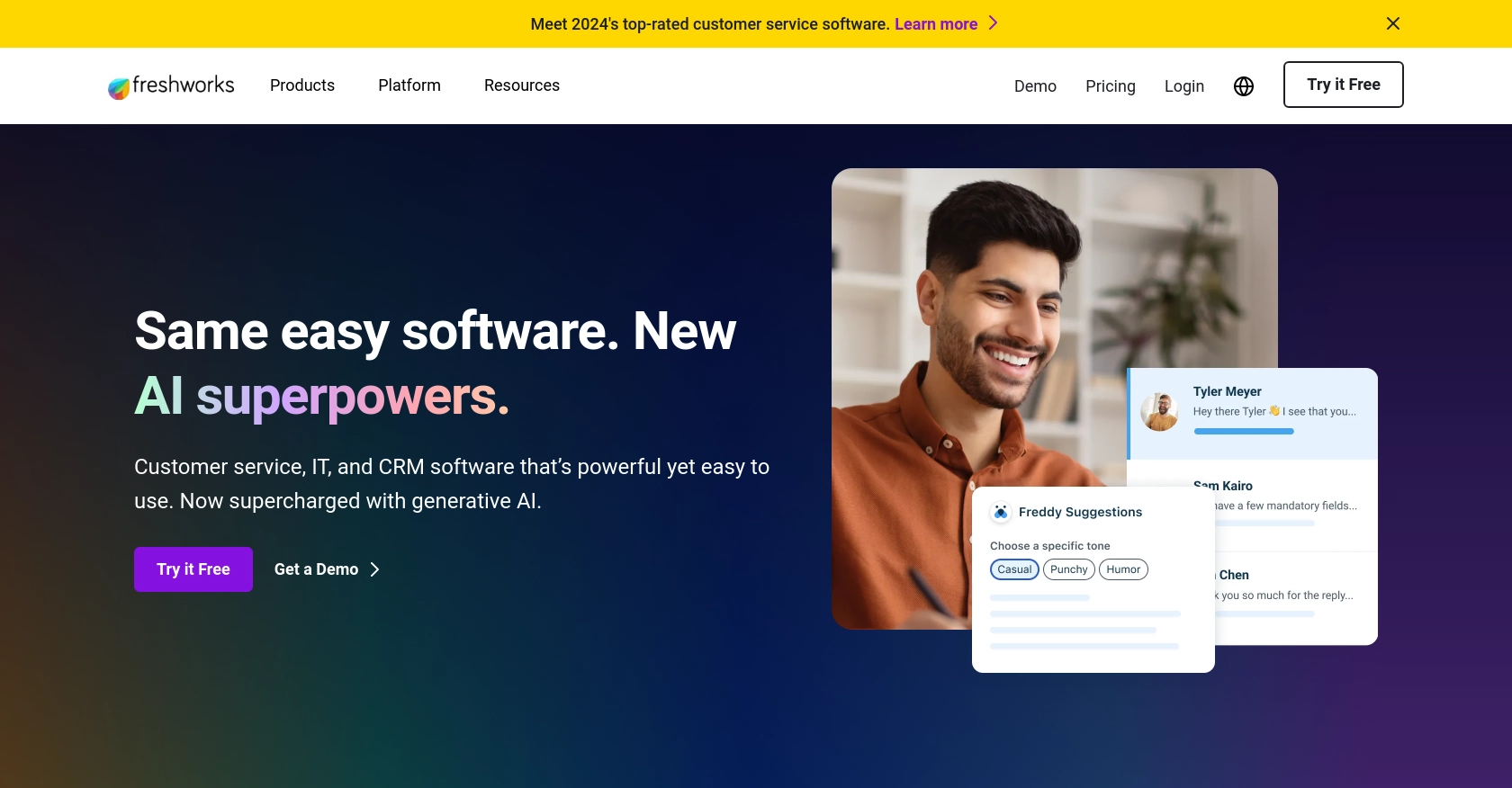
Introduction to FreshService API Integration
FreshService is a cloud-based IT service management (ITSM) solution designed to simplify IT operations for businesses. It offers a comprehensive suite of tools for incident management, asset management, change management, and more, making it a popular choice for organizations looking to streamline their IT processes.
Integrating with the FreshService API allows developers to automate and enhance IT service workflows. For example, you can use the API to create or update departments within FreshService, enabling seamless management of organizational structures and improving efficiency in handling service requests.
This article will guide you through using PHP to interact with the FreshService API, focusing on creating or updating departments. By following this tutorial, developers can leverage FreshService's capabilities to optimize their IT service management tasks.
Setting Up Your FreshService Test or Sandbox Account
Before you begin integrating with the FreshService API, it's essential to set up a test or sandbox account. This allows you to safely experiment with API calls without affecting your live data. FreshService provides a trial version that you can use for testing purposes.
Creating a FreshService Account
If you don't already have a FreshService account, follow these steps to create one:
- Visit the FreshService sign-up page.
- Fill in the required information, including your email address and company details.
- Follow the on-screen instructions to complete the registration process.
- Once registered, you will have access to a trial version of FreshService.
Generating API Key for FreshService
FreshService uses a custom authentication method that involves generating an API key. Follow these steps to obtain your API key:
- Log in to your FreshService account.
- Navigate to your profile by clicking on your profile picture in the top-right corner.
- Select Profile Settings from the dropdown menu.
- Under the API Key section, click on Generate New API Key.
- Copy the generated API key and store it securely, as you will need it to authenticate your API requests.
Configuring FreshService API Access
With your API key ready, you can now configure your application to interact with the FreshService API:
- Ensure you have PHP installed on your system. You can download it from the official PHP website.
- Use the API key in your PHP code to authenticate requests to the FreshService API.
By setting up your FreshService account and generating an API key, you're now ready to start making API calls to create or update departments within FreshService using PHP.
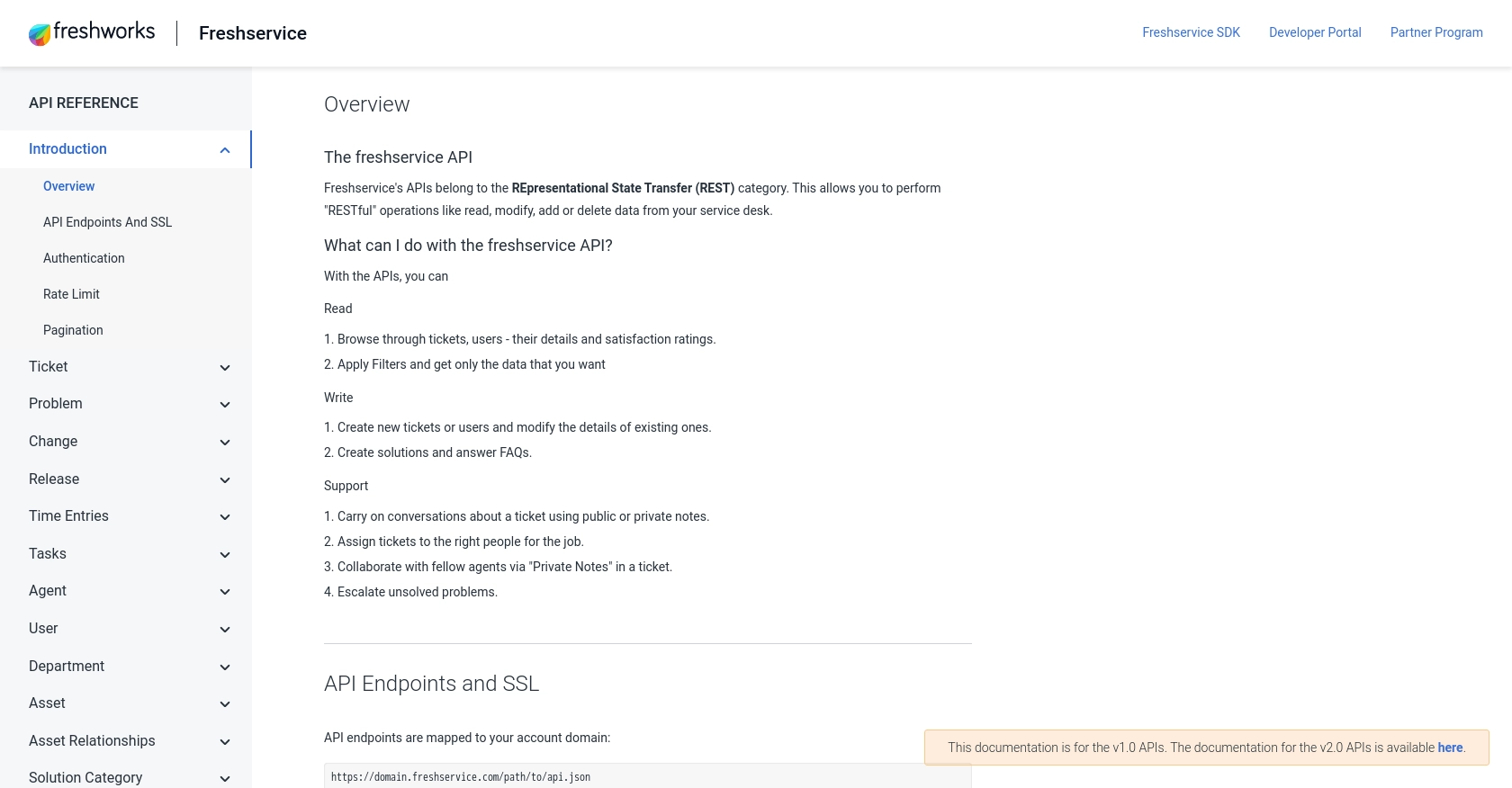
sbb-itb-96038d7
Making API Calls to FreshService for Creating or Updating Departments Using PHP
To interact with the FreshService API and manage departments, you'll need to make HTTP requests using PHP. This section will guide you through the process of setting up your PHP environment, writing the necessary code, and handling responses from the FreshService API.
Setting Up Your PHP Environment for FreshService API Integration
Before you begin coding, ensure that your PHP environment is properly configured:
- Install PHP on your system if it's not already installed. You can download it from the official PHP website.
- Ensure you have access to a text editor or an integrated development environment (IDE) for writing PHP code.
- Install the
cURL
extension for PHP, which is commonly used for making HTTP requests.
Writing PHP Code to Create or Update Departments in FreshService
With your environment ready, you can now write the PHP code to interact with the FreshService API. Below is an example of how to create or update a department:
<?php
// FreshService API endpoint for departments
$apiUrl = "https://yourdomain.freshservice.com/api/v2/departments";
// Your FreshService API key
$apiKey = "your_api_key_here";
// Department data to be created or updated
$data = array(
"department" => array(
"name" => "New Department",
"description" => "Description of the new department"
)
);
// Initialize cURL session
$ch = curl_init($apiUrl);
// Set cURL options
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
"Content-Type: application/json",
"Authorization: Basic " . base64_encode($apiKey . ":X")
));
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($data));
// Execute cURL request
$response = curl_exec($ch);
// Check for errors
if (curl_errno($ch)) {
echo "cURL error: " . curl_error($ch);
} else {
// Decode the response
$responseData = json_decode($response, true);
if (isset($responseData['department'])) {
echo "Department created/updated successfully.";
} else {
echo "Failed to create/update department.";
}
}
// Close cURL session
curl_close($ch);
?>
Verifying API Call Success in FreshService
After executing the PHP script, you can verify the success of your API call by checking the FreshService dashboard:
- Log in to your FreshService account.
- Navigate to the Departments section to see if the new department has been created or updated.
Handling Errors and Troubleshooting FreshService API Calls
When making API calls, it's essential to handle potential errors effectively:
- Check the HTTP response code to determine if the request was successful. A 200 status code indicates success.
- Use
curl_errno()
andcurl_error()
to capture and display any cURL errors. - Review the response data for any error messages returned by the FreshService API.
By following these steps, you can efficiently create or update departments in FreshService using PHP, enhancing your IT service management capabilities.
Conclusion and Best Practices for FreshService API Integration Using PHP
Integrating with the FreshService API to create or update departments can significantly enhance your IT service management workflows. By automating these tasks, you can improve efficiency and ensure that your organizational structures are always up to date.
Best Practices for Secure and Efficient FreshService API Usage
- Securely Store API Credentials: Always store your FreshService API key securely. Avoid hardcoding it in your scripts; instead, use environment variables or secure vaults.
- Handle Rate Limiting: Be aware of any rate limits imposed by FreshService. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Standardize Data Formats: Ensure that the data you send to FreshService is correctly formatted and validated to prevent errors and data inconsistencies.
Enhancing Your Integration Strategy with Endgrate
While integrating with FreshService directly using PHP is effective, managing multiple integrations can become complex. Endgrate offers a streamlined solution by providing a unified API endpoint for various platforms, including FreshService.
By leveraging Endgrate, you can save time and resources, allowing your team to focus on core product development rather than managing individual integrations. Explore how Endgrate can simplify your integration strategy by visiting Endgrate's website.
With these best practices and tools, you can optimize your FreshService API integrations, ensuring a robust and efficient IT service management system.
Read More
Ready to get started?