Using the Monday.com API to Get Board Items in Javascript
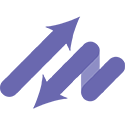
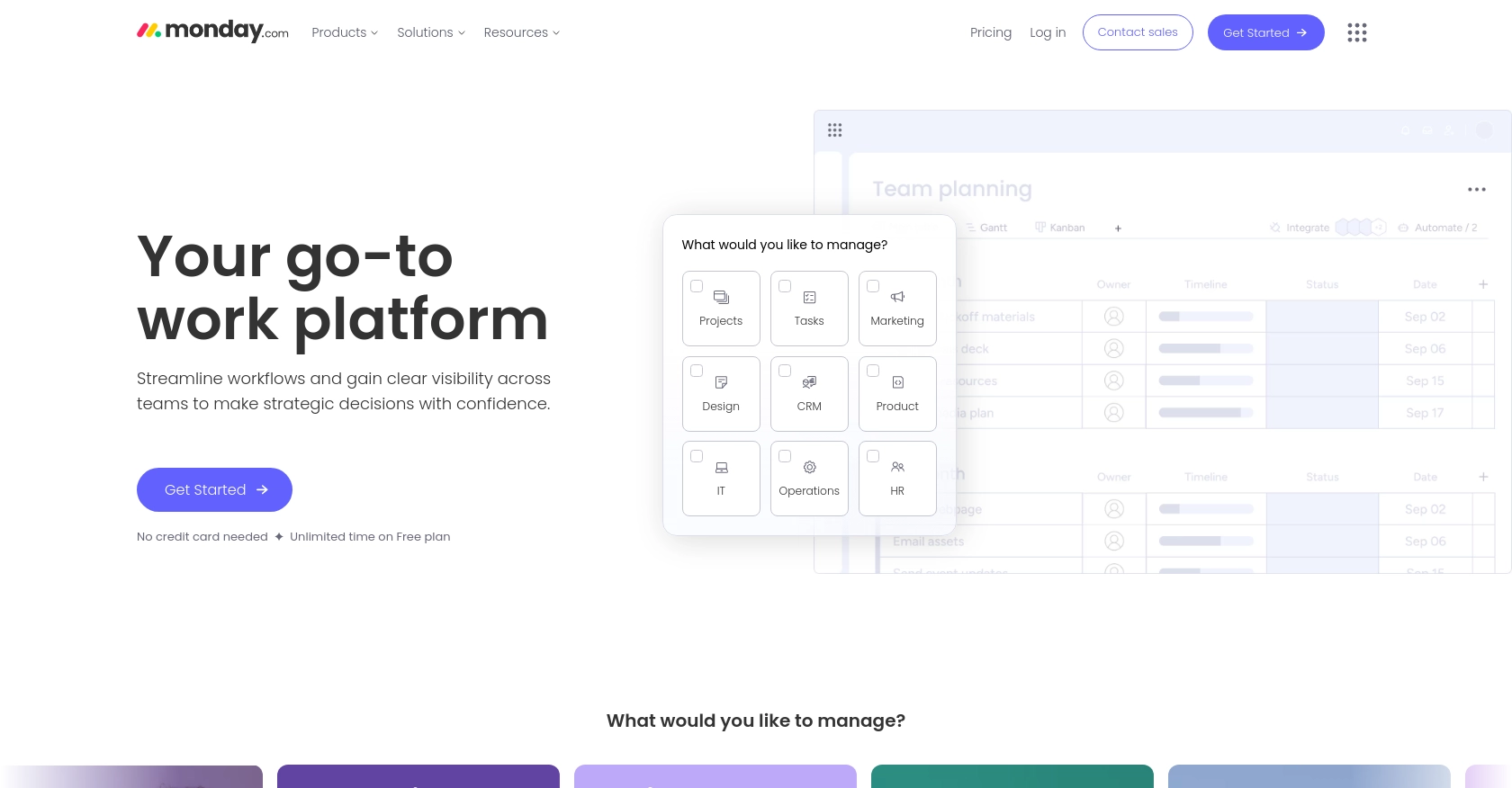
Introduction to Monday.com API Integration
Monday.com is a versatile work operating system that empowers teams to run projects and workflows with confidence. It offers a highly customizable platform where users can manage tasks, projects, and team collaboration in one place. With its intuitive interface and robust features, Monday.com is a popular choice for businesses looking to enhance productivity and streamline operations.
For developers, integrating with the Monday.com API opens up a world of possibilities to automate and enhance workflows. By accessing board items programmatically, developers can create custom solutions that interact with Monday.com data, such as generating reports, syncing data with other applications, or automating repetitive tasks.
In this article, we will explore how to use JavaScript to interact with the Monday.com API to retrieve board items. This integration can be particularly useful for developers looking to build applications that require real-time data from Monday.com boards, such as dashboards or custom notifications.
Setting Up Your Monday.com Account for API Access
Before you can start interacting with the Monday.com API, you'll need to set up your account and obtain the necessary API token. This token will allow you to authenticate your requests and access board items programmatically.
Creating a Monday.com Account
If you don't already have a Monday.com account, you can sign up for a free trial on their website. This will give you access to the platform's features and allow you to explore its capabilities.
- Visit the Monday.com website and click on the "Get Started" button.
- Follow the on-screen instructions to create your account.
- Once your account is set up, log in to access your dashboard.
Accessing Your API Token
To authenticate API requests, you'll need a personal API token. Follow these steps to obtain it:
- Log into your Monday.com account.
- Click on your profile picture in the top right corner.
- Select Administration > Connections > API if you are an admin, or Developers if you are a member user.
- In the Developer Center, click on My Access Tokens > Show to view your personal token.
- Copy the token and store it securely, as you'll need it for API requests.
For more details on accessing API tokens, refer to the Monday.com authentication documentation.
Understanding OAuth Authentication
Monday.com uses OAuth for authentication, which provides a secure way to authorize API requests. Ensure your app has the correct permission scopes to access the necessary data. Personal tokens have all permission scopes, but app tokens require specific scopes to be set.
Testing in a Sandbox Environment
While Monday.com does not provide a dedicated sandbox environment, you can use a free trial account to test API interactions without affecting live data. This approach allows you to experiment with API calls and understand how they work within the Monday.com ecosystem.
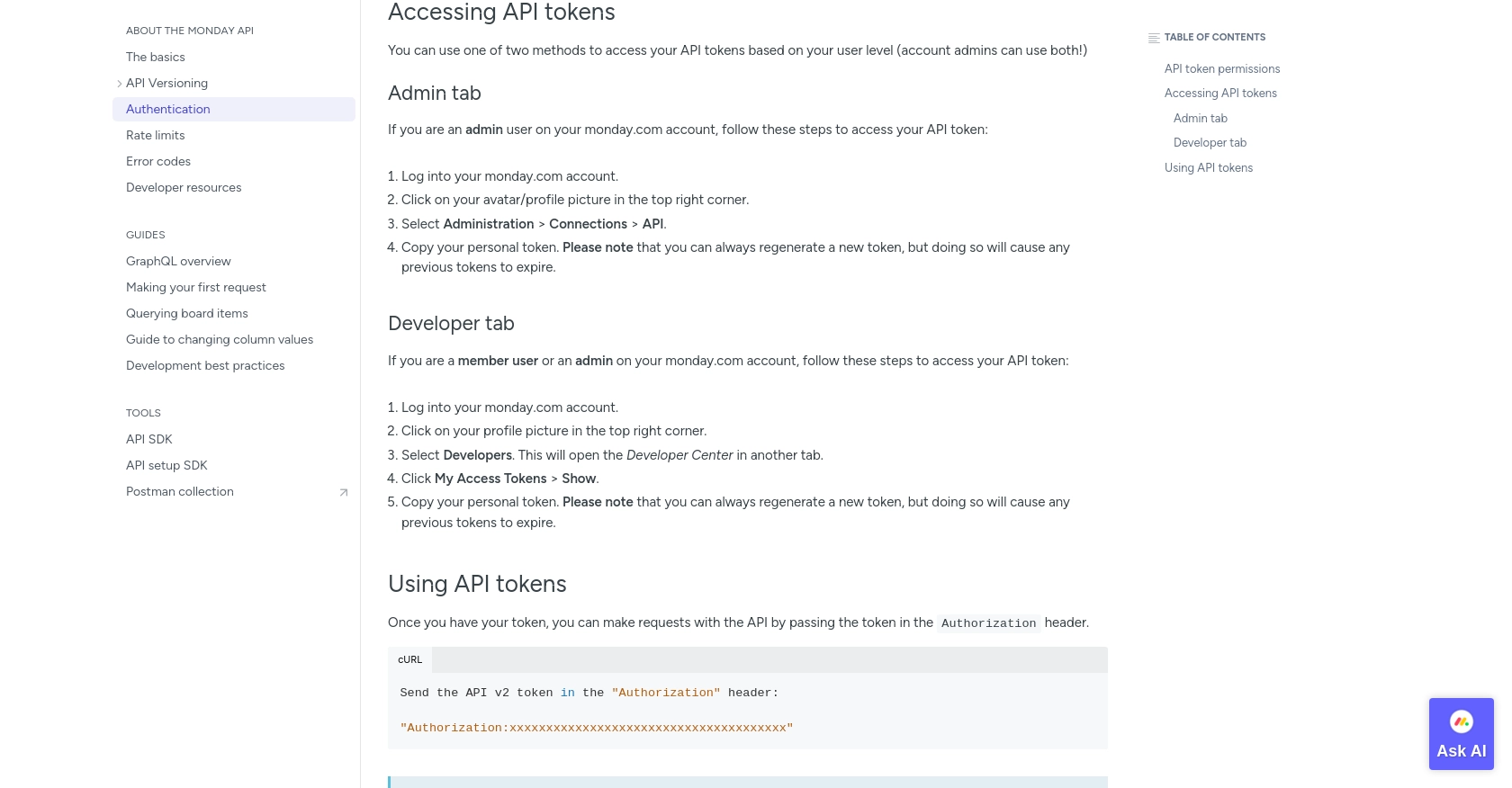
sbb-itb-96038d7
Making API Calls to Retrieve Board Items from Monday.com Using JavaScript
To interact with the Monday.com API and retrieve board items, you'll need to use JavaScript to make HTTP requests. This section will guide you through the process of setting up your environment, writing the necessary code, and handling potential errors.
Setting Up Your JavaScript Environment for Monday.com API
Before making API calls, ensure you have the following prerequisites:
- Node.js installed on your machine.
- A code editor like Visual Studio Code.
- Basic knowledge of JavaScript and HTTP requests.
You'll also need to install the node-fetch
package to make HTTP requests. Run the following command in your terminal:
npm install node-fetch
Writing JavaScript Code to Fetch Board Items from Monday.com
Now, let's write the JavaScript code to retrieve board items using the Monday.com API. Create a new file named getBoardItems.js
and add the following code:
const fetch = require('node-fetch');
// Set the API endpoint and headers
const endpoint = 'https://api.monday.com/v2';
const headers = {
'Content-Type': 'application/json',
'Authorization': 'YOUR_API_TOKEN'
};
// Define the GraphQL query to get board items
const query = `
query {
items (limit: 10) {
id
name
column_values {
id
text
}
}
}
`;
// Make a POST request to the API
fetch(endpoint, {
method: 'POST',
headers: headers,
body: JSON.stringify({ query: query })
})
.then(response => response.json())
.then(data => {
console.log(JSON.stringify(data, null, 2));
})
.catch(error => {
console.error('Error fetching board items:', error);
});
Replace YOUR_API_TOKEN
with the personal API token you obtained earlier. This code sends a GraphQL query to the Monday.com API to fetch the first 10 board items and their column values.
Verifying Successful API Requests and Handling Errors
After running the code, you should see the board items printed in the console. To verify the request's success, check the response data for the expected items.
Monday.com API provides detailed error codes to help you troubleshoot issues. Here are some common errors and how to handle them:
- Unauthorized (401): Ensure your API token is valid and included in the headers.
- Rate Limit Exceeded (429): Reduce the number of requests or implement a retry mechanism. Refer to the rate limits documentation for more details.
- Internal Server Error (500): Check for invalid arguments or malformed JSON. If the issue persists, contact Monday.com support.
For a comprehensive list of error codes, visit the Monday.com error codes documentation.
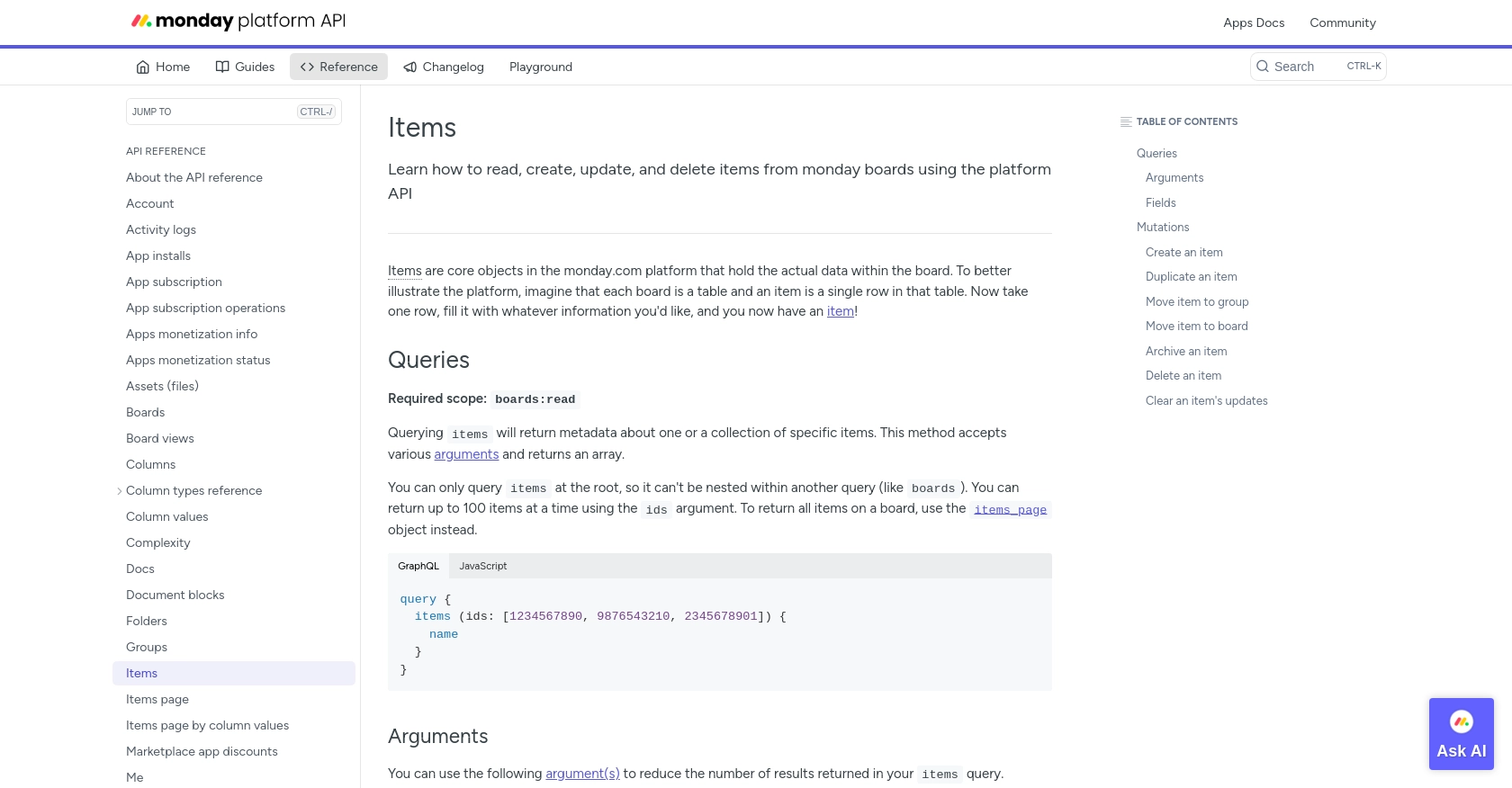
Conclusion and Best Practices for Using the Monday.com API in JavaScript
Integrating with the Monday.com API using JavaScript provides developers with powerful capabilities to automate workflows and enhance productivity. By retrieving board items programmatically, you can create custom applications that interact seamlessly with Monday.com data, offering real-time insights and automation.
Best Practices for Secure and Efficient Monday.com API Integration
- Secure API Tokens: Always store your API tokens securely and avoid hardcoding them in your source code. Consider using environment variables or secure vaults to manage sensitive information.
- Handle Rate Limits: Be mindful of the API rate limits to avoid disruptions. Implement retry mechanisms and optimize your queries to stay within the allowed limits. For more details, refer to the rate limits documentation.
- Optimize Data Retrieval: Use pagination and limit the number of items returned in your queries to reduce complexity and improve performance. This approach helps in managing large datasets efficiently.
- Error Handling: Implement robust error handling to manage API errors gracefully. Use the detailed error codes provided by Monday.com to troubleshoot and resolve issues effectively. Visit the error codes documentation for more information.
Streamline Your Integrations with Endgrate
While integrating with the Monday.com API can be rewarding, it may also require significant time and resources. Endgrate offers a solution by providing a unified API endpoint that connects to multiple platforms, including Monday.com. This allows developers to focus on core product development while outsourcing integrations, saving time and capital.
With Endgrate, you can build once for each use case and enjoy an intuitive integration experience for your customers. Explore how Endgrate can simplify your integration needs by visiting Endgrate.
Read More
Ready to get started?