How to Get Companies with the Hubspot API in Javascript
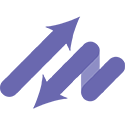
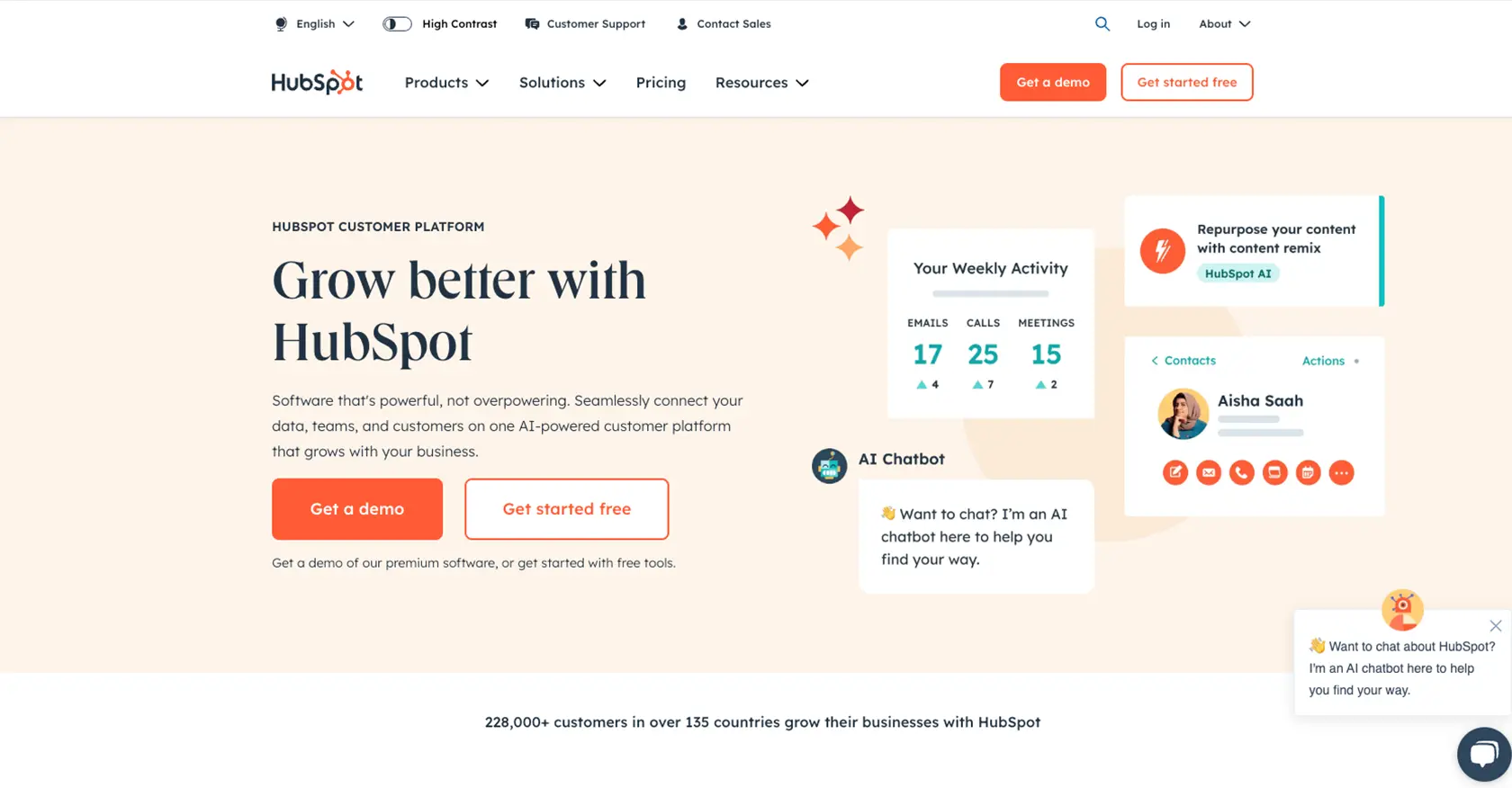
Introduction to HubSpot API Integration for Companies
HubSpot is a comprehensive CRM platform that offers a wide range of tools to help businesses manage their marketing, sales, and customer service efforts. Known for its user-friendly interface and robust features, HubSpot is a popular choice for businesses looking to streamline their operations and improve customer relationships.
For developers, integrating with HubSpot's API can unlock powerful capabilities, such as accessing and managing company data. By connecting to the HubSpot API, developers can automate processes like retrieving company information, which can be used to enhance business intelligence and streamline workflows.
For example, a developer might want to use the HubSpot API to fetch a list of companies and their details to integrate with a custom analytics dashboard. This integration can provide real-time insights into company interactions, helping businesses make data-driven decisions.
Setting Up Your HubSpot Developer Account for API Integration
Before you can start interacting with the HubSpot API to retrieve company data, you'll need to set up a developer account. This account will allow you to create a private app and obtain the necessary credentials for OAuth authentication.
Creating a HubSpot Developer Account
- Visit the HubSpot Developer Portal and click on "Create a developer account."
- Fill in the required details, such as your name, email, and password, to register for a new account.
- Once registered, log in to your developer account to access the HubSpot dashboard.
Setting Up a HubSpot Sandbox Environment
To test your API integrations without affecting live data, it's recommended to use a sandbox environment. Follow these steps to set up a sandbox:
- In your HubSpot developer account, navigate to the "Test Accounts" section.
- Click on "Create a test account" to generate a sandbox environment.
- Use this sandbox to safely test API calls and integrations.
Creating a HubSpot App for OAuth Authentication
HubSpot uses OAuth for secure API authentication. To set up OAuth, you need to create a private app:
- In your HubSpot account, go to "Settings" and select "Integrations" from the sidebar.
- Click on "Private Apps" and then "Create a private app."
- Provide a name and description for your app, then proceed to configure the scopes.
- Under "Scopes," ensure you select
crm.objects.companies.read
to access company data. - Click "Create app" to generate your OAuth credentials, including the client ID and client secret.
- Save these credentials securely, as they will be used to authenticate API requests.
Obtaining OAuth Access Tokens
With your app created, you can now obtain OAuth access tokens:
- Use the client ID and client secret to initiate the OAuth flow. This typically involves redirecting users to a HubSpot authorization URL.
- Upon user authorization, you'll receive an authorization code, which you can exchange for an access token.
- Use this access token in the authorization header of your API requests to authenticate.
For more detailed information on OAuth setup, refer to the HubSpot OAuth Documentation.
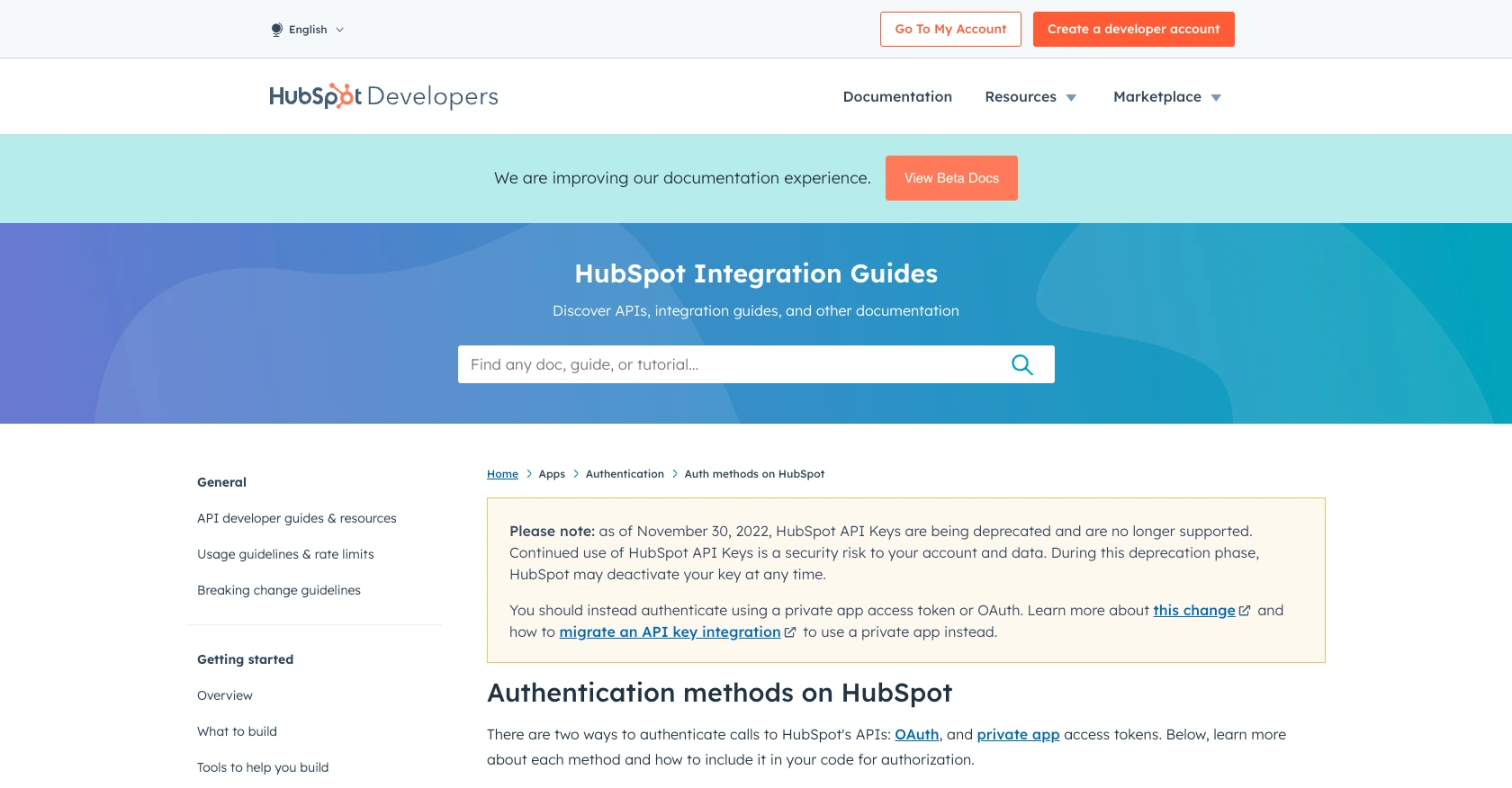
sbb-itb-96038d7
Making API Calls to Retrieve Companies with HubSpot API in JavaScript
To interact with the HubSpot API using JavaScript, you'll need to ensure you have the right environment and dependencies set up. This section will guide you through the process of making API calls to retrieve company data from HubSpot.
Setting Up Your JavaScript Environment for HubSpot API
Before you start coding, make sure you have Node.js installed on your machine. Node.js allows you to run JavaScript code outside of a web browser, which is essential for server-side operations like API calls.
- Download and install Node.js from the official website.
- Once installed, open your terminal and verify the installation by running
node -v
andnpm -v
. - Create a new project directory and navigate into it using
mkdir hubspot-api-integration && cd hubspot-api-integration
. - Initialize a new Node.js project by running
npm init -y
.
Installing Required Dependencies for HubSpot API Integration
You'll need the axios
library to make HTTP requests to the HubSpot API. Install it using npm:
npm install axios
Writing JavaScript Code to Fetch Companies from HubSpot
Now that your environment is set up, you can write the code to fetch company data from HubSpot. Create a new file named getCompanies.js
and add the following code:
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://api.hubapi.com/crm/v3/objects/companies';
const headers = {
'Authorization': 'Bearer YOUR_ACCESS_TOKEN',
'Content-Type': 'application/json'
};
// Function to get companies
async function getCompanies() {
try {
const response = await axios.get(endpoint, { headers });
const companies = response.data.results;
companies.forEach(company => {
console.log(`Company Name: ${company.properties.name}, Domain: ${company.properties.domain}`);
});
} catch (error) {
console.error('Error fetching companies:', error.response ? error.response.data : error.message);
}
}
// Execute the function
getCompanies();
Replace YOUR_ACCESS_TOKEN
with the OAuth access token you obtained earlier. This token is crucial for authenticating your requests to the HubSpot API.
Running Your JavaScript Code to Retrieve HubSpot Companies
To execute your code and fetch company data, run the following command in your terminal:
node getCompanies.js
If successful, you should see a list of companies with their names and domains printed in the console. This confirms that your API call was successful and that you have retrieved the desired data from HubSpot.
Handling Errors and Verifying API Call Success
In the code above, error handling is implemented using a try-catch
block. If an error occurs during the API call, it will be logged to the console. Common errors include authentication issues or exceeding rate limits.
To verify the success of your API call, you can cross-check the retrieved data with the records in your HubSpot sandbox environment. Ensure that the data matches and that the API call is fetching the correct information.
For more details on error codes and handling, refer to the HubSpot API documentation.
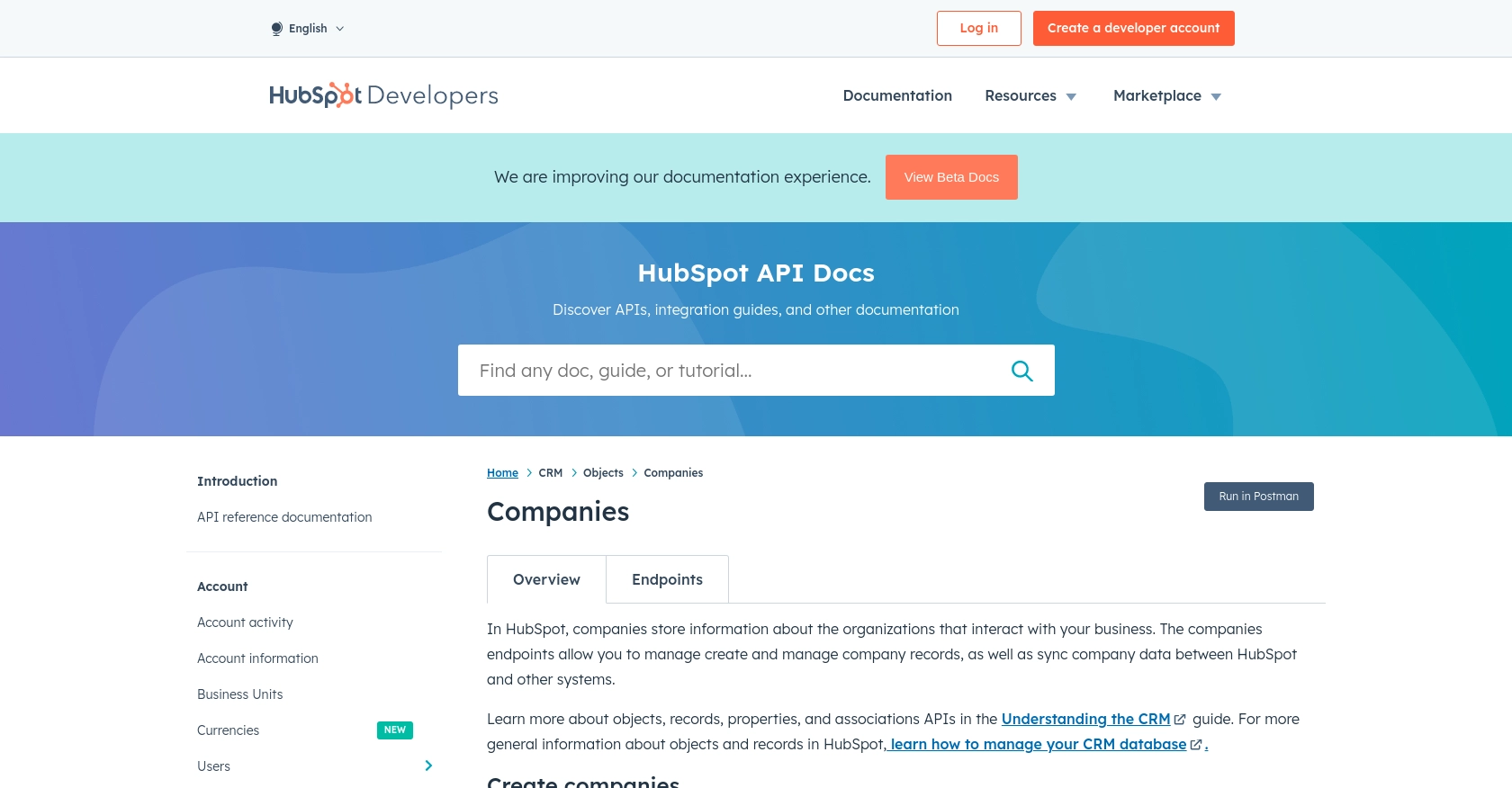
Conclusion and Best Practices for HubSpot API Integration in JavaScript
Integrating with the HubSpot API using JavaScript provides developers with powerful tools to access and manage company data efficiently. By following the steps outlined in this guide, you can set up a seamless connection to HubSpot, enabling you to automate workflows and enhance your business intelligence capabilities.
Best Practices for Secure and Efficient HubSpot API Usage
- Securely Store Credentials: Always store your OAuth credentials, such as client ID and client secret, securely. Avoid hardcoding them in your source code. Consider using environment variables or secure vaults.
- Handle Rate Limits: HubSpot imposes rate limits on API requests. For OAuth apps, the limit is 100 requests every 10 seconds per HubSpot account. Implement retry logic and exponential backoff strategies to handle 429 rate limit errors gracefully. For more details, refer to the HubSpot API usage guidelines.
- Data Transformation and Standardization: When retrieving data, ensure that it is transformed and standardized to fit your application's requirements. This will help maintain consistency across different integrations.
- Monitor API Usage: Regularly monitor your API usage to ensure compliance with HubSpot's guidelines and to optimize your integration's performance.
Streamlining Integrations with Endgrate
While building custom integrations with HubSpot can be rewarding, it can also be time-consuming and complex. Endgrate simplifies this process by providing a unified API endpoint that connects to multiple platforms, including HubSpot. With Endgrate, you can:
- Save time and resources by outsourcing integrations and focusing on your core product.
- Build once for each use case instead of multiple times for different integrations.
- Offer an intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate's website and discover the benefits of a streamlined integration process.
Read More
- https://endgrate.com/provider/hubspot
- https://developers.hubspot.com/docs/api/overview
- https://developers.hubspot.com/docs/api/intro-to-auth
- https://developers.hubspot.com/docs/api/usage-details
- https://developers.hubspot.com/docs/api/scopes
- https://developers.hubspot.com/docs/api/crm/companies
- https://developers.hubspot.com/docs/api/crm/properties
Ready to get started?