How to Create or Update Applicants with the JazzHR API in Python
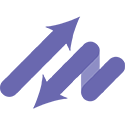
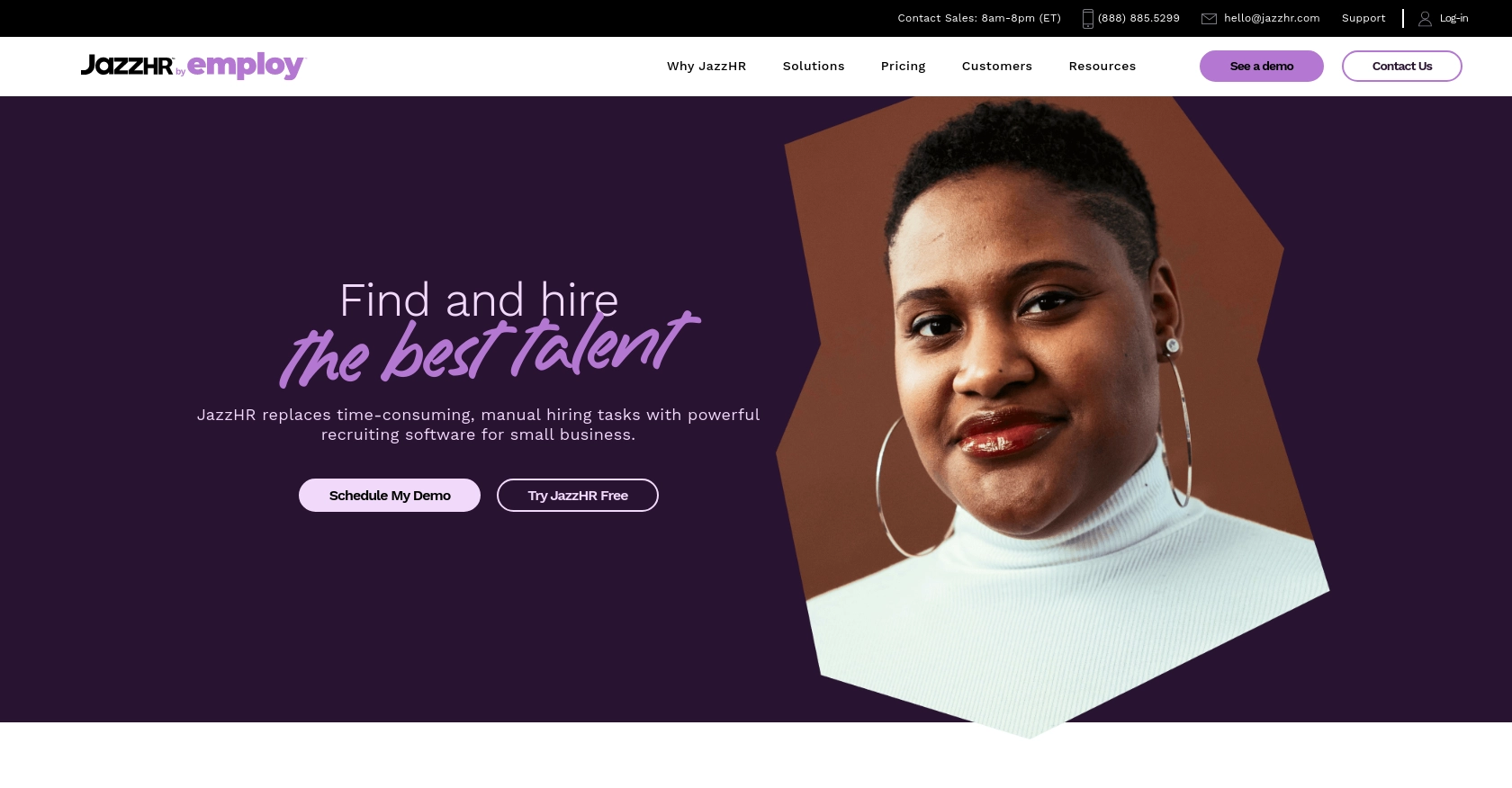
Introduction to JazzHR API Integration
JazzHR is a powerful recruiting software solution that simplifies the hiring process for businesses of all sizes. With features like job posting, candidate tracking, and interview scheduling, JazzHR helps organizations streamline their recruitment efforts and find the best talent efficiently.
Integrating with the JazzHR API allows developers to automate and enhance recruitment workflows. For example, you can create or update applicant information directly from your application, ensuring that your recruitment data is always up-to-date and synchronized across platforms.
This article will guide you through the process of using Python to interact with the JazzHR API, specifically focusing on creating or updating applicants. By following this tutorial, you can seamlessly integrate JazzHR's capabilities into your software solutions, enhancing your recruitment processes.
Setting Up Your JazzHR Test Account for API Integration
Before you can start interacting with the JazzHR API, you'll need to set up a JazzHR account and obtain your API key. This key is essential for authenticating your requests and ensuring secure communication with the JazzHR platform.
Creating a JazzHR Account
If you don't already have a JazzHR account, you can sign up for a free trial or a demo account on the JazzHR website. This will give you access to the necessary tools and features to explore the API capabilities.
- Visit the JazzHR website and click on the "Get Started" button.
- Follow the on-screen instructions to create your account.
- Once your account is set up, log in to access the JazzHR dashboard.
Generating Your JazzHR API Key
To authenticate your API requests, you'll need to generate an API key from your JazzHR account. Follow these steps to obtain your key:
- Log in to your JazzHR account and navigate to the "Integrations" section.
- Locate the API key section and generate a new API key.
- Copy the API key and store it securely, as you'll need it for making API calls.
For more detailed information on generating your API key, you can refer to the JazzHR API Overview.
Understanding API Rate Limits
It's important to be aware of the API rate limits to ensure your application functions smoothly. According to the JazzHR API documentation, each API call is limited to 100 results per page. If you need to access more results, you can paginate through the data by appending "/page/#" to the request URI, where "#" is the page number.
With your JazzHR account set up and your API key ready, you're now prepared to start integrating with the JazzHR API using Python. In the next section, we'll walk through the process of making API calls to create or update applicants.
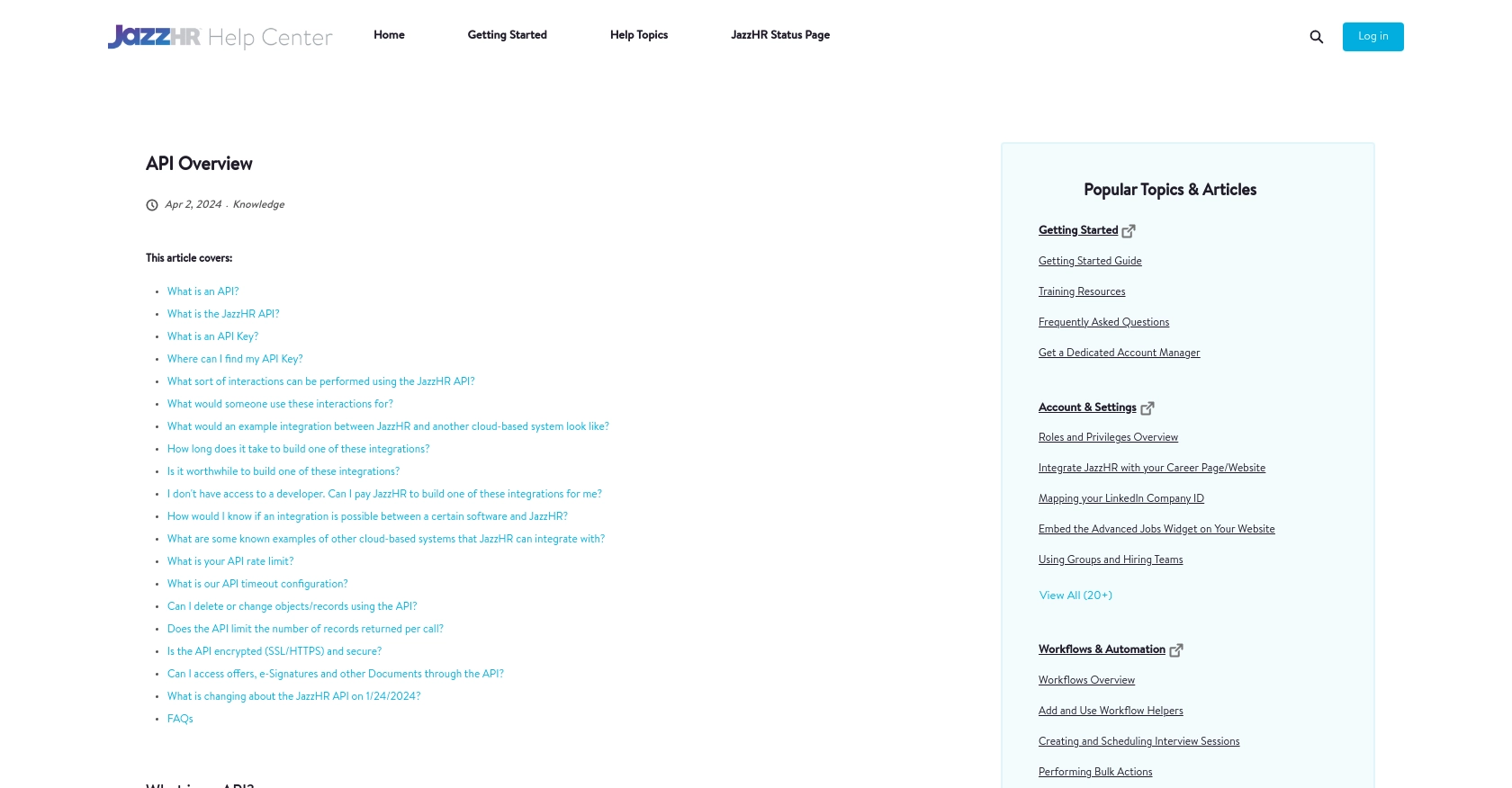
sbb-itb-96038d7
Making API Calls to Create or Update Applicants with JazzHR in Python
Now that you have your JazzHR account set up and your API key ready, it's time to dive into making API calls using Python. This section will guide you through the steps to create or update applicants in JazzHR, ensuring your recruitment data is efficiently managed.
Prerequisites for Using Python with JazzHR API
Before proceeding, ensure you have the following installed on your machine:
- Python 3.11.1 or later
- The Python package installer
pip
Once you have these prerequisites, install the requests
library, which will be used to make HTTP requests to the JazzHR API:
pip install requests
Creating or Updating Applicants with JazzHR API
To create or update applicants, you'll need to make a POST request to the JazzHR API endpoint. Below is a sample Python script to help you get started:
import requests
# Set the API endpoint
url = "https://api.resumatorapi.com/v1/applicants"
# Set the request headers
headers = {
"Content-Type": "application/json",
"Authorization": "Bearer Your_API_Key"
}
# Define the applicant data
applicant_data = {
"first_name": "John",
"last_name": "Doe",
"email": "john.doe@example.com",
"phone": "555-555-5555",
"position": "Software Engineer"
}
# Make the POST request to create or update the applicant
response = requests.post(url, json=applicant_data, headers=headers)
# Check if the request was successful
if response.status_code == 200:
print("Applicant created or updated successfully.")
else:
print(f"Failed to create or update applicant. Status code: {response.status_code}")
Replace Your_API_Key
with the API key you generated from your JazzHR account.
Verifying the API Request Success
After running the script, you should see a confirmation message indicating the applicant was created or updated successfully. To verify, log in to your JazzHR account and check the applicants' section to ensure the data reflects the changes.
Handling Errors and Common Error Codes
When making API calls, it's crucial to handle potential errors gracefully. The JazzHR API may return various error codes, such as:
- 400 Bad Request: The request was invalid. Check your data format and required fields.
- 401 Unauthorized: Authentication failed. Verify your API key.
- 404 Not Found: The requested resource could not be found.
- 500 Internal Server Error: An error occurred on the server. Try again later.
Implement error handling in your code to manage these scenarios and ensure a smooth integration experience.
Best Practices for JazzHR API Integration
Successfully integrating with the JazzHR API requires attention to best practices to ensure security, efficiency, and reliability. Here are some recommendations to enhance your integration:
- Securely Store API Keys: Always store your JazzHR API key securely. Avoid hardcoding it in your source code. Use environment variables or secure vaults to manage sensitive information.
- Implement Rate Limiting: Be mindful of the API rate limits. JazzHR allows 100 results per page. Implement pagination and handle rate limiting gracefully to avoid disruptions.
- Standardize Data Fields: Ensure that the data you send to JazzHR is standardized and validated. This helps maintain data integrity and prevents errors during API calls.
- Error Handling: Implement robust error handling in your code to manage potential API errors. Log errors for troubleshooting and provide meaningful feedback to users.
Enhance Your Integration Experience with Endgrate
Building and maintaining multiple integrations can be time-consuming and complex. Endgrate simplifies this process by offering a unified API endpoint that connects to various platforms, including JazzHR. By leveraging Endgrate, you can:
- Save Time and Resources: Focus on your core product while Endgrate handles the complexities of integration.
- Build Once, Use Everywhere: Develop a single integration for each use case and apply it across multiple platforms.
- Provide a Seamless Experience: Offer your customers an intuitive and efficient integration experience.
Explore how Endgrate can transform your integration strategy by visiting Endgrate today.
Read More
Ready to get started?