Using the Zendesk Support API to Get Organizations in PHP
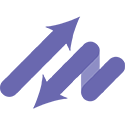
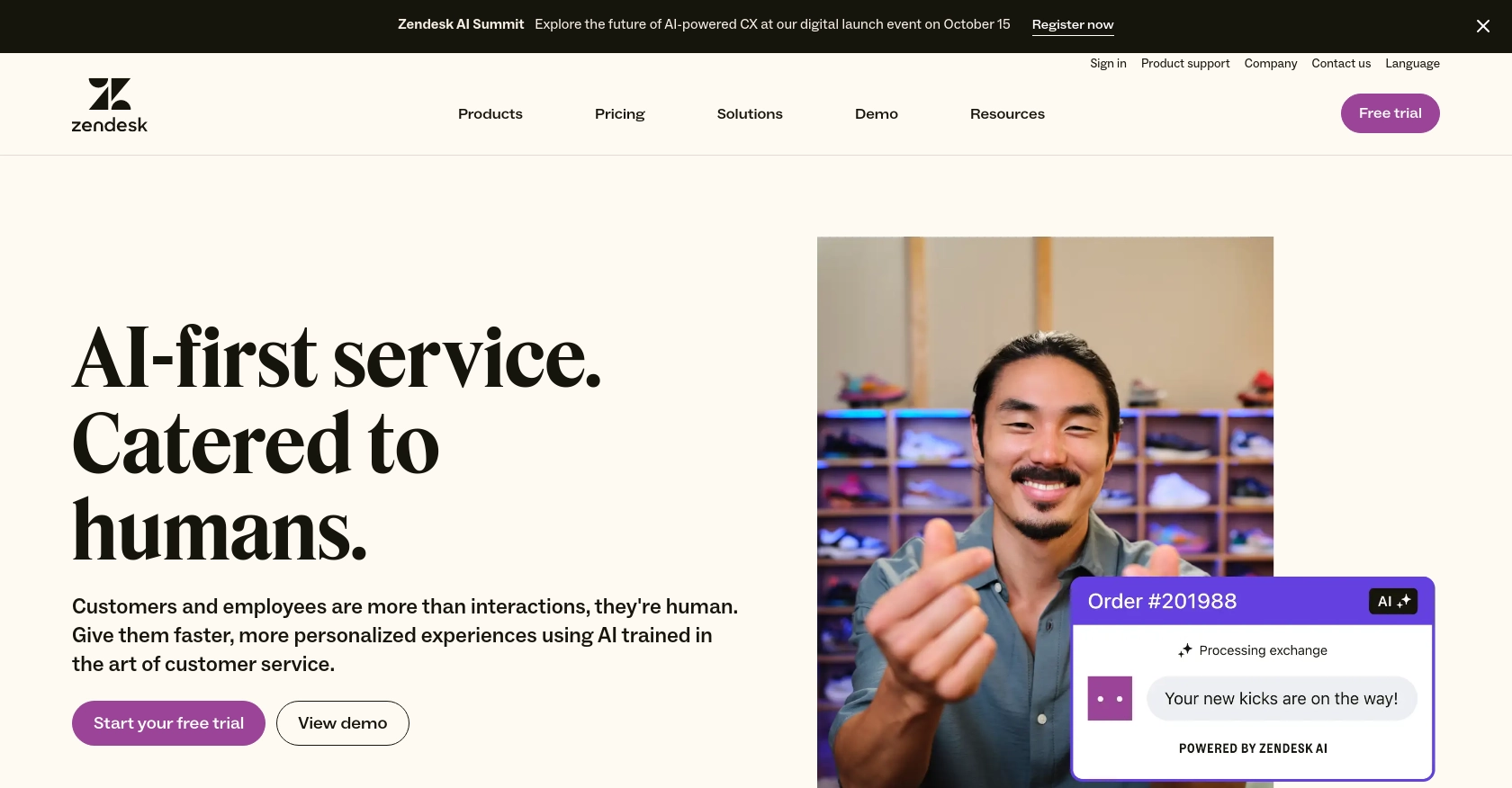
Introduction to Zendesk Support API
Zendesk Support is a robust customer service platform that helps businesses manage customer interactions across various channels. It offers a suite of tools designed to enhance customer support, streamline workflows, and improve customer satisfaction.
Developers often integrate with the Zendesk Support API to access and manage customer data, such as organizations, tickets, and users. This integration allows for automation and customization of support processes, enabling businesses to provide more personalized and efficient customer service.
For example, a developer might use the Zendesk Support API to retrieve a list of organizations associated with a customer account. This can be useful for generating reports, analyzing customer data, or integrating with other business systems to provide a seamless customer experience.
Setting Up Your Zendesk Support Test/Sandbox Account
Before diving into the integration with Zendesk Support API, it's essential to set up a test or sandbox account. This environment allows developers to experiment and test API interactions without affecting live data.
Creating a Zendesk Support Sandbox Account
If you don't already have a Zendesk account, you can sign up for a free trial or a sandbox account. Follow these steps to create one:
- Visit the Zendesk registration page.
- Fill in the required details, such as your name, email, and company information.
- Choose the option for a sandbox or trial account to ensure you have a testing environment.
- Complete the registration process and verify your email address.
Setting Up OAuth Authentication for Zendesk Support API
Zendesk Support API uses OAuth for secure authentication. Follow these steps to set up OAuth for your application:
- Log in to your Zendesk Admin Center.
- Navigate to Apps and integrations > APIs > Zendesk API.
- Click on the OAuth Clients tab and then Add OAuth client.
- Fill in the necessary fields:
- Client Name: A recognizable name for your app.
- Description: (Optional) A brief description of your app.
- Redirect URLs: The URL where Zendesk will send the authorization code.
- Click Save to generate your Client ID and Client Secret. Make sure to store these securely.
Generating an OAuth Access Token
Once your OAuth client is set up, you can generate an access token:
- Direct users to the Zendesk authorization page using the following URL format:
https://{subdomain}.zendesk.com/oauth/authorizations/new?response_type=code&redirect_uri={your_redirect_url}&client_id={your_client_id}&scope=read%20write
- After the user authorizes the application, Zendesk will redirect to the specified URL with an authorization code.
- Exchange this code for an access token by making a POST request:
curl -X POST https://{subdomain}.zendesk.com/oauth/tokens \ -H "Content-Type: application/json" \ -d '{ "grant_type": "authorization_code", "code": "{authorization_code}", "client_id": "{your_client_id}", "client_secret": "{your_client_secret}", "redirect_uri": "{your_redirect_url}" }'
For more detailed information on OAuth setup, refer to the Zendesk OAuth documentation.
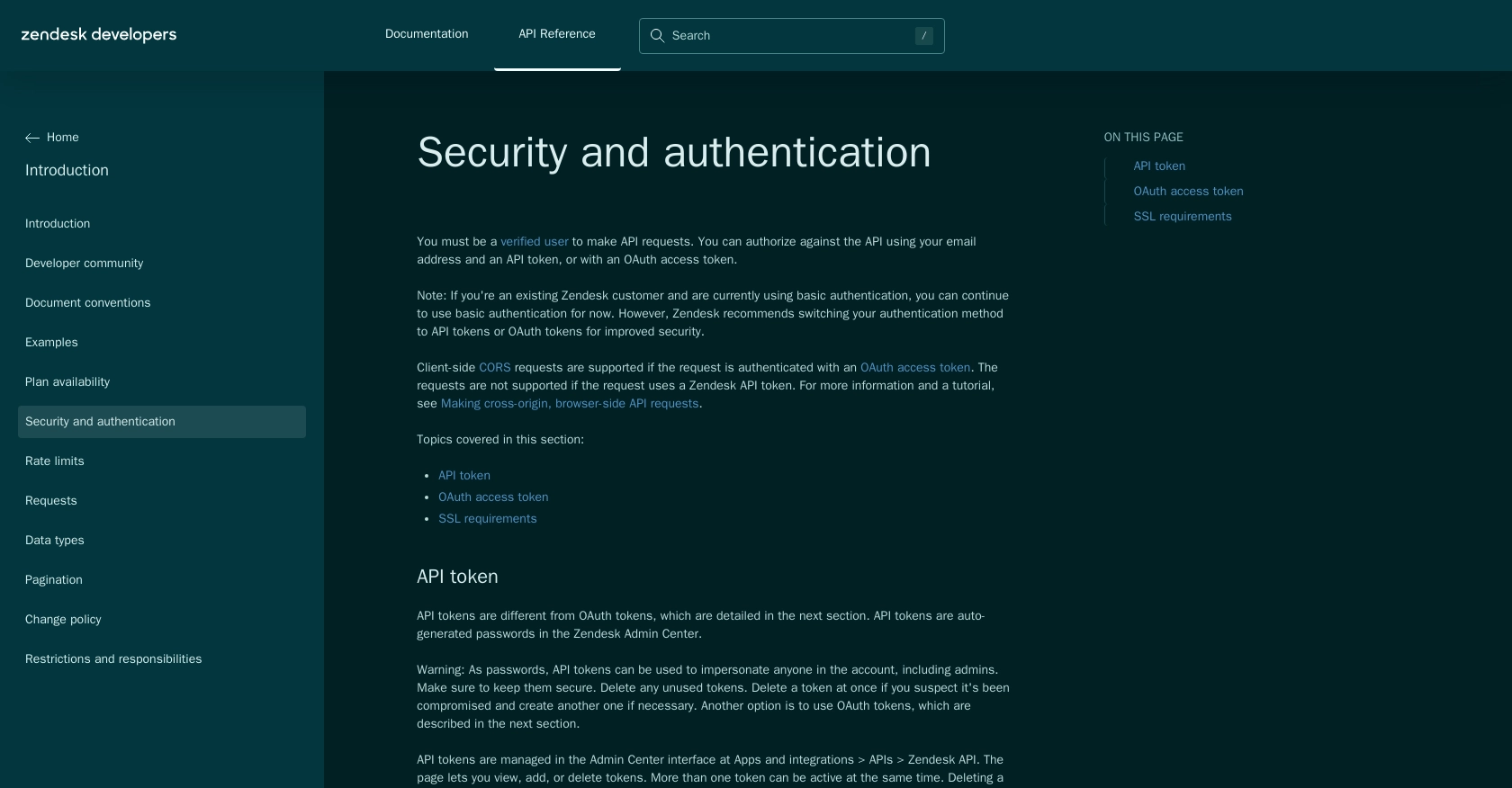
sbb-itb-96038d7
How to Make an API Call to Retrieve Organizations Using Zendesk Support API in PHP
To interact with the Zendesk Support API and retrieve a list of organizations, you'll need to set up your PHP environment and make authenticated requests. This section will guide you through the necessary steps, including setting up PHP, installing dependencies, and executing the API call.
Setting Up PHP Environment for Zendesk Support API
Ensure you have PHP installed on your machine. This tutorial assumes you are using PHP 7.4 or later. You will also need Composer, the PHP package manager, to handle dependencies.
- Download and install Composer if you haven't already.
- Create a new project directory and navigate into it:
mkdir zendesk-api-integration cd zendesk-api-integration
composer init
composer require guzzlehttp/guzzle
Making the API Call to Zendesk Support to Get Organizations
With your environment set up, you can now write a PHP script to make the API call. This script will use the Guzzle HTTP client to send a GET request to the Zendesk Support API endpoint for organizations.
<?php require 'vendor/autoload.php'; use GuzzleHttp\Client; use GuzzleHttp\Exception\RequestException; // Set your Zendesk subdomain and access token $subdomain = 'your_subdomain'; $accessToken = 'your_access_token'; // Create a new Guzzle client $client = new Client(); try { // Make the GET request to the Zendesk Support API $response = $client->request('GET', "https://{$subdomain}.zendesk.com/api/v2/organizations.json", [ 'headers' => [ 'Authorization' => "Bearer {$accessToken}", 'Content-Type' => 'application/json', ] ]); // Parse the JSON response $data = json_decode($response->getBody(), true); // Output the list of organizations foreach ($data['organizations'] as $organization) { echo "Organization ID: " . $organization['id'] . "\n"; echo "Name: " . $organization['name'] . "\n\n"; } } catch (RequestException $e) { // Handle errors echo "Error: " . $e->getMessage(); } ?>
Replace your_subdomain
and your_access_token
with your actual Zendesk subdomain and OAuth access token. This script sends a GET request to the organizations endpoint and prints out the ID and name of each organization.
Verifying the API Call and Handling Errors
After running the script, you should see a list of organizations printed to your console. If the request fails, the script will output an error message. Common errors include:
- 401 Unauthorized: Check your access token and ensure it has the correct permissions.
- 429 Too Many Requests: You have exceeded the rate limit. Refer to the Zendesk rate limits documentation for more information.
For further troubleshooting, you can log the full response or error details to understand the issue better.
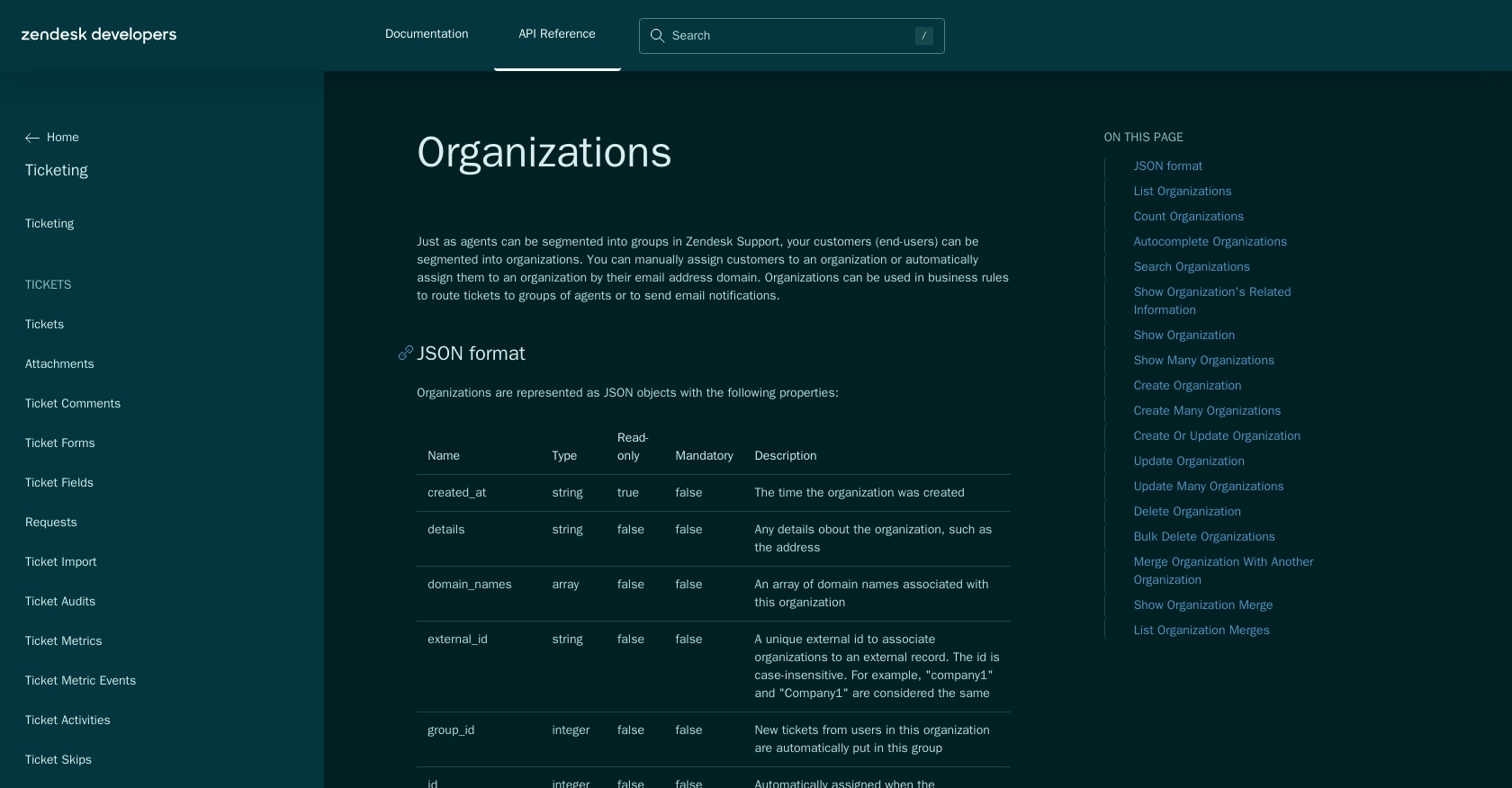
Conclusion and Best Practices for Using Zendesk Support API in PHP
Integrating with the Zendesk Support API allows developers to efficiently manage and access customer data, enhancing the overall customer service experience. By following the steps outlined in this guide, you can successfully retrieve organization data using PHP, enabling seamless integration with other business systems.
Best Practices for Secure and Efficient Zendesk Support API Integration
- Secure Storage of Credentials: Always store your OAuth client ID, client secret, and access tokens securely. Consider using environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Be mindful of Zendesk's rate limits, which are 700 requests per minute. Implement logic to handle 429 Too Many Requests errors by waiting for the specified Retry-After interval before retrying.
- Data Standardization: Ensure that data retrieved from Zendesk is standardized and transformed as needed to fit your application's requirements, maintaining consistency across systems.
Enhance Your Integration Strategy with Endgrate
While building integrations with Zendesk Support API can be rewarding, it can also be time-consuming and complex. Endgrate offers a streamlined solution for managing multiple integrations through a single API endpoint. By leveraging Endgrate, you can save time and resources, allowing your team to focus on core product development while providing an intuitive integration experience for your customers.
Explore how Endgrate can simplify your integration processes by visiting Endgrate today.
Read More
- https://endgrate.com/provider/zendesksupport
- https://developer.zendesk.com/api-reference/introduction/security-and-auth/
- https://support.zendesk.com/hc/en-us/articles/4408845965210-Using-OAuth-authentication-with-your-application
- https://developer.zendesk.com/api-reference/introduction/rate-limits/
- https://developer.zendesk.com/api-reference/introduction/pagination/
- https://developer.zendesk.com/api-reference/ticketing/organizations/organizations/
Ready to get started?