Using the Zendesk Sell API to Create or Update Leads (with Javascript examples)
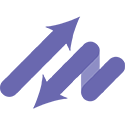
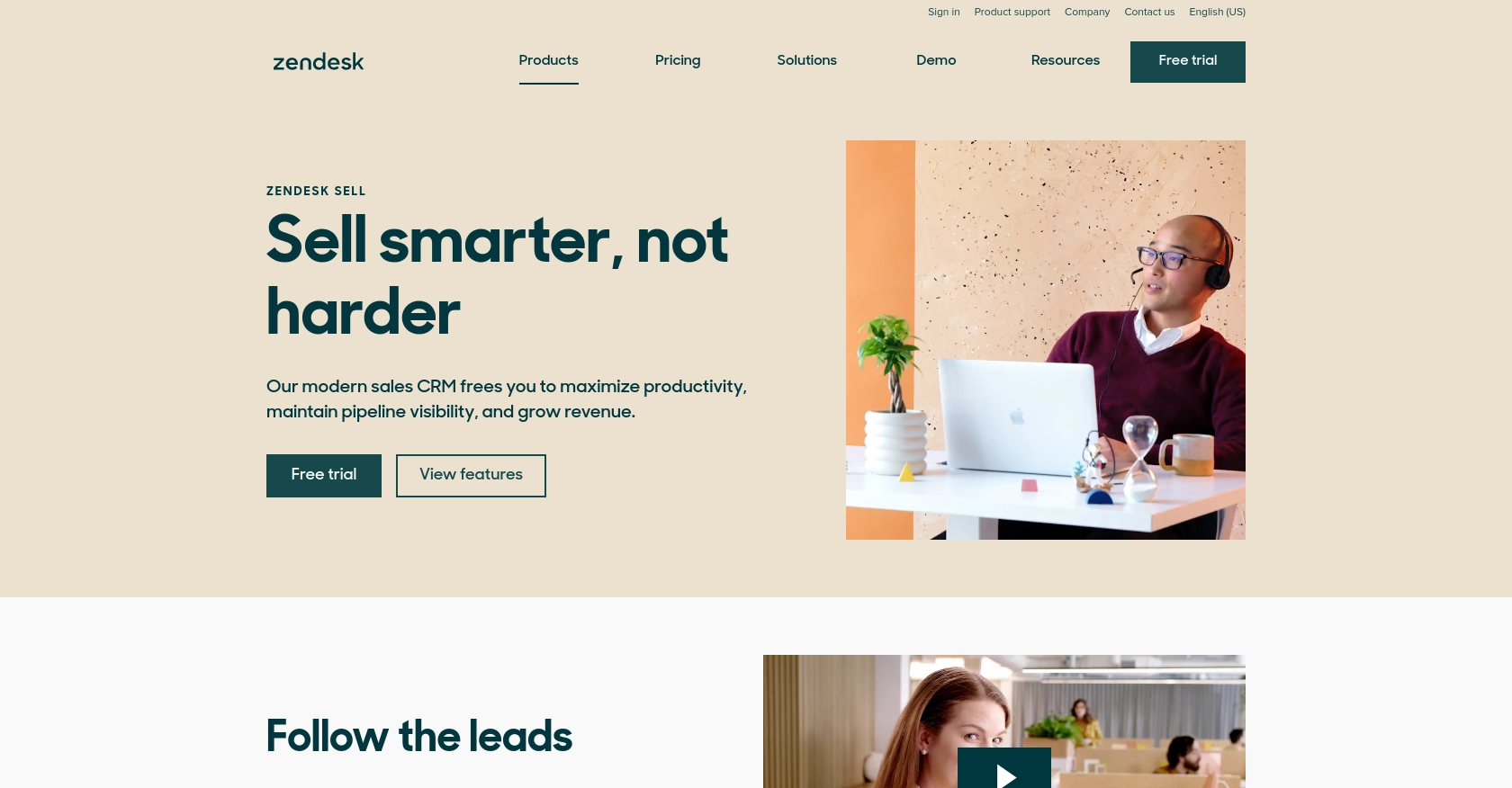
Introduction to Zendesk Sell API
Zendesk Sell is a powerful sales CRM platform designed to enhance productivity and streamline sales processes for businesses. It offers a comprehensive suite of tools to manage leads, contacts, and deals, making it an essential tool for sales teams looking to optimize their workflow.
Developers may want to integrate with the Zendesk Sell API to automate lead management tasks, such as creating or updating leads directly from their applications. For example, a developer could use the Zendesk Sell API to automatically update lead information from a web form, ensuring that sales teams have the most current data at their fingertips.
Setting Up Your Zendesk Sell Test Account
Before you can start integrating with the Zendesk Sell API, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting live data.
Creating a Zendesk Sell Sandbox Account
If you don't already have a Zendesk Sell account, you can sign up for a free trial or demo account on the Zendesk Sell website. This will give you access to a sandbox environment where you can test API interactions.
- Visit the Zendesk Sell website and click on the "Free Trial" button.
- Follow the instructions to create your account. Once completed, you'll have access to the Zendesk Sell dashboard.
Setting Up OAuth Authentication for Zendesk Sell API
The Zendesk Sell API uses OAuth 2.0 for authentication. Follow these steps to create an app and obtain the necessary credentials:
- Log in to your Zendesk Sell account and navigate to the "Settings" section.
- Under "Integrations," select "API & Apps" and click on "Create App."
- Fill in the required details for your app, such as the app name and description.
- After creating the app, you'll receive a Client ID and Client Secret. Keep these credentials secure as they are essential for API authentication.
Obtaining Access Tokens for API Requests
To interact with the Zendesk Sell API, you'll need to obtain an access token using the OAuth 2.0 protocol. Here's how to do it:
// Example using Node.js and Axios
const axios = require('axios');
const getAccessToken = async () => {
const response = await axios.post('https://api.getbase.com/oauth2/token', {
grant_type: 'authorization_code',
client_id: 'Your_Client_ID',
client_secret: 'Your_Client_Secret',
redirect_uri: 'Your_Redirect_URI',
code: 'Authorization_Code'
});
return response.data.access_token;
};
getAccessToken().then(token => console.log(token)).catch(error => console.error(error));
Replace Your_Client_ID
, Your_Client_Secret
, Your_Redirect_URI
, and Authorization_Code
with the actual values from your app setup.
Once you have your access token, you can use it to authenticate your API requests to Zendesk Sell.
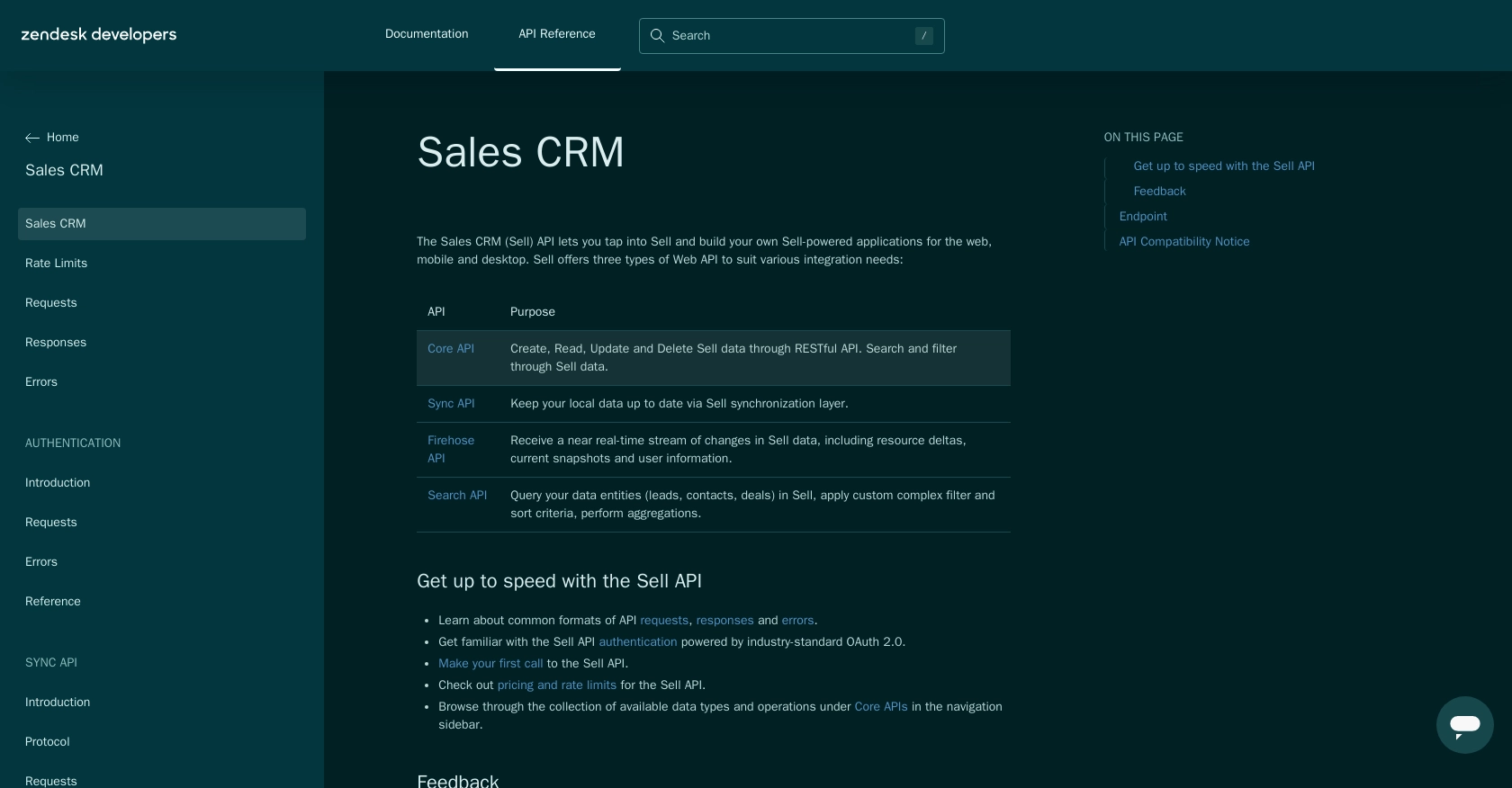
sbb-itb-96038d7
Making API Calls to Zendesk Sell for Lead Management Using JavaScript
To interact with the Zendesk Sell API for creating or updating leads, you'll need to use JavaScript. This section will guide you through the process of making API calls, including setting up your environment and handling responses.
Setting Up Your JavaScript Environment for Zendesk Sell API
Before making API calls, ensure you have Node.js installed on your machine. You'll also need the Axios library to handle HTTP requests. Install Axios using the following command:
npm install axios
Creating a New Lead with Zendesk Sell API
To create a new lead, you'll use the POST method. Here's an example of how to do this using JavaScript and Axios:
const axios = require('axios');
const createLead = async () => {
const accessToken = 'Your_Access_Token'; // Replace with your actual access token
const leadData = {
data: {
first_name: 'John',
last_name: 'Doe',
organization_name: 'Example Corp',
email: 'john.doe@example.com'
}
};
try {
const response = await axios.post('https://api.getbase.com/v2/leads', leadData, {
headers: {
'Authorization': `Bearer ${accessToken}`,
'Content-Type': 'application/json'
}
});
console.log('Lead Created:', response.data);
} catch (error) {
console.error('Error Creating Lead:', error.response.data);
}
};
createLead();
Replace Your_Access_Token
with the access token obtained from the OAuth process. This code sends a POST request to create a new lead with specified details.
Updating an Existing Lead with Zendesk Sell API
To update an existing lead, use the PUT method. Here's how you can update a lead's information:
const updateLead = async (leadId) => {
const accessToken = 'Your_Access_Token'; // Replace with your actual access token
const updatedData = {
data: {
first_name: 'Jane',
last_name: 'Doe',
email: 'jane.doe@example.com'
}
};
try {
const response = await axios.put(`https://api.getbase.com/v2/leads/${leadId}`, updatedData, {
headers: {
'Authorization': `Bearer ${accessToken}`,
'Content-Type': 'application/json'
}
});
console.log('Lead Updated:', response.data);
} catch (error) {
console.error('Error Updating Lead:', error.response.data);
}
};
updateLead(1); // Replace 1 with the actual lead ID you wish to update
Ensure you replace Your_Access_Token
and the lead ID with actual values. This code updates the specified lead's information.
Handling API Responses and Errors
When making API calls, it's crucial to handle responses and potential errors effectively. The Zendesk Sell API uses standard HTTP status codes to indicate success or failure:
- 200 OK: The request was successful.
- 201 Created: A new resource was successfully created.
- 400 Bad Request: The request was malformed.
- 401 Unauthorized: Authentication failed.
- 429 Too Many Requests: Rate limit exceeded. You can make up to 36,000 requests per hour (10 requests/token/second) as per Zendesk's rate limits documentation.
Always check the response status and handle errors gracefully to ensure a robust integration.
For more detailed information on error codes, refer to the Zendesk Sell API error documentation.
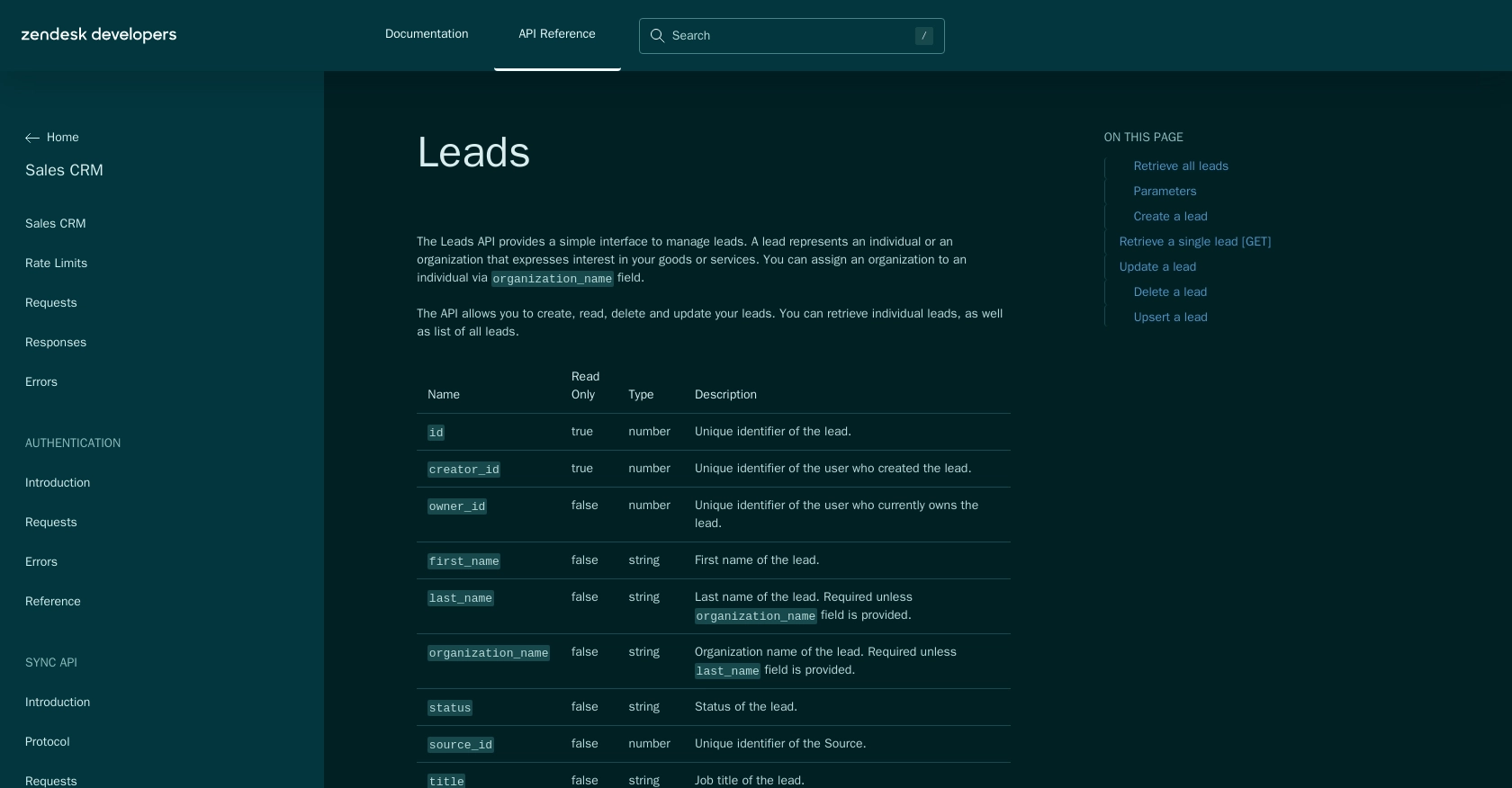
Conclusion and Best Practices for Integrating with Zendesk Sell API
Integrating with the Zendesk Sell API can significantly enhance your sales processes by automating lead management tasks. By following the steps outlined in this guide, you can efficiently create and update leads using JavaScript, ensuring your sales team has access to the most current data.
Best Practices for Secure and Efficient API Integration
- Secure Storage of Credentials: Always store your Client ID, Client Secret, and access tokens securely. Consider using environment variables or secure vaults to protect sensitive information.
- Handle Rate Limiting: Be mindful of Zendesk's rate limits, which allow up to 36,000 requests per hour. Implement logic to handle the
429 Too Many Requests
error by retrying requests after a delay. - Data Standardization: Ensure consistent data formats when sending and receiving information to avoid errors and maintain data integrity.
- Error Handling: Implement robust error handling to manage different HTTP status codes and provide meaningful feedback to users or logs for debugging.
Streamline Your Integrations with Endgrate
While integrating with Zendesk Sell API can be straightforward, managing multiple integrations can become complex. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Zendesk Sell. By using Endgrate, you can:
- Save time and resources by outsourcing integrations and focusing on your core product.
- Build once for each use case instead of multiple times for different integrations.
- Offer an intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate.
Read More
- https://endgrate.com/provider/zendesksell
- https://developer.zendesk.com/api-reference/sales-crm/introduction/
- https://developer.zendesk.com/api-reference/sales-crm/rate-limits/
- https://developer.zendesk.com/api-reference/sales-crm/requests/
- https://developer.zendesk.com/api-reference/sales-crm/errors/
- https://developer.zendesk.com/api-reference/sales-crm/authentication/introduction/
- https://developer.zendesk.com/api-reference/sales-crm/authentication/requests/#client-authentication
- https://developer.zendesk.com/api-reference/sales-crm/resources/leads/
Ready to get started?