Using the Sage 100 API to Get Sales Orders in PHP
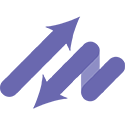
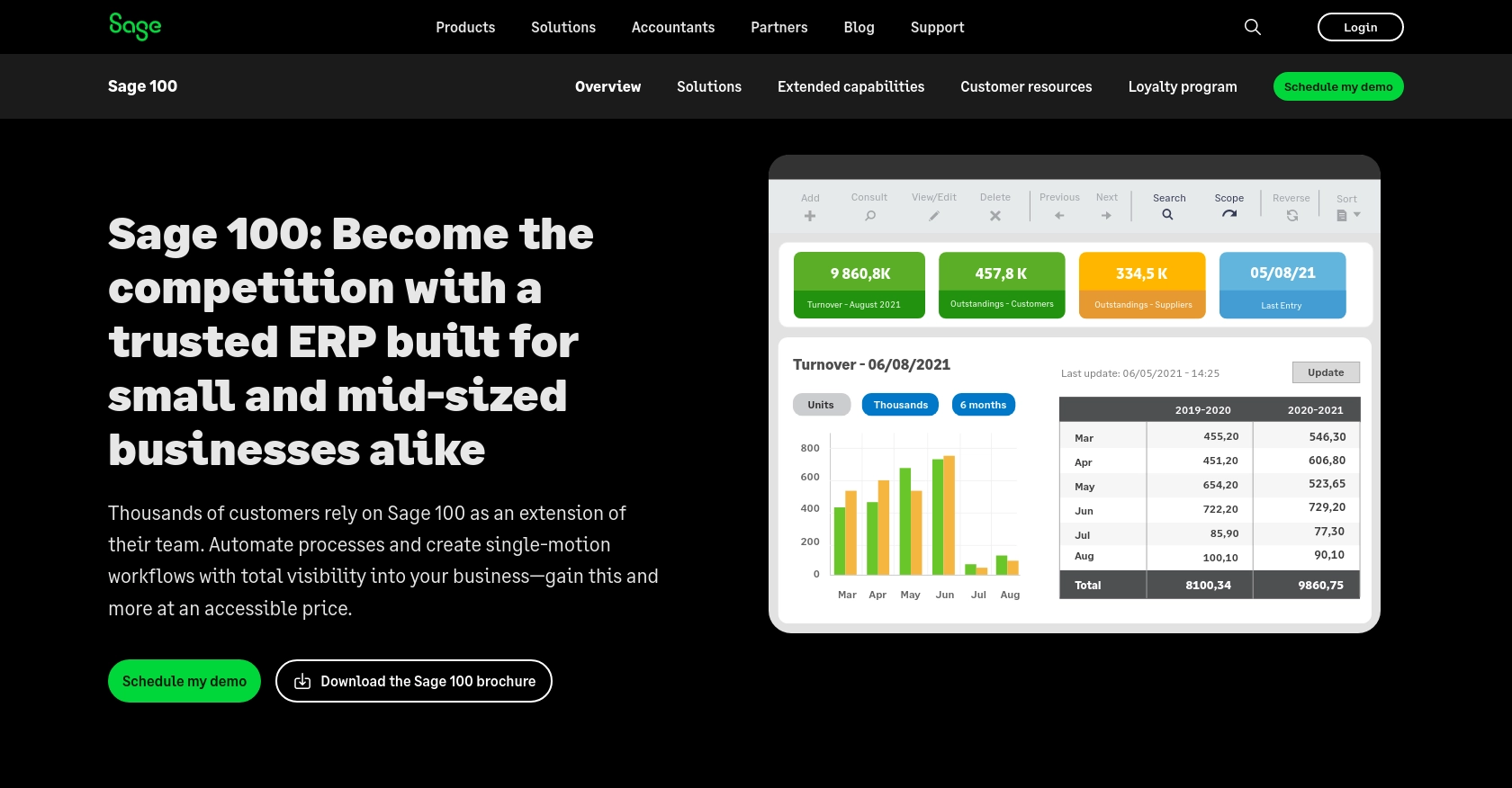
Introduction to Sage 100 and Its API Capabilities
Sage 100 is a comprehensive enterprise resource planning (ERP) solution tailored for small to medium-sized businesses. It offers robust features for accounting, inventory management, sales, and customer relationship management, making it a popular choice for businesses looking to streamline their operations.
Developers often seek to integrate with Sage 100 to automate and enhance business processes. By leveraging the Sage 100 API, developers can access critical data, such as sales orders, to improve reporting, analytics, and decision-making. For example, a developer might use the Sage 100 API to retrieve sales order data and integrate it with a custom dashboard for real-time sales tracking and analysis.
Setting Up a Sage 100 Test Account for API Integration
Before you can begin interacting with the Sage 100 API to retrieve sales orders, it's essential to set up a test environment. This involves configuring the Sage 100 ODBC driver and creating a Data Source Name (DSN) to connect to the Sage 100 database. Follow these steps to ensure a smooth setup process.
Installing and Configuring the Sage 100 ODBC Driver
To start, ensure that the Sage 100 ODBC driver is installed on your system. This driver is crucial for establishing a connection between your PHP application and the Sage 100 database.
- Access the ODBC Data Source Administrator on your system.
- Create a new DSN by selecting the appropriate Sage 100 ODBC driver.
- Configure the DSN with the correct server, database, and authentication settings.
For detailed instructions, refer to the Sage 100 ODBC Driver Configuration Guide.
Creating a Sage 100 Application for API Access
Once the ODBC driver is configured, you need to create an application within Sage 100 to obtain the necessary credentials for API access.
- Log in to your Sage 100 account and navigate to the application management section.
- Create a new application and note down the client ID and client secret provided.
- Ensure the application has the necessary permissions to access sales order data.
Testing the ODBC Connection
After setting up the DSN and application, it's important to test the ODBC connection to ensure everything is configured correctly.
- Open the ODBC Data Source Administrator and locate your newly created DSN.
- Select the DSN and click on "Test Connection" to verify the setup.
- If the connection is successful, you are ready to proceed with making API calls.
With your Sage 100 test account and ODBC driver configured, you are now prepared to interact with the Sage 100 API using PHP to retrieve sales orders and enhance your business processes.
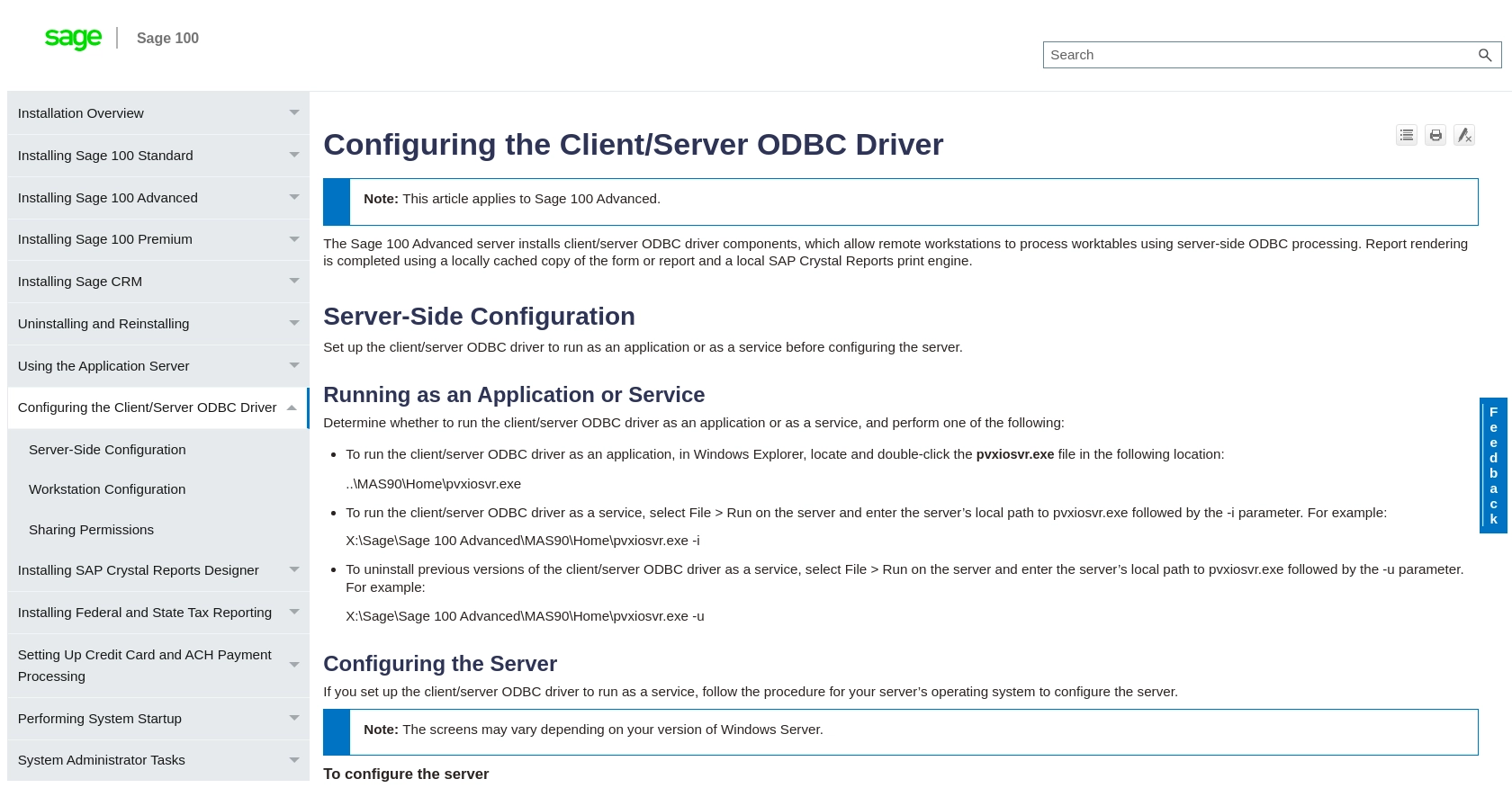
sbb-itb-96038d7
Making API Calls to Retrieve Sales Orders from Sage 100 Using PHP
To interact with the Sage 100 API and retrieve sales orders, you'll need to set up your PHP environment and write the necessary code to make API calls. This section will guide you through the process, including setting up PHP, installing dependencies, and executing the API call.
Setting Up Your PHP Environment for Sage 100 API Integration
Before making API calls, ensure your PHP environment is correctly configured. You'll need PHP version 7.4 or higher and the necessary extensions to handle ODBC connections.
- Verify your PHP installation by running
php -v
in your terminal. - Ensure the ODBC extension is enabled in your
php.ini
file. Look for the lineextension=odbc
and remove the semicolon if it's commented out. - Restart your web server to apply changes.
Installing Required PHP Libraries for Sage 100 API Calls
To interact with the Sage 100 API, you'll need the odbc
extension. If it's not already installed, you can add it using the following command:
sudo apt-get install php-odbc
For Windows users, ensure the ODBC driver is installed and configured as described in the Sage 100 ODBC Driver Configuration Guide.
Writing PHP Code to Retrieve Sales Orders from Sage 100
With your environment set up, you can now write PHP code to retrieve sales orders from Sage 100. The following example demonstrates how to connect to the Sage 100 database and execute a query to fetch sales order data.
<?php
// Define the DSN and credentials
$dsn = 'SOTAMAS90';
$user = 'your_username';
$password = 'your_password';
// Establish the ODBC connection
$conn = odbc_connect($dsn, $user, $password);
if (!$conn) {
die('Connection failed: ' . odbc_errormsg());
}
// Define the SQL query to retrieve sales orders
$query = "SELECT * FROM SO_SalesOrderHeader";
// Execute the query
$result = odbc_exec($conn, $query);
if (!$result) {
die('Query failed: ' . odbc_errormsg());
}
// Fetch and display the sales orders
while ($row = odbc_fetch_array($result)) {
echo 'Order Number: ' . $row['OrderNumber'] . '<br>';
echo 'Customer Number: ' . $row['CustomerNo'] . '<br>';
echo 'Order Date: ' . $row['OrderDate'] . '<br>';
echo '<br>';
}
// Close the connection
odbc_close($conn);
?>
Replace your_username
and your_password
with your actual Sage 100 credentials. This script connects to the Sage 100 database, executes a query to retrieve sales orders, and displays the results.
Verifying API Call Success and Handling Errors
After running the script, verify that the sales orders are retrieved successfully. Check the output for the expected sales order data. If there are any issues, ensure the DSN and credentials are correct and that the ODBC connection is properly configured.
To handle errors, use try-catch blocks to catch exceptions and log error messages for troubleshooting. This will help you identify and resolve any issues with the API calls.
By following these steps, you can efficiently retrieve sales orders from Sage 100 using PHP, enabling you to integrate this data into your applications and enhance your business processes.
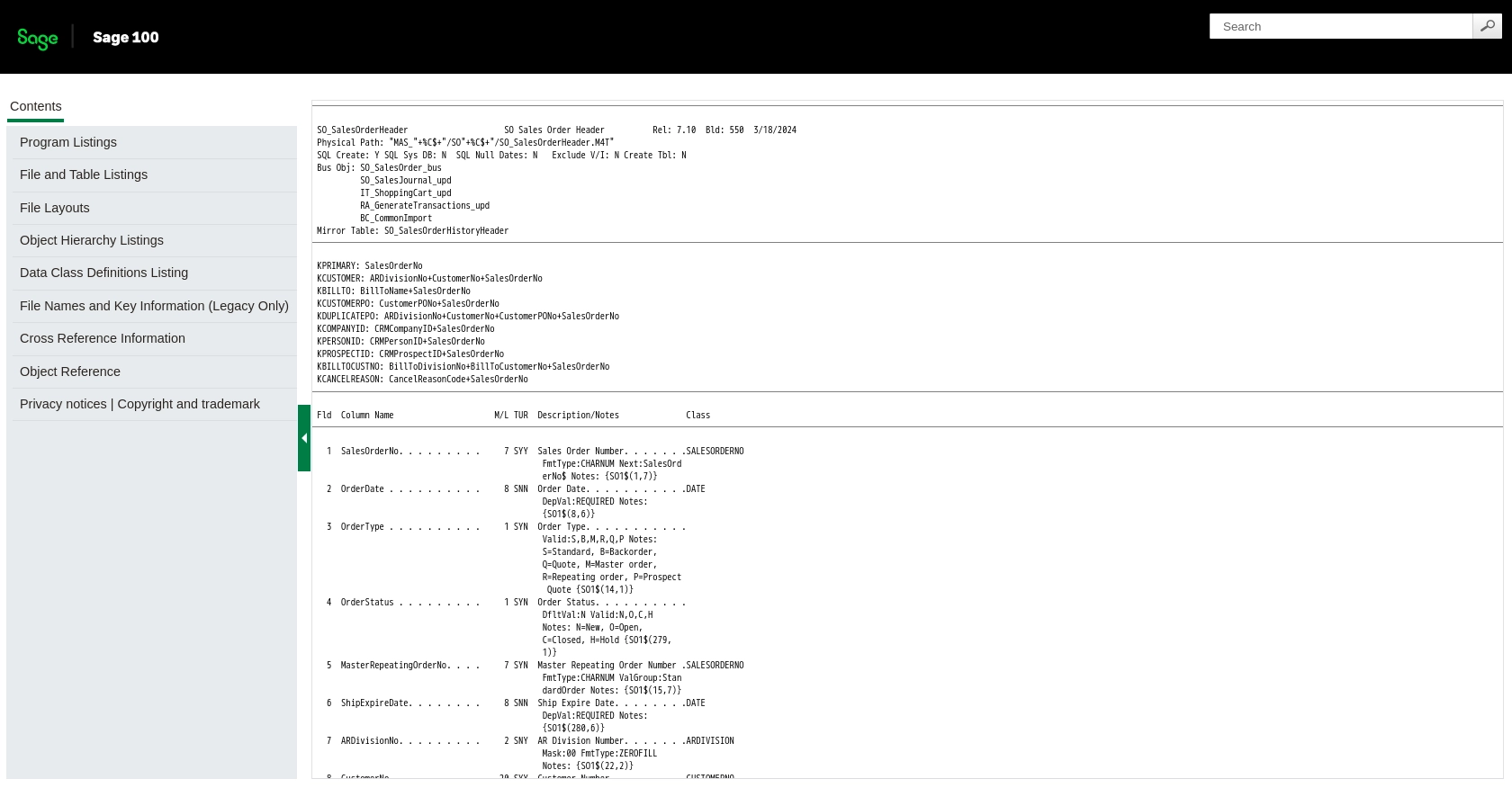
Conclusion and Best Practices for Sage 100 API Integration Using PHP
Integrating with the Sage 100 API to retrieve sales orders using PHP can significantly enhance your business processes by providing real-time access to critical sales data. By following the steps outlined in this guide, you can efficiently set up your environment, establish a connection, and execute API calls to access valuable information.
Best Practices for Secure and Efficient API Integration
- Secure Storage of Credentials: Always store your Sage 100 credentials securely. Consider using environment variables or a secure vault to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of any rate limits imposed by the Sage 100 API. Implement logic to handle retries or backoff strategies to avoid exceeding limits.
- Data Transformation and Standardization: Ensure that the data retrieved from Sage 100 is transformed and standardized to fit your application's requirements. This will facilitate seamless integration and data consistency.
- Error Handling: Implement robust error handling to manage connection issues, query failures, and other potential errors. Use logging to track and diagnose issues effectively.
Streamlining Integration Processes with Endgrate
While integrating with Sage 100 can be rewarding, managing multiple integrations can be complex and time-consuming. Endgrate offers a solution by providing a unified API endpoint that connects to various platforms, including Sage 100. By leveraging Endgrate, you can streamline your integration processes, save time, and focus on your core product development.
Explore how Endgrate can simplify your integration needs by visiting Endgrate's website and discover how you can build once for each use case instead of multiple times for different integrations.
Read More
- https://endgrate.com/provider/sage100
- https://help-sage100.na.sage.com/InstallGuide/2022/Content/InstallGuide/Config_ODBC_Driver.htm
- https://help-sage100.na.sage.com/2022/FLOR/index.htm#File_Layouts/Sales_Order/SO_SalesOrderHeader.htm?Highlight=SO_SalesOrderHeader
- https://help-sage100.na.sage.com/2022/FLOR/index.htm#File_Layouts/Sales_Order/SO_SalesOrderDetail.htm?Highlight=SO_SalesOrderDetail
Ready to get started?