Using the Cloze API to Create or Update Companies in PHP
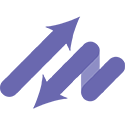
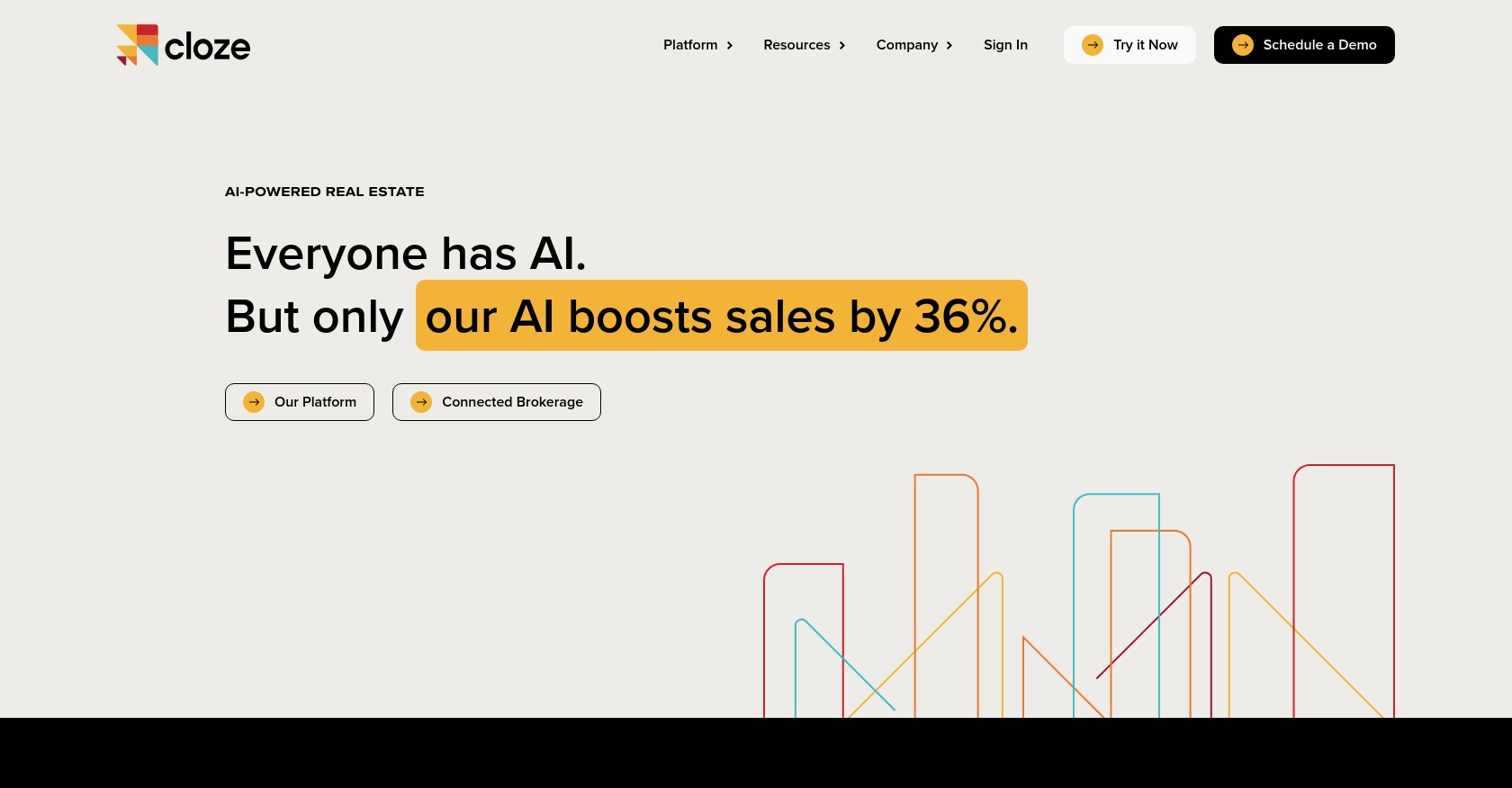
Introduction to Cloze API for Company Management
Cloze is a comprehensive relationship management platform that integrates seamlessly with various business tools to enhance productivity and streamline workflows. It offers a robust API that allows developers to interact with its features programmatically, making it an ideal choice for businesses looking to automate and optimize their relationship management processes.
Integrating with the Cloze API can empower developers to efficiently manage company data, such as creating or updating company profiles. For example, a developer might use the Cloze API to automatically update company information from a CRM system, ensuring that all data remains consistent and up-to-date across platforms.
This guide will walk you through using PHP to interact with the Cloze API for creating or updating company records, providing a step-by-step approach to streamline your integration efforts.
Setting Up Your Cloze API Test or Sandbox Account
Before you begin integrating with the Cloze API, it's essential to set up a test or sandbox account. This allows you to safely experiment with API calls without affecting live data. Follow these steps to create your account and obtain the necessary credentials for authentication.
Creating a Cloze Account for API Access
- Visit the Cloze website and sign up for a free trial or demo account if you don't already have one.
- Follow the on-screen instructions to complete the registration process and verify your email address.
- Once your account is set up, log in to access the Cloze dashboard.
Generating an API Key for Cloze API Authentication
The Cloze API uses API key-based authentication to secure access. Here's how to generate your API key:
- Navigate to the settings section of your Cloze account.
- Look for the API settings or developer options.
- Follow the instructions to generate a new API key. Make sure to store this key securely, as it will be used to authenticate your API requests.
- Note that you'll need to set the
user
parameter to your Cloze account email address and theapi_key
parameter to the API key you just created.
For more detailed instructions, refer to the Cloze API documentation.
Configuring OAuth for Public Integrations
If you plan to build a public integration with Cloze, you'll need to use OAuth for authentication. While initial development and testing can be done with an API key, publishing your integration requires OAuth. Contact support@cloze.com to get started with OAuth-enabled integration.
With your test account and API key ready, you're now set to begin integrating with the Cloze API using PHP.
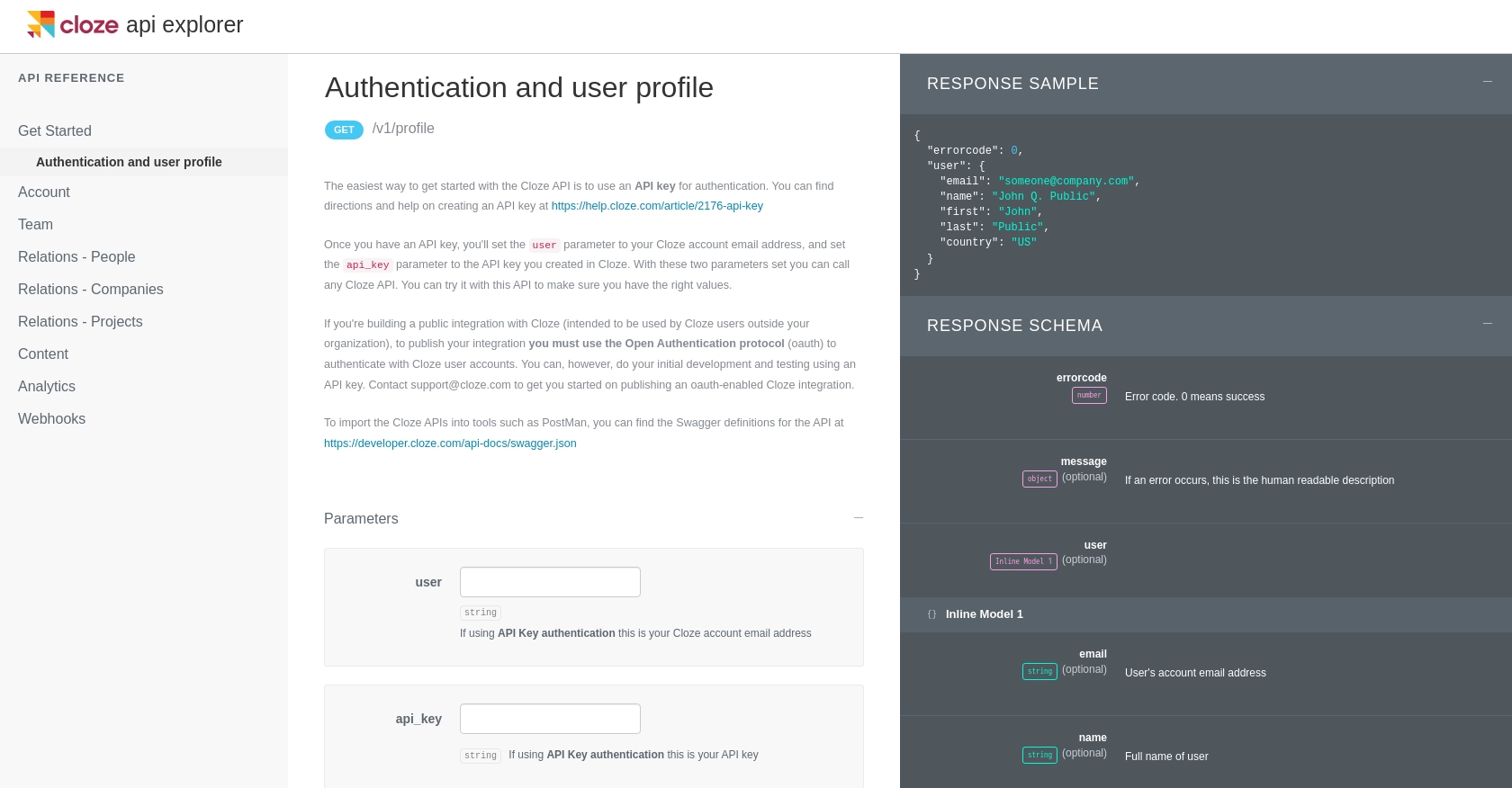
sbb-itb-96038d7
Making API Calls to Cloze for Company Management Using PHP
To interact with the Cloze API for creating or updating company records, you'll need to set up your PHP environment and make HTTP requests to the appropriate endpoints. This section will guide you through the process, including setting up PHP, installing necessary dependencies, and crafting the API requests.
Setting Up PHP Environment for Cloze API Integration
Before making API calls, ensure your development environment is ready:
- Install PHP 7.4 or later on your machine.
- Ensure you have Composer installed for managing dependencies.
Installing Required PHP Dependencies
You'll need the Guzzle HTTP client to make HTTP requests. Install it using Composer:
composer require guzzlehttp/guzzle
Creating or Updating Companies with Cloze API in PHP
Now that your environment is set up, you can create or update company records using the Cloze API. Here's a sample PHP script to guide you:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$response = $client->post('https://api.cloze.com/v1/companies/create', [
'query' => [
'user' => 'your_email@domain.com',
'api_key' => 'your_api_key'
],
'json' => [
'name' => 'New Company Name',
'description' => 'Description of the company',
'stage' => 'lead',
'segment' => 'customer',
'domains' => ['newcompany.com']
]
]);
if ($response->getStatusCode() == 200) {
echo "Company created or updated successfully.";
} else {
echo "Failed to create or update company.";
}
Replace your_email@domain.com
and your_api_key
with your Cloze account email and API key. Customize the JSON payload with the company details you wish to create or update.
Verifying API Call Success in Cloze Sandbox
After running the script, verify the success of your API call by checking the company records in your Cloze sandbox account. If the company appears as expected, your integration is working correctly.
Handling Errors and Debugging API Requests
It's crucial to handle potential errors in your API requests. The Cloze API returns error codes and messages that you can use to debug issues. Here's how you can handle errors in your PHP script:
try {
$response = $client->post('https://api.cloze.com/v1/companies/create', [
'query' => [
'user' => 'your_email@domain.com',
'api_key' => 'your_api_key'
],
'json' => [
'name' => 'New Company Name',
'description' => 'Description of the company',
'stage' => 'lead',
'segment' => 'customer',
'domains' => ['newcompany.com']
]
]);
$data = json_decode($response->getBody(), true);
if ($data['errorcode'] === 0) {
echo "Company created or updated successfully.";
} else {
echo "Error: " . $data['message'];
}
} catch (Exception $e) {
echo "Request failed: " . $e->getMessage();
}
By implementing error handling, you can ensure that your application gracefully manages any issues that arise during API interactions.
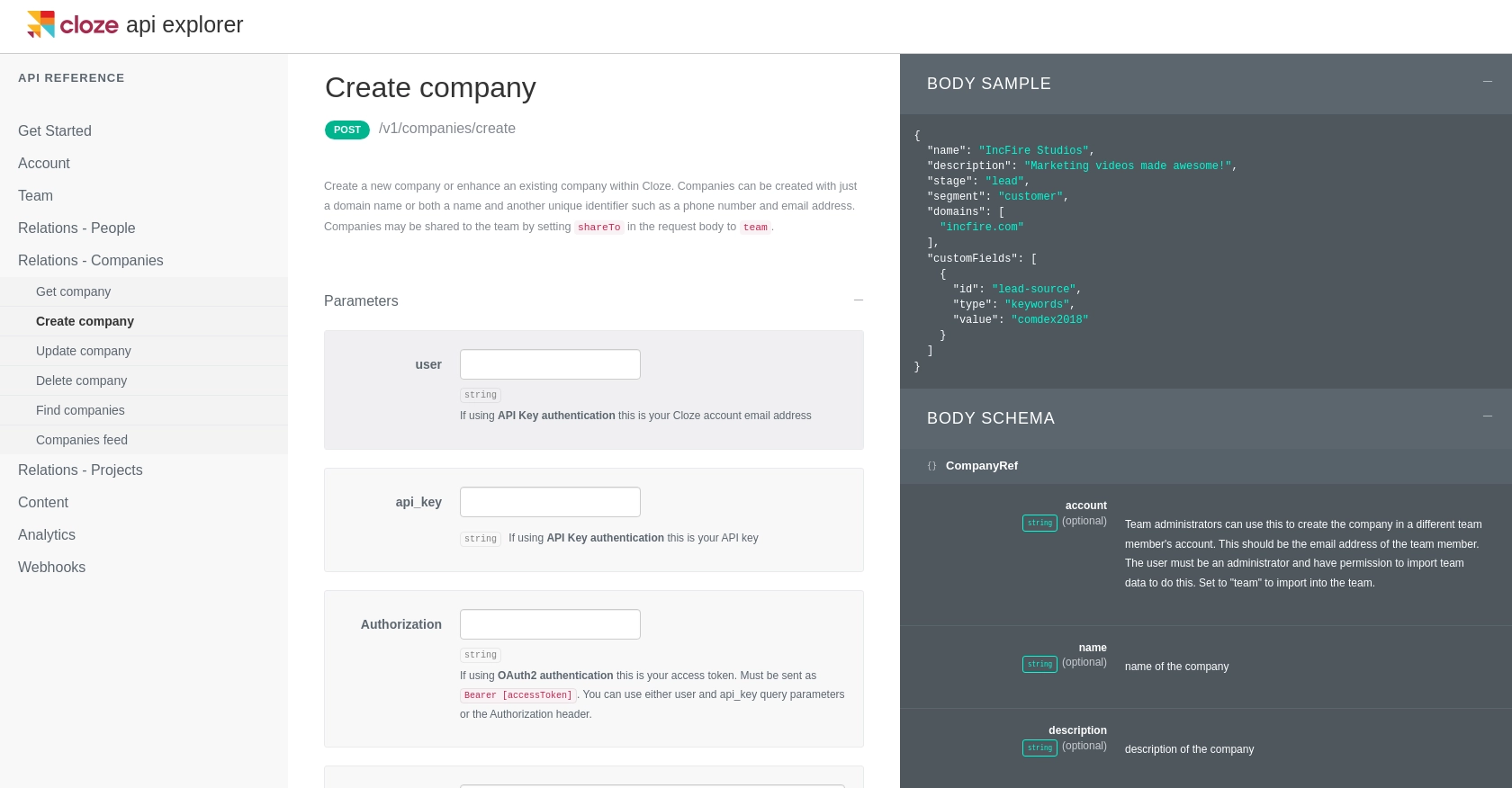
Conclusion and Best Practices for Using Cloze API with PHP
Integrating with the Cloze API using PHP can significantly enhance your ability to manage company data efficiently. By following the steps outlined in this guide, you can create or update company records seamlessly, ensuring data consistency across platforms.
Best Practices for Secure and Efficient API Integration
- Secure API Keys: Always store your API keys securely and avoid hardcoding them in your scripts. Consider using environment variables or secure vaults.
- Handle Rate Limiting: Be mindful of the API rate limits to avoid throttling. Implement exponential backoff strategies for retrying requests.
- Data Standardization: Ensure that data fields are standardized before sending them to the API to maintain consistency and avoid errors.
- Error Handling: Implement robust error handling to manage API response errors gracefully and provide meaningful feedback to users.
Streamline Your Integration Process with Endgrate
While integrating with the Cloze API can be straightforward, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies integration processes, allowing you to focus on your core product development. By leveraging Endgrate, you can build once for each use case and enjoy an intuitive integration experience for your customers.
Explore how Endgrate can help you save time and resources by visiting Endgrate today.
Read More
Ready to get started?