Using the Copper API to Get Opportunities in PHP
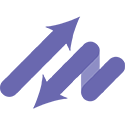
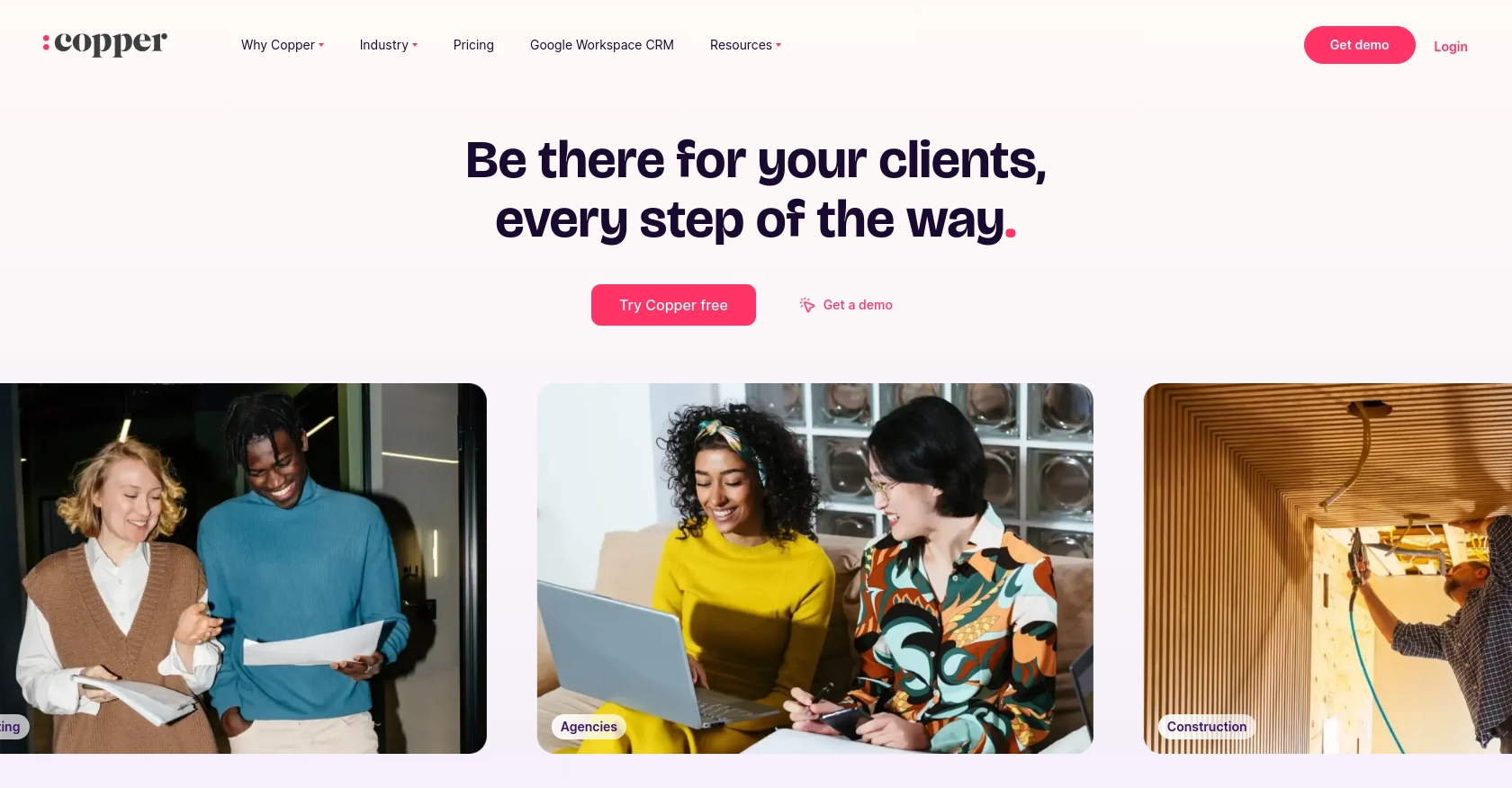
Introduction to Copper CRM API
Copper is a powerful CRM platform designed to seamlessly integrate with Google Workspace, providing businesses with an efficient way to manage customer relationships directly from their inbox. Known for its user-friendly interface and robust features, Copper helps teams streamline their sales processes and improve productivity.
Developers may want to connect with Copper's API to automate tasks such as retrieving and managing sales opportunities. For example, using the Copper API in PHP, a developer can fetch a list of sales opportunities to analyze and prioritize leads, enhancing the sales strategy and decision-making process.
Setting Up Your Copper Test or Sandbox Account
Creating a Copper Account for API Integration
To begin integrating with the Copper API, you'll need to set up a Copper account. If you don't already have one, you can sign up for a free trial on the Copper website. This will give you access to the necessary tools and features to explore the API capabilities.
Follow these steps to create your account:
- Visit the Copper website and click on the "Sign Up" button.
- Fill in the required information, such as your name, email, and company details.
- Choose the free trial option to explore Copper's features without any initial cost.
- Complete the registration process and verify your email address.
Generating API Key and Secret for Copper API Access
Once your Copper account is set up, you'll need to generate an API key and secret to authenticate your API requests. Copper uses a custom authentication method, which involves these credentials.
Here's how to generate your API key and secret:
- Log in to your Copper account and navigate to the "Settings" section.
- Under the "Integrations" tab, find the "API Keys" option.
- Click on "Create API Key" and provide a name for your key.
- Copy the generated API key and secret. Store them securely, as you'll need them for API requests.
Configuring Your PHP Environment for Copper API
Before making API calls, ensure your PHP environment is properly configured. You'll need PHP 7.4 or later and the cURL extension enabled.
To install the necessary dependencies, use Composer:
composer require guzzlehttp/guzzle
This will install Guzzle, a PHP HTTP client that simplifies making API requests.
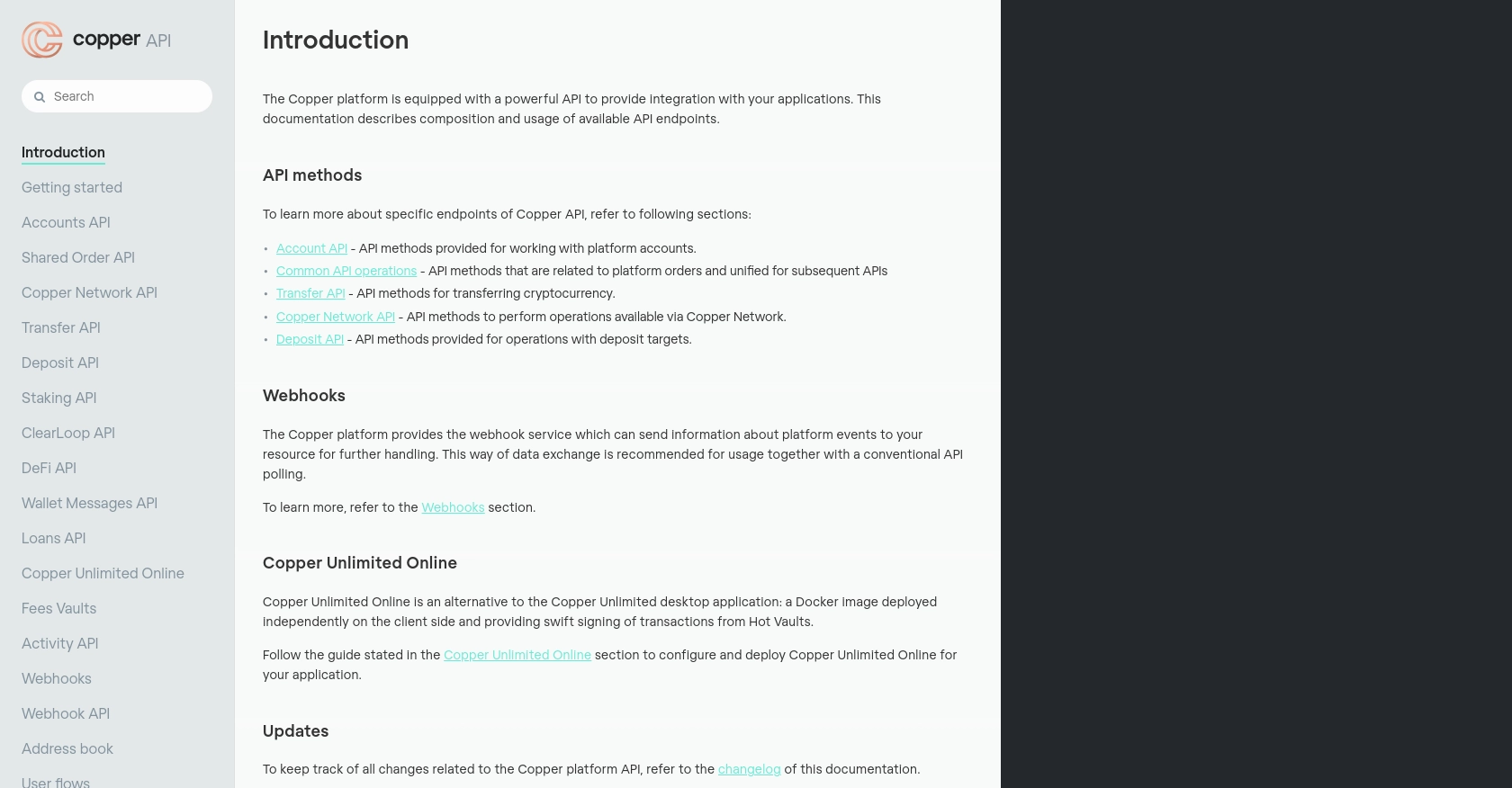
sbb-itb-96038d7
Making API Calls to Retrieve Opportunities Using Copper API in PHP
Setting Up PHP for Copper API Requests
To interact with the Copper API using PHP, ensure your environment is ready with PHP 7.4 or later and the cURL extension enabled. Additionally, you'll need Guzzle, a PHP HTTP client, to simplify API requests.
Install Guzzle using Composer:
composer require guzzlehttp/guzzle
Writing PHP Code to Fetch Opportunities from Copper API
With your environment set up, you can now write the PHP code to fetch opportunities from the Copper API. Below is a sample script to help you get started:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$apiKey = 'Your_API_Key';
$apiSecret = 'Your_API_Secret';
$timestamp = round(microtime(true) * 1000);
$method = 'GET';
$path = '/opportunities';
$body = '';
$signature = hash_hmac('sha256', $timestamp . $method . $path . $body, $apiSecret);
$response = $client->request('GET', 'https://api.copper.co' . $path, [
'headers' => [
'Authorization' => 'ApiKey ' . $apiKey,
'X-Signature' => $signature,
'X-Timestamp' => $timestamp,
'Content-Type' => 'application/json'
]
]);
$data = json_decode($response->getBody(), true);
foreach ($data['opportunities'] as $opportunity) {
echo 'Opportunity Name: ' . $opportunity['name'] . "\n";
}
Understanding the PHP Code for Copper API Integration
In the code above, we use Guzzle to send a GET request to the Copper API. The request includes headers for authentication, such as the API key, signature, and timestamp. The signature is generated using HMAC SHA256 with the API secret.
The response is parsed as JSON, and we loop through the opportunities to display their names.
Verifying Successful API Requests in Copper
To verify that your API requests are successful, check the response status code. A 2xx status code indicates success. You can also log into your Copper account to see if the opportunities data matches the API response.
Handling Errors and Common Error Codes in Copper API
When making API calls, it's crucial to handle potential errors. Copper API may return various error codes, such as:
- 400 Bad Request: Validation errors, such as missing required fields.
- 401 Unauthorized: Invalid API key or signature.
- 403 Forbidden: Access denied to the requested resource.
- 404 Not Found: The requested endpoint does not exist.
- 429 Too Many Requests: Rate limit exceeded.
Implement error handling in your code to manage these responses gracefully.
Best Practices for Copper API Integration in PHP
When integrating with the Copper API using PHP, it's essential to follow best practices to ensure secure and efficient interactions. Here are some recommendations:
- Secure Storage of Credentials: Always store your API key and secret securely, using environment variables or a secure vault, to prevent unauthorized access.
- Rate Limiting: Copper API has a rate limit of 30,000 requests per 5 minutes per IP address. Implement logic to handle rate limiting gracefully, such as retrying requests after a delay.
- Data Standardization: Ensure that data retrieved from the API is standardized and transformed as needed to fit your application's requirements.
- Error Handling: Implement robust error handling to manage different HTTP status codes and error messages returned by the API.
Leveraging Endgrate for Streamlined Copper API Integration
Integrating with multiple APIs can be time-consuming and complex. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Copper. By using Endgrate, you can:
- Save Time and Resources: Focus on your core product while Endgrate handles the integration complexities.
- Build Once, Deploy Anywhere: Create a single integration for each use case and deploy it across multiple platforms.
- Enhance User Experience: Offer your customers a seamless and intuitive integration experience.
Explore how Endgrate can optimize your integration strategy by visiting Endgrate.
Read More
Ready to get started?