Using the PipelineCRM API to Create or Update People (with Javascript examples)
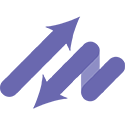
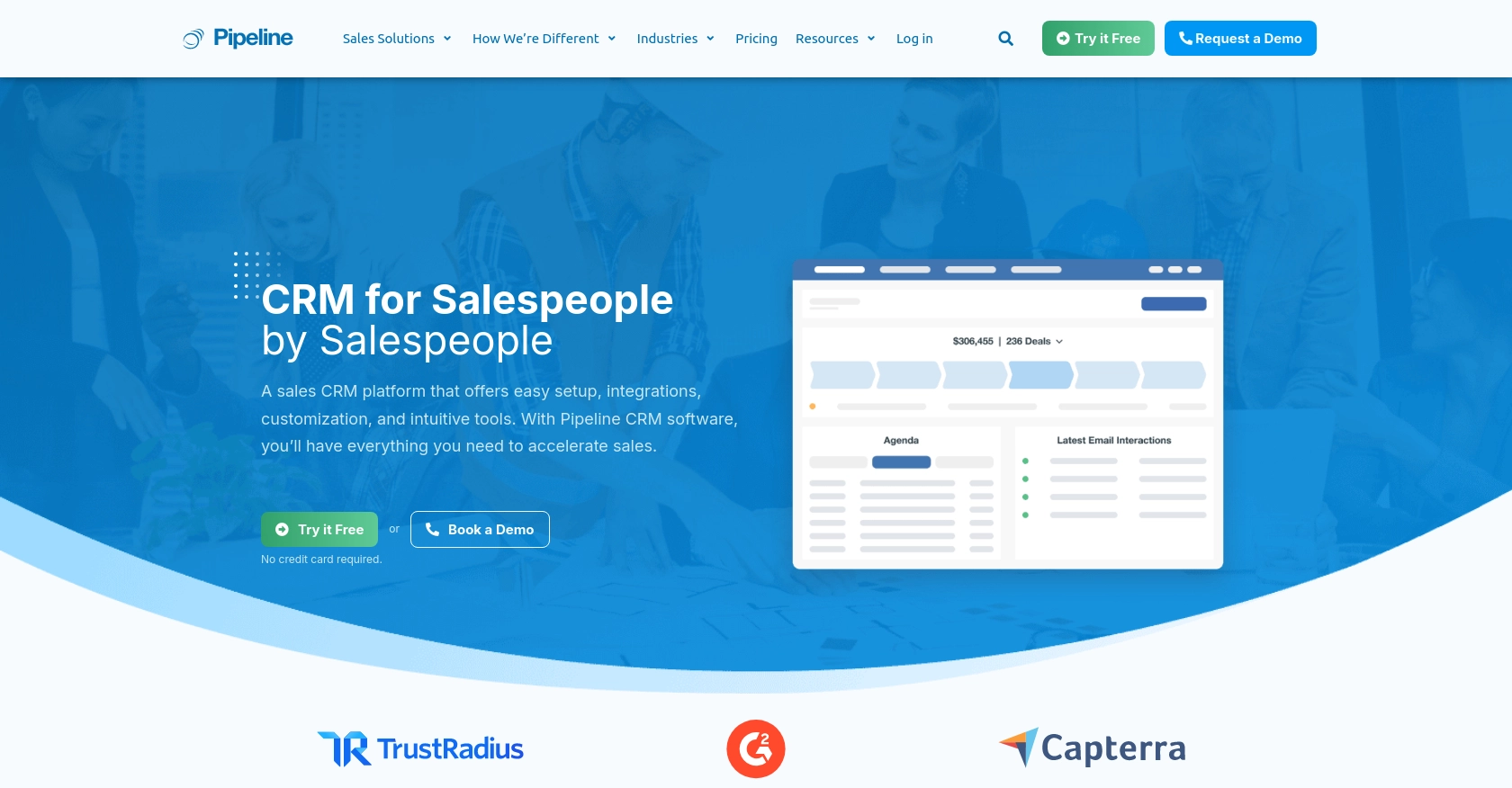
Introduction to PipelineCRM
PipelineCRM is a robust customer relationship management platform designed to help businesses manage their sales processes efficiently. With features like contact management, sales tracking, and reporting, PipelineCRM empowers sales teams to streamline their workflows and enhance productivity.
Developers may want to integrate with PipelineCRM's API to automate and optimize sales operations. For example, using the PipelineCRM API, you can create or update contact information seamlessly, ensuring that your sales team always has access to the most current data. This integration can be particularly useful for syncing data from other platforms or applications, reducing manual data entry and minimizing errors.
Setting Up Your PipelineCRM Test Account
Before you can start integrating with the PipelineCRM API, you need to set up a test account. This will allow you to safely experiment with API calls without affecting your live data.
Creating a PipelineCRM Account
If you don't already have a PipelineCRM account, you can sign up for a free trial on their website. This will give you access to the necessary features to test the API integration.
- Visit the PipelineCRM website.
- Click on the "Sign Up" button and follow the instructions to create your account.
- Once your account is set up, log in to access the dashboard.
Generating Your API Key for PipelineCRM
PipelineCRM uses API key-based authentication to authorize requests. Follow these steps to generate your API key:
- Log in to your PipelineCRM account.
- Navigate to the "Settings" section from the dashboard.
- Find the "API Keys" tab and click on it.
- Click on "Generate New API Key" and give it a descriptive name for easy identification.
- Copy the generated API key and store it securely, as you will need it for authentication in your API calls.
For more detailed information, you can refer to the PipelineCRM API documentation.
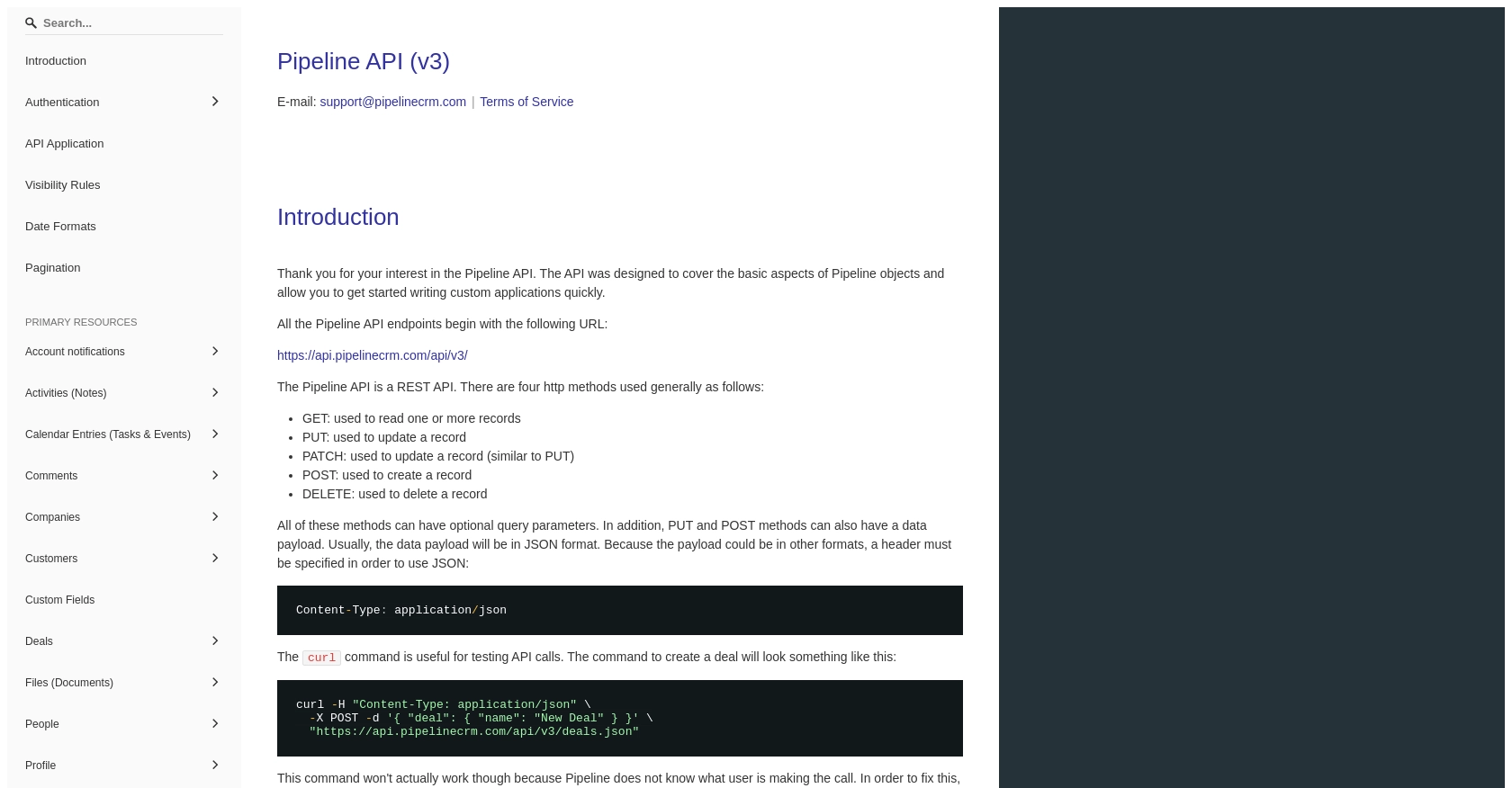
sbb-itb-96038d7
Making API Calls to Create or Update People in PipelineCRM Using JavaScript
To interact with the PipelineCRM API for creating or updating people, you'll need to use JavaScript to make HTTP requests. This section will guide you through the process, including setting up your environment, writing the code, and handling responses and errors effectively.
Setting Up Your JavaScript Environment for PipelineCRM API Integration
Before making API calls, ensure you have Node.js installed on your machine. Node.js provides the runtime for executing JavaScript outside the browser, which is essential for server-side operations like API requests.
- Download and install Node.js from the official website.
- Once installed, verify the installation by running
node -v
andnpm -v
in your terminal.
Next, you'll need to install the axios
library, a popular HTTP client for making requests:
npm install axios
Writing JavaScript Code to Create or Update People in PipelineCRM
With your environment set up, you can now write the JavaScript code to interact with the PipelineCRM API. Below is an example of how to create or update a person using the API.
const axios = require('axios');
// Your PipelineCRM API key
const apiKey = 'YOUR_API_KEY';
// Function to create or update a person
async function createOrUpdatePerson(personData) {
try {
const response = await axios.post('https://api.pipelinecrm.com/api/v3/people', personData, {
headers: {
'Authorization': `Bearer ${apiKey}`,
'Content-Type': 'application/json'
}
});
console.log('Person created/updated successfully:', response.data);
} catch (error) {
console.error('Error creating/updating person:', error.response ? error.response.data : error.message);
}
}
// Example person data
const personData = {
first_name: 'John',
last_name: 'Doe',
email: 'john.doe@example.com'
};
// Call the function
createOrUpdatePerson(personData);
Replace YOUR_API_KEY
with the API key you generated earlier. The personData
object contains the details of the person you wish to create or update. Adjust the fields as necessary to match your requirements.
Verifying API Call Success and Handling Errors
After executing the code, check the response to ensure the person was created or updated successfully. The response data will contain the details of the operation.
If an error occurs, the catch block will log the error message. Common issues include invalid API keys, incorrect data formats, or network problems. Always ensure your API key is correct and that the data you send matches the expected format.
Testing and Validating API Calls in PipelineCRM
To verify the success of your API calls, log in to your PipelineCRM test account and check the people section. You should see the newly created or updated person listed there. This confirms that your integration is working as expected.
For more detailed information on handling errors and response codes, refer to the PipelineCRM API documentation.
Conclusion and Best Practices for PipelineCRM API Integration
Integrating with the PipelineCRM API using JavaScript allows developers to efficiently manage contact data, ensuring that sales teams have access to up-to-date information. By automating the creation and updating of people in PipelineCRM, you can streamline workflows and reduce manual data entry errors.
Best Practices for Secure and Efficient API Usage
- Secure API Key Storage: Always store your API keys securely. Avoid hardcoding them in your source code. Consider using environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Be mindful of API rate limits to avoid service disruptions. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Validation: Ensure that the data being sent to the API is validated and sanitized to prevent errors and maintain data integrity.
- Error Handling: Implement robust error handling to manage different types of errors, such as network issues or invalid data formats. Log errors for troubleshooting and monitoring purposes.
Enhancing Integration Capabilities with Endgrate
While integrating with PipelineCRM is a powerful way to optimize your sales processes, managing multiple integrations can be complex and time-consuming. Endgrate offers a unified API solution that simplifies integration management across various platforms, including PipelineCRM.
By leveraging Endgrate, you can save time and resources, allowing your team to focus on core product development. With a single API endpoint, you can build once and deploy across multiple platforms, providing an intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate today.
Read More
Ready to get started?