How to Create or Update Invoices with the Younium API in Javascript
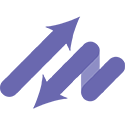
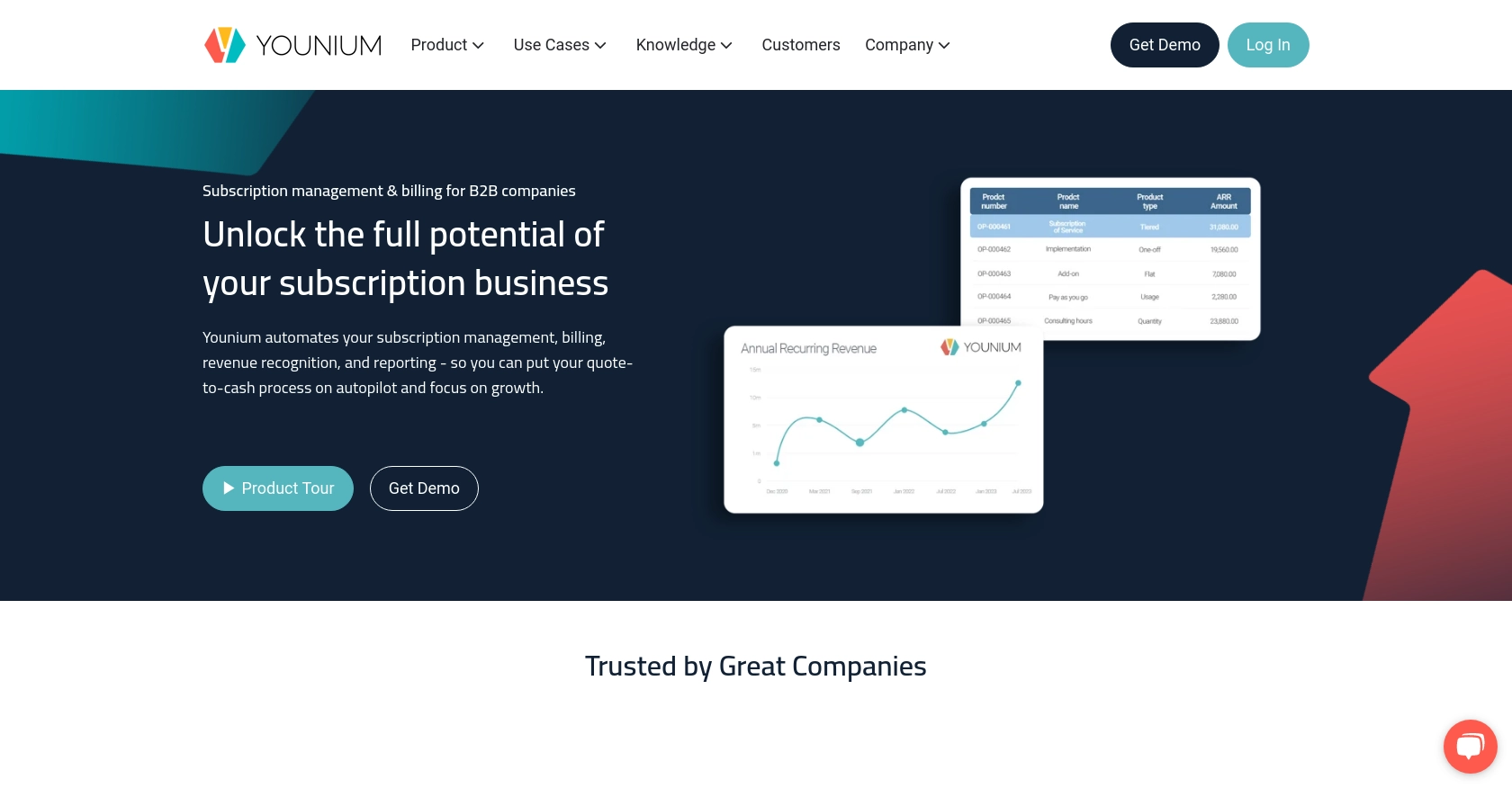
Introduction to Younium API for Invoice Management
Younium is a comprehensive subscription management platform designed to streamline billing and invoicing processes for B2B SaaS companies. It offers robust tools for managing subscriptions, invoicing, and financial reporting, making it an essential solution for businesses looking to automate and optimize their financial operations.
Integrating with the Younium API allows developers to efficiently create and update invoices, enabling seamless financial transactions and record-keeping. For example, a developer might use the Younium API to automatically generate invoices based on subscription renewals, ensuring accurate billing and reducing manual errors.
Setting Up Your Younium Test/Sandbox Account for API Integration
Before you can start creating or updating invoices using the Younium API, you'll need to set up a test or sandbox account. This environment allows you to safely test API interactions without affecting your production data.
Creating a Younium Sandbox Account
If you don't already have a Younium account, you can sign up for a sandbox account on the Younium website. This account will provide you with the necessary environment to test API calls and ensure everything functions as expected.
- Visit the Younium Developer Portal and sign up for a sandbox account.
- Follow the on-screen instructions to complete the registration process.
- Once your account is set up, log in to access the sandbox environment.
Generating API Tokens and Client Credentials
To authenticate your API requests, you'll need to generate API tokens and client credentials. This process involves creating a personal token that will be used to acquire a JWT access token.
- Log in to your Younium account and open the user profile menu by clicking your name in the top right corner.
- Select "Privacy & Security" from the dropdown menu.
- Navigate to "Personal Tokens" and click "Generate Token".
- Provide a relevant description for the token and click "Create".
- Copy the generated Client ID and Secret Key. These credentials will not be visible again, so ensure they are stored securely.
Acquiring a JWT Access Token
With your client credentials ready, you can now generate a JWT access token. This token is required for authenticating API requests.
// Example POST request to acquire JWT token
const axios = require('axios');
const getToken = async () => {
try {
const response = await axios.post('https://api.sandbox.younium.com/auth/token', {
clientId: 'Your_Client_ID',
secret: 'Your_Secret_Key'
}, {
headers: {
'Content-Type': 'application/json'
}
});
console.log('Access Token:', response.data.accessToken);
} catch (error) {
console.error('Error acquiring token:', error.response.data);
}
};
getToken();
Replace Your_Client_ID
and Your_Secret_Key
with the credentials you generated earlier. If successful, this request will return a JWT access token, valid for 24 hours.
Handling Authentication Errors
Be aware of common authentication errors:
- 401 Unauthorized: Indicates an expired, missing, or incorrect access token.
- 403 Forbidden: Occurs if the legal entity provided is invalid or if the user lacks necessary permissions.
For more details, refer to the Younium Authentication Documentation.
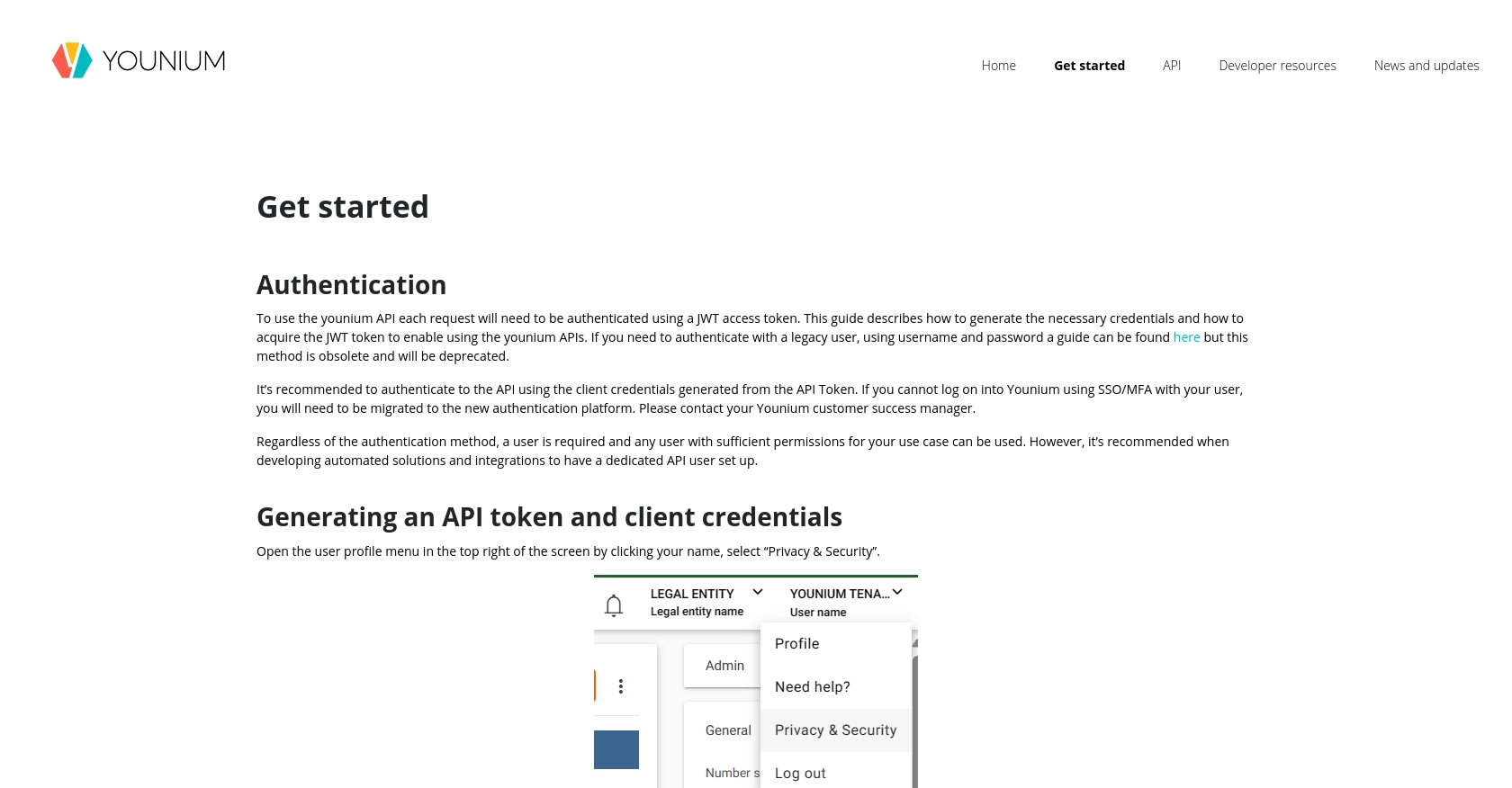
sbb-itb-96038d7
Making API Calls to Create or Update Invoices with Younium in JavaScript
To interact with the Younium API for creating or updating invoices, you'll need to make authenticated API calls using JavaScript. This section will guide you through the process, including setting up your environment, writing the code, and handling potential errors.
Setting Up Your JavaScript Environment for Younium API Integration
Before making API calls, ensure you have the necessary tools and libraries installed. You'll need Node.js and npm (Node Package Manager) to manage dependencies.
- Ensure you have Node.js installed. You can download it from the official Node.js website.
- Use npm to install the Axios library, which simplifies making HTTP requests:
npm install axios
Creating or Updating Invoices with Younium API
Once your environment is set up, you can proceed to create or update invoices using the Younium API. Below is an example of how to make a POST request to create an invoice.
const axios = require('axios');
const createOrUpdateInvoice = async () => {
const accessToken = 'Your_JWT_Access_Token';
const invoiceData = {
// Replace with actual invoice data
"name": "Invoice Name",
"currency": "USD",
"defaultPaymentTerm": "NET30",
"invoiceEmailAddress": "example@company.com"
};
try {
const response = await axios.post('https://api.sandbox.younium.com/invoices', invoiceData, {
headers: {
'Authorization': `Bearer ${accessToken}`,
'Content-Type': 'application/json',
'api-version': '2.1'
}
});
console.log('Invoice Created/Updated:', response.data);
} catch (error) {
console.error('Error creating/updating invoice:', error.response.data);
}
};
createOrUpdateInvoice();
Replace Your_JWT_Access_Token
with the token obtained from the authentication process. Ensure the invoiceData
object contains the necessary fields as per your requirements.
Verifying Successful API Requests in Younium Sandbox
After executing the API call, you can verify the success of the operation by checking the Younium sandbox environment. The newly created or updated invoice should be visible in your account.
Handling Errors and Common Issues with Younium API
When making API calls, you might encounter errors. Here are some common issues and how to handle them:
- 400 Bad Request: Indicates invalid input data. Check the request body for any missing or incorrect fields.
- 401 Unauthorized: Ensure your JWT access token is valid and not expired.
- 403 Forbidden: Verify that the legal entity specified in the headers is correct and that you have the necessary permissions.
For detailed error descriptions, refer to the Younium API Documentation.
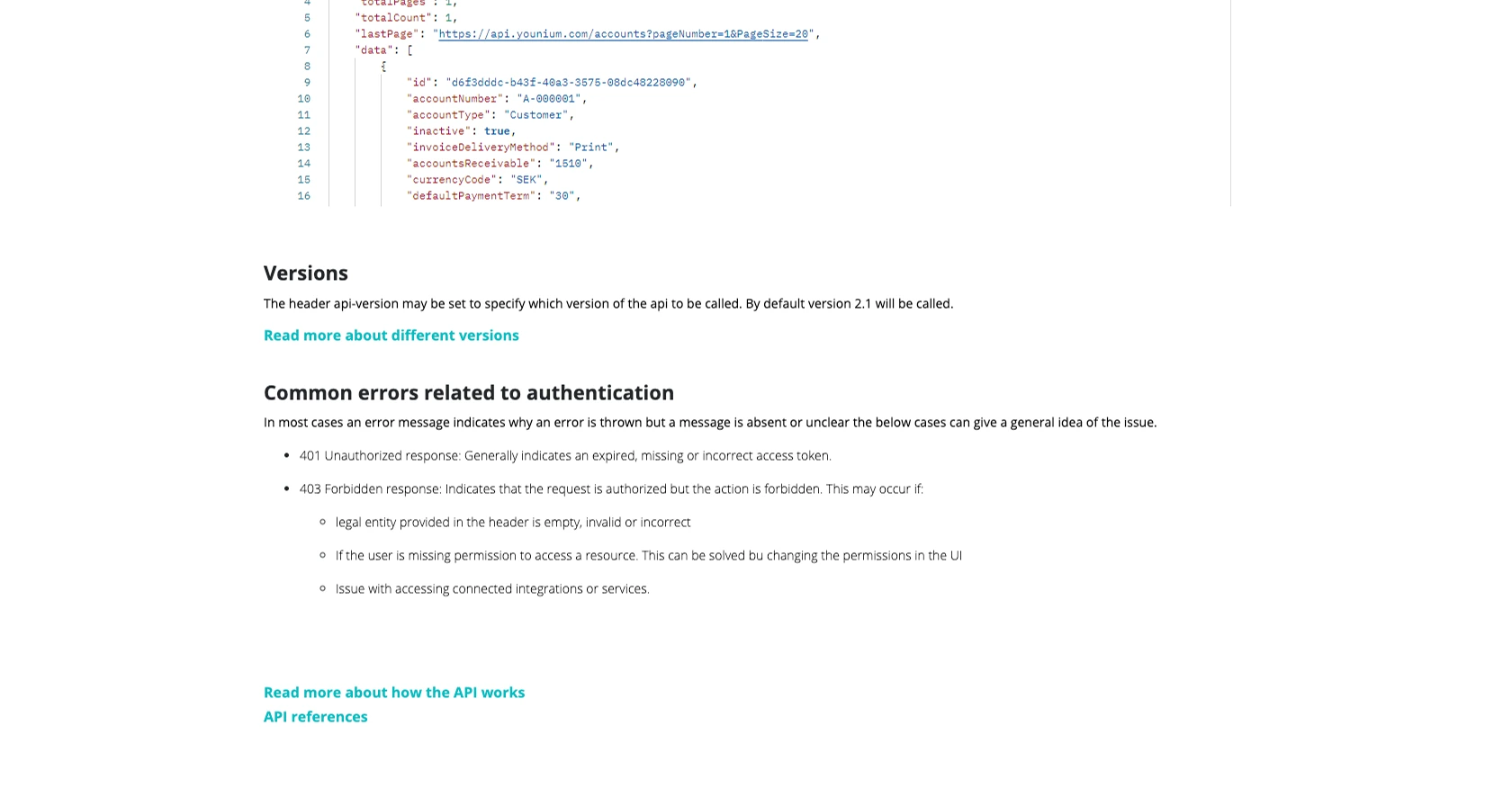
Conclusion and Best Practices for Using Younium API in JavaScript
Integrating with the Younium API provides a powerful way to automate and streamline your invoicing processes. By following the steps outlined in this guide, you can efficiently create and update invoices, ensuring accurate financial management for your B2B SaaS operations.
Best Practices for Secure and Efficient Younium API Integration
- Secure Storage of Credentials: Always store your API credentials securely. Consider using environment variables or secure vaults to protect sensitive information like the Client ID, Secret Key, and JWT access tokens.
- Handle Rate Limiting: Be mindful of the API's rate limits to avoid throttling. Implement retry logic with exponential backoff to manage requests efficiently.
- Data Standardization: Ensure that the data fields in your API requests are standardized and validated to prevent errors and maintain consistency across your invoicing system.
- Regular Token Refresh: Since JWT tokens expire after 24 hours, implement a mechanism to refresh tokens automatically to maintain uninterrupted API access.
Enhancing Integration Capabilities with Endgrate
For developers looking to simplify and expand their integration capabilities, consider leveraging Endgrate. With Endgrate, you can manage multiple integrations through a single API endpoint, saving time and resources. Focus on your core product while Endgrate handles the complexities of integration, providing an intuitive experience for your customers.
Explore how Endgrate can streamline your integration processes by visiting Endgrate today.
Read More
Ready to get started?