How to Send Emails with the SendGrid API in PHP
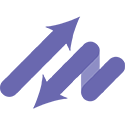
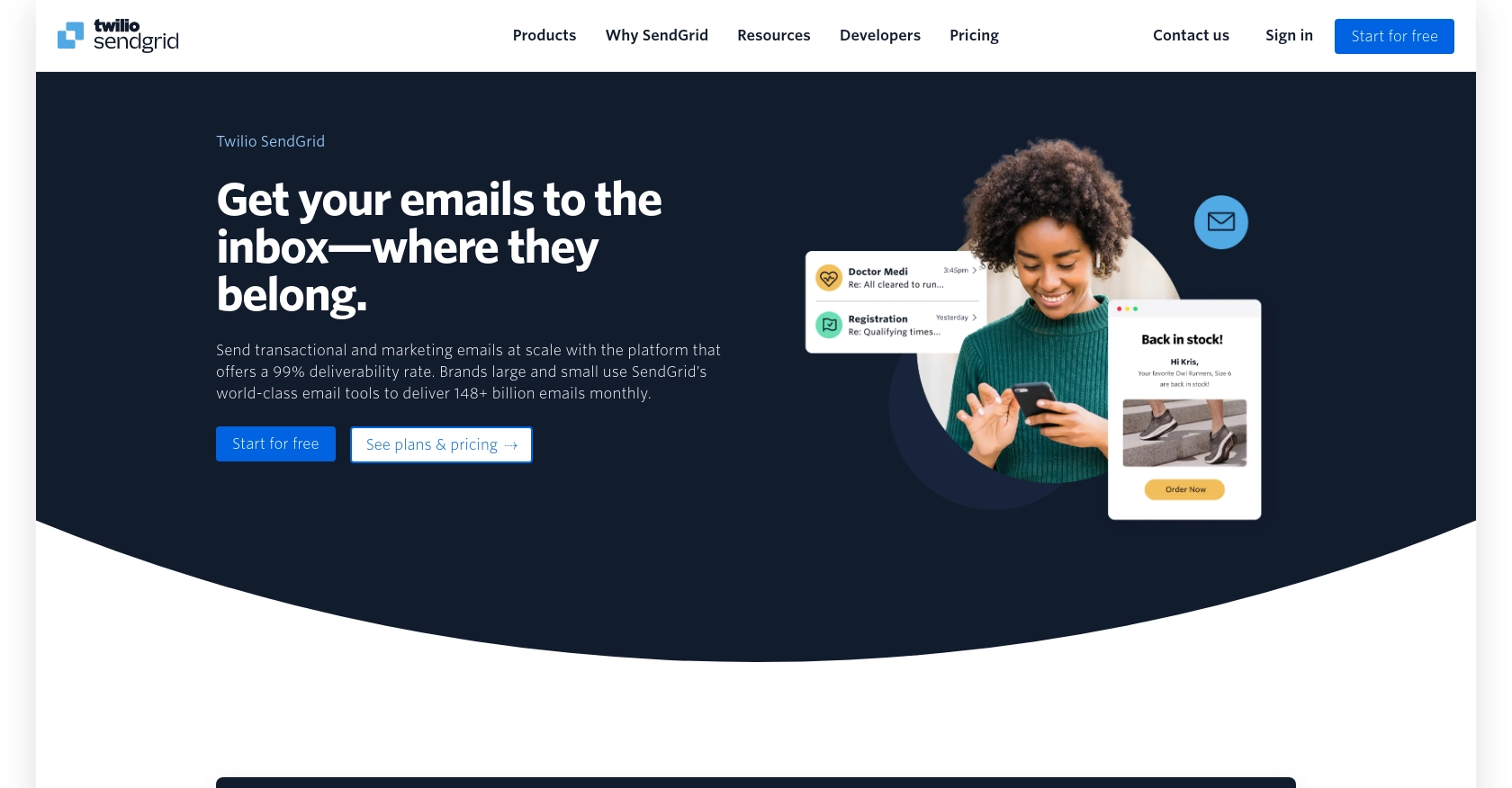
Introduction to SendGrid Email API
SendGrid is a powerful cloud-based email delivery service that helps businesses send transactional and marketing emails with ease. Known for its reliability and scalability, SendGrid is trusted by developers and marketers worldwide to ensure their emails reach the inbox.
Integrating with SendGrid's API allows developers to automate email sending processes, enhancing communication efficiency. For example, you can use the SendGrid API to send personalized order confirmations or newsletters directly from your application, ensuring timely and relevant communication with your users.
Setting Up Your SendGrid Test Account
Before you can start sending emails using the SendGrid API in PHP, you'll need to set up a SendGrid account. This setup process is straightforward and allows you to access the API key required for authentication.
Creating a SendGrid Account
If you don't already have a SendGrid account, follow these steps to create one:
- Visit the SendGrid website and click on the "Sign Up" button.
- Choose the free plan to get started, which provides you with access to essential features and a limited number of emails per month.
- Fill in the required information, including your email address and password, to create your account.
- Verify your email address by clicking on the link sent to your registered email.
Generating a SendGrid API Key
Once your account is set up, you need to generate an API key to authenticate your requests:
- Log in to your SendGrid account and navigate to the "Settings" section in the left-hand menu.
- Click on "API Keys" and then "Create API Key."
- Provide a name for your API key, such as "PHP Email Integration."
- Select the permissions you require. For sending emails, you can choose "Full Access" or customize the permissions as needed.
- Click "Create & View" to generate your API key. Make sure to copy and store this key securely, as it will not be displayed again.
Testing Your SendGrid Setup
To ensure your SendGrid setup is working correctly, you can send a test email:
- Use the SendGrid dashboard to send a test email to your verified email address.
- Check your inbox to confirm that the email was delivered successfully.
With your SendGrid account and API key ready, you can now proceed to integrate the SendGrid API with your PHP application.
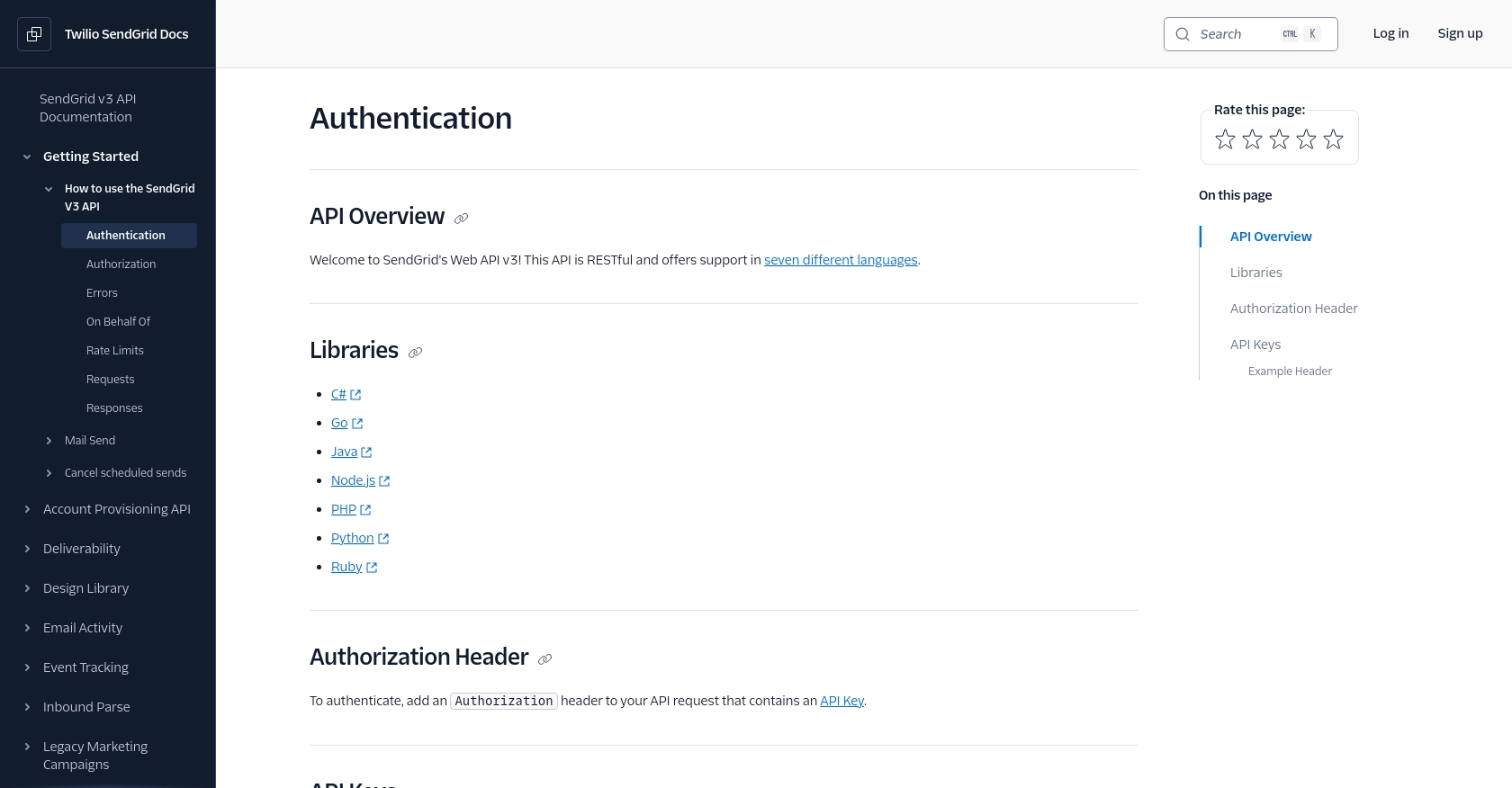
sbb-itb-96038d7
How to Make an API Call to Send Emails with SendGrid in PHP
To send emails using the SendGrid API in PHP, you need to set up your environment and write the necessary code to interact with the API. This section will guide you through the process of making an API call to SendGrid using PHP, ensuring you can send emails efficiently and effectively.
Setting Up Your PHP Environment for SendGrid API
Before making an API call, ensure your PHP environment is correctly configured. You will need PHP 7.4 or later and the Composer package manager to install dependencies.
- Ensure PHP is installed on your system. You can check your PHP version by running
php -v
in your terminal. - Install Composer by following the instructions on the Composer website.
Installing the SendGrid PHP Library
To interact with the SendGrid API, you need to install the SendGrid PHP library. Use Composer to add this library to your project:
composer require sendgrid/sendgrid
Writing PHP Code to Send an Email with SendGrid
With the library installed, you can write PHP code to send an email using the SendGrid API. Create a new PHP file, for example, send_email.php
, and add the following code:
require 'vendor/autoload.php';
$sendgrid = new \SendGrid('YOUR_API_KEY');
$email = new \SendGrid\Mail\Mail();
$email->setFrom("you@example.com", "Your Name");
$email->setSubject("Sending with SendGrid is Fun");
$email->addTo("recipient@example.com", "Recipient Name");
$email->addContent("text/plain", "and easy to do anywhere, even with PHP");
$email->addContent(
"text/html", "and easy to do anywhere, even with PHP"
);
try {
$response = $sendgrid->send($email);
echo 'Email sent successfully. Status Code: ' . $response->statusCode();
} catch (Exception $e) {
echo 'Caught exception: ' . $e->getMessage();
}
Replace 'YOUR_API_KEY'
with the API key you generated earlier. This code sets up the email details, including the sender, recipient, subject, and content. It then sends the email using the SendGrid client.
Verifying the API Call and Handling Errors
After running your script, check your inbox to verify that the email was sent successfully. If there are any issues, the script will catch exceptions and display error messages.
Common error codes include:
- 401 Unauthorized: Check your API key and permissions.
- 403 Forbidden: Ensure your account has the necessary permissions.
- 429 Too Many Requests: You have exceeded the rate limit. Refer to the SendGrid rate limits documentation for more information.
For more detailed error handling, refer to the SendGrid API documentation.
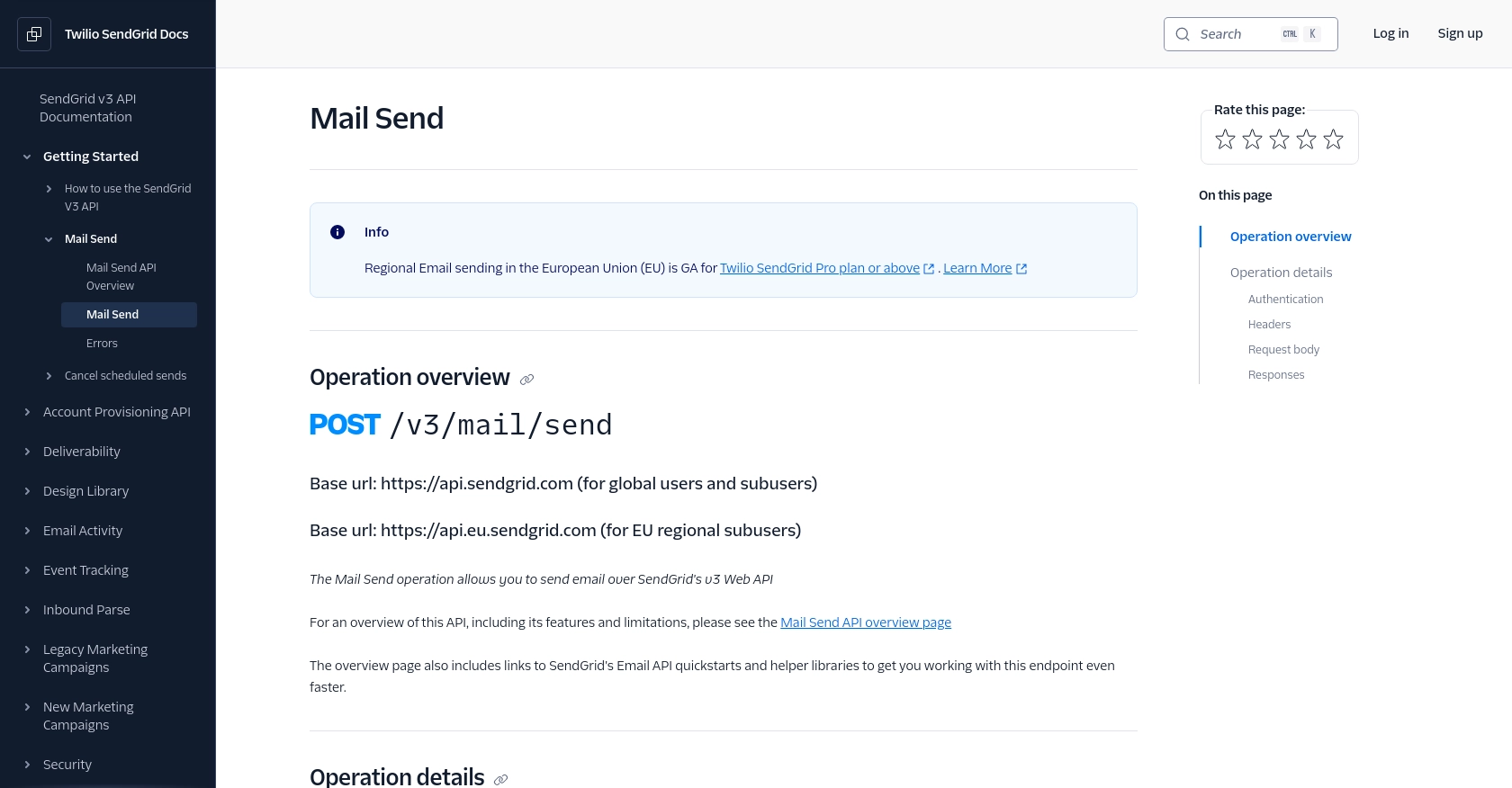
Conclusion and Best Practices for Using SendGrid API in PHP
Integrating the SendGrid API with your PHP application can significantly enhance your email communication strategy by automating the sending of transactional and marketing emails. By following the steps outlined in this guide, you can efficiently set up your environment, authenticate using API keys, and send emails using the SendGrid PHP library.
Best Practices for Secure and Efficient Email Integration with SendGrid
- Secure API Key Management: Always store your SendGrid API keys securely. Avoid hardcoding them in your source code. Instead, use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limits: Be mindful of SendGrid's rate limits to prevent service interruptions. Implement logic to handle status code 429 errors gracefully by retrying requests after a delay. For more details, refer to the SendGrid rate limits documentation.
- Error Handling: Implement robust error handling in your application to manage API response errors effectively. This includes checking for common error codes such as 401, 403, and 429, and taking appropriate actions.
- Data Transformation: Ensure that your email content is properly formatted and validated before sending. Use SendGrid's dynamic templates for personalized and consistent email content.
Streamlining Email Integrations with Endgrate
While integrating with SendGrid is straightforward, managing multiple integrations can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint for various platforms, including SendGrid. This allows you to focus on your core product while outsourcing integration complexities.
With Endgrate, you can build once for each use case and leverage an intuitive integration experience for your customers. Explore how Endgrate can help you save time and resources by visiting Endgrate.
Read More
- https://endgrate.com/provider/sendgrid
- https://www.twilio.com/docs/sendgrid/api-reference/how-to-use-the-sendgrid-v3-api/authentication
- https://www.twilio.com/docs/sendgrid/api-reference/how-to-use-the-sendgrid-v3-api/authorization
- https://www.twilio.com/docs/sendgrid/api-reference/how-to-use-the-sendgrid-v3-api/rate-limits
- https://www.twilio.com/docs/sendgrid/api-reference/mail-send/mail-send
Ready to get started?