Using the Microsoft Dynamics 365 API to Create or Update Account in PHP
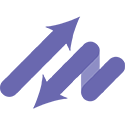
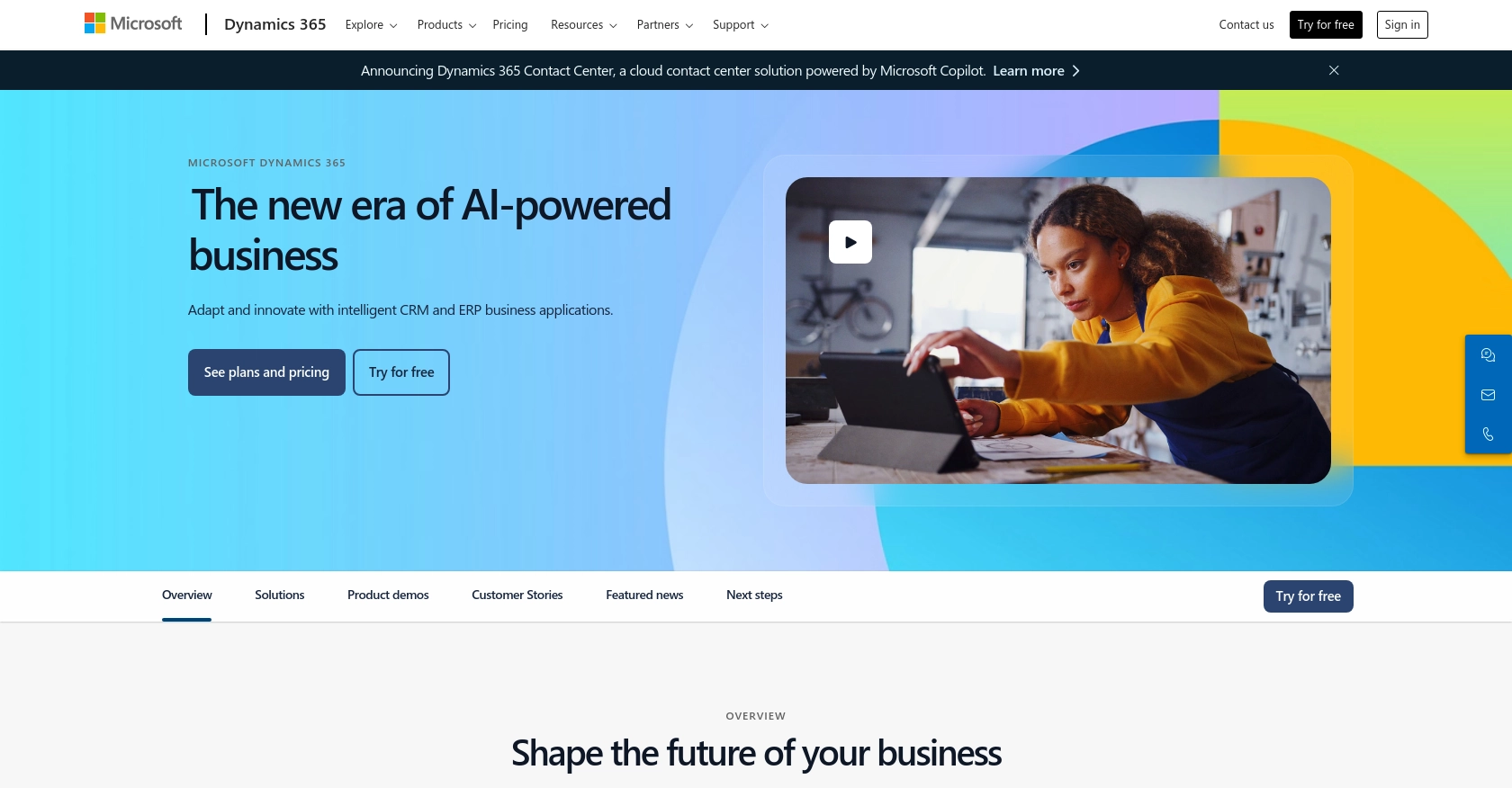
Introduction to Microsoft Dynamics 365 API
Microsoft Dynamics 365 is a comprehensive suite of business applications designed to enhance customer engagement and streamline operations. It integrates CRM and ERP capabilities, offering tools for sales, customer service, finance, and operations. This makes it a powerful platform for businesses looking to improve efficiency and customer satisfaction.
Developers may want to connect with Microsoft Dynamics 365 to automate and manage business processes, such as creating or updating accounts. For example, a developer could use the Microsoft Dynamics 365 API to automatically update account information from an external database, ensuring that all customer data is current and accurate.
Setting Up a Microsoft Dynamics 365 Test or Sandbox Account
Before you can start integrating with the Microsoft Dynamics 365 API, you'll need to set up a test or sandbox account. This environment allows you to safely develop and test your applications without affecting live data.
Registering for a Microsoft Dynamics 365 Sandbox Account
If you don't already have a Microsoft Dynamics 365 account, you can sign up for a free trial or a sandbox account. Follow these steps to get started:
- Visit the Microsoft Dynamics 365 website and navigate to the trial or sandbox registration page.
- Fill out the registration form with your details and submit it.
- Once registered, you'll receive an email with instructions to access your sandbox environment.
Creating an App for OAuth Authentication
Microsoft Dynamics 365 uses OAuth 2.0 for authentication. To interact with the API, you need to register an application in your Microsoft Entra ID tenant. Here's how:
- Go to the Azure Portal and sign in with your Microsoft account.
- Navigate to "Azure Active Directory" and select "App registrations".
- Click on "New registration" and fill in the necessary details such as the name of your app.
- Set the "Redirect URI" to a valid URL, such as
https://localhost
, for development purposes. - After registration, note down the "Application (client) ID" and "Directory (tenant) ID".
Generating Client Secret for Microsoft Dynamics 365 API
To authenticate your application, you need a client secret. Follow these steps to generate one:
- In the Azure Portal, navigate to your registered app and select "Certificates & secrets".
- Under "Client secrets", click on "New client secret".
- Provide a description and select an expiration period for the secret.
- Click "Add" and copy the generated secret value. Store it securely as it will not be shown again.
Configuring API Permissions for Microsoft Dynamics 365
To access the Microsoft Dynamics 365 API, you need to configure the appropriate permissions:
- In your app registration, go to "API permissions".
- Click on "Add a permission" and select "Dynamics CRM".
- Choose the necessary permissions, such as "user_impersonation", to allow your app to access the API on behalf of a user.
- Click "Add permissions" and ensure admin consent is granted for these permissions.
With your sandbox account and app registration set up, you're ready to start integrating with the Microsoft Dynamics 365 API using PHP.
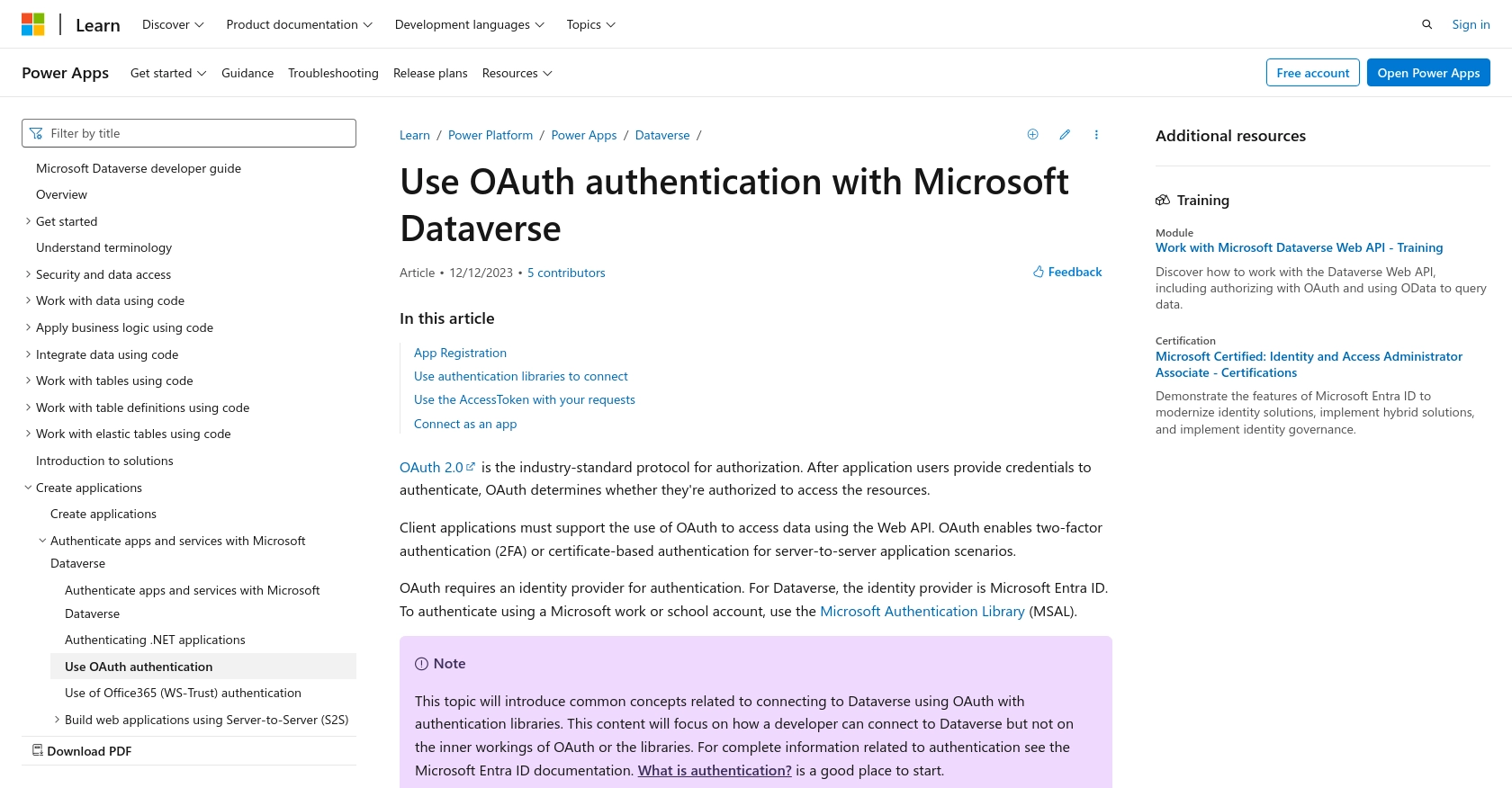
sbb-itb-96038d7
Making API Calls to Microsoft Dynamics 365 Using PHP
To interact with the Microsoft Dynamics 365 API using PHP, you'll need to set up your environment and write code to create or update accounts. This section will guide you through the necessary steps, including setting up PHP, installing dependencies, and writing the code to make API requests.
Setting Up PHP Environment for Microsoft Dynamics 365 API Integration
Before making API calls, ensure you have the following prerequisites installed on your machine:
- PHP 7.4 or higher
- Composer, the PHP package manager
To install Composer, follow the instructions on the Composer download page.
Installing Required PHP Libraries for Microsoft Dynamics 365 API
You'll need the Guzzle HTTP client to make HTTP requests to the Microsoft Dynamics 365 API. Install it using Composer with the following command:
composer require guzzlehttp/guzzle
Writing PHP Code to Create or Update an Account in Microsoft Dynamics 365
With your environment set up, you can now write the PHP code to interact with the Microsoft Dynamics 365 API. Below is a sample script to create or update an account:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$accessToken = 'Your_Access_Token'; // Replace with your access token
// Define the API endpoint and headers
$endpoint = 'https://yourorg.api.crm.dynamics.com/api/data/v9.2/accounts';
$headers = [
'Authorization' => 'Bearer ' . $accessToken,
'Content-Type' => 'application/json',
'OData-MaxVersion' => '4.0',
'OData-Version' => '4.0',
'Accept' => 'application/json'
];
// Define the account data
$accountData = [
'name' => 'New Account Name',
'accountnumber' => '12345',
'telephone1' => '555-1234'
];
try {
// Make a POST request to create a new account
$response = $client->post($endpoint, [
'headers' => $headers,
'json' => $accountData
]);
if ($response->getStatusCode() == 204) {
echo "Account created successfully.";
} else {
echo "Failed to create account. Status code: " . $response->getStatusCode();
}
} catch (Exception $e) {
echo "Error: " . $e->getMessage();
}
Replace Your_Access_Token
with the access token obtained during authentication. This script uses Guzzle to send a POST request to the Microsoft Dynamics 365 API, creating a new account with the specified data.
Verifying API Request Success in Microsoft Dynamics 365
After running the script, verify the success of the API request by checking the Microsoft Dynamics 365 sandbox environment. The newly created or updated account should appear in the accounts list.
Handling Errors and Common Error Codes in Microsoft Dynamics 365 API
When making API calls, you may encounter errors. Common HTTP status codes include:
- 400 Bad Request: The request was invalid. Check the request parameters.
- 401 Unauthorized: Authentication failed. Verify the access token.
- 403 Forbidden: The request is not allowed. Check API permissions.
- 404 Not Found: The requested resource does not exist.
- 500 Internal Server Error: An error occurred on the server. Try again later.
For more detailed error handling, refer to the Microsoft Dynamics 365 API documentation.
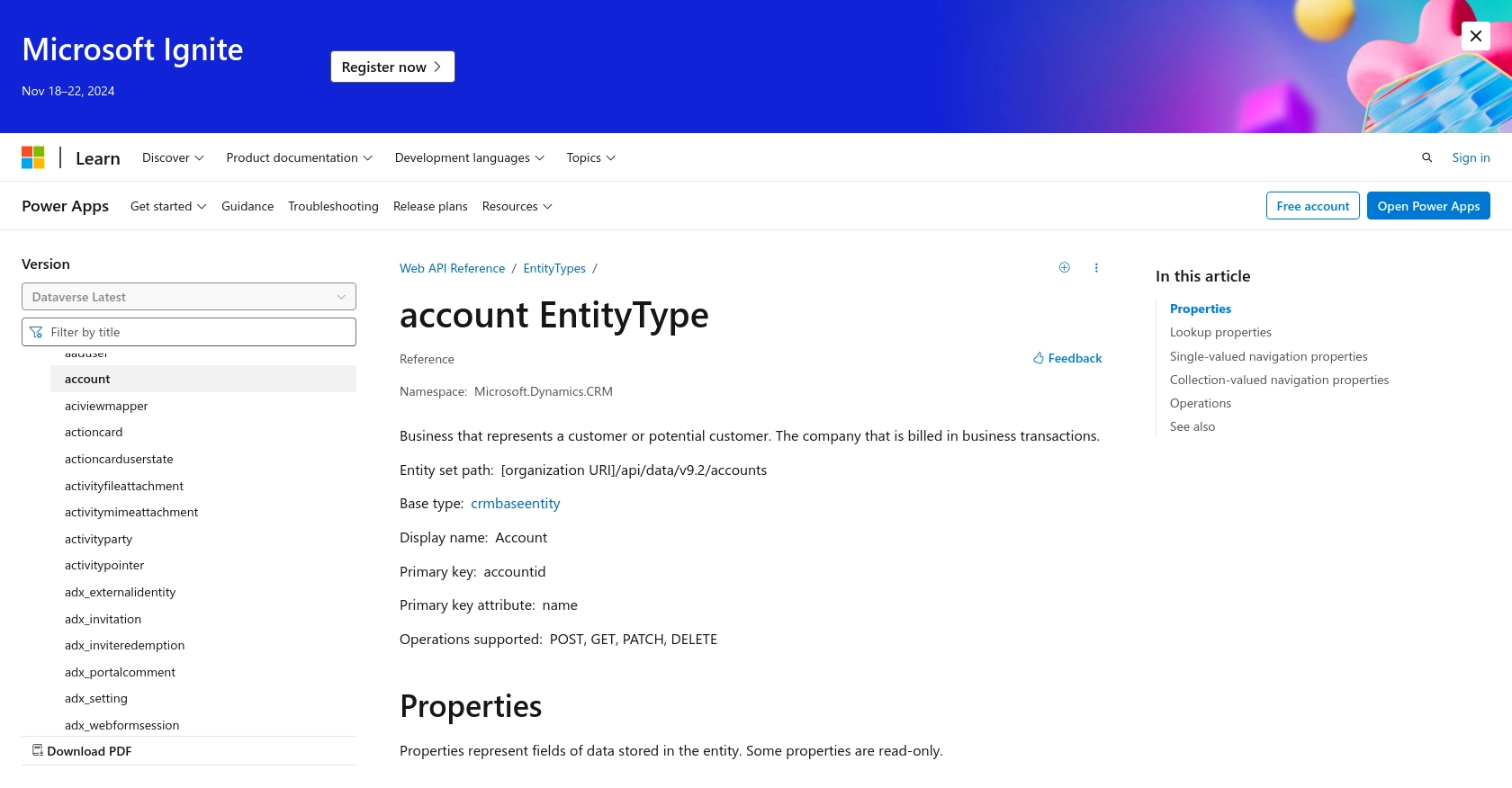
Best Practices for Using Microsoft Dynamics 365 API in PHP
When working with the Microsoft Dynamics 365 API, it's crucial to follow best practices to ensure secure and efficient integration. Here are some recommendations:
- Securely Store Credentials: Always store your client ID, client secret, and access tokens securely. Use environment variables or secure vaults to prevent unauthorized access.
- Handle Rate Limiting: Microsoft Dynamics 365 may impose rate limits on API requests. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Standardization: Ensure that data fields are standardized and validated before sending them to the API. This helps maintain data integrity and prevents errors.
- Monitor API Usage: Regularly monitor your API usage and performance. Use logging and analytics tools to track requests and identify potential issues.
Streamlining Integrations with Endgrate
Building and maintaining integrations with platforms like Microsoft Dynamics 365 can be time-consuming and complex. Endgrate simplifies this process by providing a unified API endpoint that connects to multiple platforms, including Microsoft Dynamics 365.
With Endgrate, you can:
- Save time and resources by outsourcing integrations and focusing on your core product.
- Build once for each use case instead of multiple times for different integrations.
- Offer an intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate.
Read More
- https://endgrate.com/provider/microsoftdynamics365
- https://learn.microsoft.com/en-us/power-apps/developer/data-platform/authenticate-oauth
- https://learn.microsoft.com/en-us/graph/use-the-api
- https://learn.microsoft.com/en-us/power-apps/developer/data-platform/webapi/reference/account?view=dataverse-latest
Ready to get started?