Using the BigCommerce API to Get Customers (with Python examples)
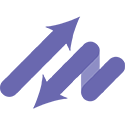
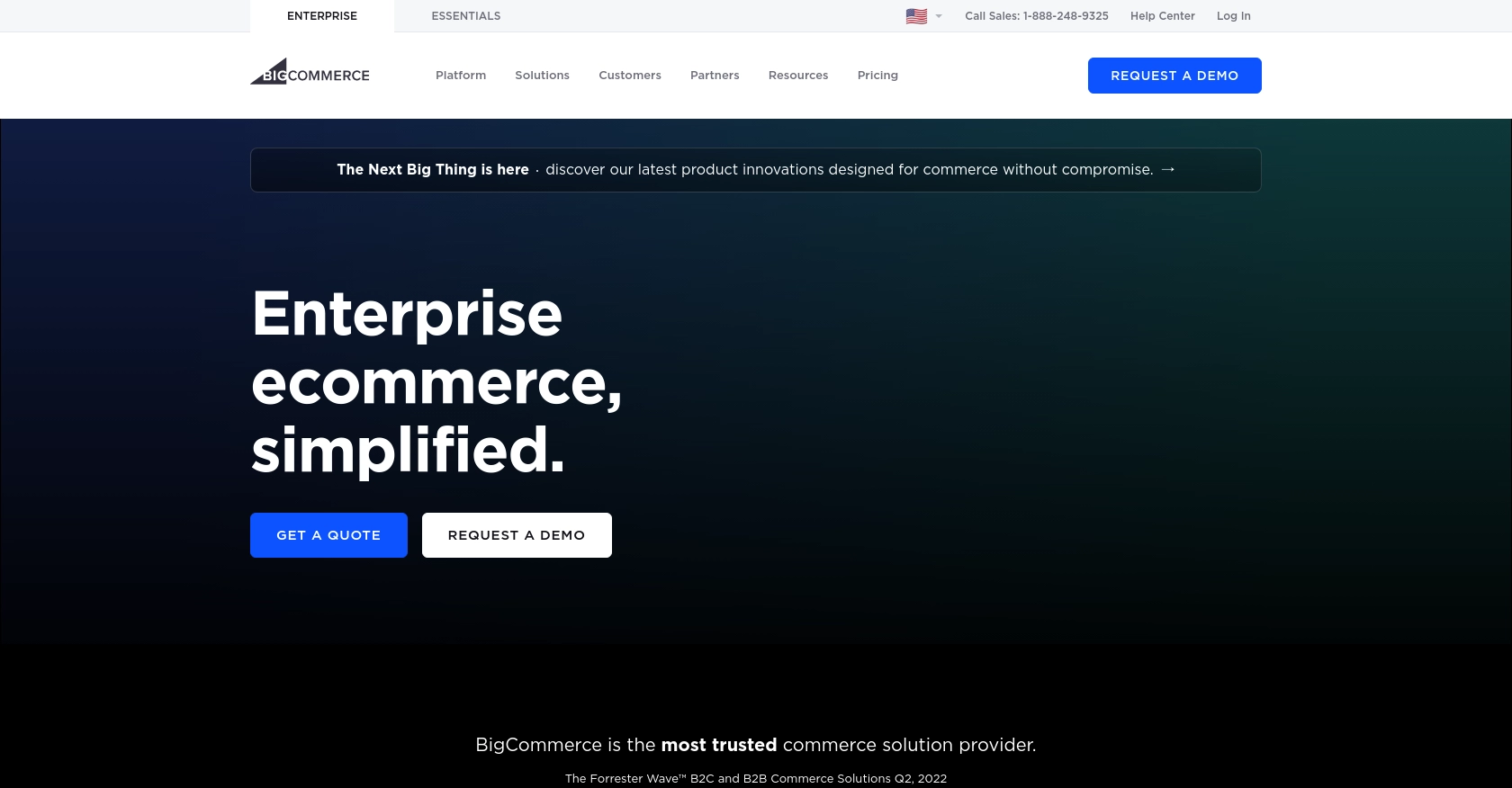
Introduction to BigCommerce API Integration
BigCommerce is a powerful e-commerce platform that provides businesses with the tools they need to create and manage online stores. Known for its scalability and flexibility, BigCommerce offers a range of features including product management, order processing, and customer engagement tools.
Integrating with the BigCommerce API allows developers to access and manipulate store data programmatically. This can be particularly useful for automating tasks such as retrieving customer information, which can enhance customer relationship management and streamline marketing efforts. For example, a developer might use the BigCommerce API to fetch customer data and integrate it with a CRM system to personalize marketing campaigns.
Setting Up Your BigCommerce Test Account
Before you can start interacting with the BigCommerce API, you need to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting a live store. Follow these steps to get started:
Create a BigCommerce Sandbox Account
If you don't already have a BigCommerce account, you can sign up for a sandbox account. This is a free version that provides the necessary tools for testing and development.
- Visit the BigCommerce Sandbox Signup Page.
- Fill out the registration form with your details and submit it.
- Once your account is created, log in to access your sandbox store.
Generate API Credentials for BigCommerce
To interact with the BigCommerce API, you'll need to generate API credentials. These include an access token that will authenticate your requests.
- Navigate to the API Accounts section in your BigCommerce dashboard.
- Click on Create API Account and select V2/V3 API Token.
- Provide a name for your API account and select the necessary OAuth scopes for accessing customer data.
- Click Save to generate your API credentials, including the Client ID and Access Token.
- Store these credentials securely, as you'll need them to authenticate your API requests.
Configure OAuth Scopes for BigCommerce API
Ensure that your API account has the correct OAuth scopes configured to access customer data. This is crucial for successful API interactions.
- In the API account settings, review the list of available scopes.
- Select the scopes that correspond to the customer data you wish to access, such as Customers and Orders.
- Save your changes to apply the selected scopes to your API account.
With your sandbox account and API credentials set up, you're ready to start making API calls to BigCommerce. In the next section, we'll explore how to use Python to interact with the BigCommerce API and retrieve customer data.
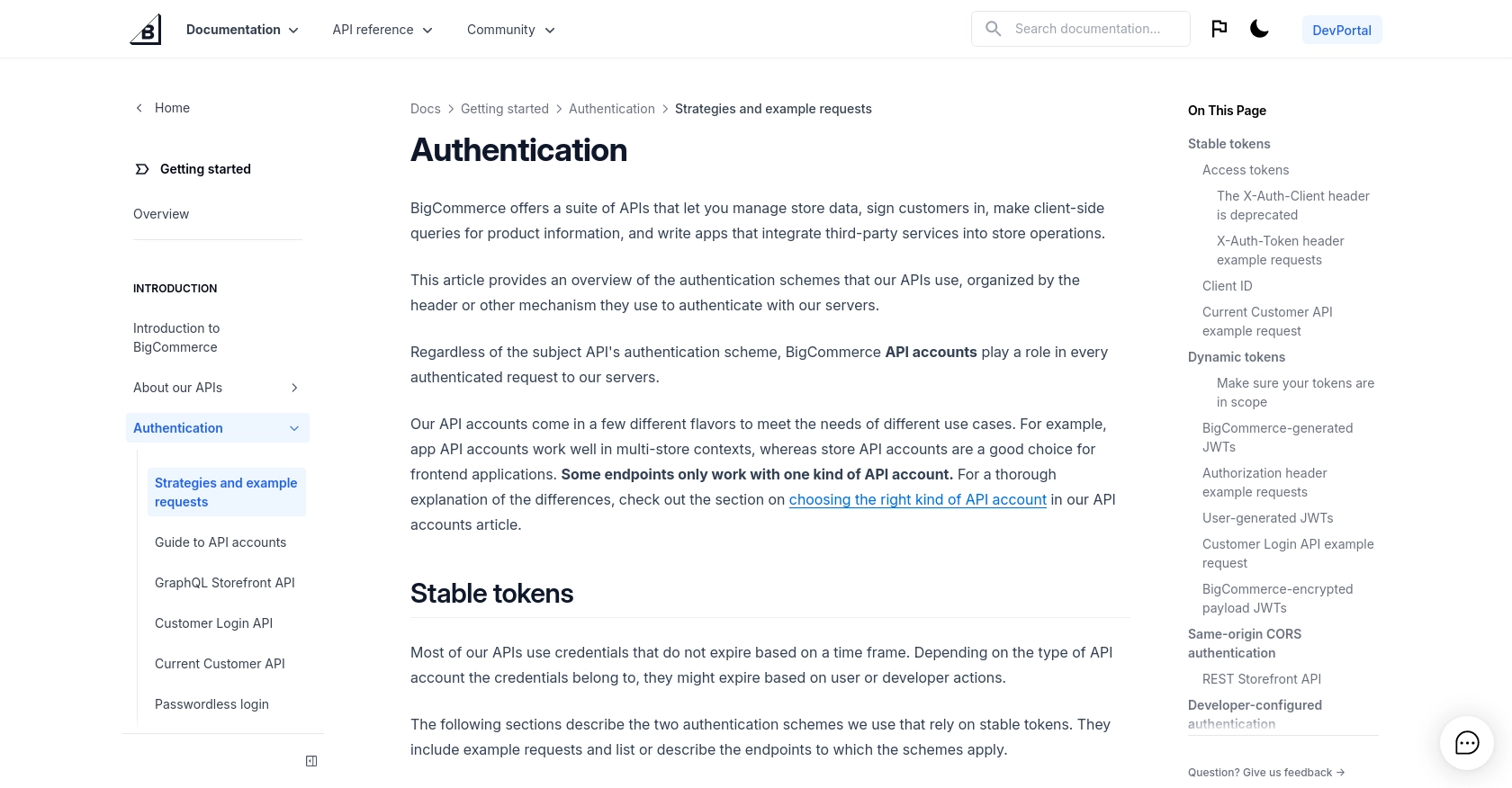
sbb-itb-96038d7
Making API Calls to Retrieve Customers from BigCommerce Using Python
Once you have your BigCommerce sandbox account and API credentials ready, you can start making API calls to retrieve customer data. In this section, we'll guide you through the process of using Python to interact with the BigCommerce API.
Prerequisites for Using Python with BigCommerce API
Before you begin, ensure you have the following installed on your machine:
- Python 3.11.1 or later
- The Python package installer, pip
You'll also need to install the requests
library to handle HTTP requests. You can do this by running the following command in your terminal:
pip install requests
Setting Up Your Python Script to Fetch Customers
Create a new Python file named get_bigcommerce_customers.py
and add the following code to it:
import requests
# Define the API endpoint and headers
store_hash = 'your_store_hash'
endpoint = f"https://api.bigcommerce.com/stores/{store_hash}/v3/customers"
headers = {
"X-Auth-Token": "your_access_token",
"Accept": "application/json"
}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
customers = response.json()
for customer in customers['data']:
print(f"Customer ID: {customer['id']}, Email: {customer['email']}")
else:
print(f"Failed to retrieve customers: {response.status_code} - {response.text}")
Replace your_store_hash
and your_access_token
with your actual store hash and access token obtained from the BigCommerce dashboard.
Running the Python Script and Verifying the Output
Execute the script by running the following command in your terminal:
python get_bigcommerce_customers.py
If successful, the script will output a list of customers with their IDs and email addresses. You can verify the retrieved data by cross-checking it with the customer information in your BigCommerce sandbox account.
Handling Errors and Understanding Response Codes
When making API calls, it's crucial to handle potential errors gracefully. The BigCommerce API may return various status codes indicating the success or failure of your request:
- 200 OK: The request was successful, and the response contains the requested data.
- 401 Unauthorized: The request lacks valid authentication credentials. Check your access token.
- 403 Forbidden: The server understood the request, but it refuses to authorize it. Verify your OAuth scopes.
- 404 Not Found: The requested resource could not be found. Ensure the endpoint URL is correct.
For more detailed information on error codes, refer to the BigCommerce authentication documentation.
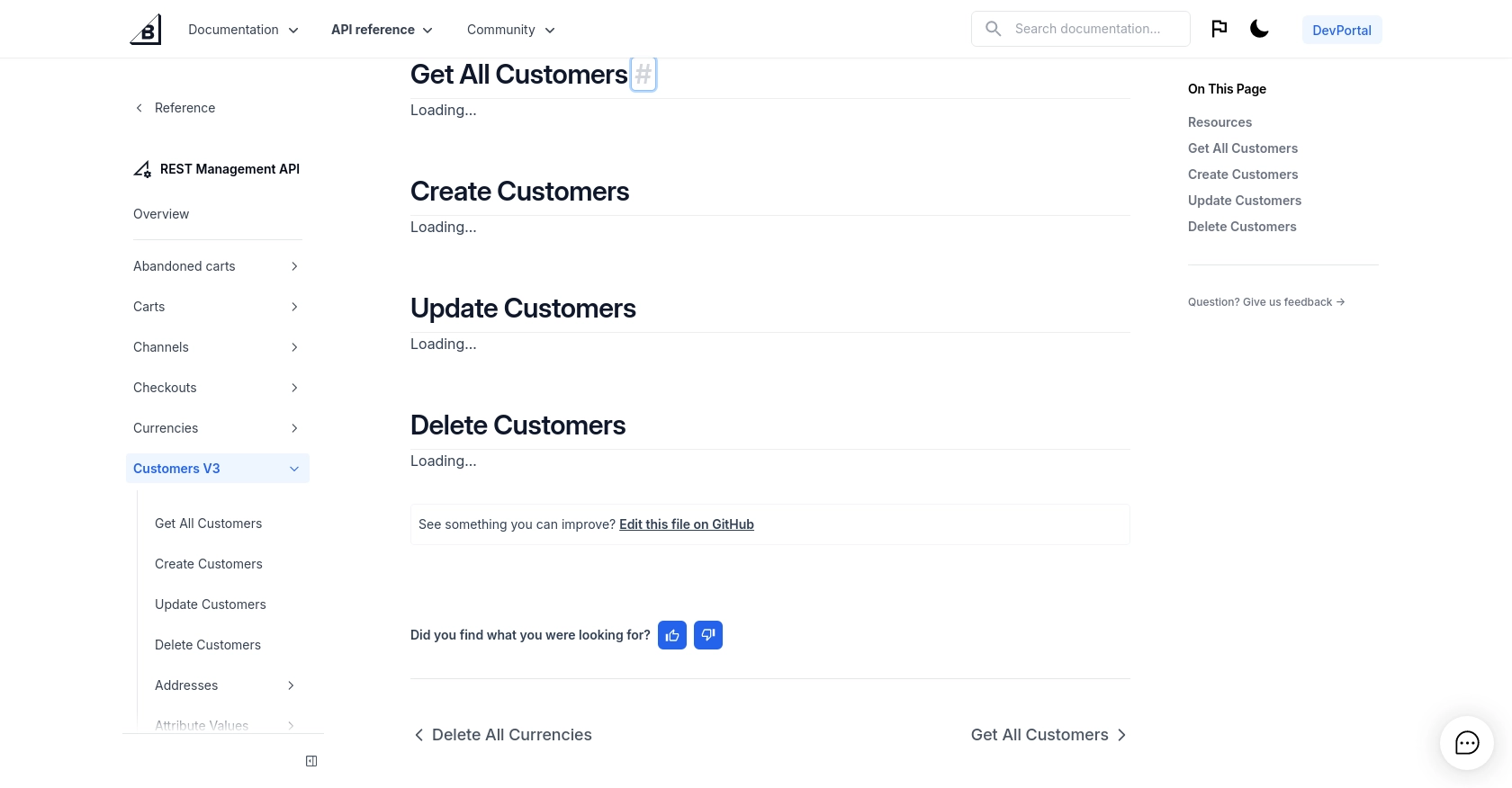
Conclusion and Best Practices for Using BigCommerce API with Python
Integrating with the BigCommerce API using Python provides a powerful way to automate and enhance your e-commerce operations. By following the steps outlined in this guide, you can efficiently retrieve customer data and integrate it with other systems to improve customer relationship management and marketing strategies.
Best Practices for Secure and Efficient BigCommerce API Integration
- Secure Storage of Credentials: Always store your API credentials, such as the access token, securely. Consider using environment variables or a secure vault to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of BigCommerce's API rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit responses gracefully.
- Data Transformation and Standardization: When integrating customer data with other systems, ensure that data fields are transformed and standardized to maintain consistency across platforms.
- Regularly Review OAuth Scopes: Periodically review and update the OAuth scopes for your API account to ensure they align with your current integration needs and adhere to the principle of least privilege.
Streamlining Integrations with Endgrate
While integrating with the BigCommerce API can be straightforward, managing multiple integrations across different platforms can become complex and time-consuming. Endgrate offers a solution by providing a unified API endpoint that connects to various platforms, including BigCommerce.
By leveraging Endgrate, you can save time and resources by building once for each use case instead of multiple times for different integrations. This allows you to focus on your core product while providing an easy and intuitive integration experience for your customers.
Explore how Endgrate can simplify your integration process by visiting Endgrate's website and discover how it can benefit your development workflow.
Read More
Ready to get started?