Using the Shopify API to Get Customers (with Python examples)
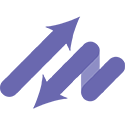
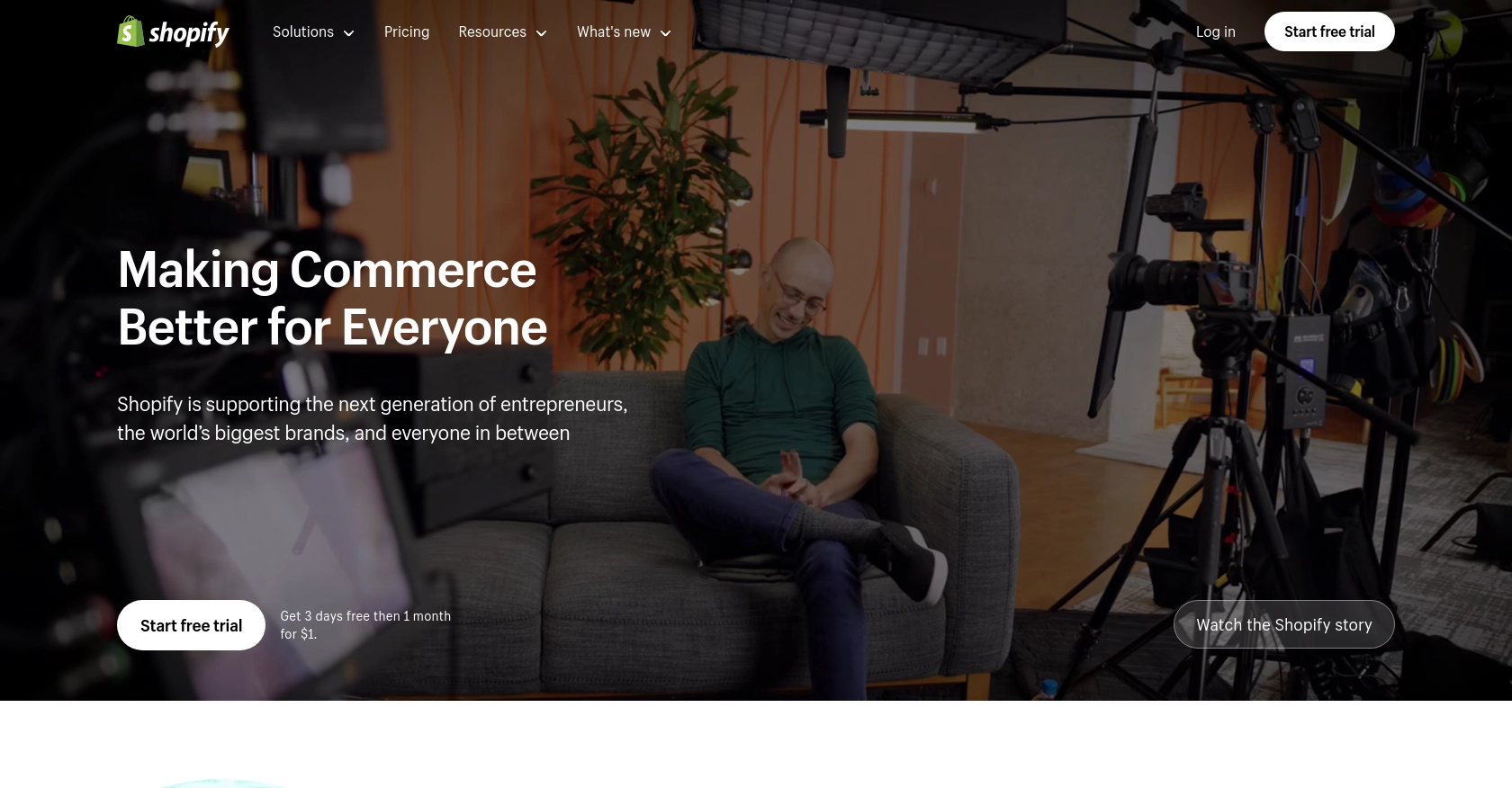
Introduction to Shopify API Integration
Shopify is a leading e-commerce platform that empowers businesses to create and manage online stores with ease. It offers a comprehensive suite of tools for managing products, processing orders, and engaging with customers, making it a popular choice for businesses of all sizes.
Integrating with Shopify's API allows developers to access and manipulate store data programmatically, enabling automation and customization of various e-commerce processes. For example, a developer might use the Shopify API to retrieve customer information and analyze purchasing patterns, helping businesses tailor their marketing strategies and improve customer engagement.
This article will guide you through using Python to interact with Shopify's API, specifically focusing on retrieving customer data. By following this tutorial, you'll learn how to efficiently access and manage customer information on the Shopify platform using Python.
Setting Up Your Shopify Test Account for API Integration
Before diving into the Shopify API, you'll need to set up a test or sandbox account. This allows you to safely experiment with API calls without affecting a live store. Shopify provides a development store option through its Partner Dashboard, which is perfect for testing and development purposes.
Creating a Shopify Partner Account
To get started, you'll need a Shopify Partner account. Follow these steps to create one:
- Visit the Shopify Partners page and sign up for an account.
- Fill in the required information and submit your application.
- Once approved, log in to your Shopify Partner Dashboard.
Setting Up a Development Store
With your Partner account ready, you can now create a development store:
- In the Partner Dashboard, navigate to the Stores section.
- Click on Add store and select Development store.
- Fill in the store details, such as store name and password, and click Create store.
Your development store is now ready for testing API integrations.
Creating a Shopify App for OAuth Authentication
Shopify uses OAuth for authentication, which requires creating an app to obtain the necessary credentials:
- In your Partner Dashboard, go to Apps and click Create app.
- Enter the app name and select the development store you created earlier.
- Under App setup, note down the API key and API secret key. These will be used for authentication.
- Set the App URL and Redirect URLs to your development environment.
Configuring OAuth Scopes
To access customer data, you'll need to configure the appropriate OAuth scopes:
- In the app settings, navigate to API scopes.
- Select the read_customers and write_customers scopes to allow access to customer data.
- Save your changes.
With your Shopify app configured, you're ready to authenticate and start making API calls to retrieve customer data. For more details on authentication, refer to the Shopify API authentication documentation.
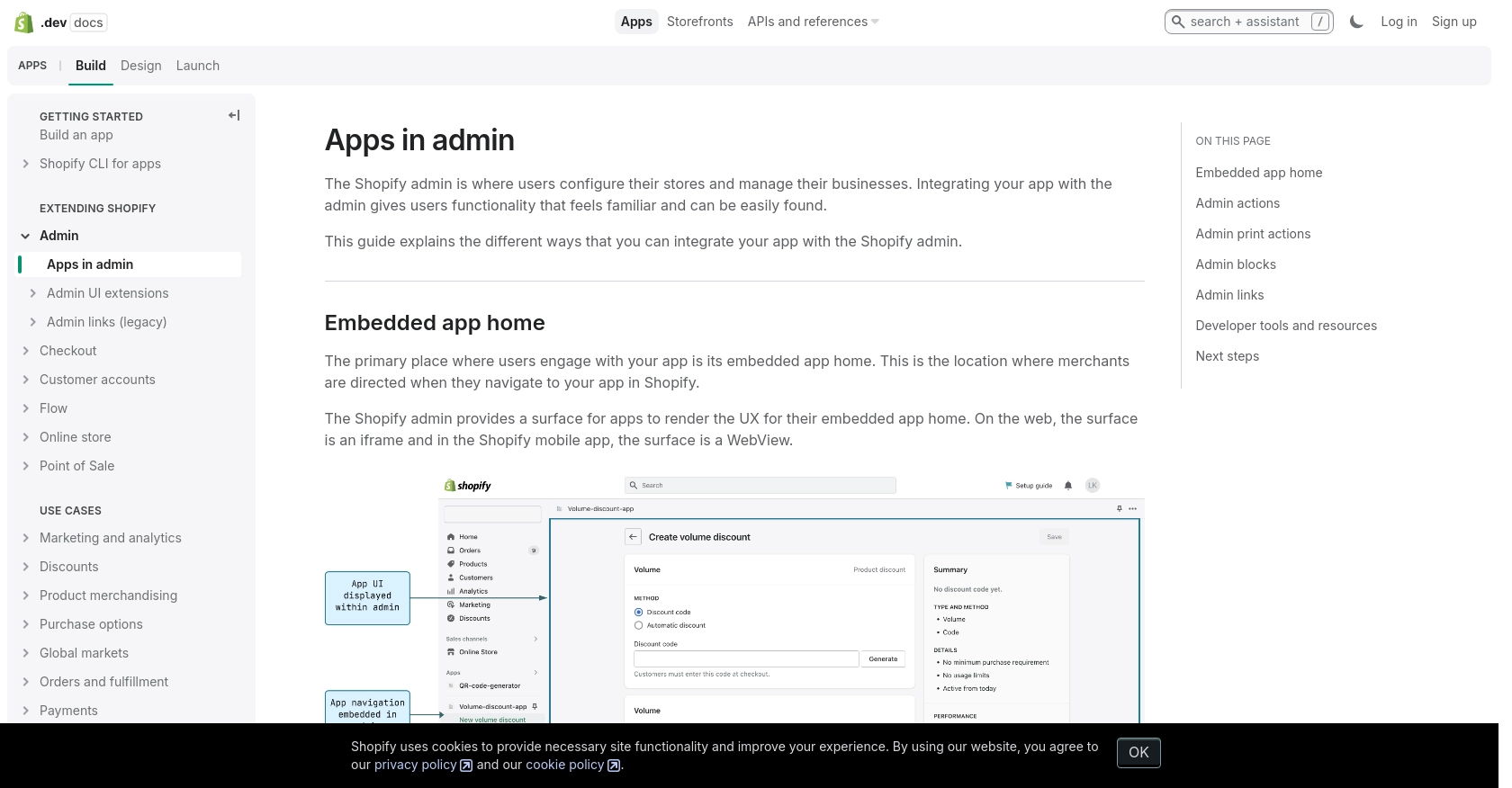
sbb-itb-96038d7
Making API Calls to Retrieve Shopify Customer Data Using Python
To interact with Shopify's API and retrieve customer data, you'll need to use Python. This section will guide you through the process of setting up your Python environment, making API calls, and handling responses effectively.
Setting Up Your Python Environment for Shopify API Integration
Before making API calls, ensure you have the following prerequisites installed:
- Python 3.11.1 or later
- The Python package installer
pip
Once installed, open your terminal or command prompt and install the requests
library, which will be used to make HTTP requests:
pip install requests
Writing Python Code to Retrieve Shopify Customers
Create a new Python file named get_shopify_customers.py
and add the following code:
import requests
# Set your Shopify store name and API version
store_name = "your-development-store"
api_version = "2024-07"
# Set the API endpoint and headers
endpoint = f"https://{store_name}.myshopify.com/admin/api/{api_version}/customers.json"
headers = {
"Content-Type": "application/json",
"X-Shopify-Access-Token": "Your_Access_Token"
}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
customers = response.json()["customers"]
for customer in customers:
print(f"Customer ID: {customer['id']}, Email: {customer['email']}")
else:
print(f"Failed to retrieve customers: {response.status_code} - {response.text}")
Replace Your_Access_Token
with the access token obtained during the OAuth setup. This script sets up the API endpoint and headers, makes a GET request to retrieve customers, and prints their IDs and emails if successful.
Running the Python Script and Verifying Results
Execute the script using the following command:
python get_shopify_customers.py
If successful, you should see a list of customer IDs and emails printed in the terminal. Verify the results by checking the customer data in your Shopify development store.
Handling Errors and Understanding Shopify API Response Codes
When making API calls, it's crucial to handle potential errors. Shopify's API may return various status codes, such as:
- 401 Unauthorized: Incorrect authentication credentials.
- 403 Forbidden: Incorrect access scopes.
- 404 Not Found: Resource not found.
- 429 Too Many Requests: Rate limit exceeded.
For more details on error codes, refer to the Shopify API documentation.
Managing Shopify API Rate Limits
Shopify's API has a rate limit of 40 requests per app per store per minute. If you exceed this limit, you'll receive a 429 error. To manage rate limits effectively, consider implementing retry logic with exponential backoff.
For more information on rate limits, visit the Shopify API rate limits documentation.
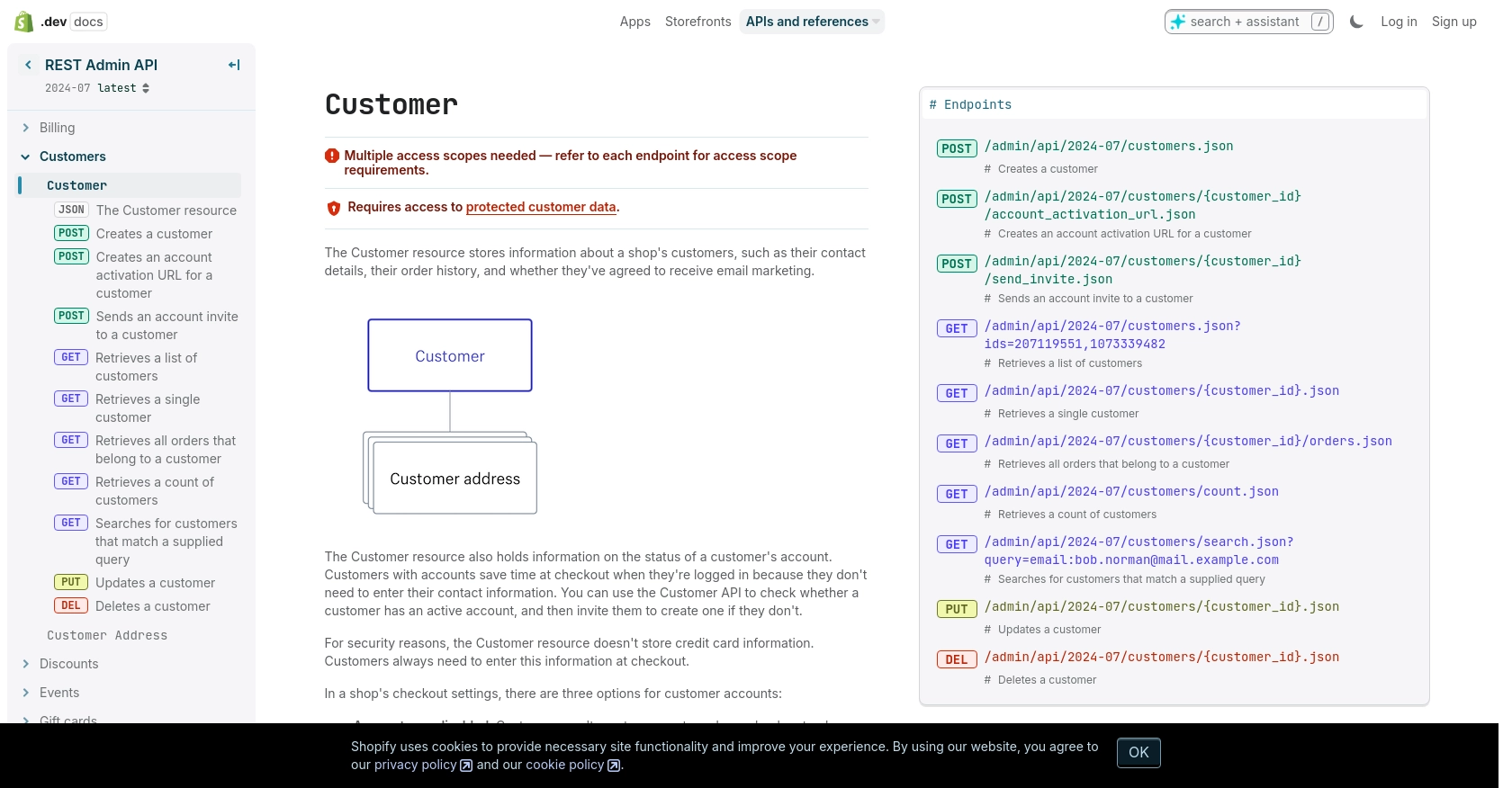
Conclusion and Best Practices for Shopify API Integration
Integrating with the Shopify API using Python provides a powerful way to access and manage customer data, enabling businesses to enhance their e-commerce operations. By following the steps outlined in this guide, you can efficiently retrieve customer information and leverage it to improve marketing strategies and customer engagement.
Best Practices for Storing Shopify User Credentials
When handling user credentials, it's crucial to prioritize security. Store access tokens securely, using environment variables or secure vaults, and never hard-code them in your application. Regularly rotate credentials and monitor for unauthorized access.
Handling Shopify API Rate Limits Effectively
Shopify's API rate limits require careful management to avoid disruptions. Implement retry logic with exponential backoff to handle 429 errors gracefully. Monitor your application's API usage and optimize requests to stay within the allowed limits.
Transforming and Standardizing Shopify Customer Data
Standardizing customer data ensures consistency across your applications. Transform data fields as needed to match your internal data structures, and validate data integrity to prevent errors during processing.
Streamlining Integrations with Endgrate
For developers seeking to simplify integration processes, Endgrate offers a unified API solution. By using Endgrate, you can save time and resources, focusing on your core product while outsourcing complex integrations. Build once for each use case and enjoy an intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration capabilities by visiting Endgrate today.
Read More
Ready to get started?