Using the Recruitee API to Get Candidates in Python
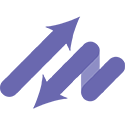
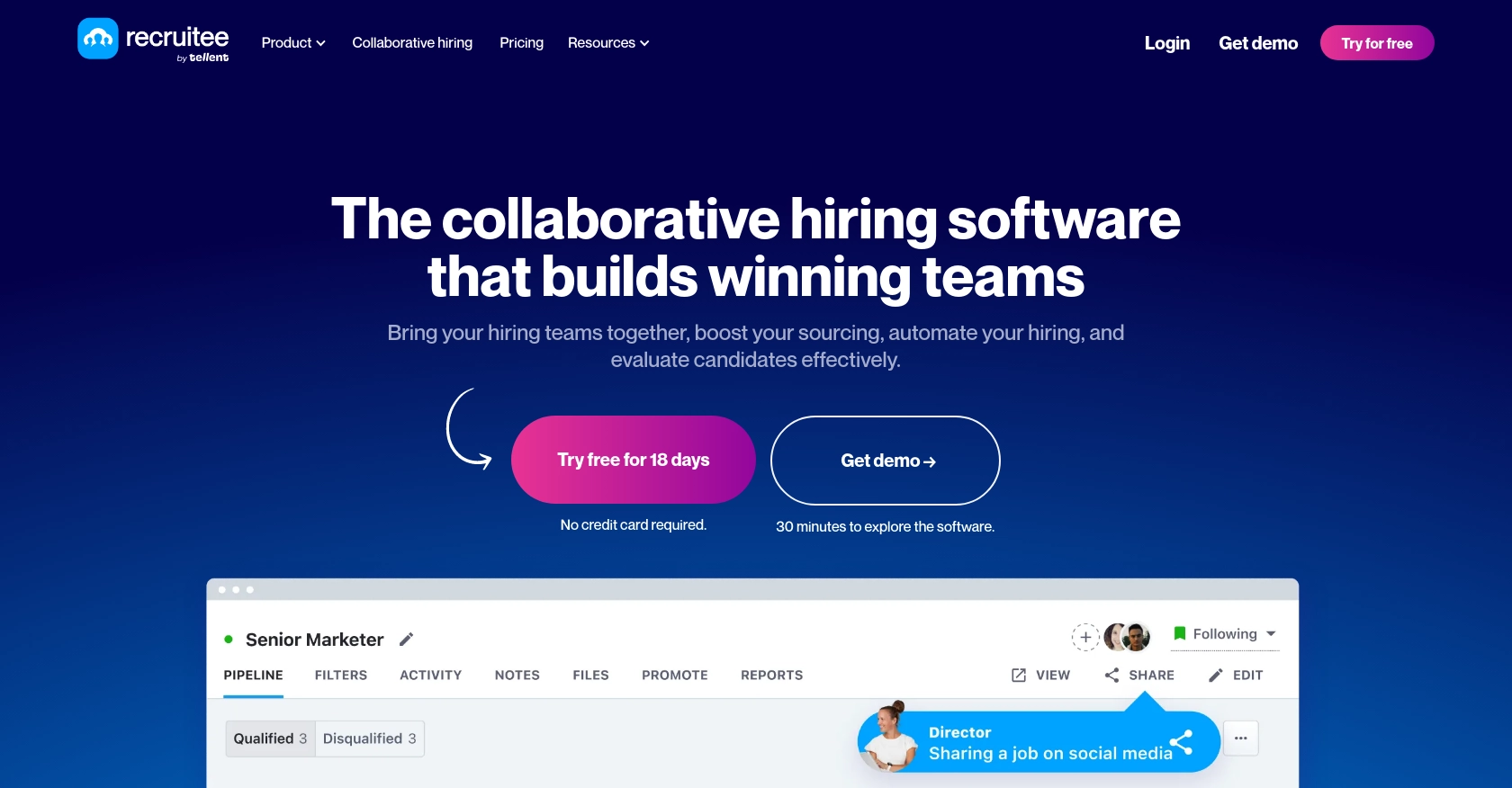
Introduction to Recruitee's ATS API
Recruitee is a powerful applicant tracking system (ATS) designed to enhance the recruitment process for businesses of all sizes. It offers a comprehensive suite of tools to manage job postings, track candidates, and streamline hiring workflows.
Developers may want to integrate with Recruitee's ATS API to automate and optimize recruitment tasks. For example, using the API, you can retrieve candidate information to analyze hiring trends or integrate with other HR systems for seamless data flow.
This article will guide you through using Python to interact with Recruitee's API, specifically focusing on retrieving candidate data. By the end of this tutorial, you'll be able to efficiently access and manage candidate information within your Recruitee account.
Setting Up Your Recruitee Test Account
Before you can start interacting with Recruitee's ATS API, you'll need to set up a test account and obtain the necessary API credentials. This will allow you to authenticate your requests and access candidate data securely.
Creating a Recruitee Account
If you don't already have a Recruitee account, you can sign up for a free trial on the Recruitee website. This will give you access to the platform's features and allow you to test the API integration.
- Visit the Recruitee website and click on the "Sign Up" button.
- Follow the on-screen instructions to create your account.
- Once your account is set up, log in to access the dashboard.
Generating a Personal API Token in Recruitee
Recruitee's ATS API uses API key-based authentication. You'll need to generate a personal API token to authenticate your requests. Follow these steps to create your token:
- Navigate to Settings in the top navigation bar.
- Select Apps and plugins from the menu.
- Click on Personal API tokens.
- Click + New token in the top-right corner to generate a new token.
- Copy the generated token and store it securely, as it will be used in your API requests.
Note: A personal API token does not expire unless revoked by the user. Ensure you keep it confidential to prevent unauthorized access.
For more detailed information, you can refer to the Recruitee API documentation.
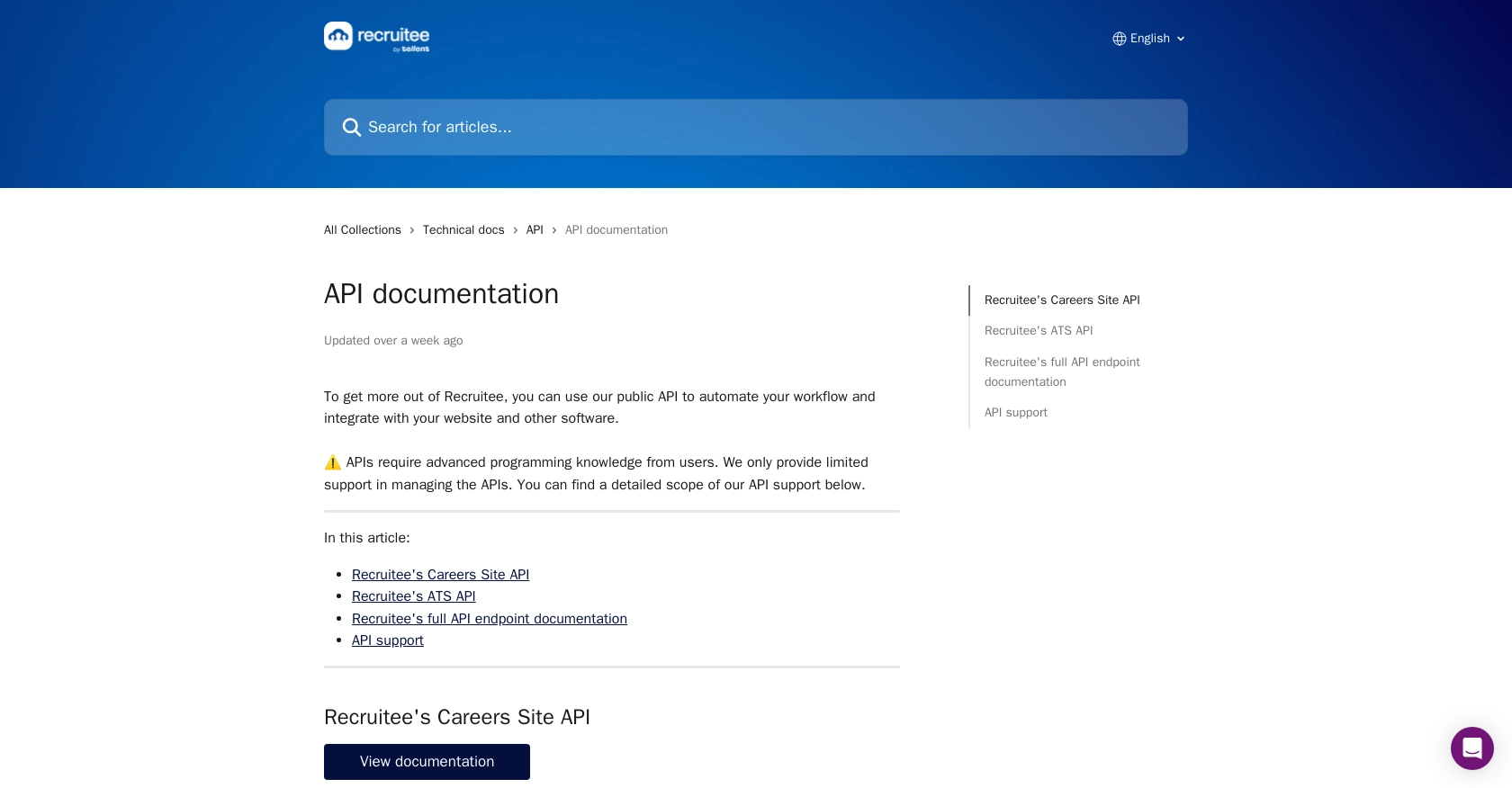
sbb-itb-96038d7
Making API Calls to Retrieve Candidates from Recruitee Using Python
To interact with Recruitee's ATS API and retrieve candidate data, you'll need to use Python. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling potential errors.
Setting Up Your Python Environment
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests.
- Install Python from the official Python website if you haven't already.
- Use the following command to install the
requests
library:
pip install requests
Writing Python Code to Access Recruitee's Candidates API
With your environment set up, you can now write the Python script to fetch candidate data from Recruitee. Create a file named get_candidates.py
and add the following code:
import requests
# Replace 'your_company_id' and 'your_api_token' with your actual company ID and API token
company_id = 'your_company_id'
api_token = 'your_api_token'
# Set the API endpoint
url = f"https://api.recruitee.com/c/{company_id}/candidates"
# Set the request headers
headers = {
"Authorization": f"Bearer {api_token}"
}
# Make a GET request to the API
response = requests.get(url, headers=headers)
# Check if the request was successful
if response.status_code == 200:
candidates = response.json()
for candidate in candidates:
print(candidate)
else:
print(f"Failed to retrieve candidates: {response.status_code} - {response.text}")
Replace your_company_id
and your_api_token
with your actual Recruitee company ID and API token.
Running the Python Script and Verifying Output
Run the script from your terminal or command line using the following command:
python get_candidates.py
If successful, the script will output a list of candidates from your Recruitee account. Verify the data by checking your Recruitee dashboard to ensure it matches the retrieved information.
Handling Errors and Troubleshooting
While making API calls, you might encounter errors. Here are some common issues and how to handle them:
- 401 Unauthorized: Ensure your API token is correct and has the necessary permissions.
- 404 Not Found: Verify the API endpoint URL and your company ID.
- 500 Internal Server Error: This might be a temporary issue with Recruitee's servers. Try again later.
For more detailed error handling, refer to the Recruitee API documentation.
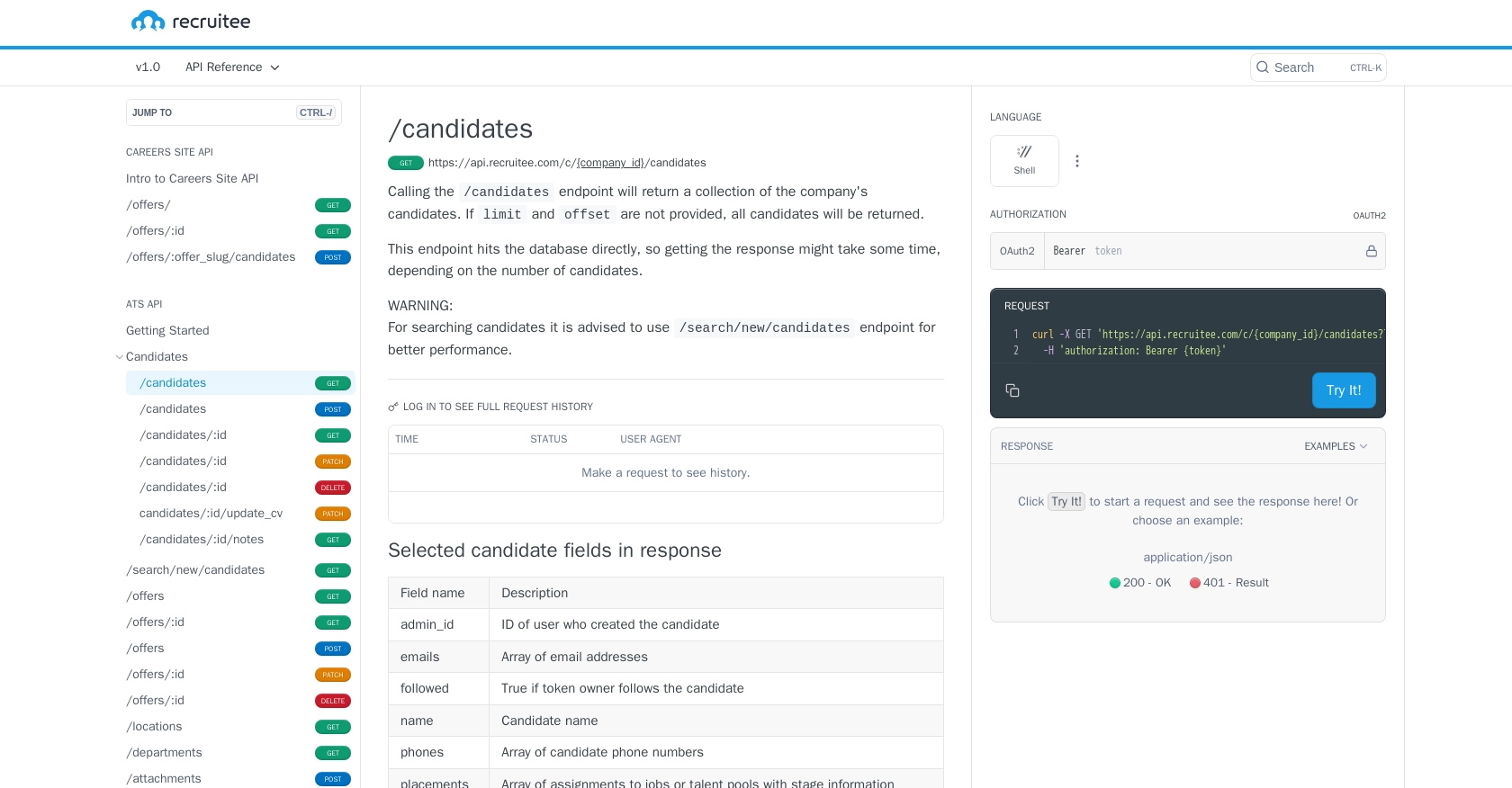
Conclusion and Best Practices for Using Recruitee's ATS API with Python
Integrating with Recruitee's ATS API using Python allows developers to efficiently manage and retrieve candidate data, streamlining recruitment processes and enhancing data-driven decision-making. By following the steps outlined in this article, you can seamlessly access candidate information and integrate it with other HR systems.
Best Practices for Secure and Efficient API Integration with Recruitee
- Secure API Credentials: Always store your API token securely and avoid hardcoding it in your scripts. Use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Be mindful of Recruitee's API rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Standardization: Ensure that the data retrieved from Recruitee is standardized and transformed as needed to integrate smoothly with other systems.
- Error Handling: Implement robust error handling to manage different HTTP status codes and unexpected API responses effectively.
Streamlining Integrations with Endgrate
While integrating with Recruitee's API can be straightforward, managing multiple integrations across different platforms can become complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process, allowing you to focus on your core product development.
With Endgrate, you can build once for each use case and leverage a single API endpoint to connect with various platforms, including Recruitee. This approach not only saves time and resources but also provides an intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration capabilities by visiting Endgrate's website and discover the benefits of outsourcing integrations to streamline your development workflow.
Read More
Ready to get started?