Using the Capsule API to Create or Update People (with Javascript examples)
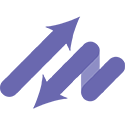
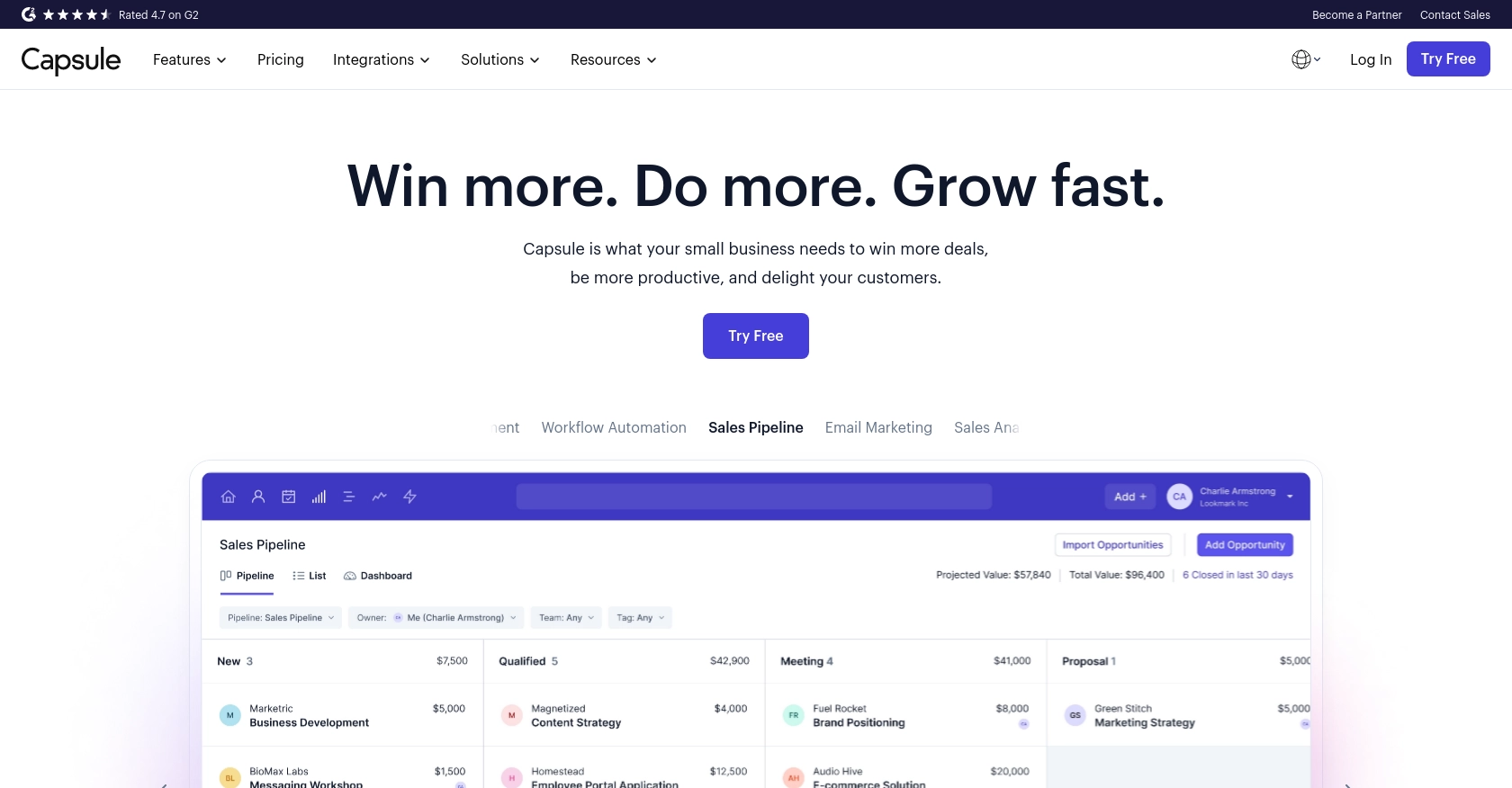
Introduction to Capsule CRM
Capsule CRM is a versatile customer relationship management platform designed to help businesses manage their contacts, sales opportunities, and customer interactions efficiently. Known for its user-friendly interface and robust features, Capsule CRM is a popular choice for businesses looking to streamline their CRM processes.
Integrating with Capsule's API allows developers to automate and enhance CRM functionalities, such as creating or updating contact information seamlessly. For example, a developer might use the Capsule API to automatically update customer details from an external data source, ensuring that the CRM database remains current and accurate.
Setting Up Your Capsule CRM Test Account
Before you begin integrating with Capsule's API, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting live data. Capsule offers a free trial that you can use to explore its features and test your integration.
Creating a Capsule CRM Account
To get started, visit the Capsule CRM sign-up page and create a free trial account. Follow the on-screen instructions to complete the registration process. Once your account is set up, you'll have access to the Capsule dashboard where you can manage your CRM data.
Generating an OAuth 2.0 Bearer Token
Capsule uses OAuth 2.0 for authentication, which requires you to generate a bearer token. Follow these steps to create an app and obtain the necessary credentials:
- Log in to your Capsule account and navigate to My Preferences > API Authentication Tokens.
- Click on Create New Token and provide a name for your token.
- Copy the generated token and store it securely, as you'll need it to authenticate your API requests.
For more complex integrations, you may want to use the OAuth 2.0 flow to obtain tokens dynamically. This involves registering your application and using the client ID and client secret to request tokens. For detailed instructions, refer to the Capsule API Authentication documentation.
Registering Your Application for OAuth 2.0
If you choose to use the OAuth 2.0 flow, you'll need to register your application:
- Go to the Developer Portal on the Capsule website.
- Register your application by providing the necessary details, such as the application name and redirect URI.
- Once registered, you'll receive a client ID and client secret. Keep these credentials secure.
Use these credentials to implement the OAuth 2.0 authorization code flow, allowing users to authorize your application to access their Capsule data.
Testing Your Capsule API Integration
With your test account and authentication tokens ready, you can now begin testing your API integration. Use the bearer token in the Authorization header of your API requests to interact with Capsule's resources. For example, you can create or update people in your Capsule account using the API endpoints.
Refer to the Capsule API documentation for detailed information on making API calls and handling responses.
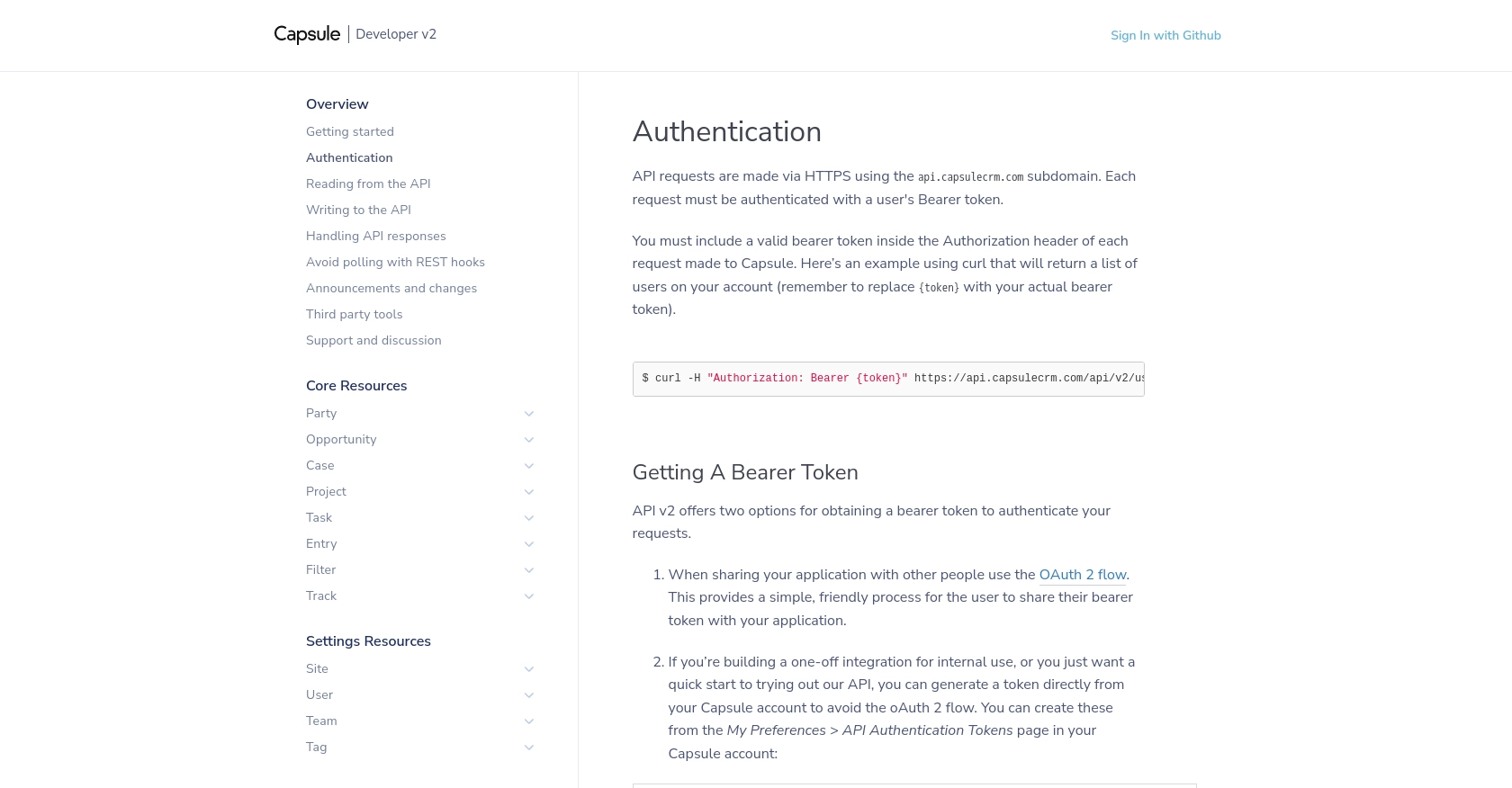
sbb-itb-96038d7
Making API Calls to Create or Update People in Capsule CRM Using JavaScript
Setting Up Your JavaScript Environment for Capsule API Integration
To interact with the Capsule API using JavaScript, ensure you have a modern JavaScript environment set up. This includes Node.js and npm for managing packages. You will also need the axios
library to handle HTTP requests.
npm install axios
Creating a New Person in Capsule CRM
To create a new person in Capsule CRM, you will use the POST
method to send a request to the Capsule API. Below is a sample code snippet demonstrating how to create a new person:
const axios = require('axios');
const createPerson = async () => {
const url = 'https://api.capsulecrm.com/api/v2/parties';
const token = 'Your_Bearer_Token'; // Replace with your actual token
const personData = {
party: {
type: 'person',
firstName: 'John',
lastName: 'Doe',
emailAddresses: [
{ type: 'Work', address: 'john.doe@example.com' }
],
phoneNumbers: [
{ type: 'Work', number: '123-456-7890' }
]
}
};
try {
const response = await axios.post(url, personData, {
headers: {
'Authorization': `Bearer ${token}`,
'Content-Type': 'application/json'
}
});
console.log('Person created:', response.data);
} catch (error) {
console.error('Error creating person:', error.response.data);
}
};
createPerson();
Replace Your_Bearer_Token
with the token you generated earlier. This code sends a request to create a new person with specified details. If successful, the response will include the newly created person's data.
Updating an Existing Person in Capsule CRM
To update an existing person, use the PUT
method. You need the person's unique ID to update their information. Here's how you can update a person's details:
const updatePerson = async (personId) => {
const url = `https://api.capsulecrm.com/api/v2/parties/${personId}`;
const token = 'Your_Bearer_Token'; // Replace with your actual token
const updatedData = {
party: {
about: 'Updated information about John Doe',
emailAddresses: [
{ id: 12137, type: 'Home', address: 'john.doe@home.com' }
]
}
};
try {
const response = await axios.put(url, updatedData, {
headers: {
'Authorization': `Bearer ${token}`,
'Content-Type': 'application/json'
}
});
console.log('Person updated:', response.data);
} catch (error) {
console.error('Error updating person:', error.response.data);
}
};
updatePerson(11587); // Replace with the actual person ID
Ensure you replace 11587
with the actual ID of the person you wish to update. This code updates the specified person's details and logs the updated data if successful.
Handling API Responses and Errors in Capsule CRM
When making API calls, it's crucial to handle responses and errors appropriately. Capsule API returns various HTTP status codes to indicate success or failure. For instance, a 201 Created
status indicates a successful creation, while a 400 Bad Request
indicates an error in the request format.
Refer to the Capsule API Handling API Responses documentation for more details on interpreting these responses and managing errors effectively.
Verifying API Call Success in Capsule CRM
After making API calls, verify their success by checking the Capsule CRM dashboard or using API endpoints to retrieve and confirm the updated data. This ensures that your integration works as expected and that data consistency is maintained.
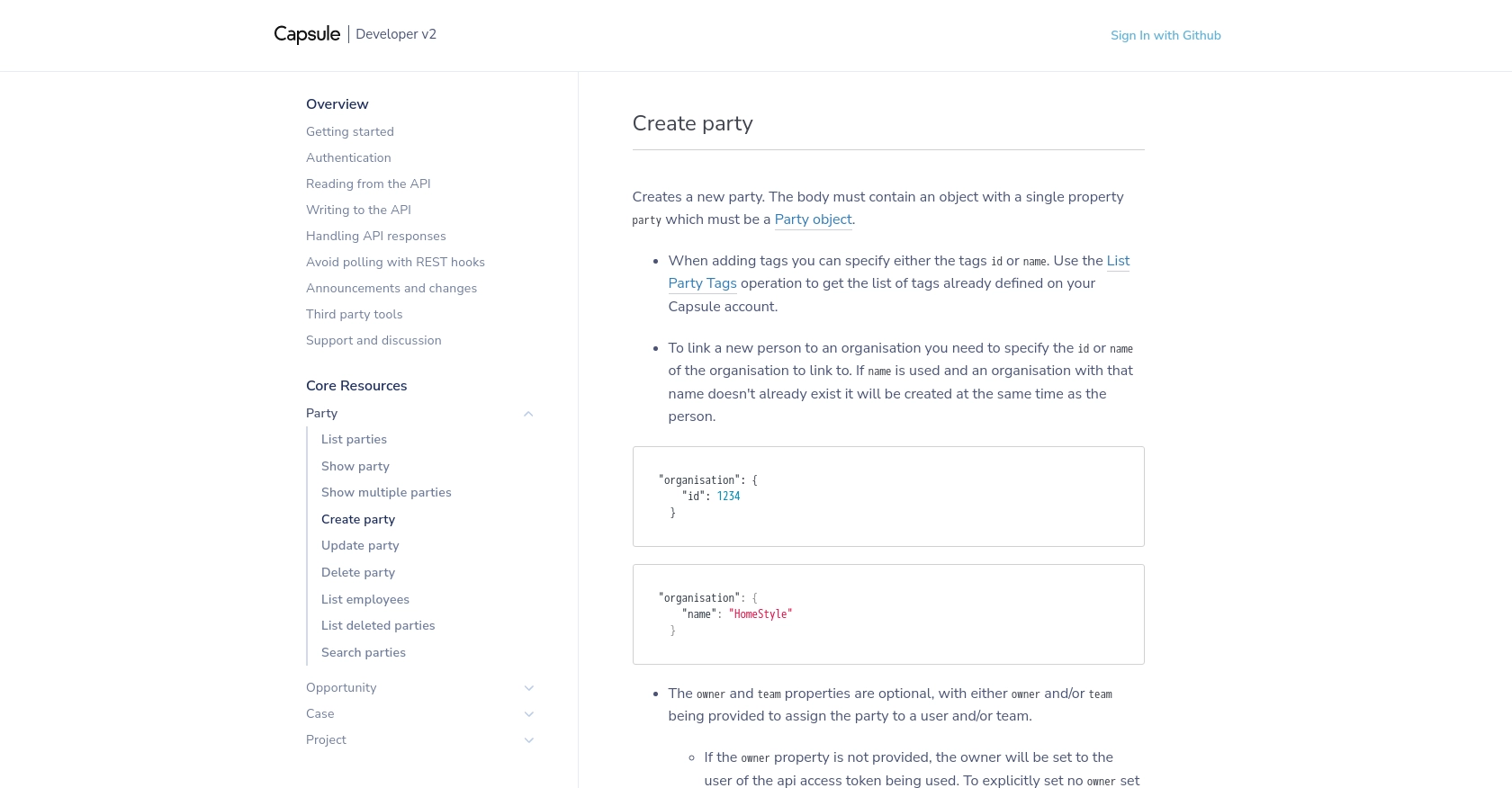
Best Practices for Capsule API Integration
When integrating with the Capsule API, it's essential to follow best practices to ensure a secure and efficient implementation. Here are some key recommendations:
- Secure Storage of Credentials: Always store your OAuth 2.0 credentials securely. Avoid hardcoding them in your source code. Use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Capsule API allows up to 4,000 requests per hour per user. Monitor the
X-RateLimit-Remaining
header to avoid exceeding this limit. Implement exponential backoff or delay mechanisms to handle rate limit errors gracefully. - Data Standardization: Ensure that data fields are standardized before sending them to Capsule. This helps maintain consistency and prevents validation errors.
- Error Handling: Implement robust error handling to manage different HTTP status codes. Use the error messages provided in the response to debug and resolve issues efficiently.
Leveraging Endgrate for Seamless Capsule Integrations
Integrating multiple platforms can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various services, including Capsule. By using Endgrate, you can:
- Save time and resources by outsourcing integrations, allowing you to focus on your core product.
- Build once for each use case instead of multiple times for different integrations.
- Offer an intuitive integration experience for your customers, enhancing user satisfaction.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate's website.
Conclusion: Enhancing CRM Capabilities with Capsule API
By integrating with the Capsule API, developers can automate and enhance CRM functionalities, ensuring that customer data is always up-to-date and accessible. Following best practices and leveraging tools like Endgrate can significantly improve the efficiency and reliability of your integrations. Start exploring the possibilities with Capsule API today and take your CRM capabilities to the next level.
Read More
- https://endgrate.com/provider/capsulecrm
- https://developer.capsulecrm.com/v2/overview/authentication
- https://developer.capsulecrm.com/v2/overview/handling-api-responses
- https://developer.capsulecrm.com/v2/overview/avoid-polling-with-rest-hooks
- https://developer.capsulecrm.com/v2/operations/Party#createParty
Ready to get started?