Using the Zoho Books API to Get Customers in PHP
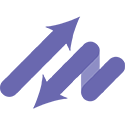
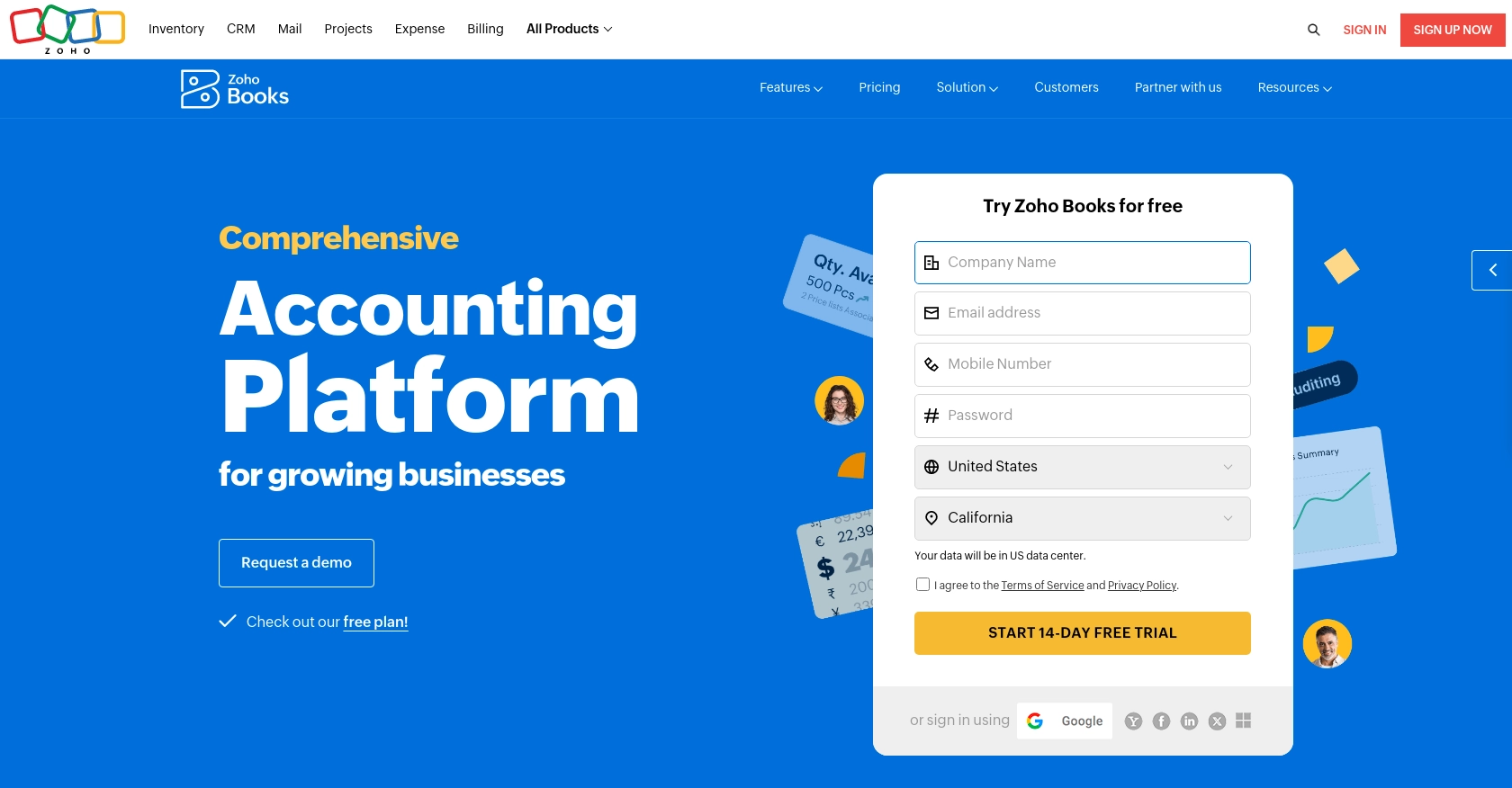
Introduction to Zoho Books API Integration
Zoho Books is a comprehensive cloud-based accounting software designed to streamline financial management for businesses of all sizes. It offers a wide range of features including invoicing, expense tracking, and financial reporting, making it a popular choice for businesses looking to manage their finances efficiently.
Integrating with the Zoho Books API allows developers to automate and enhance their financial processes. For example, you can use the API to retrieve customer data, enabling seamless synchronization with your internal systems. This can be particularly useful for generating reports, managing customer relationships, and ensuring data consistency across platforms.
In this article, we will explore how to use PHP to interact with the Zoho Books API to retrieve customer information. This integration can help developers automate customer data retrieval, making it easier to maintain up-to-date records and improve business operations.
Setting Up Your Zoho Books Test/Sandbox Account
Before you can begin integrating with the Zoho Books API, you'll need to set up a test or sandbox account. This will allow you to safely experiment with the API without affecting your live data.
Creating a Zoho Books Account
If you don't already have a Zoho Books account, you can sign up for a free trial on the Zoho Books website. Follow the instructions to create your account. Once your account is set up, you'll be able to access the Zoho Books dashboard.
Registering Your Application for OAuth Authentication
Zoho Books uses OAuth 2.0 for authentication. To interact with the API, you need to register your application and obtain the necessary credentials.
- Go to the Zoho Developer Console and click on Add Client ID.
- Fill in the required details to register your application. This includes providing a name, description, and redirect URI.
- After registration, you'll receive a Client ID and Client Secret. Keep these credentials secure as they are essential for authenticating your API requests.
Generating OAuth Tokens
With your Client ID and Client Secret, you can generate OAuth tokens to authenticate API requests.
- Redirect users to the following authorization URL, replacing placeholders with your details:
- Upon user consent, Zoho will redirect to your specified URI with a code parameter. Use this code to request an access token:
- The response will include an access_token and a refresh_token. The access token is used for API calls, while the refresh token can be used to obtain new access tokens.
https://accounts.zoho.com/oauth/v2/auth?scope=ZohoBooks.contacts.READ&client_id=YOUR_CLIENT_ID&response_type=code&redirect_uri=YOUR_REDIRECT_URI&access_type=offline
https://accounts.zoho.com/oauth/v2/token?code=YOUR_CODE&client_id=YOUR_CLIENT_ID&client_secret=YOUR_CLIENT_SECRET&redirect_uri=YOUR_REDIRECT_URI&grant_type=authorization_code
Testing Your Zoho Books API Integration
With your OAuth tokens, you can now make API calls to Zoho Books. Use the sandbox environment to test your integration and ensure everything works as expected before deploying to production.
For more details on Zoho Books API authentication, refer to the Zoho Books OAuth documentation.
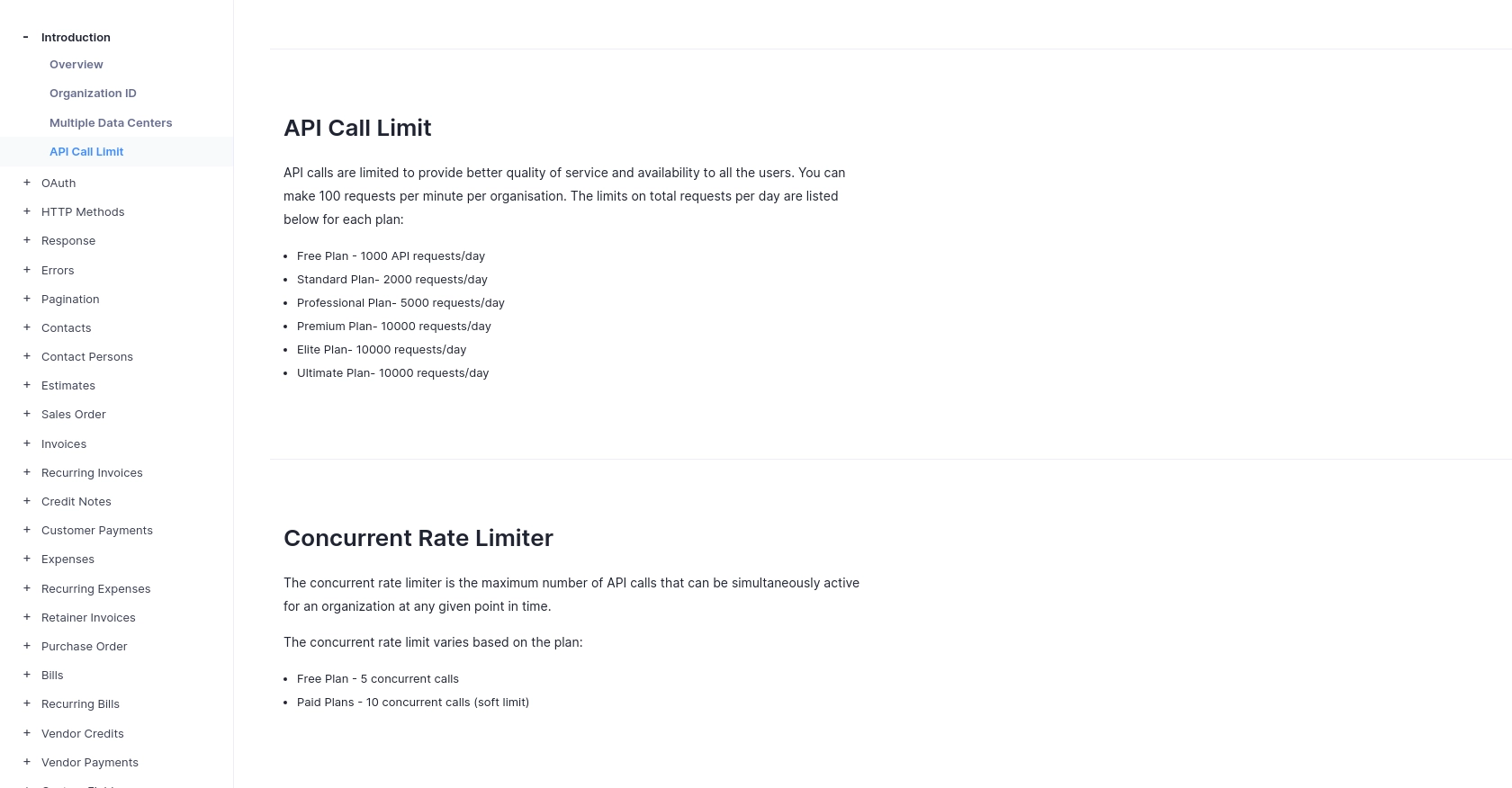
sbb-itb-96038d7
Making API Calls to Retrieve Customers from Zoho Books Using PHP
To interact with the Zoho Books API and retrieve customer data, you'll need to make HTTP requests using PHP. This section will guide you through the necessary steps, including setting up your PHP environment, writing the code to make API calls, and handling responses.
Setting Up Your PHP Environment for Zoho Books API Integration
Before making API calls, ensure your PHP environment is configured correctly. You'll need PHP 7.4 or later and the cURL
extension enabled. You can verify your PHP version and extensions by running the following command:
php -v
To enable the cURL
extension, locate your php.ini
file and ensure the following line is uncommented:
extension=curl
Installing Required PHP Dependencies
For making HTTP requests, you can use the GuzzleHTTP
library, which simplifies the process. Install it via Composer:
composer require guzzlehttp/guzzle
Writing PHP Code to Retrieve Customers from Zoho Books
With your environment set up, you can now write the PHP code to make API calls. Create a file named get_customers.php
and add the following code:
<?php
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$accessToken = 'YOUR_ACCESS_TOKEN';
$organizationId = 'YOUR_ORGANIZATION_ID';
$response = $client->request('GET', 'https://www.zohoapis.com/books/v3/contacts', [
'headers' => [
'Authorization' => 'Zoho-oauthtoken ' . $accessToken,
],
'query' => [
'organization_id' => $organizationId,
'contact_type' => 'customer'
]
]);
$data = json_decode($response->getBody(), true);
foreach ($data['contacts'] as $customer) {
echo 'Customer Name: ' . $customer['contact_name'] . "\n";
echo 'Email: ' . $customer['email'] . "\n\n";
}
?>
Replace YOUR_ACCESS_TOKEN
and YOUR_ORGANIZATION_ID
with your actual access token and organization ID. This script sends a GET request to the Zoho Books API to retrieve customers, filtering by contact_type
as 'customer'.
Handling API Responses and Errors
After executing the script, you should see a list of customer names and emails. If the request fails, handle errors by checking the response status code:
if ($response->getStatusCode() !== 200) {
echo 'Error: ' . $response->getReasonPhrase();
}
Refer to the Zoho Books API error documentation for more information on handling specific error codes.
Verifying Successful API Calls in Zoho Books
To verify that your API calls are successful, log in to your Zoho Books account and check the customer list. The data retrieved by your script should match the records in your Zoho Books account.
For more details on API call limits, refer to the Zoho Books API call limit documentation.
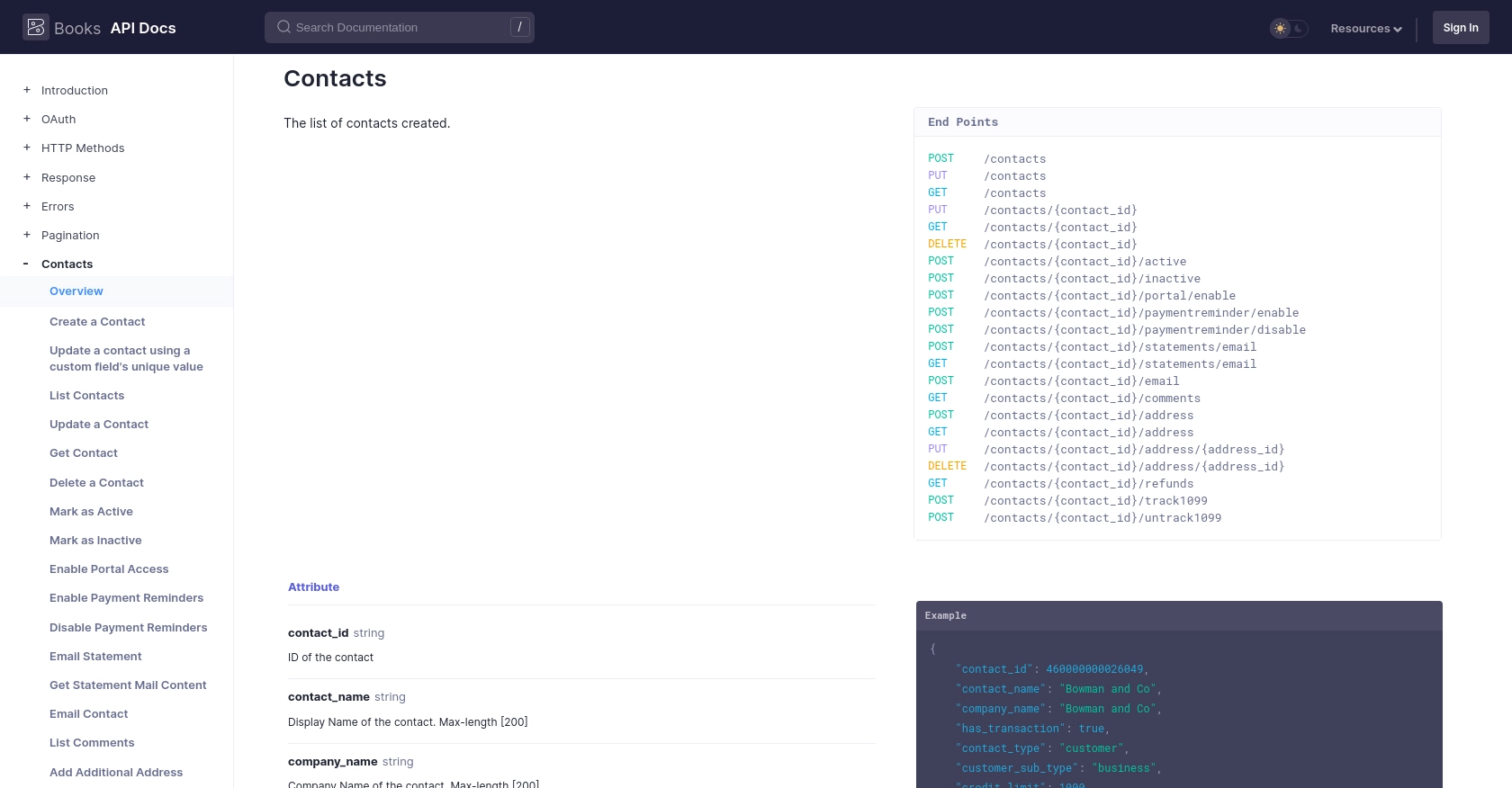
Conclusion and Best Practices for Zoho Books API Integration Using PHP
Integrating with the Zoho Books API using PHP provides a powerful way to automate and enhance your financial management processes. By retrieving customer data programmatically, you can ensure that your internal systems are always up-to-date, improving efficiency and accuracy in your business operations.
Best Practices for Secure and Efficient Zoho Books API Integration
- Securely Store Credentials: Always store your OAuth credentials, such as the Client ID, Client Secret, and tokens, securely. Avoid hardcoding them in your scripts and consider using environment variables or secure vaults.
- Handle Rate Limiting: Zoho Books API has rate limits, with a maximum of 100 requests per minute per organization. Implement logic to handle rate limiting gracefully, such as retrying requests after a delay. For more details, refer to the API call limit documentation.
- Manage Error Responses: Implement robust error handling by checking HTTP status codes and handling specific error messages. This ensures that your application can recover gracefully from API errors. Refer to the error documentation for guidance.
- Optimize Data Handling: When retrieving large datasets, consider using pagination to manage data efficiently. This helps in reducing memory usage and improving performance.
Leverage Endgrate for Simplified Integration Management
While integrating with Zoho Books API can be highly beneficial, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API endpoint that connects to various platforms, including Zoho Books, simplifying the integration process. By using Endgrate, you can focus on your core product while outsourcing the complexities of integration management.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate's website and discover how it can save you time and resources.
Read More
- https://endgrate.com/provider/zohobooks
- https://www.zoho.com/books/api/v3/introduction/#api-call-limit
- https://www.zoho.com/books/api/v3/oauth/#overview
- https://www.zoho.com/books/api/v3/errors/#overview
- https://www.zoho.com/books/api/v3/pagination/#overview
- https://www.zoho.com/books/api/v3/contacts/#overview
Ready to get started?