Using the Sap Business One API to Get Items (with Javascript examples)
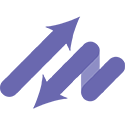
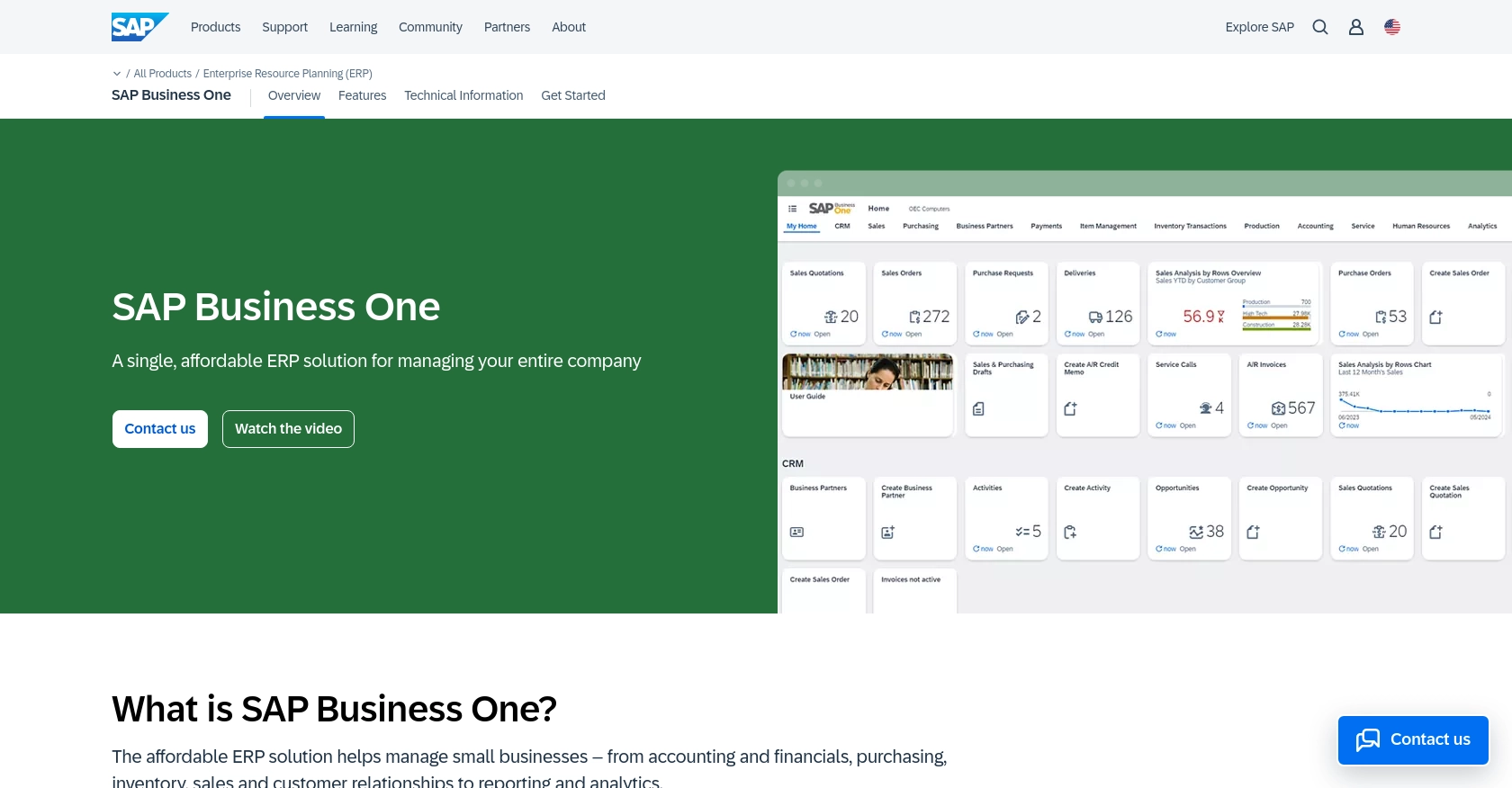
Introduction to SAP Business One API
SAP Business One is a comprehensive enterprise resource planning (ERP) solution designed for small to medium-sized businesses. It offers a wide range of functionalities, including financial management, sales, customer relationship management, and inventory control, all within a single platform.
Developers often seek to integrate with the SAP Business One API to streamline business processes and enhance operational efficiency. By accessing the API, developers can automate tasks such as retrieving item data, which can be used for inventory management, reporting, or syncing with other systems. For example, a developer might use the SAP Business One API to fetch item details and update an e-commerce platform's inventory in real-time.
Setting Up a Test/Sandbox Account for SAP Business One API Integration
Before you can start interacting with the SAP Business One API, it's essential to set up a test or sandbox account. This environment allows developers to experiment and test API calls without affecting live data, ensuring a smooth integration process.
Creating a SAP Business One Sandbox Account
To begin, you need access to a SAP Business One environment. If your organization already uses SAP Business One, you can request access to a sandbox instance from your system administrator. If not, consider reaching out to SAP or a certified partner to explore trial or demo options.
Configuring OAuth-Based Authentication for SAP Business One API
SAP Business One uses a custom authentication method to secure API interactions. Follow these steps to configure your authentication:
- Log in to your SAP Business One sandbox account.
- Navigate to the Service Layer settings, which can typically be found under the administration or integration settings.
- Create a new application by providing necessary details such as application name and description.
- Generate the required credentials, including the client ID and client secret. These will be used to authenticate API requests.
- Ensure that the application has the appropriate permissions to access item data. This may involve setting scopes or roles within the SAP Business One interface.
Obtaining API Access Credentials
Once your application is set up, you will need to obtain the API access credentials:
- Client ID: A unique identifier for your application.
- Client Secret: A secret key used to authenticate your application.
- Access Token: A token that grants access to the API. This is typically obtained by exchanging the client ID and client secret through an authentication endpoint.
Keep these credentials secure and never expose them in client-side code or public repositories.
For more detailed information on setting up and authenticating with the SAP Business One API, refer to the official documentation: SAP Business One Service Layer Documentation.
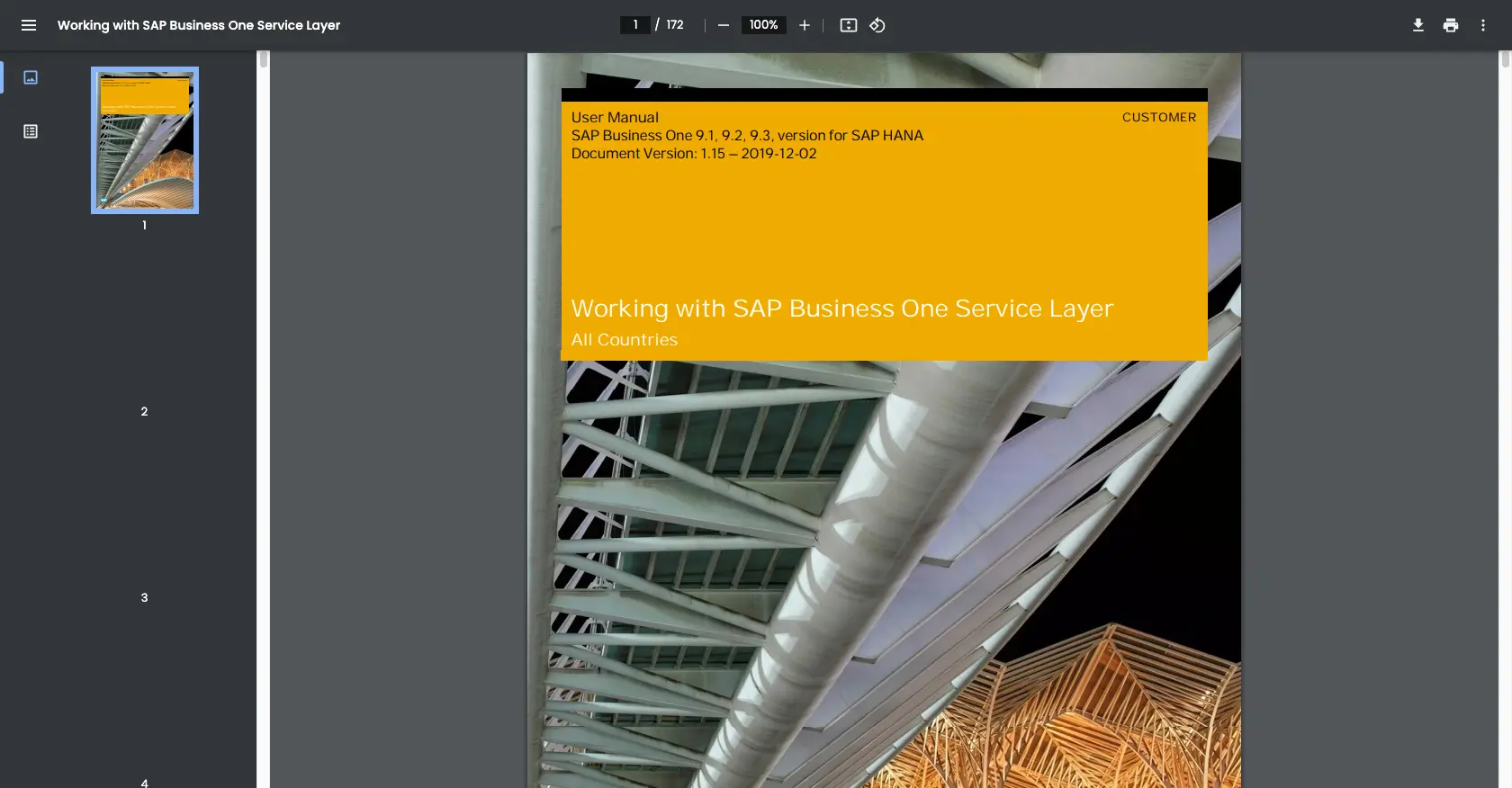
sbb-itb-96038d7
How to Make API Calls to Retrieve Items Using SAP Business One API with JavaScript
To interact with the SAP Business One API and retrieve item data, you'll need to use JavaScript to make HTTP requests. This section will guide you through the process of setting up your environment, writing the code, and handling the responses effectively.
Setting Up Your JavaScript Environment for SAP Business One API
Before making API calls, ensure you have the following prerequisites:
- A modern JavaScript runtime environment, such as Node.js.
- Installation of the
axios
library for making HTTP requests. You can install it using npm:
npm install axios
Writing JavaScript Code to Fetch Items from SAP Business One API
Once your environment is ready, you can write the JavaScript code to interact with the SAP Business One API. Below is an example of how to retrieve items:
const axios = require('axios');
// Define the API endpoint and headers
const endpoint = 'https://your-sap-business-one-instance.com/b1s/v1/Items';
const headers = {
'Content-Type': 'application/json',
'Authorization': 'Bearer Your_Access_Token'
};
// Function to get items
async function getItems() {
try {
const response = await axios.get(endpoint, { headers });
const items = response.data.value;
console.log('Retrieved Items:', items);
} catch (error) {
console.error('Error fetching items:', error.response ? error.response.data : error.message);
}
}
// Call the function to fetch items
getItems();
Replace Your_Access_Token
with the access token obtained during authentication.
Verifying API Call Success and Handling Errors
After executing the code, you should see the list of items retrieved from your SAP Business One sandbox environment. Verify the success of the API call by checking the console output for the expected item data.
If the request fails, the error handling in the code will log the error message. Common error codes include:
- 401 Unauthorized: Check your access token and ensure it is valid.
- 404 Not Found: Verify the API endpoint URL.
- 500 Internal Server Error: This may indicate an issue with the SAP Business One server.
For more detailed error information, refer to the official SAP Business One API documentation: SAP Business One Service Layer API Reference.
Conclusion and Best Practices for SAP Business One API Integration
Integrating with the SAP Business One API using JavaScript can significantly enhance your business operations by automating data retrieval and synchronization processes. By following the steps outlined in this guide, you can efficiently access item data and ensure seamless integration with your existing systems.
Best Practices for Secure and Efficient SAP Business One API Usage
- Secure Credential Storage: Always store your API credentials, such as client ID, client secret, and access tokens, securely. Avoid hardcoding them in your source code and consider using environment variables or secure vaults.
- Handle Rate Limiting: Be mindful of any rate limits imposed by the SAP Business One API. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Transformation and Standardization: Ensure that the data retrieved from the API is transformed and standardized according to your application's requirements. This will help maintain data consistency across different systems.
- Error Handling: Implement robust error handling to manage API call failures. Log errors for further analysis and provide meaningful feedback to users when issues occur.
Streamlining Integrations with Endgrate
While integrating with SAP Business One API can be rewarding, it may also be complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process by providing a single endpoint to connect with multiple platforms, including SAP Business One.
By leveraging Endgrate, you can save time and resources, allowing your team to focus on core product development. With its intuitive integration experience, you can build once for each use case and easily extend support to other platforms.
Explore how Endgrate can transform your integration strategy by visiting Endgrate today.
Read More
Ready to get started?