How to Get Users with the Zoho CRM API in Javascript
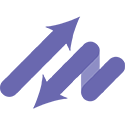
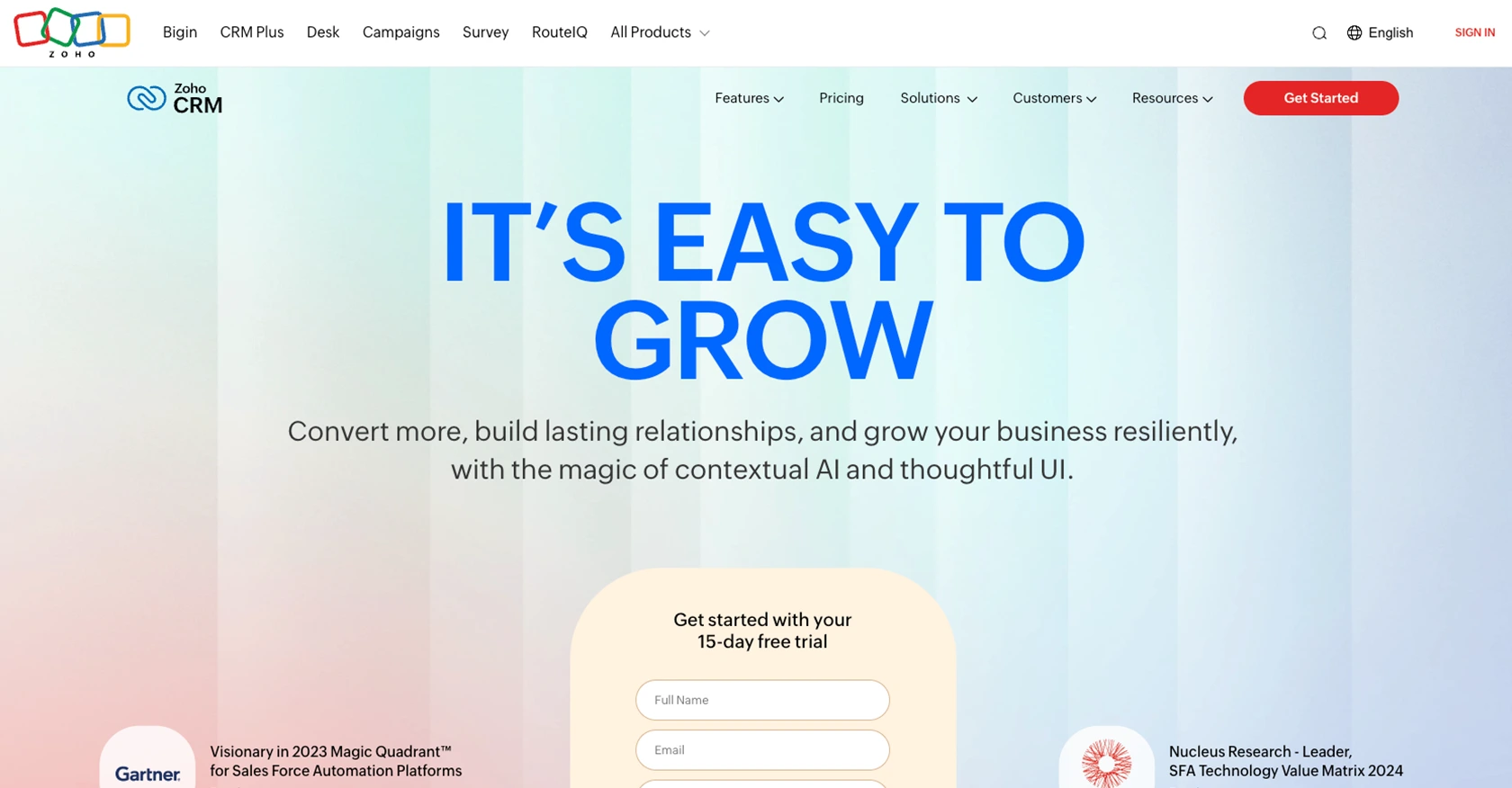
Introduction to Zoho CRM
Zoho CRM is a comprehensive customer relationship management platform that empowers businesses to manage their sales, marketing, and support in a unified system. Known for its flexibility and robust features, Zoho CRM is a popular choice for organizations aiming to enhance their customer engagement and streamline operations.
Developers often seek to integrate with Zoho CRM's API to automate and enhance their workflows. By accessing user data through the Zoho CRM API, developers can create custom solutions that improve team collaboration and efficiency. For example, retrieving user information can help in synchronizing CRM data with other business applications, ensuring that all team members have access to up-to-date information.
Setting Up Your Zoho CRM Test/Sandbox Account
Before you can start interacting with the Zoho CRM API, you need to set up a test or sandbox account. This allows you to safely experiment with API calls without affecting live data. Zoho CRM offers a developer sandbox environment that mirrors your production account, providing a risk-free space for testing integrations.
Creating a Zoho CRM Developer Account
If you don't already have a Zoho CRM account, you can sign up for a free developer account:
- Visit the Zoho Developer Console.
- Click on "Sign Up For Free" and follow the registration process.
- Once registered, log in to access the developer console.
Registering Your Application for OAuth Authentication
Zoho CRM uses OAuth 2.0 for authentication, which requires you to register your application to obtain the necessary credentials:
- In the Zoho Developer Console, select "Register Client".
- Choose "JavaScript" as the client type since your application will run in a browser.
- Enter the following details:
- Client Name: A name for your application.
- Homepage URL: The URL of your application.
- Authorized Redirect URIs: A valid URL where Zoho will redirect with the authorization code.
- Click "CREATE" to register your application.
Upon successful registration, you will receive a Client ID and Client Secret. Keep these credentials secure as they are required for API authentication.
Generating OAuth Tokens
To interact with the Zoho CRM API, you need to generate access and refresh tokens:
- Direct users to the Zoho authorization URL with the required scopes, such as
scope=ZohoCRM.users.ALL
. - Upon user consent, Zoho will redirect to your specified URI with an authorization code.
- Exchange this code for access and refresh tokens by making a POST request to Zoho's token endpoint.
Refer to the detailed steps in the Zoho CRM OAuth documentation for more information.
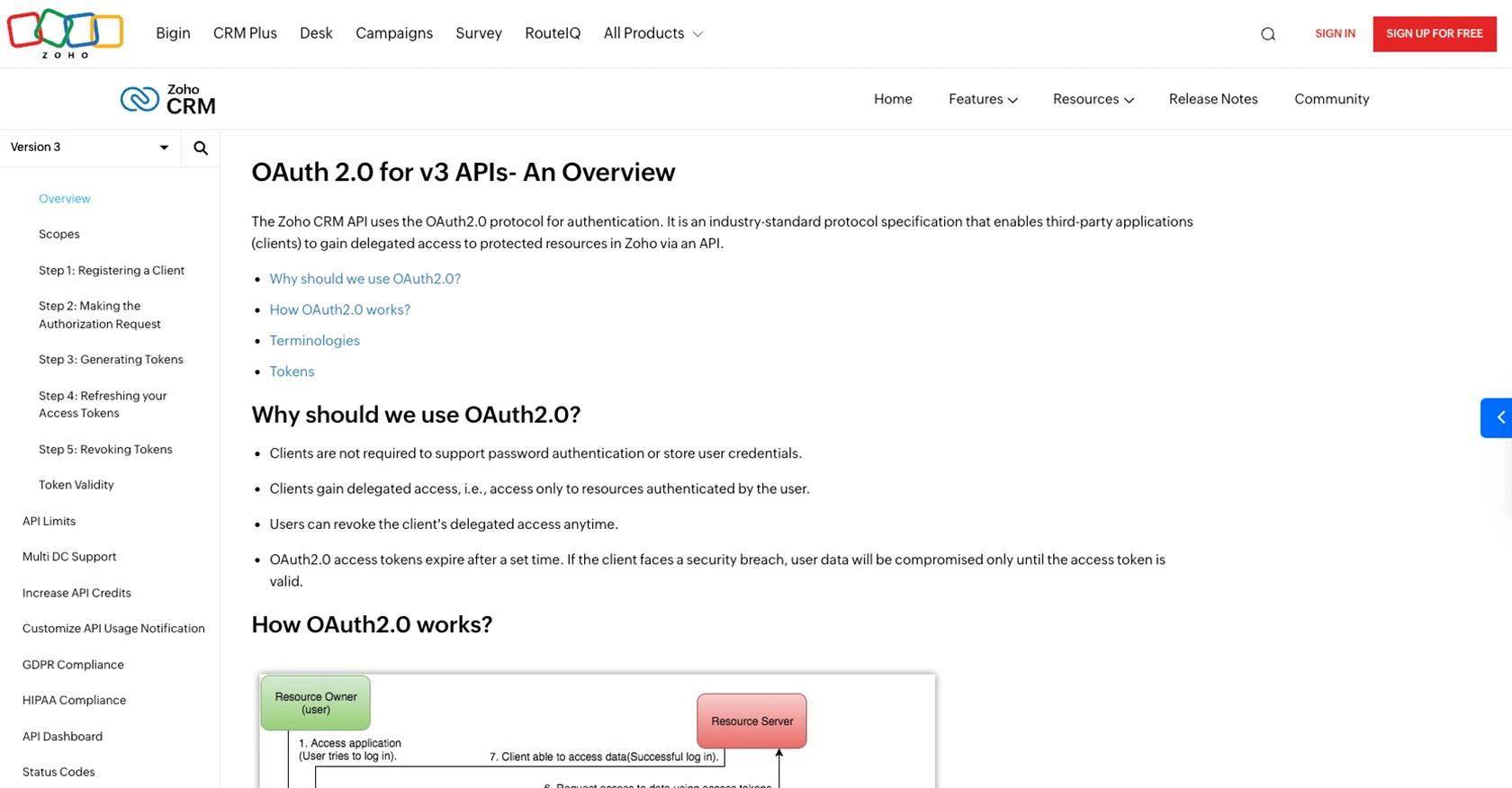
sbb-itb-96038d7
Making API Calls to Retrieve Users with Zoho CRM API in JavaScript
To interact with the Zoho CRM API and retrieve user data, you'll need to use JavaScript to make HTTP requests. This section will guide you through the process of setting up your environment, writing the code, and handling the responses effectively.
Setting Up Your JavaScript Environment for Zoho CRM API
Before you start coding, ensure you have the following prerequisites:
- Node.js installed on your machine.
- A text editor or IDE for writing JavaScript code.
- Access to the Zoho CRM API with valid OAuth tokens.
Installing Required JavaScript Libraries
To make HTTP requests in JavaScript, you can use the popular axios
library. Install it using npm:
npm install axios
Writing JavaScript Code to Call Zoho CRM API
Create a new JavaScript file, for example, getZohoCRMUsers.js
, and add the following code:
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://www.zohoapis.com/crm/v3/users';
const headers = {
'Authorization': 'Zoho-oauthtoken YOUR_ACCESS_TOKEN'
};
// Function to get users from Zoho CRM
async function getUsers() {
try {
const response = await axios.get(endpoint, { headers });
const users = response.data.users;
// Log user information
users.forEach(user => {
console.log(`Name: ${user.full_name}, Email: ${user.email}`);
});
} catch (error) {
console.error('Error fetching users:', error.response ? error.response.data : error.message);
}
}
// Call the function
getUsers();
Replace YOUR_ACCESS_TOKEN
with the access token you obtained during the OAuth authentication process.
Running the JavaScript Code
Execute the script using Node.js:
node getZohoCRMUsers.js
You should see a list of users printed in the console, displaying their names and email addresses.
Handling API Responses and Errors
When making API calls, it's crucial to handle responses and potential errors effectively:
- Check the response status code to ensure the request was successful.
- Log any error messages to help with debugging.
- Refer to the Zoho CRM API status codes documentation for more details on handling specific error codes.
Verifying API Call Success in Zoho CRM Sandbox
After running your script, verify the retrieved data by checking the user list in your Zoho CRM sandbox account. Ensure the data matches the output from your script.
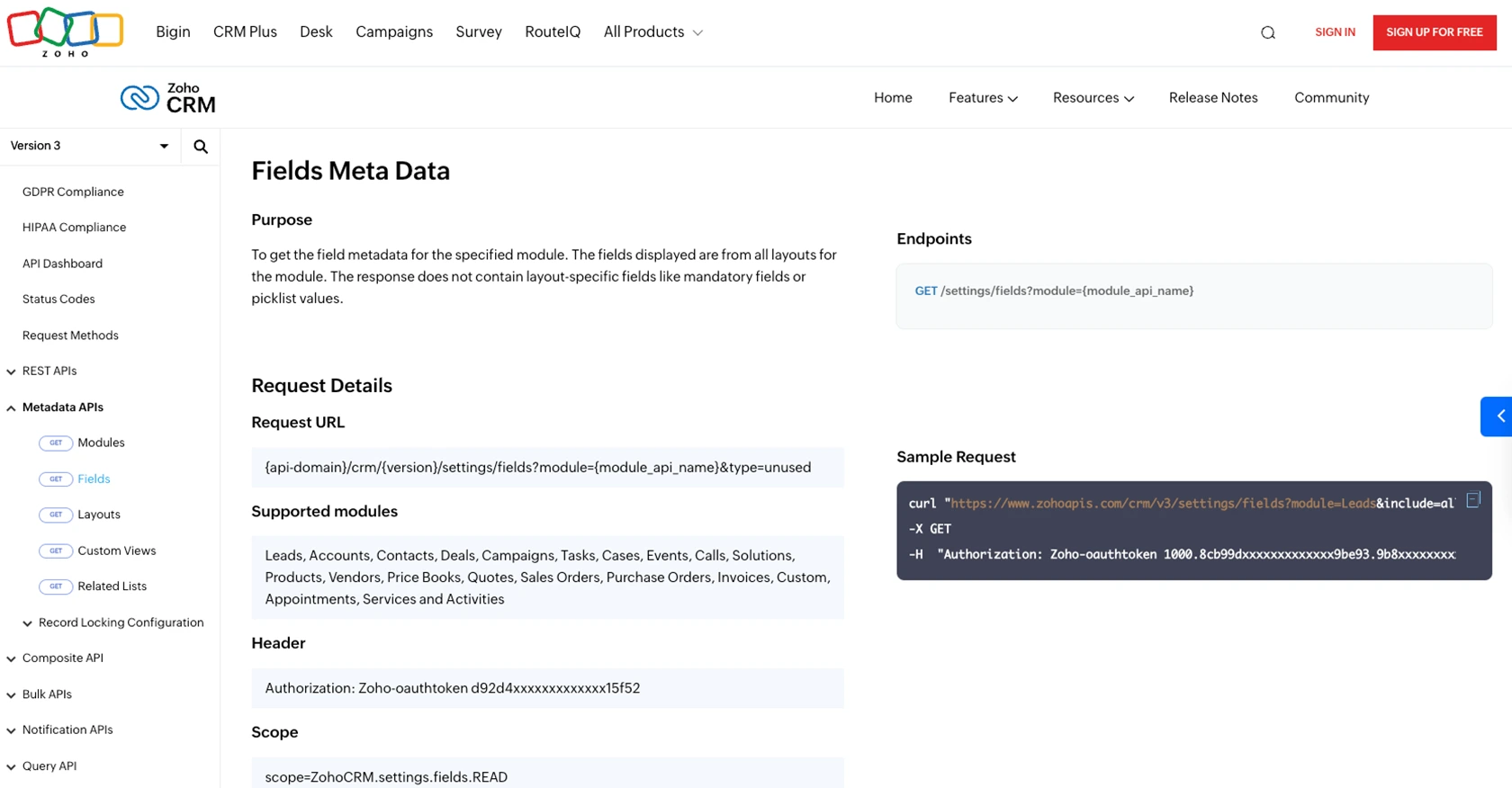
Conclusion and Best Practices for Zoho CRM API Integration
Integrating with the Zoho CRM API using JavaScript can significantly enhance your application's capabilities by allowing seamless access to user data. This integration not only improves team collaboration but also ensures that your CRM data is synchronized across various business applications.
Best Practices for Secure and Efficient Zoho CRM API Usage
- Secure Storage of Credentials: Always store your OAuth tokens securely. Avoid hardcoding them in your source code and consider using environment variables or secure vaults.
- Handle Rate Limiting: Zoho CRM imposes API rate limits. Monitor your API usage and implement retry logic with exponential backoff to handle rate limit errors gracefully. For more details, refer to the Zoho CRM API limits documentation.
- Data Transformation and Standardization: Ensure that the data retrieved from Zoho CRM is transformed and standardized to match your application's data model. This helps maintain consistency across different systems.
- Error Handling: Implement robust error handling to manage API response errors effectively. Use the information provided in the Zoho CRM API status codes documentation to handle specific error codes.
Streamline Your Integrations with Endgrate
While integrating with Zoho CRM can be highly beneficial, managing multiple integrations can become complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Zoho CRM. With Endgrate, you can:
- Save time and resources by outsourcing integrations and focusing on your core product.
- Build once for each use case instead of multiple times for different integrations.
- Offer an easy, intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate's website.
Read More
- https://endgrate.com/provider/zohocrm
- https://www.zoho.com/crm/developer/docs/api/v3/oauth-overview.html
- https://www.zoho.com/crm/developer/docs/api/v3/scopes.html
- https://www.zoho.com/crm/developer/docs/api/v3/register-client.html
- https://www.zoho.com/crm/developer/docs/api/v3/api-limits.html
- https://www.zoho.com/crm/developer/docs/api/v3/status-codes.html
- https://www.zoho.com/crm/developer/docs/api/v3/field-meta.html
- https://www.zoho.com/crm/developer/docs/api/v3/get-users.html
Ready to get started?