Using the Xero API to Create or Update Customers (with Javascript examples)
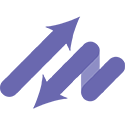
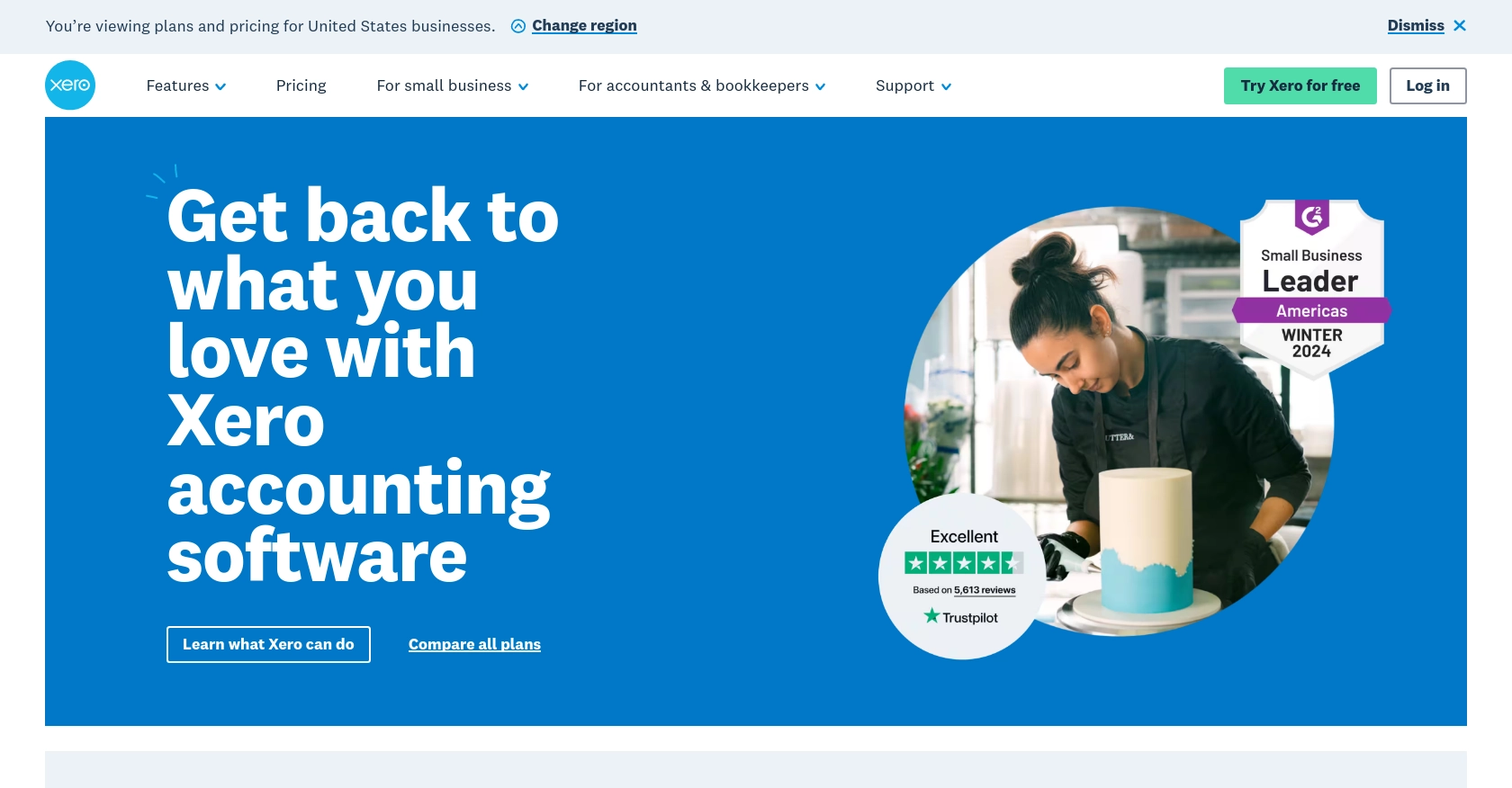
Introduction to Xero API Integration
Xero is a powerful cloud-based accounting software platform designed to help small and medium-sized businesses manage their finances efficiently. With features like invoicing, payroll, and expense tracking, Xero provides a comprehensive solution for financial management.
Developers often seek to integrate with Xero's API to automate and streamline accounting processes. For example, using the Xero API, a developer can create or update customer records directly from their application, ensuring that customer data is always up-to-date and synchronized across platforms.
This article will guide you through using JavaScript to interact with the Xero API, focusing on creating or updating customer records. By following this tutorial, you'll learn how to leverage Xero's API to enhance your application's functionality and improve data management.
Setting Up Your Xero Sandbox Account for API Integration
Before you can start integrating with the Xero API, you'll need to set up a sandbox account. This allows you to test your application without affecting live data. Xero provides a free demo company that you can use for this purpose.
Creating a Xero Developer Account
To begin, you'll need a Xero developer account. Follow these steps to create one:
- Visit the Xero Developer Portal.
- Click on "Sign Up" and fill out the registration form.
- Verify your email address to activate your account.
Accessing the Xero Demo Company
Once your developer account is set up, you can access the Xero demo company:
- Log in to your Xero account.
- Navigate to the "My Xero" tab.
- Select "Try the Demo Company" to access a sandbox environment.
Creating a Xero App for OAuth 2.0 Authentication
To interact with the Xero API, you'll need to create an app that uses OAuth 2.0 for authentication. Follow these steps:
- Go to the "My Apps" section in the Xero Developer Portal.
- Click on "New App" and fill in the required details, such as the app name and company URL.
- Set the redirect URI, which is the URL where users will be redirected after authentication.
- Save your app to generate the client ID and client secret.
Configuring OAuth 2.0 Scopes
Scopes define the level of access your app has to a user's data. For creating or updating customers, ensure you have the following scopes:
accounting.contacts
- Allows access to contact data.offline_access
- Enables refresh token functionality for long-term access.
Refer to the Xero OAuth 2.0 Scopes Documentation for more details.
Generating Access Tokens
With your app set up, you can now generate access tokens to authenticate API requests:
- Use the client ID and client secret to request an authorization code.
- Exchange the authorization code for an access token and refresh token.
- Store these tokens securely for future API calls.
For a detailed guide on the OAuth 2.0 flow, visit the Xero OAuth 2.0 Authorization Flow Documentation.
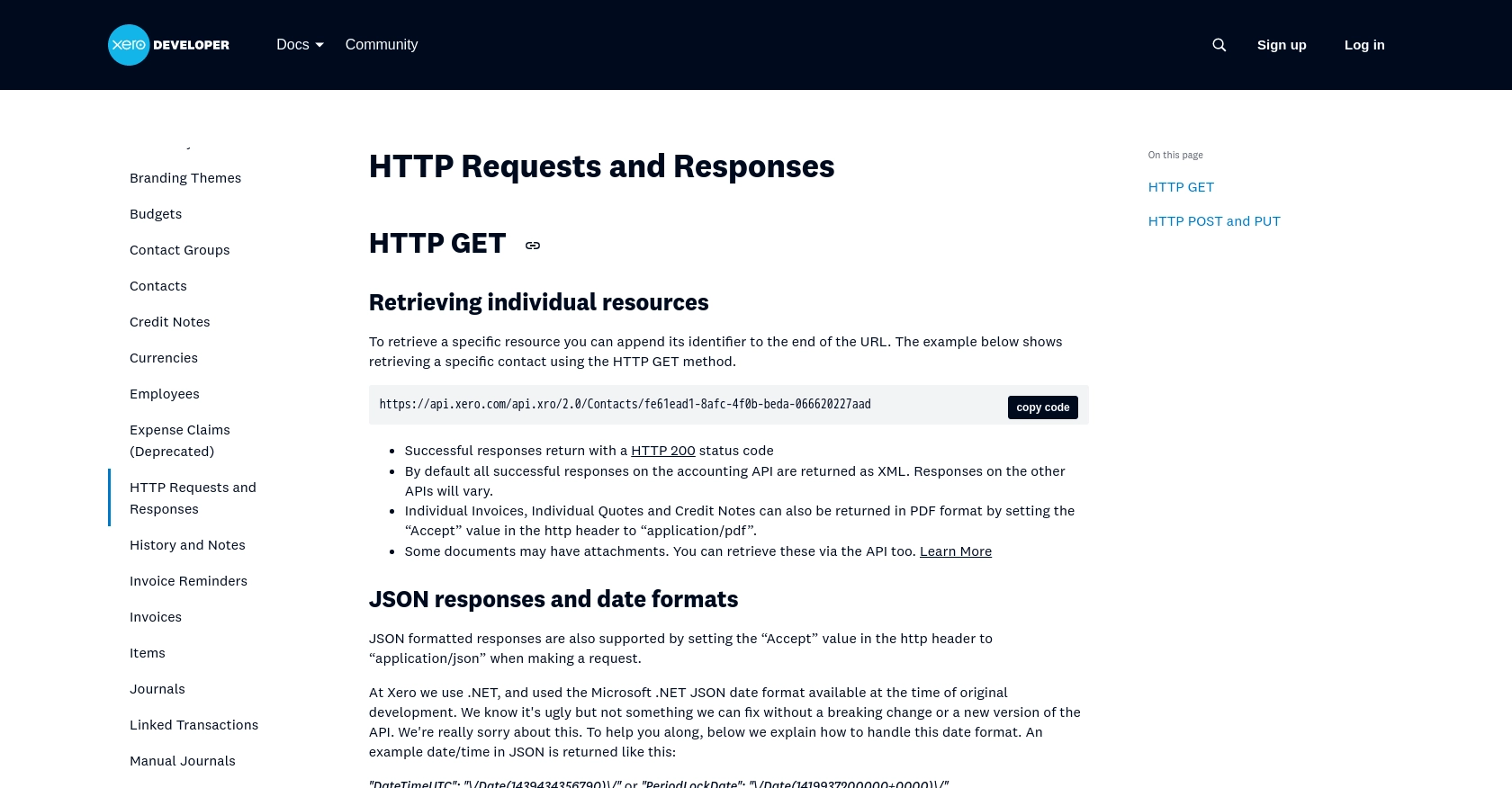
sbb-itb-96038d7
Making API Calls to Xero for Creating or Updating Customers Using JavaScript
To interact with the Xero API and manage customer records, you'll need to make HTTP requests using JavaScript. This section will guide you through the process of setting up your environment, writing the necessary code, and handling responses effectively.
Setting Up Your JavaScript Environment for Xero API Integration
Before making API calls, ensure you have the following prerequisites:
- Node.js installed on your machine.
- A package manager like npm or yarn.
- Familiarity with JavaScript and asynchronous programming.
Start by creating a new project directory and initializing it:
mkdir xero-api-integration
cd xero-api-integration
npm init -y
Installing Required Dependencies
You'll need the axios
library to make HTTP requests. Install it using npm:
npm install axios
Writing JavaScript Code to Create or Update Customers in Xero
Create a new file named manageCustomers.js
and add the following code:
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://api.xero.com/api.xro/2.0/Contacts';
const headers = {
'Authorization': 'Bearer YOUR_ACCESS_TOKEN',
'Content-Type': 'application/json'
};
// Define the customer data
const customerData = {
"Contacts": [{
"Name": "John Doe",
"EmailAddress": "john.doe@example.com"
}]
};
// Function to create or update a customer
async function manageCustomer() {
try {
const response = await axios.post(endpoint, customerData, { headers });
console.log('Customer created or updated successfully:', response.data);
} catch (error) {
console.error('Error creating or updating customer:', error.response.data);
}
}
manageCustomer();
Replace YOUR_ACCESS_TOKEN
with the access token you obtained during the OAuth 2.0 authentication process.
Running the JavaScript Code and Verifying Results
Execute the script using Node.js:
node manageCustomers.js
If successful, you should see a confirmation message in the console. Verify the changes in your Xero demo company to ensure the customer record has been created or updated.
Handling Errors and Understanding Xero API Responses
When making API calls, it's crucial to handle potential errors. The Xero API provides detailed error messages and codes. Common error codes include:
400
- Bad Request: Check your request syntax and data.401
- Unauthorized: Verify your access token and authentication setup.403
- Forbidden: Ensure your app has the necessary scopes.
Refer to the Xero API Contacts Documentation for more details on handling responses and errors.
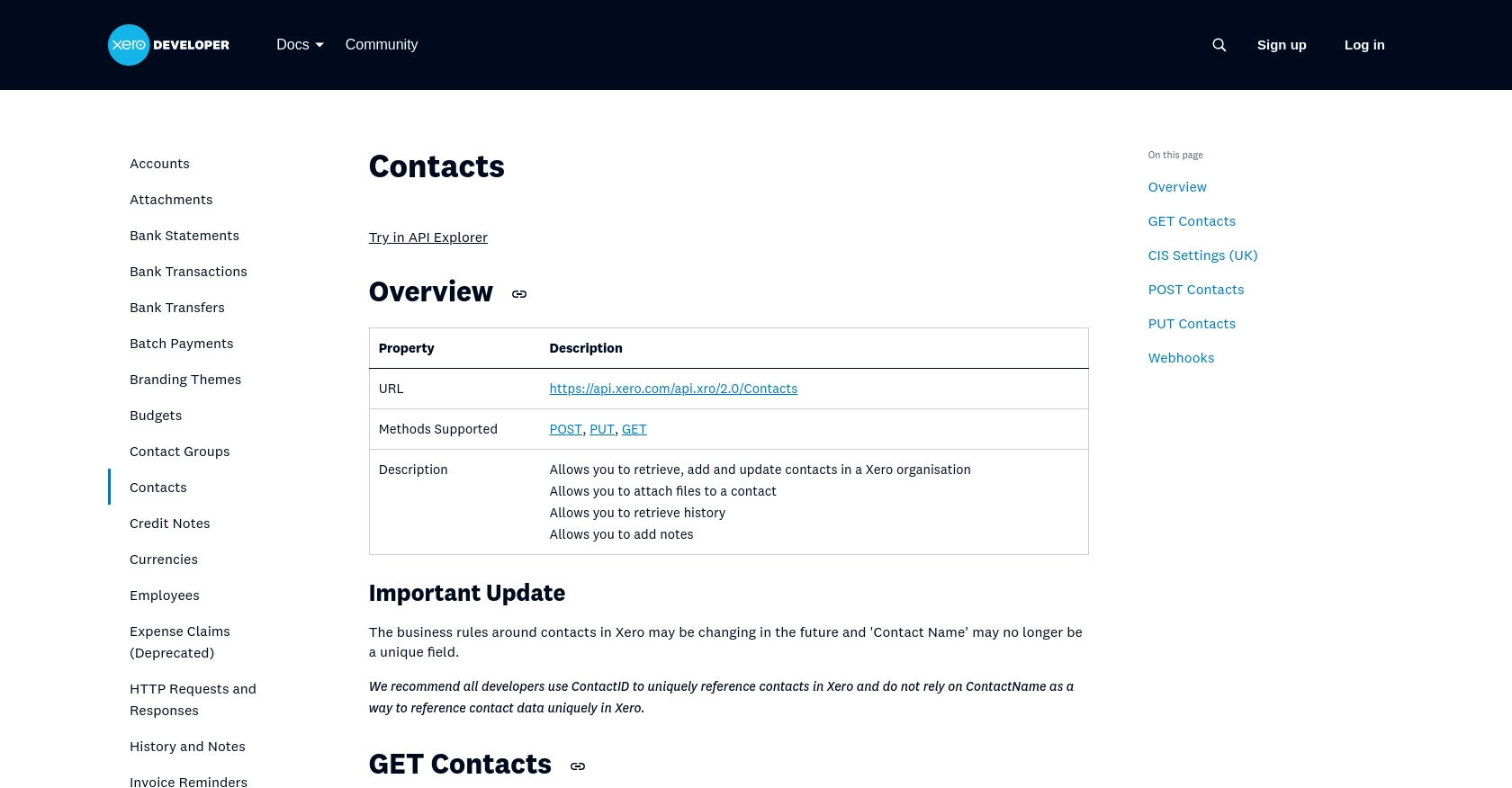
Conclusion and Best Practices for Xero API Integration
Integrating with the Xero API using JavaScript allows developers to efficiently manage customer data, ensuring synchronization across platforms. By following the steps outlined in this guide, you can create or update customer records seamlessly, enhancing your application's functionality.
Best Practices for Secure and Efficient Xero API Usage
- Securely Store Credentials: Always store your client ID, client secret, and access tokens securely. Consider using environment variables or secure vaults to protect sensitive information.
- Handle Rate Limiting: Xero's API has rate limits to prevent abuse. Monitor your API usage and implement retry logic with exponential backoff to handle rate limit errors gracefully. For more details, refer to the Xero OAuth 2.0 API Limits Documentation.
- Data Transformation and Standardization: Ensure that customer data is consistently formatted before making API calls. This helps prevent errors and maintains data integrity across systems.
Enhance Your Integration Strategy with Endgrate
While integrating with Xero is a powerful way to streamline accounting processes, managing multiple integrations can be complex and time-consuming. Endgrate simplifies this by providing a unified API endpoint that connects to various platforms, including Xero.
With Endgrate, you can:
- Save time and resources by outsourcing integrations and focusing on your core product.
- Build once for each use case instead of multiple times for different integrations.
- Offer an intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate today.
Read More
- https://endgrate.com/provider/xero
- https://developer.xero.com/documentation/api/accounting/requests-and-responses
- https://developer.xero.com/documentation/guides/oauth2/limits/
- https://developer.xero.com/documentation/guides/oauth2/overview
- https://developer.xero.com/documentation/guides/oauth2/scopes
- https://developer.xero.com/documentation/guides/oauth2/auth-flow
- https://developer.xero.com/documentation/guides/oauth2/tenants
- https://developer.xero.com/documentation/api/accounting/contacts
Ready to get started?