How to Get Departments with the FreshService API in PHP
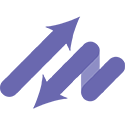
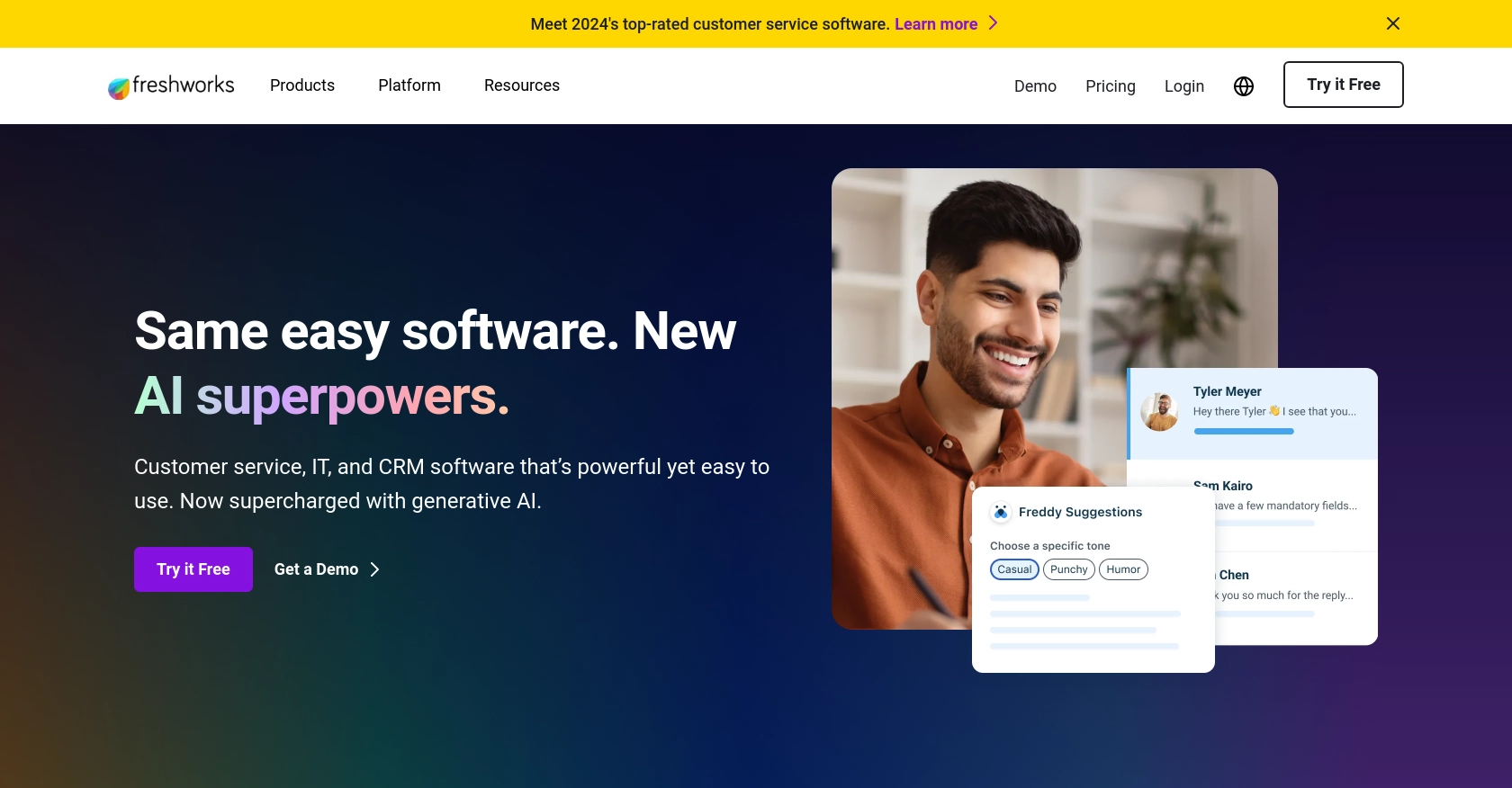
Introduction to FreshService API Integration
FreshService is a powerful cloud-based IT service management solution designed to help organizations streamline their IT operations. It offers a comprehensive suite of tools for managing incidents, assets, changes, and more, making it an ideal choice for businesses looking to enhance their IT service delivery.
Developers may want to integrate with the FreshService API to automate and optimize various IT service management tasks. For example, accessing department information through the FreshService API can enable developers to build custom dashboards or reports that provide insights into departmental IT resource usage and service requests.
Setting Up Your FreshService Test or Sandbox Account
Before you can start interacting with the FreshService API, you'll need to set up a test or sandbox account. This allows you to safely experiment with API calls without affecting your live data.
Creating a FreshService Account
If you don't already have a FreshService account, you can sign up for a free trial on the FreshService website. This trial will give you access to the features necessary for testing API interactions.
- Visit the FreshService signup page.
- Fill in the required information, such as your name, email, and company details.
- Follow the instructions to complete the registration process.
Generating API Key for FreshService Authentication
FreshService uses a custom authentication method that requires an API key. Here's how you can generate it:
- Log in to your FreshService account.
- Navigate to your profile settings by clicking on your profile icon in the top right corner.
- Select API Key from the dropdown menu.
- Copy the API key displayed on the screen. Keep it secure, as you'll need it to authenticate your API requests.
Configuring Your FreshService Sandbox Environment
Once you have your API key, you can configure your sandbox environment to test API calls:
- Ensure that your sandbox environment is set up with sample data to test API interactions effectively.
- Use the API key to authenticate your requests when making API calls in your sandbox environment.
With your FreshService account and API key ready, you're all set to start making API calls to retrieve department information using PHP.
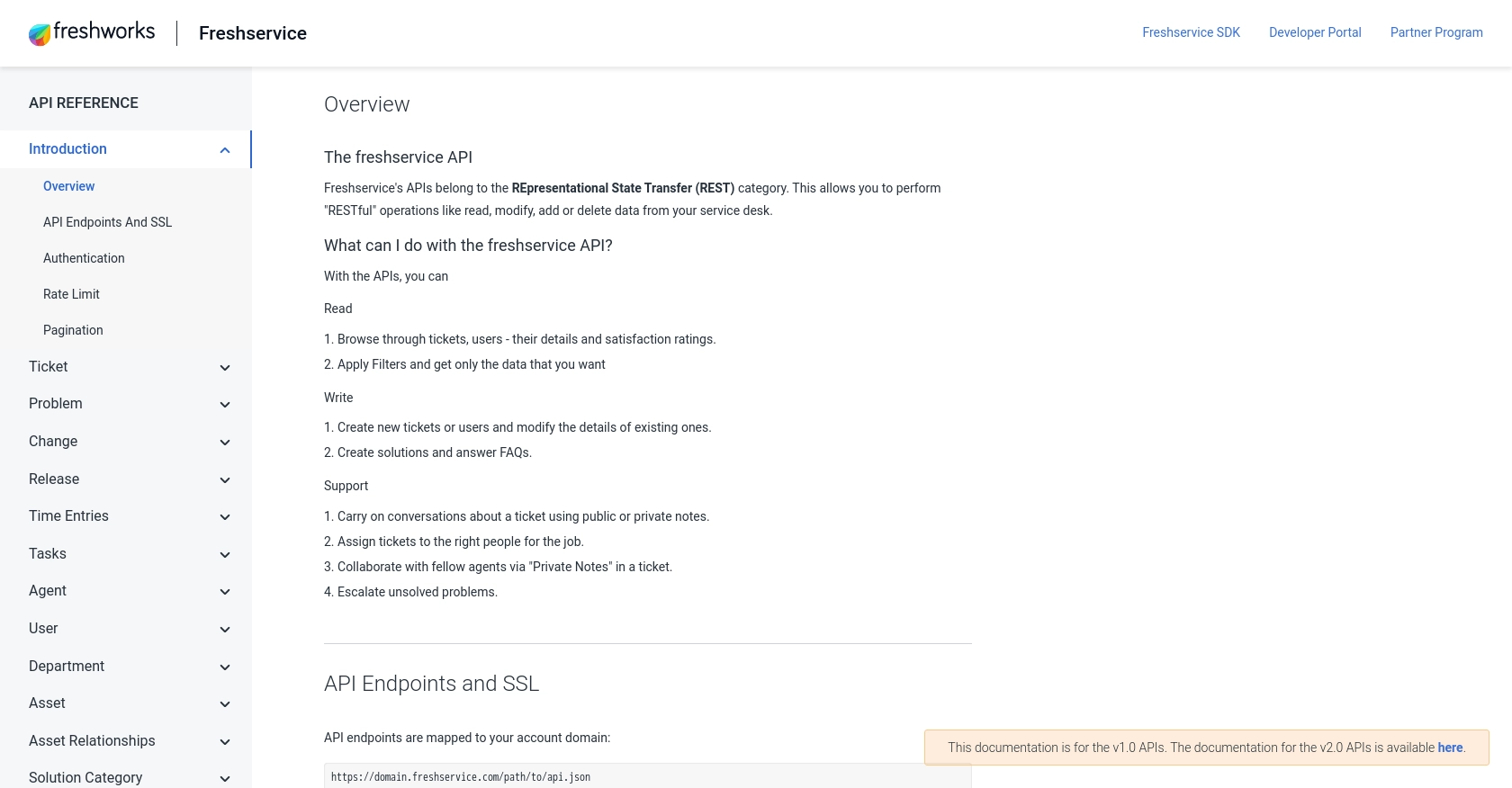
sbb-itb-96038d7
Making API Calls to Retrieve Departments with FreshService API in PHP
Now that you have your FreshService account and API key set up, it's time to dive into making API calls using PHP. This section will guide you through the process of retrieving department information from FreshService, ensuring you have the right tools and code to get started.
Setting Up Your PHP Environment for FreshService API Integration
Before making API calls, ensure your PHP environment is correctly configured. You'll need:
- PHP 7.4 or later
- cURL extension enabled
To verify cURL is enabled, you can create a phpinfo()
page or check your php.ini
file.
Installing Necessary PHP Dependencies
For this tutorial, we'll use PHP's built-in cURL functions to make HTTP requests. Ensure your PHP environment supports cURL, as it is essential for interacting with the FreshService API.
Example Code to Retrieve Departments from FreshService
Below is a sample PHP script to retrieve department information from FreshService:
<?php
// FreshService API endpoint for departments
$endpoint = "https://yourdomain.freshservice.com/api/v2/departments";
// Your FreshService API key
$api_key = "Your_API_Key";
// Initialize cURL session
$ch = curl_init($endpoint);
// Set cURL options
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, "$api_key:X");
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
"Content-Type: application/json"
));
// Execute cURL request
$response = curl_exec($ch);
// Check for errors
if (curl_errno($ch)) {
echo 'Error:' . curl_error($ch);
} else {
// Decode JSON response
$departments = json_decode($response, true);
// Display department information
foreach ($departments['departments'] as $department) {
echo "Department ID: " . $department['id'] . "\n";
echo "Department Name: " . $department['name'] . "\n\n";
}
}
// Close cURL session
curl_close($ch);
?>
Replace Your_API_Key
with your actual FreshService API key and yourdomain
with your FreshService domain.
Verifying API Call Success and Handling Errors
After running the script, you should see a list of departments printed to the console. If the request fails, ensure:
- Your API key is correct and has the necessary permissions.
- Your FreshService domain is correctly specified in the endpoint URL.
- cURL is enabled and properly configured in your PHP environment.
Handle errors by checking the response code and using curl_error()
to diagnose issues.
Conclusion and Best Practices for FreshService API Integration in PHP
Integrating with the FreshService API using PHP allows developers to efficiently manage and access IT service management data, such as department information. By following the steps outlined in this guide, you can successfully retrieve and utilize department data to enhance your IT operations.
Best Practices for Secure and Efficient FreshService API Usage
- Secure API Key Storage: Always store your FreshService API key securely. Avoid hardcoding it in your scripts. Consider using environment variables or secure vaults to manage sensitive credentials.
- Handle Rate Limiting: Be mindful of FreshService's API rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Transformation: Standardize and transform data fields as needed to ensure consistency across your applications and integrations.
- Error Handling: Implement robust error handling to capture and log errors effectively. Use HTTP status codes and error messages to diagnose issues promptly.
Streamlining Integrations with Endgrate
While integrating with FreshService directly can be rewarding, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process, allowing you to focus on your core product development.
With Endgrate, you can build once for each use case and leverage a single API endpoint to connect with various platforms, including FreshService. This approach not only saves time and resources but also provides an intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate's website and discover the benefits of outsourcing your integration needs.
Read More
Ready to get started?