Using the Woodpecker API to Create or Update Prospects (with Javascript examples)
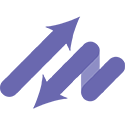
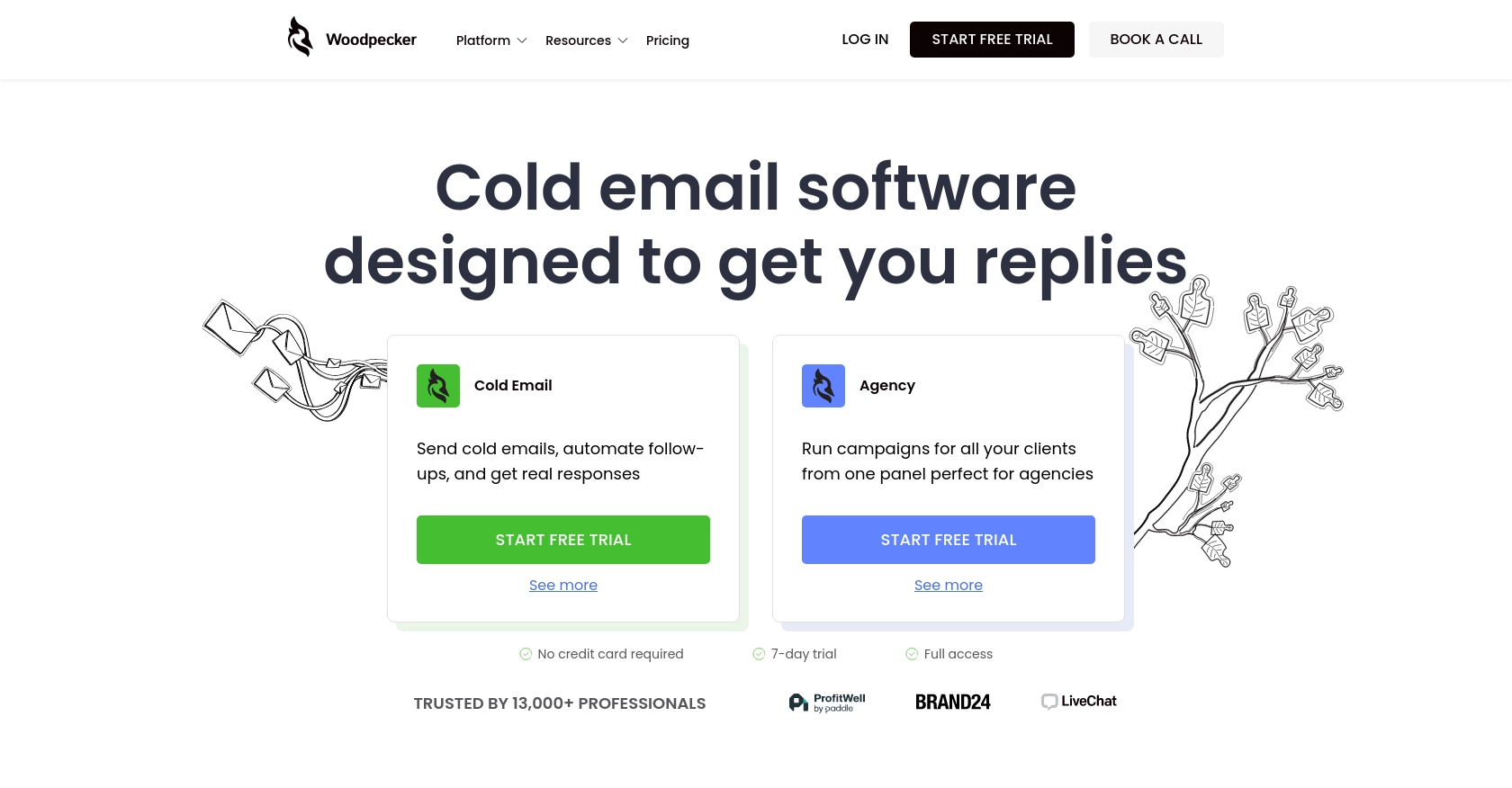
Introduction to Woodpecker API
Woodpecker is a powerful tool designed to enhance cold email campaigns for businesses, enabling them to automate and personalize outreach efforts. It offers features like email tracking, follow-ups, and integration capabilities that make it a preferred choice for sales teams and marketers.
Integrating with the Woodpecker API allows developers to efficiently manage prospects, automate data updates, and streamline communication processes. For example, a developer might use the Woodpecker API to automatically update prospect information from a CRM system, ensuring that the sales team always has the most current data for their outreach campaigns.
Setting Up Your Woodpecker Account for API Integration
Before you can start using the Woodpecker API to manage prospects, you'll need to set up your Woodpecker account and generate an API key. This key will allow you to authenticate your requests and interact with the API securely.
Creating a Woodpecker Account
If you don't already have a Woodpecker account, follow these steps to create one:
- Visit the Woodpecker website and click on "Start free trial" to sign up.
- Fill out the registration form with your details and submit it.
- Once your account is created, log in to access the Woodpecker dashboard.
Generating an API Key in Woodpecker
To interact with the Woodpecker API, you'll need to generate an API key. Follow these steps to obtain your key:
- Log into your Woodpecker account at app.woodpecker.co.
- Navigate to the "Marketplace" and select "INTEGRATIONS".
- Click on "API keys" and then use the green button to "CREATE A KEY".
- Copy the generated API key and store it securely, as you'll need it for authentication in your API requests.
For more detailed instructions, you can refer to the Woodpecker API key generation guide.
Understanding API Key Authentication
Woodpecker uses API key-based authentication. When making API requests, include your API key in the headers as follows:
headers: {
"Authorization": "Basic <API_KEY>"
}
Ensure your API key is encoded in Base64 format before using it in your requests. For more information on authentication, visit the Woodpecker authentication documentation.
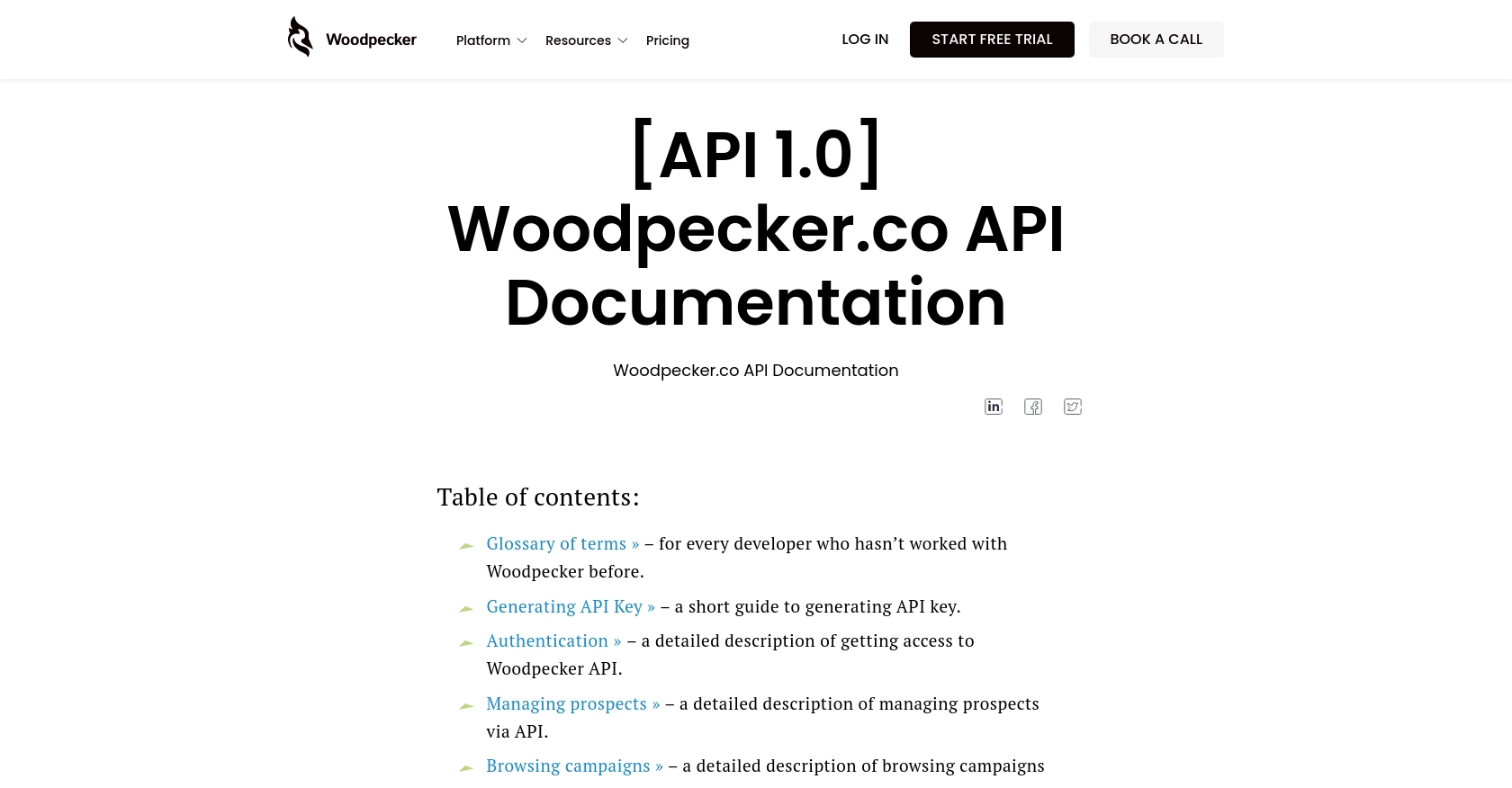
sbb-itb-96038d7
Making API Calls to Create or Update Prospects with Woodpecker API Using JavaScript
To interact with the Woodpecker API for creating or updating prospects, you'll need to make HTTP requests using JavaScript. This section will guide you through the process, including setting up your environment, writing the code, and handling responses.
Setting Up Your JavaScript Environment for Woodpecker API Integration
Before making API calls, ensure you have the following prerequisites:
- Node.js installed on your machine.
- A code editor like Visual Studio Code.
- The
axios
library for making HTTP requests. You can install it using npm:
npm install axios
Creating Prospects Using Woodpecker API with JavaScript
To create a new prospect in Woodpecker, use the following JavaScript code:
const axios = require('axios');
const createProspect = async () => {
const url = 'https://api.woodpecker.co/rest/v1/add_prospects_list';
const headers = {
'Authorization': 'Basic <API_KEY>',
'Content-Type': 'application/json'
};
const data = {
update: 'true',
prospects: [
{
email: 'newprospect@example.com',
first_name: 'John',
last_name: 'Doe',
company: 'Example Corp',
industry: 'Technology'
}
]
};
try {
const response = await axios.post(url, data, { headers });
console.log('Prospect Created:', response.data);
} catch (error) {
console.error('Error Creating Prospect:', error.response.data);
}
};
createProspect();
Replace <API_KEY>
with your actual API key. This code sends a POST request to the Woodpecker API to create a new prospect. If successful, it logs the response data.
Updating Prospects Using Woodpecker API with JavaScript
To update an existing prospect's information, use the following code:
const updateProspect = async () => {
const url = 'https://api.woodpecker.co/rest/v1/add_prospects_list';
const headers = {
'Authorization': 'Basic <API_KEY>',
'Content-Type': 'application/json'
};
const data = {
update: 'true',
prospects: [
{
id: 47356575,
email: 'updatedprospect@example.com',
first_name: 'Jane',
last_name: 'Smith'
}
]
};
try {
const response = await axios.post(url, data, { headers });
console.log('Prospect Updated:', response.data);
} catch (error) {
console.error('Error Updating Prospect:', error.response.data);
}
};
updateProspect();
This code updates the prospect's details by sending a POST request with the prospect's ID and new information.
Handling API Responses and Errors
When making API calls, it's crucial to handle responses and potential errors. The Woodpecker API will return a status code and message indicating the success or failure of your request. Check the response status and log any errors for troubleshooting.
For more details on error codes, refer to the Woodpecker API documentation.
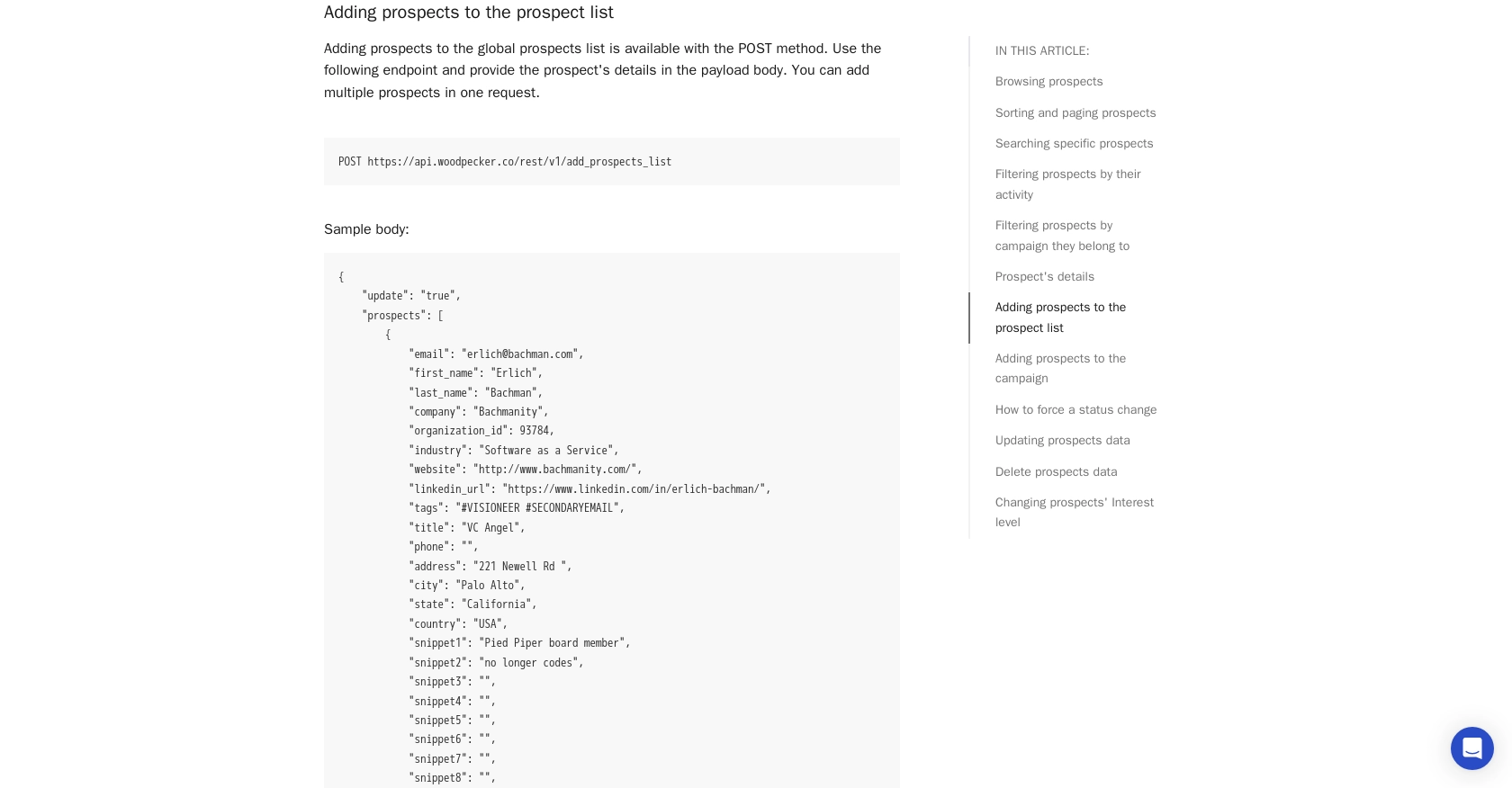
Conclusion and Best Practices for Using Woodpecker API with JavaScript
Integrating with the Woodpecker API using JavaScript provides a powerful way to automate and enhance your cold email campaigns. By efficiently managing prospects and keeping your data up-to-date, you can ensure that your sales and marketing efforts are always aligned with the latest information.
Best Practices for Secure and Efficient Woodpecker API Integration
- Secure API Key Storage: Always store your API key securely and avoid hardcoding it in your source files. Consider using environment variables or secure vaults to manage sensitive information.
- Handle Rate Limits: Although Woodpecker allows unlimited API calls per month, remember that only one request can be processed at a time, with a 15-second limit for queued requests. Plan your API calls accordingly to avoid dropped requests.
- Data Standardization: Ensure that data fields are standardized before sending them to the API. This helps in maintaining consistency across your systems and improves data integrity.
- Error Handling: Implement robust error handling to manage API response errors effectively. Log errors for troubleshooting and ensure your application can gracefully handle failures.
Streamlining Integrations with Endgrate
If managing multiple integrations becomes overwhelming, consider using Endgrate to simplify the process. Endgrate allows you to build once for each use case and leverage a unified API endpoint for various platforms, including Woodpecker. This approach saves time and resources, allowing you to focus on your core product while providing an intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate today.
Read More
Ready to get started?