How to Create Or Update Companies with the Pipeliner CRM API in PHP
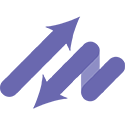
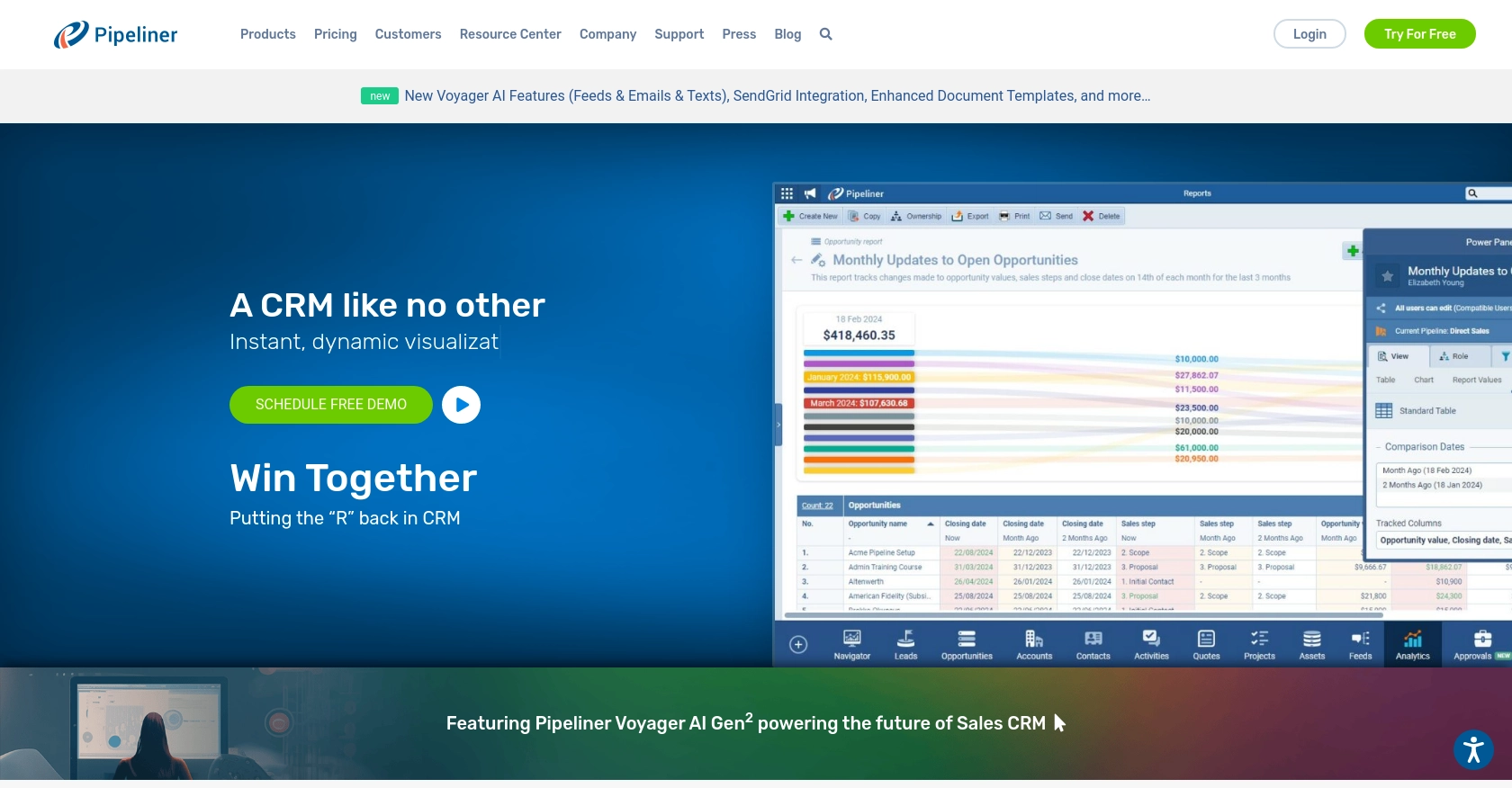
Introduction to Pipeliner CRM
Pipeliner CRM is a powerful customer relationship management platform designed to enhance sales processes and improve team collaboration. With its intuitive interface and robust features, Pipeliner CRM helps businesses manage leads, track opportunities, and streamline customer interactions.
Integrating with the Pipeliner CRM API allows developers to automate and optimize various CRM tasks, such as creating or updating company records. For example, a developer might use the API to automatically update company information in Pipeliner CRM whenever changes occur in an external database, ensuring that sales teams always have access to the most current data.
Setting Up Your Pipeliner CRM Test Account
Before you can start integrating with the Pipeliner CRM API, you need to set up a test account. This will allow you to safely experiment with API calls without affecting live data.
Creating a Pipeliner CRM Account
To begin, you'll need a Pipeliner CRM account. If you don't already have one, follow these steps to create a free trial account:
- Visit the Pipeliner CRM website and sign up for a free trial.
- Follow the on-screen instructions to complete the registration process.
- Once your account is created, log in to access your Pipeliner CRM space.
Generating API Keys for Pipeliner CRM
Pipeliner CRM uses Basic authentication, which requires an API key consisting of a username and password. Follow these steps to obtain your API keys:
- Log in to your Pipeliner CRM space.
- Navigate to the administration section by selecting your space.
- Under the "Unit, Users & Roles" tab, open "Applications."
- Create a new application and click "Show API Access" to generate your API keys.
- Note that the username and password are generated only once, so store them securely.
These credentials will be used for authenticating your API requests.
Understanding Pipeliner CRM API Authentication
With your API keys ready, you can now authenticate your requests to the Pipeliner CRM API. The authentication process involves using the generated username and password as part of the Basic authentication header in your API calls.
For more details on authentication, refer to the Pipeliner CRM Authentication Documentation.
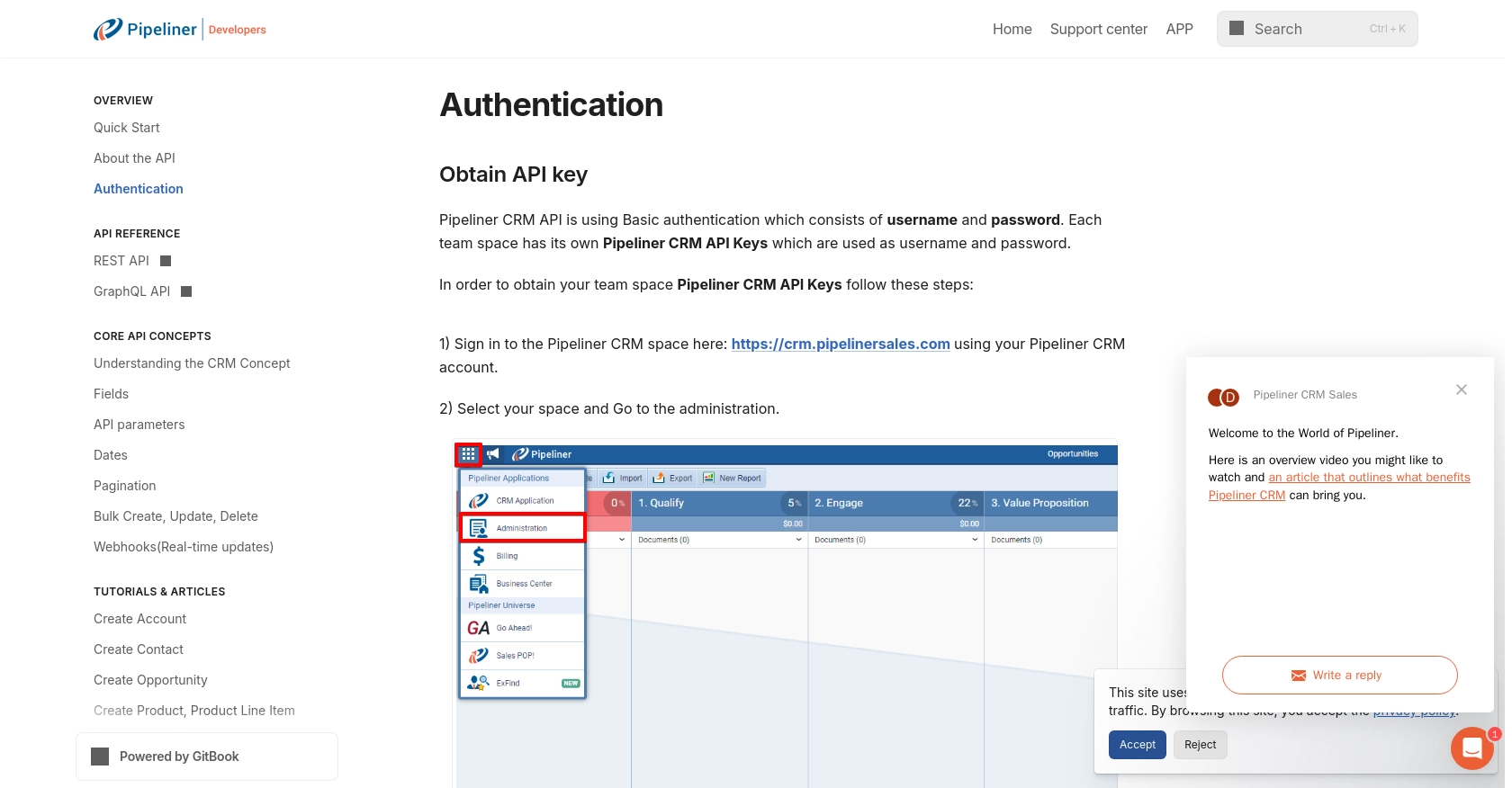
sbb-itb-96038d7
Making API Calls to Create or Update Companies in Pipeliner CRM Using PHP
To interact with the Pipeliner CRM API for creating or updating company records, you'll need to use PHP. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling responses.
Setting Up Your PHP Environment for Pipeliner CRM API Integration
Before making API calls, ensure you have the following prerequisites installed on your machine:
- PHP 7.4 or higher
- cURL extension for PHP
To install the cURL extension, you can use the following command:
sudo apt-get install php-curl
Creating a Company with the Pipeliner CRM API Using PHP
To create a new company in Pipeliner CRM, you'll need to make a POST request to the appropriate endpoint. Here's a sample PHP script to achieve this:
<?php
$apiUrl = 'https://api.pipelinersales.com/v1/companies';
$username = 'your_api_username';
$password = 'your_api_password';
$data = [
'name' => 'New Company Name',
'industry' => 'Technology',
'website' => 'https://newcompany.com'
];
$options = [
CURLOPT_URL => $apiUrl,
CURLOPT_RETURNTRANSFER => true,
CURLOPT_POST => true,
CURLOPT_POSTFIELDS => json_encode($data),
CURLOPT_HTTPHEADER => [
'Content-Type: application/json'
],
CURLOPT_USERPWD => $username . ':' . $password
];
$ch = curl_init();
curl_setopt_array($ch, $options);
$response = curl_exec($ch);
curl_close($ch);
echo $response;
?>
Replace your_api_username
and your_api_password
with your actual API credentials. This script sends a POST request to create a new company with specified details.
Updating a Company with the Pipeliner CRM API Using PHP
To update an existing company, you'll need to make a PUT request. Here's how you can do it:
<?php
$apiUrl = 'https://api.pipelinersales.com/v1/companies/{company_id}';
$username = 'your_api_username';
$password = 'your_api_password';
$data = [
'name' => 'Updated Company Name',
'industry' => 'Finance'
];
$options = [
CURLOPT_URL => $apiUrl,
CURLOPT_RETURNTRANSFER => true,
CURLOPT_CUSTOMREQUEST => 'PUT',
CURLOPT_POSTFIELDS => json_encode($data),
CURLOPT_HTTPHEADER => [
'Content-Type: application/json'
],
CURLOPT_USERPWD => $username . ':' . $password
];
$ch = curl_init();
curl_setopt_array($ch, $options);
$response = curl_exec($ch);
curl_close($ch);
echo $response;
?>
Ensure you replace {company_id}
with the actual ID of the company you wish to update. This script updates the company's name and industry.
Handling API Responses and Errors in Pipeliner CRM
After making an API call, it's crucial to handle the response correctly. Check the HTTP status code to determine if the request was successful:
- 200 OK: The request was successful.
- 400 Bad Request: The request was invalid. Check the data sent.
- 401 Unauthorized: Authentication failed. Verify your credentials.
- 404 Not Found: The specified resource could not be found.
For more detailed error handling, refer to the Pipeliner CRM API Documentation.
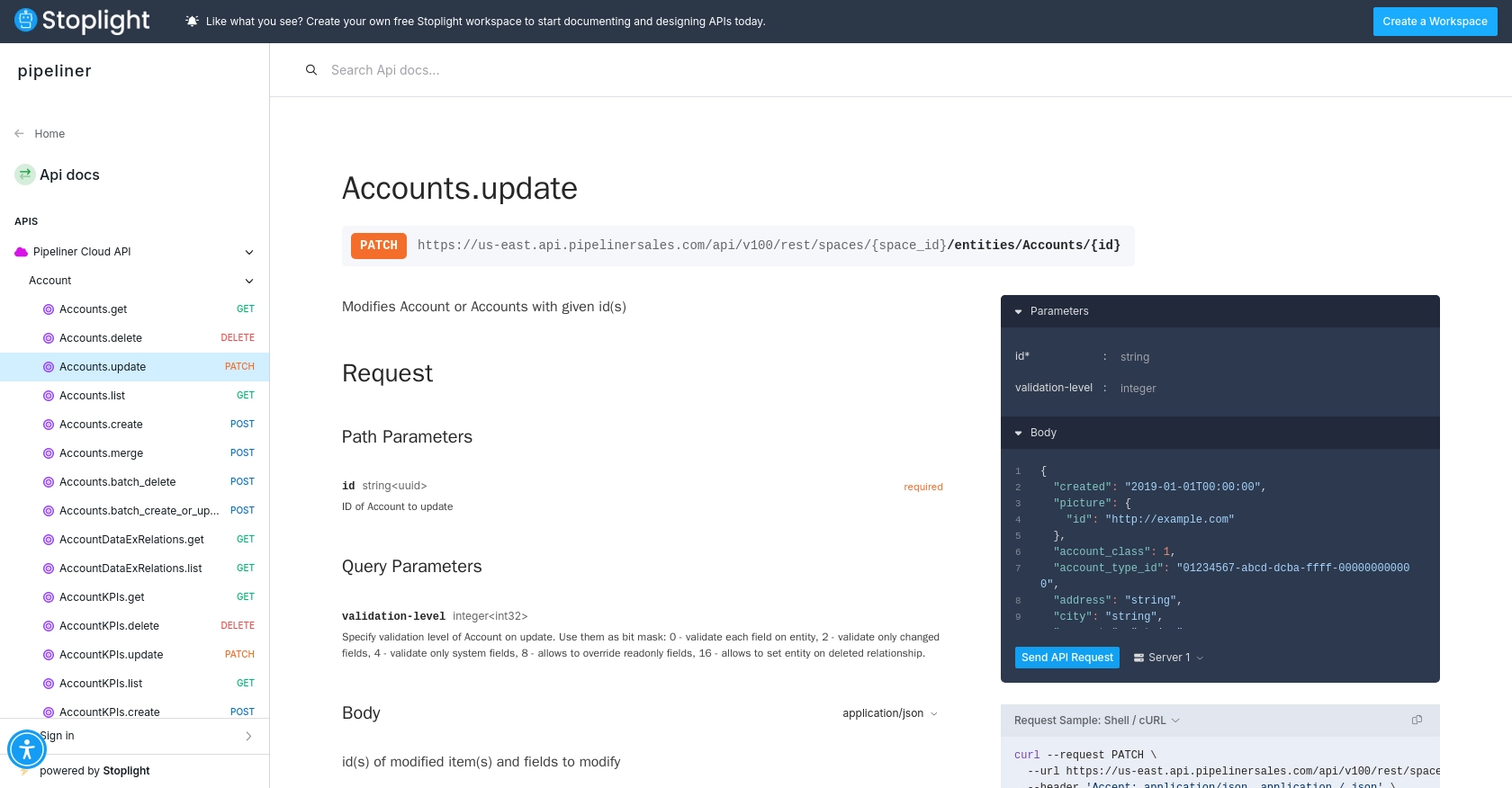
Conclusion and Best Practices for Pipeliner CRM API Integration
Integrating with the Pipeliner CRM API using PHP allows developers to efficiently manage company records, ensuring that sales teams have access to up-to-date information. By automating the creation and updating of company data, businesses can streamline their CRM processes and enhance team productivity.
Best Practices for Secure and Efficient Pipeliner CRM API Usage
- Secure Storage of Credentials: Always store your API credentials securely. Consider using environment variables or a secure vault to protect your username and password.
- Handle Rate Limiting: Be mindful of API rate limits to avoid service disruptions. Implement logic to handle rate limit responses and retry requests after a delay if necessary.
- Data Standardization: Ensure that data fields are standardized before sending them to the API. This helps maintain consistency and prevents errors during data processing.
- Error Handling: Implement robust error handling to manage different HTTP status codes and provide meaningful feedback to users or logs for debugging.
Streamline Your Integrations with Endgrate
While integrating with Pipeliner CRM can greatly enhance your CRM capabilities, managing multiple integrations can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Pipeliner CRM.
By using Endgrate, you can save time and resources, allowing your team to focus on core product development. Whether you need to build once for multiple integrations or provide an intuitive integration experience for your customers, Endgrate offers a scalable solution to meet your needs.
Explore how Endgrate can transform your integration strategy by visiting Endgrate's website today.
Read More
- https://endgrate.com/provider/pipelinercrm
- https://developers.pipelinersales.com/api-docs/overview/authentication
- https://developers.pipelinersales.com/api-docs/core-api-concepts/pagination
- https://pipeliner.stoplight.io/docs/api-docs/p5b3cyeorbick-accounts-update
- https://pipeliner.stoplight.io/docs/api-docs/89p8xi32mv7cj-accounts-create
Ready to get started?