How to Get Questions with the Typeform API in Python
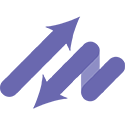
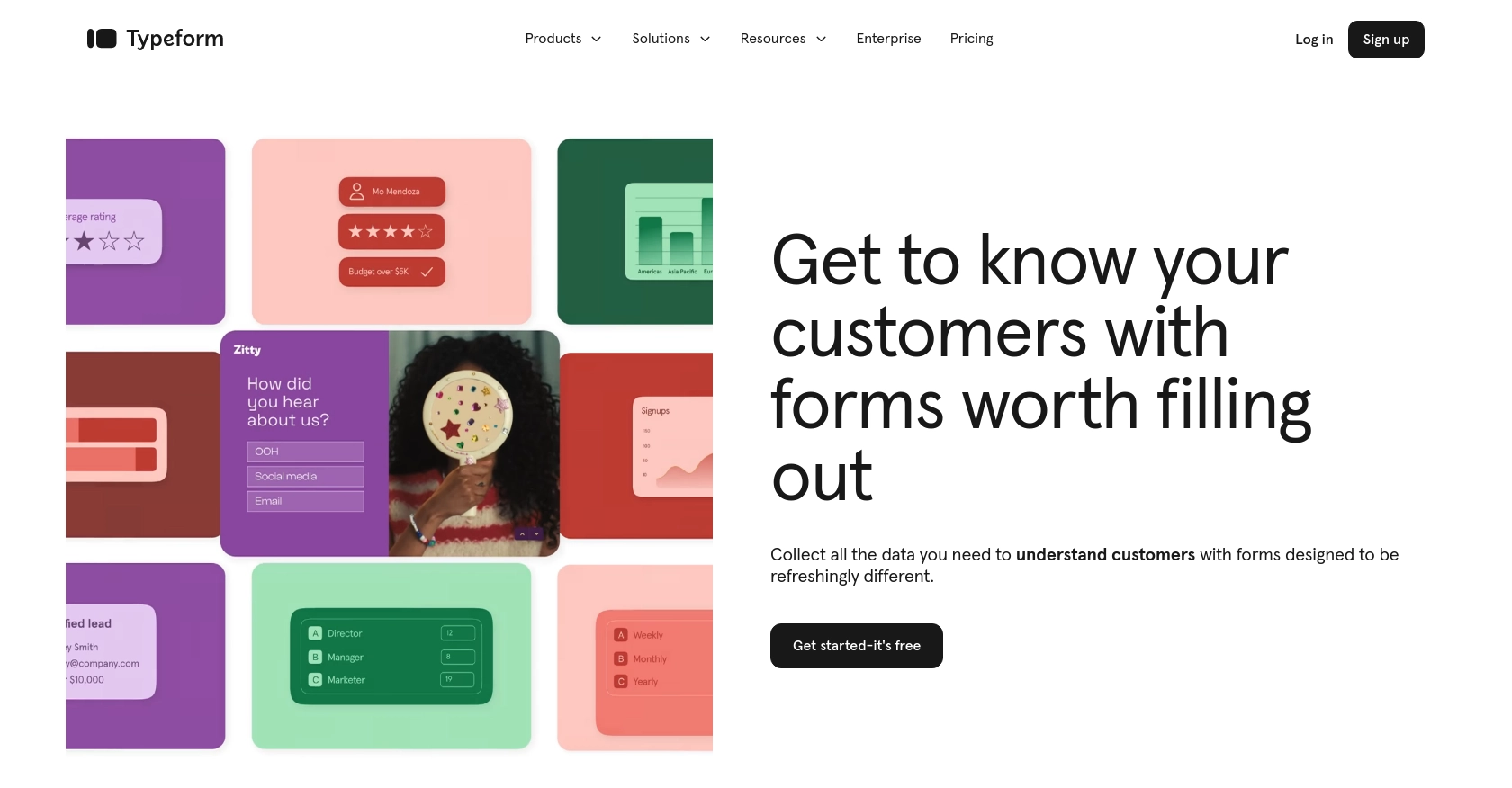
Introduction to Typeform API Integration
Typeform is a versatile online form builder that allows businesses to create engaging and interactive forms, surveys, and quizzes. Its user-friendly interface and customizable templates make it a popular choice for collecting data and feedback from users.
Integrating with Typeform's API enables developers to automate data retrieval and management processes. For example, a developer might want to access questions from a Typeform survey using Python to analyze responses or integrate them into a larger data processing workflow.
This article will guide you through the process of using Python to interact with the Typeform API, specifically focusing on retrieving questions from a form. By the end of this tutorial, you'll be able to efficiently access and manage Typeform data within your applications.
Setting Up Your Typeform Developer Account and Application
Before you can start interacting with the Typeform API, you need to set up a developer account and create an application. This will allow you to authenticate your requests and access the necessary API endpoints.
Creating a Typeform Developer Account
If you don't already have a Typeform account, you'll need to create one. Follow these steps to get started:
- Visit the Typeform website and sign up for a free account.
- Once your account is created, log in to access the Typeform dashboard.
Registering a New Application in Typeform
To interact with the Typeform API, you need to register a new application. This process will provide you with the client_id
and client_secret
required for OAuth 2.0 authentication.
- Log in to your Typeform account and navigate to the Developer Apps section.
- Click on Register a new app.
- Fill in the required fields, including the App Name, App Website, and Redirect URI(s).
- Click Register app to complete the registration.
After registration, you'll receive your client_id
and client_secret
. Make sure to store these securely, as they are essential for authenticating your API requests.
Setting Up OAuth 2.0 Authentication
Typeform uses OAuth 2.0 for secure API access. Follow these steps to set up authentication:
- Direct users to the authorization URL:
https://api.typeform.com/oauth/authorize
, including yourclient_id
,redirect_uri
, and desired scopes. - Once users grant access, you'll receive a temporary authorization code at your specified redirect URI.
- Exchange the authorization code for an access token by making a POST request to
https://api.typeform.com/oauth/token
with yourclient_id
,client_secret
, and authorization code.
For more details on OAuth 2.0 setup, refer to the Typeform Applications documentation and OAuth 2.0 scopes documentation.
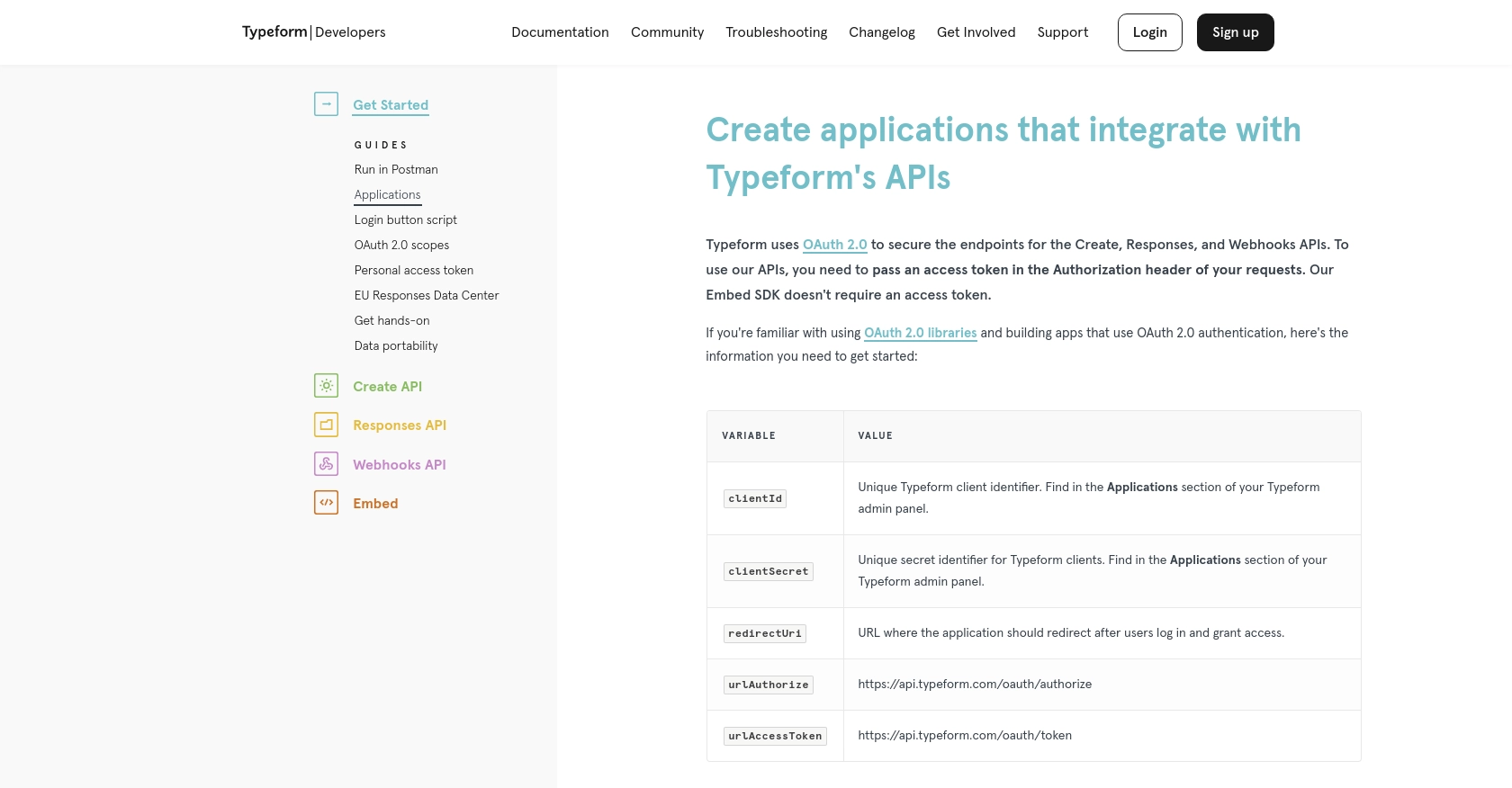
sbb-itb-96038d7
Making API Calls to Retrieve Questions from Typeform Forms Using Python
To interact with the Typeform API and retrieve questions from a form, you'll need to use Python. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling potential errors.
Setting Up Your Python Environment for Typeform API Integration
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests. Install it using pip:
pip install requests
Writing Python Code to Retrieve Questions from Typeform Forms
Once your environment is set up, you can write a Python script to fetch questions from a Typeform form. Create a file named get_typeform_questions.py
and add the following code:
import requests
# Replace with your actual access token
access_token = "Your_Access_Token"
# Replace with your form ID
form_id = "Your_Form_ID"
# Set the API endpoint
url = f"https://api.typeform.com/forms/{form_id}"
# Set the request headers
headers = {
"Authorization": f"Bearer {access_token}"
}
# Make a GET request to the API
response = requests.get(url, headers=headers)
# Check if the request was successful
if response.status_code == 200:
data = response.json()
questions = data.get("fields", [])
for question in questions:
print(f"Question: {question.get('title')}")
else:
print(f"Failed to retrieve questions: {response.status_code} - {response.text}")
Replace Your_Access_Token
and Your_Form_ID
with your actual Typeform access token and form ID.
Verifying API Call Success and Handling Errors
After running the script, you should see the list of questions from your Typeform form printed in the console. If the request fails, the script will output an error message with the status code and response text.
Common error codes include:
- 401 Unauthorized: Check your access token and ensure it is valid.
- 404 Not Found: Verify the form ID is correct.
- 429 Too Many Requests: You have hit the rate limit. Wait before making more requests.
For more details on error handling, refer to the Typeform API documentation.
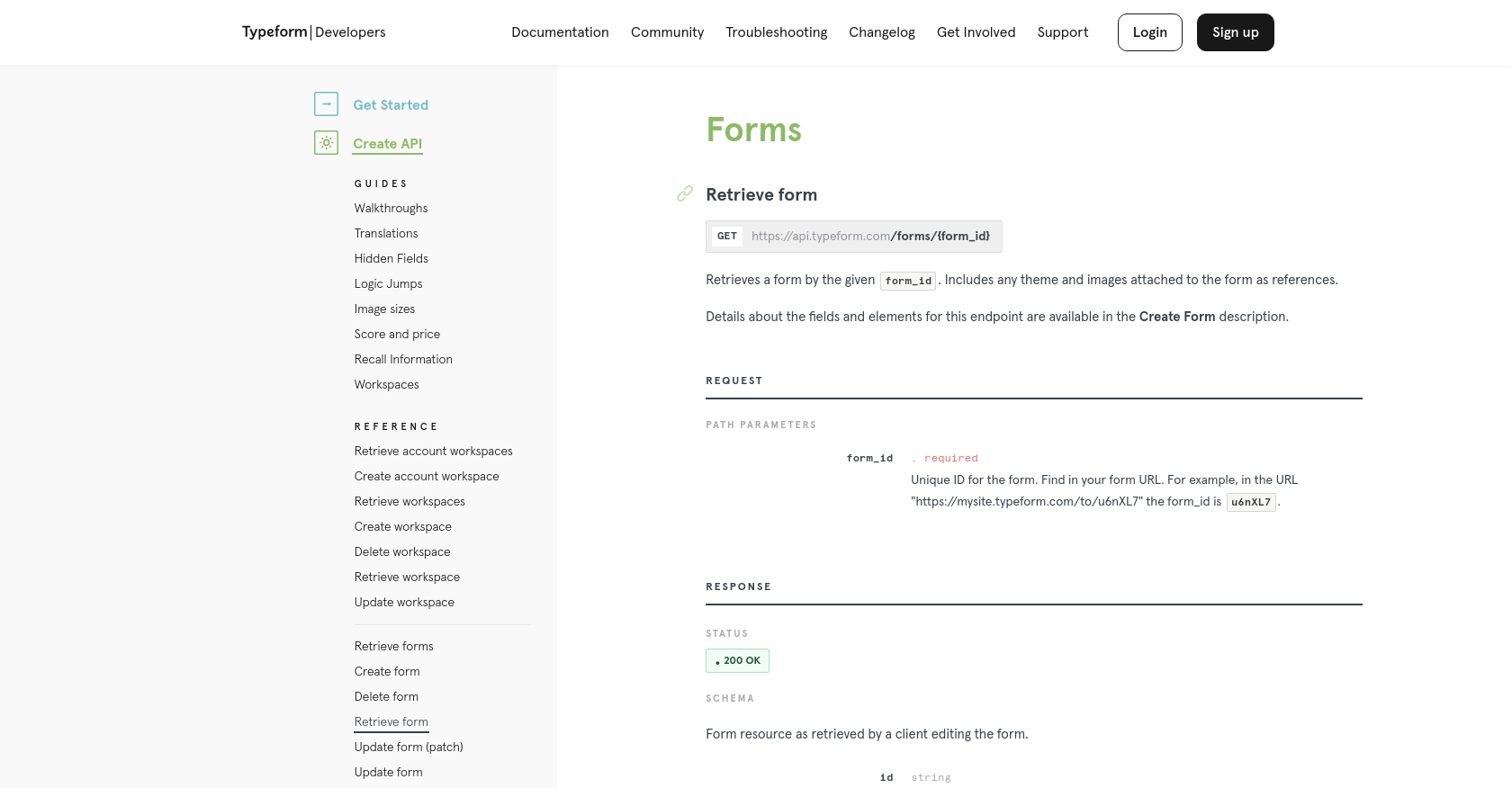
Conclusion and Best Practices for Typeform API Integration
Integrating with the Typeform API using Python allows developers to efficiently manage and retrieve form data, enhancing data-driven decision-making processes. By following the steps outlined in this guide, you can seamlessly access Typeform questions and incorporate them into your applications.
Best Practices for Secure and Efficient Typeform API Usage
- Securely Store Credentials: Always keep your
client_id
,client_secret
, and access tokens secure. Avoid hardcoding them in your source code and consider using environment variables or secure vaults. - Handle Rate Limiting: Be mindful of Typeform's rate limits to avoid disruptions. Implement retry logic with exponential backoff to manage
429 Too Many Requests
errors gracefully. - Data Transformation: Standardize and transform data fields as needed to ensure compatibility with your application's data models and workflows.
Streamline Your Integration Process with Endgrate
While integrating with Typeform is straightforward, managing multiple integrations can become complex. Endgrate simplifies this process by providing a unified API endpoint for various platforms, including Typeform. By leveraging Endgrate, you can save time and resources, allowing you to focus on your core product development.
Explore how Endgrate can enhance your integration experience by visiting Endgrate and discover how it can help you build efficient, scalable integrations across multiple platforms.
Read More
Ready to get started?