How to Get Companies with the Copper API in Javascript
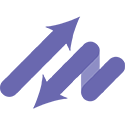
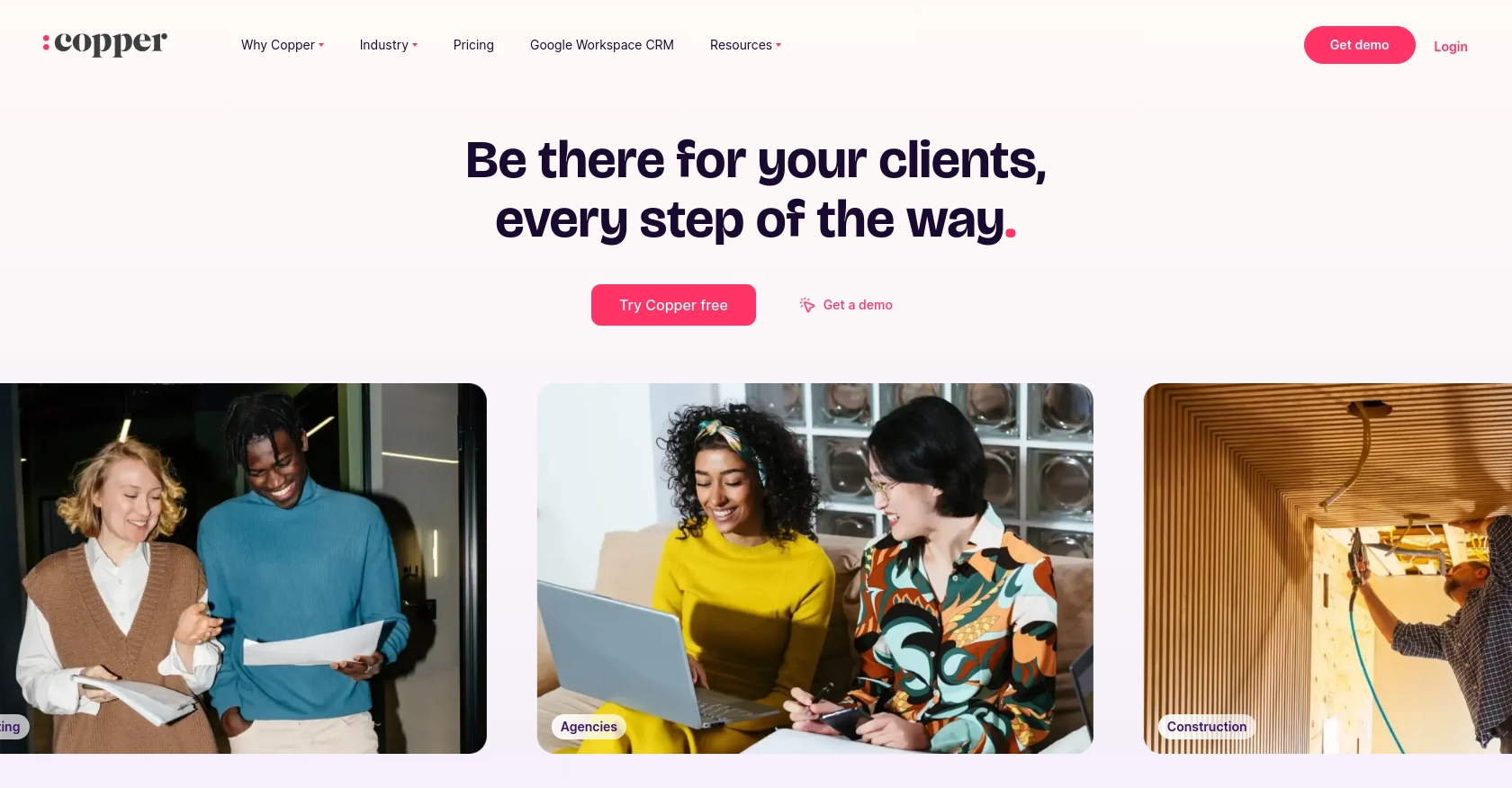
Introduction to Copper API for Company Data Retrieval
Copper is a robust platform offering a suite of tools for managing digital assets and financial operations. It provides a powerful API that allows developers to integrate and interact with various functionalities, including retrieving company data.
Integrating with Copper's API can be highly beneficial for developers looking to streamline data management processes. For example, you might want to retrieve detailed company information to enhance your CRM system or to automate reporting tasks.
Setting Up Your Copper API Test/Sandbox Account
Before you can start retrieving company data using the Copper API, you'll need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting live data.
Create a Copper Account
If you don't already have a Copper account, you can sign up for a free trial or demo account on the Copper website. This will give you access to the platform's features and allow you to explore the API capabilities.
- Visit the Copper website and navigate to the sign-up page.
- Follow the instructions to create your account.
- Once your account is created, log in to access the Copper dashboard.
Generate Copper API Key and Secret
To authenticate your API requests, you'll need an API key and secret. Follow these steps to generate them:
- Log in to your Copper account and go to the API settings section.
- Click on "Create API Key" to generate a new key.
- Copy the API key and secret provided. Store them securely as you'll need them for authentication.
Configure API Authentication
With your API key and secret, you can now configure authentication for your API requests. Copper uses a custom authentication method that involves signing requests with your API secret.
// Example of generating a signature in JavaScript
const crypto = require('crypto');
const apiKey = 'your_api_key';
const secret = 'your_api_secret';
const timestamp = Date.now().toString();
const method = 'GET';
const path = '/platform/companies';
const body = '';
const signature = crypto.createHmac('sha256', secret)
.update(timestamp + method + path + body)
.digest('hex');
console.log('Signature:', signature);
Include the following headers in your API requests:
- Authorization: ApiKey your_api_key
- X-Signature: generated_signature
- X-Timestamp: current_timestamp_in_milliseconds
Verify API Access
To ensure that your setup is correct, make a test API call to verify access. You can use a tool like Postman or write a simple script to send a request to the Copper API endpoint.
// Example of a test API call using fetch
fetch('https://api.copper.co/platform/companies', {
method: 'GET',
headers: {
'Authorization': `ApiKey ${apiKey}`,
'X-Signature': signature,
'X-Timestamp': timestamp
}
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
Check the response to confirm that your API key and secret are working correctly. You should receive a list of companies if everything is set up properly.
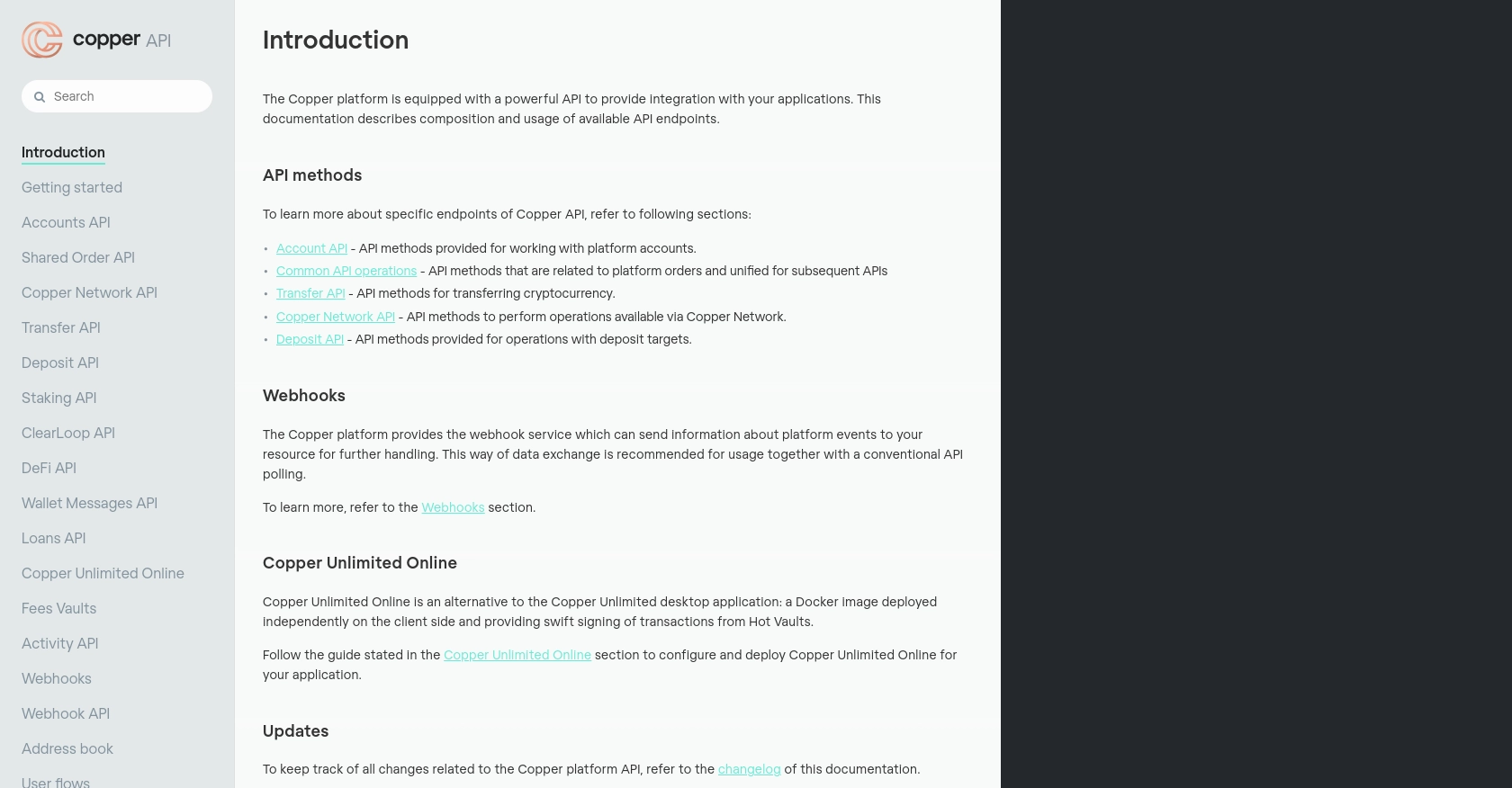
sbb-itb-96038d7
Executing Copper API Calls in JavaScript for Company Data Retrieval
To effectively interact with the Copper API and retrieve company data, you'll need to ensure that your JavaScript environment is properly configured. This section will guide you through the necessary steps to make successful API calls using JavaScript.
Setting Up Your JavaScript Environment for Copper API
Before making API calls, ensure you have Node.js installed on your machine. This will allow you to run JavaScript code outside of a browser environment. Additionally, you'll need the node-fetch
package to handle HTTP requests.
npm install node-fetch
Making a GET Request to Retrieve Company Data from Copper API
With your environment set up, you can now write a script to make a GET request to the Copper API to retrieve company data. Below is an example of how to achieve this using JavaScript:
const fetch = require('node-fetch');
const crypto = require('crypto');
const apiKey = 'your_api_key';
const secret = 'your_api_secret';
const timestamp = Date.now().toString();
const method = 'GET';
const path = '/platform/companies';
const body = '';
const signature = crypto.createHmac('sha256', secret)
.update(timestamp + method + path + body)
.digest('hex');
fetch('https://api.copper.co/platform/companies', {
method: 'GET',
headers: {
'Authorization': `ApiKey ${apiKey}`,
'X-Signature': signature,
'X-Timestamp': timestamp
}
})
.then(response => response.json())
.then(data => console.log('Company Data:', data))
.catch(error => console.error('Error:', error));
Handling API Responses and Errors
When making API calls, it's crucial to handle responses and potential errors effectively. The example above logs the retrieved company data to the console. If an error occurs, it will be caught and logged, allowing you to troubleshoot any issues with your request.
Ensure that you check the response status and handle different HTTP status codes appropriately. For example, a 200 status code indicates success, while a 401 status code suggests an authentication issue.
Verifying Successful API Requests in Copper Dashboard
After executing your API call, you can verify the success of your request by checking the Copper dashboard. If your request was to retrieve data, ensure that the data returned matches the expected output. For any data creation or updates, confirm that the changes reflect in your Copper account.
By following these steps, you can efficiently interact with the Copper API using JavaScript, enabling seamless integration and data management within your applications.
Best Practices for Using Copper API in JavaScript
When working with the Copper API, it's essential to follow best practices to ensure secure and efficient integration. Here are some key recommendations:
- Securely Store API Credentials: Always keep your API key and secret secure. Avoid hardcoding them in your scripts; instead, use environment variables or secure vaults.
- Handle Rate Limiting: Copper API has rate limits to prevent abuse. Implement logic to handle HTTP 429 errors by pausing requests and retrying after a delay.
- Validate API Responses: Always check the response status and handle errors gracefully. Log errors for troubleshooting and ensure your application can recover from failures.
- Optimize Data Handling: When retrieving large datasets, consider implementing pagination to manage data efficiently and reduce load times.
Leveraging Endgrate for Seamless Copper API Integration
Integrating multiple APIs can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Copper. By using Endgrate, you can:
- Save Time and Resources: Focus on your core product while Endgrate handles the intricacies of API integrations.
- Build Once, Deploy Anywhere: Create a single integration for multiple platforms, reducing development overhead.
- Enhance User Experience: Provide your customers with a seamless integration experience, improving satisfaction and retention.
Visit Endgrate to learn more about how you can streamline your API integrations and focus on delivering value to your users.
Read More
Ready to get started?