How to Get Active Users Count with the Mode API in Python
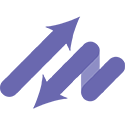
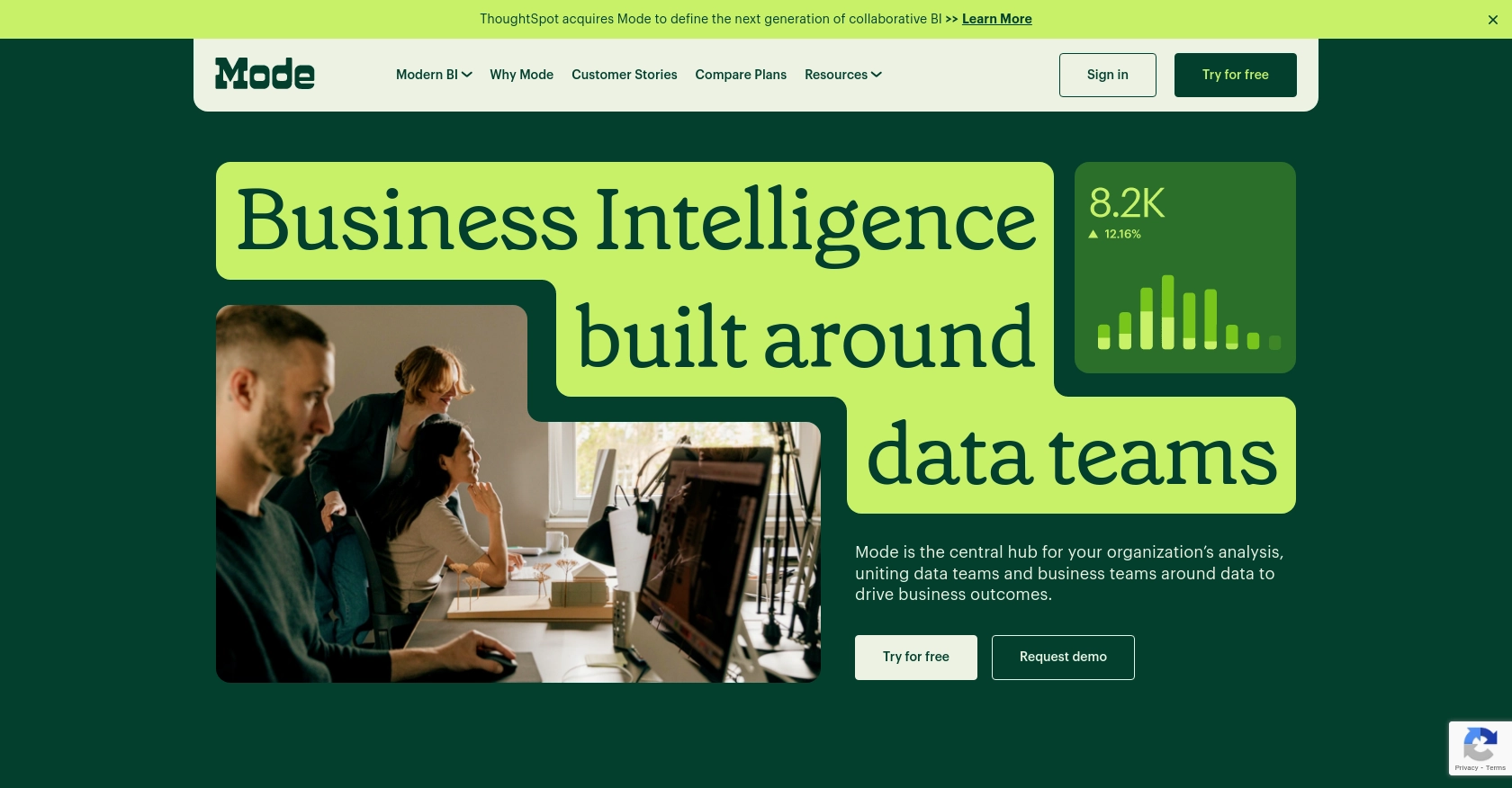
Introduction to Mode API for Active User Management
Mode is a collaborative analytics platform that empowers teams to make data-driven decisions by providing a comprehensive suite of tools for data analysis and visualization. With Mode, businesses can seamlessly integrate data workflows, enabling efficient collaboration and insightful reporting.
For developers, integrating with the Mode API offers a powerful way to programmatically access and manage analytics data. One common use case is retrieving the count of active users within a workspace. This can be particularly useful for monitoring user engagement and optimizing resource allocation.
In this article, we will explore how to use Python to interact with the Mode API to get the active users count. By following this guide, developers can efficiently integrate Mode's analytics capabilities into their applications, enhancing data-driven decision-making processes.
Setting Up Your Mode API Test Account for Active User Retrieval
Before you can interact with the Mode API to retrieve active user counts, you need to set up a Mode account and obtain the necessary API credentials. This section will guide you through creating a Mode account and generating an API access token, which is essential for authenticating your API requests.
Step 1: Create a Mode Account and Workspace
If you don't already have a Mode account, you can sign up for one by visiting the Mode website. Once registered, you'll need to create a Workspace, which is a collaborative environment where your team can work together on data analysis and reporting.
- Navigate to the Mode website and click on "Sign Up" to create a new account.
- Follow the prompts to set up your account and create a new Workspace.
- Once your Workspace is set up, you can start adding data sources or use public data for testing purposes.
Step 2: Generate a Mode API Access Token
To access the Mode API, you need an API access token. This token acts as a credential to authenticate your API requests. Follow these steps to create a Workspace API token:
- Log in to your Mode account and navigate to your Workspace Settings.
- Go to the "Privacy & Security" section and find the "API" tab.
- Click on the gear icon and select "Create new API token."
- Provide a display name for your token and save it securely. The token consists of a public component (username) and a private component (secret).
Note: Ensure that you store your API token and secret securely, as they are only displayed once during creation.
Step 3: Test Your API Access
With your API token ready, you can now test your access to the Mode API. Use the following Python code snippet to send a test request:
import requests
from requests.auth import HTTPBasicAuth
# Replace 'your_api_token' and 'your_api_secret' with your actual API credentials
username = 'your_api_token'
password = 'your_api_secret'
url = 'https://app.mode.com/api/account'
response = requests.get(url, auth=HTTPBasicAuth(username, password))
if response.status_code == 200:
print("API access successful!")
else:
print("Failed to access API:", response.status_code)
Run this code to verify that your API credentials are working correctly. A successful response indicates that you can proceed with making API calls to retrieve active user counts.
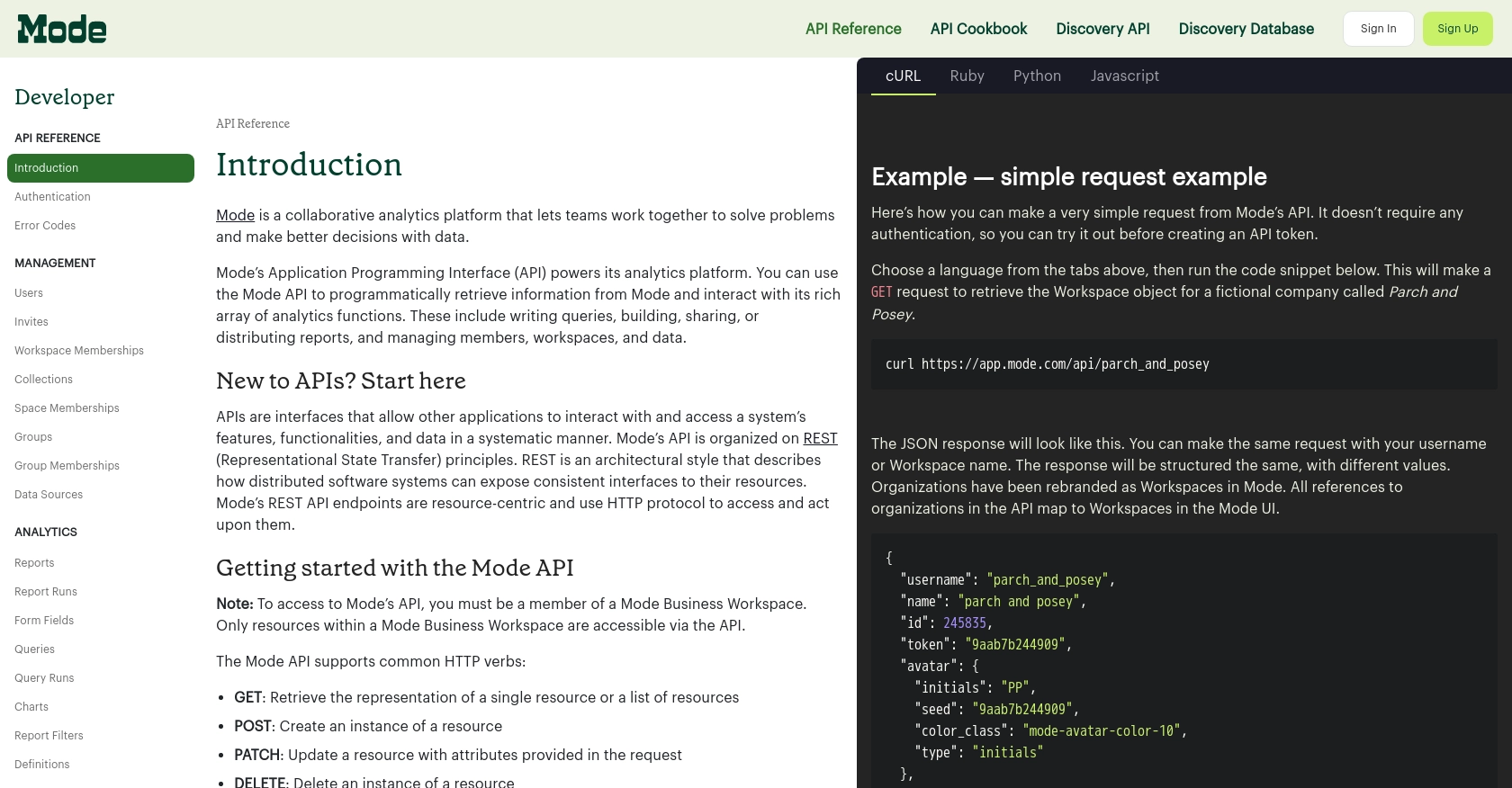
sbb-itb-96038d7
Making the Mode API Call to Retrieve Active Users Count
Now that you have your Mode API credentials set up, it's time to make the actual API call to retrieve the active users count. This section will guide you through the process of using Python to interact with the Mode API, ensuring you have the necessary tools and understanding to execute the call successfully.
Python Setup and Dependencies for Mode API Integration
Before making the API call, ensure you have Python installed on your machine. This guide uses Python 3.11.1, and you will need the requests
library to handle HTTP requests. If you haven't installed it yet, you can do so using pip:
pip install requests
Example Code to Get Active Users Count from Mode API
With the prerequisites in place, you can now write the Python code to interact with the Mode API. The following code snippet demonstrates how to retrieve the active users count from your Mode Workspace:
import requests
from requests.auth import HTTPBasicAuth
# Replace 'your_api_token' and 'your_api_secret' with your actual API credentials
username = 'your_api_token'
password = 'your_api_secret'
workspace = 'your_workspace_name'
url = f'https://app.mode.com/api/{workspace}/memberships'
response = requests.get(url, auth=HTTPBasicAuth(username, password))
if response.status_code == 200:
memberships = response.json()
active_users_count = sum(1 for member in memberships if member['state'] == 'active')
print(f"Active Users Count: {active_users_count}")
else:
print("Failed to retrieve active users:", response.status_code)
Replace your_api_token
, your_api_secret
, and your_workspace_name
with your actual API credentials and workspace name. This code sends a GET request to the Mode API to list all memberships in your workspace and counts the number of active users.
Verifying the API Request Success in Mode
After running the code, you should see the active users count printed in the console. To verify the request's success, you can cross-check the count with the data available in your Mode Workspace. If the count matches, your API call was successful.
Handling Errors and Understanding Mode API Error Codes
While making API calls, you might encounter errors. Mode uses standard HTTP response codes to indicate the success or failure of an API request. Here are some common error codes you might encounter:
- 400 Bad Request: The request was malformed or contained invalid parameters.
- 401 Unauthorized: Authentication failed. Check your API credentials.
- 403 Forbidden: You do not have permission to access the resource.
- 404 Not Found: The requested resource could not be found.
For more detailed information on error codes, refer to the Mode API Error Codes documentation.
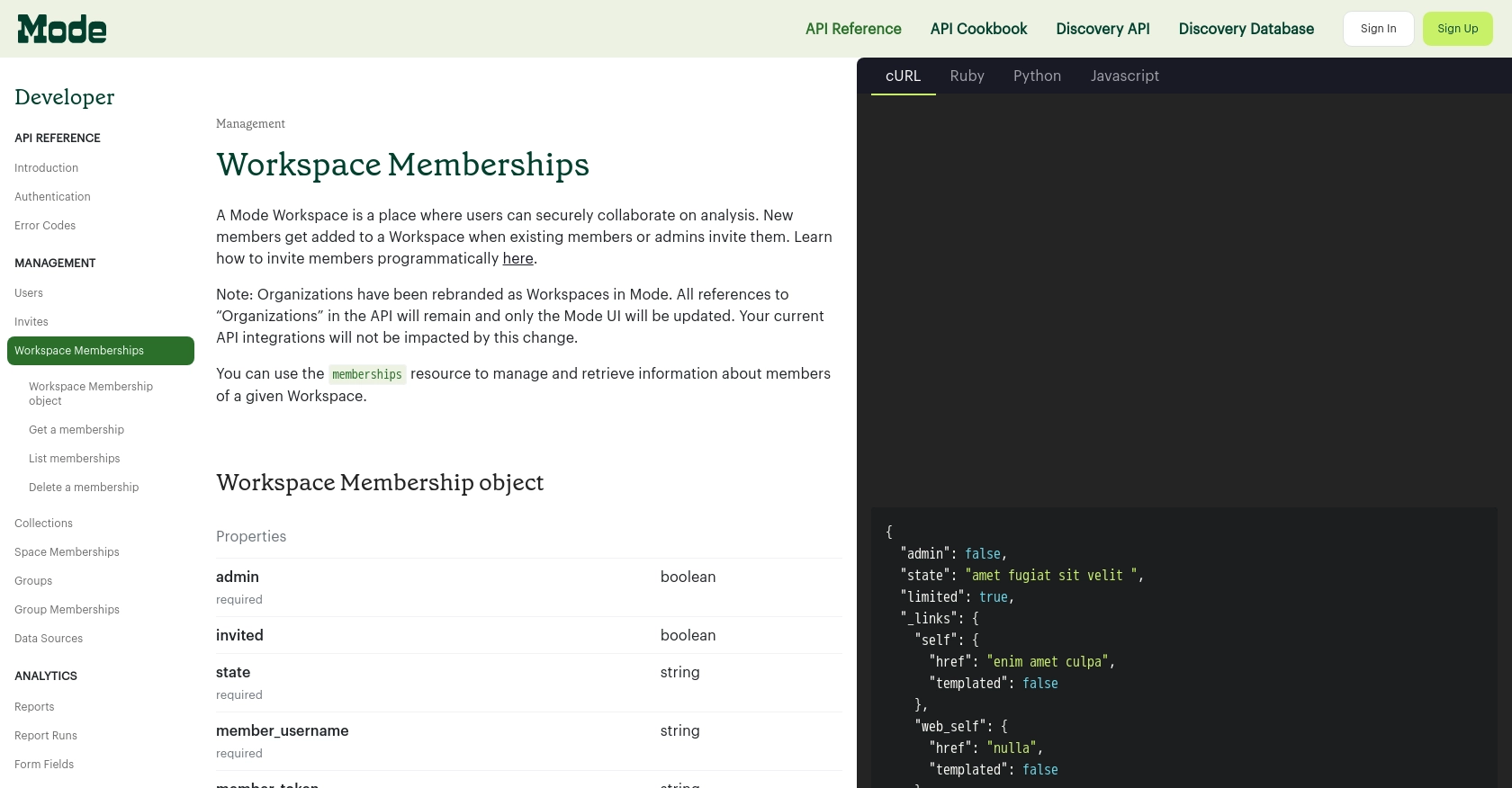
Conclusion and Best Practices for Using Mode API in Python
Integrating with the Mode API to retrieve active user counts can significantly enhance your ability to monitor user engagement and optimize resource allocation within your workspace. By following the steps outlined in this guide, you can seamlessly incorporate Mode's analytics capabilities into your applications, enabling data-driven decision-making.
Best Practices for Secure and Efficient Mode API Integration
- Secure Storage of API Credentials: Always store your API token and secret securely. Avoid exposing them in public repositories or client-side code.
- Handling Rate Limits: Be mindful of Mode's API rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Standardization: Ensure that data retrieved from the Mode API is standardized and transformed as needed for consistent integration with your systems.
Enhancing Integration Efficiency with Endgrate
While integrating with Mode's API provides powerful capabilities, managing multiple integrations can be complex and time-consuming. Endgrate offers a streamlined solution by providing a unified API endpoint that connects to various platforms, including Mode. This allows you to build once for each use case and avoid the hassle of maintaining multiple integrations.
By leveraging Endgrate, you can save time and resources, allowing your team to focus on core product development while delivering an intuitive integration experience for your customers. Explore how Endgrate can simplify your integration processes by visiting Endgrate's website.
Read More
Ready to get started?