How to Get Organizations with the Zendesk Support API in Javascript
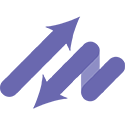
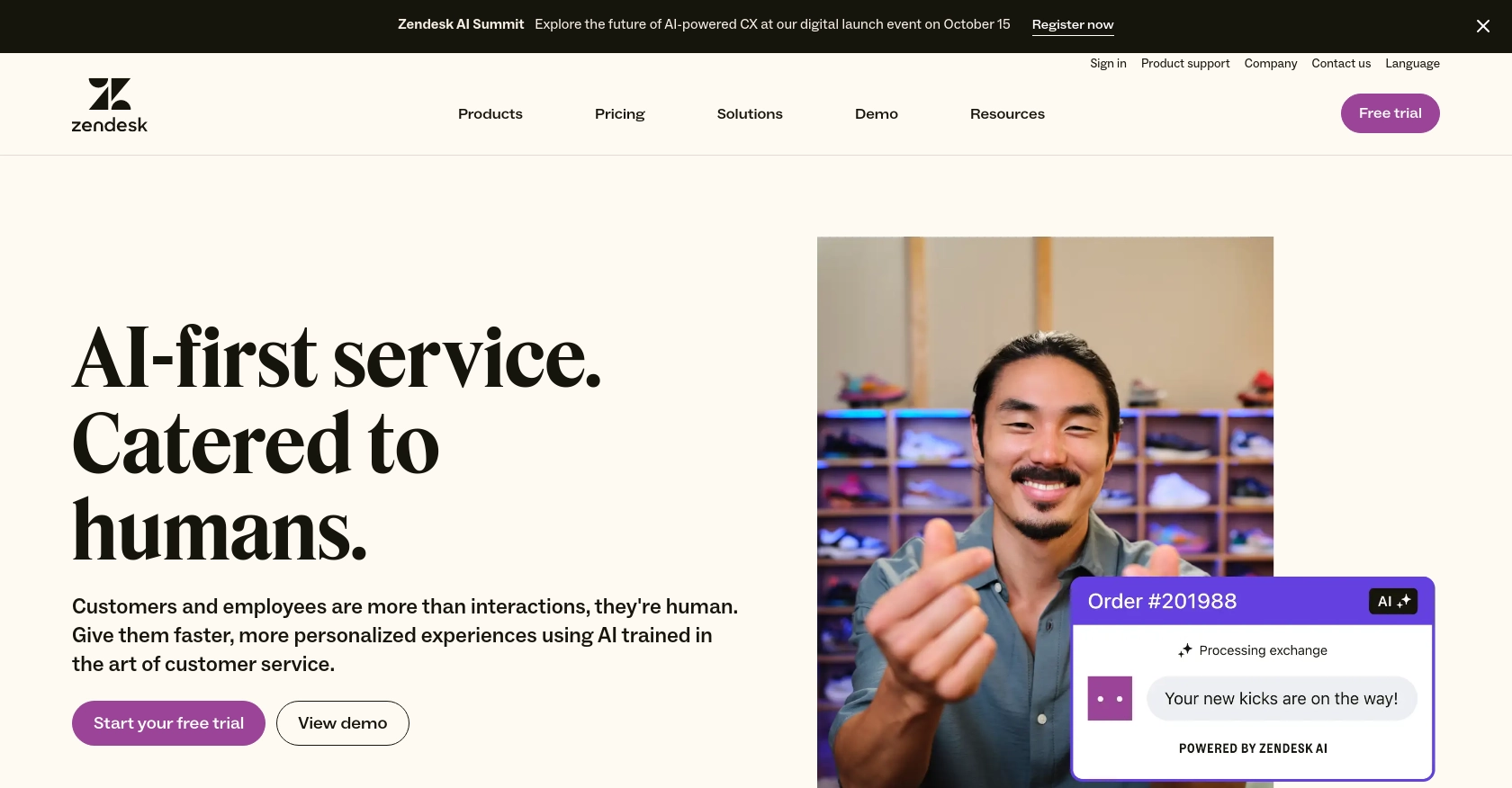
Introduction to Zendesk Support API
Zendesk Support is a powerful customer service platform that helps businesses manage and improve their customer interactions. It offers a suite of tools designed to streamline support processes, enhance customer satisfaction, and provide valuable insights into customer behavior.
Integrating with the Zendesk Support API allows developers to access and manage various resources, such as tickets, users, and organizations. This integration can be particularly useful for automating support workflows and enhancing customer data management. For example, developers might want to retrieve organization data to analyze customer segments or automate ticket routing based on organization-specific rules.
Setting Up Your Zendesk Support Test Account
Before you can start interacting with the Zendesk Support API, you'll need to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting your live data.
Creating a Zendesk Support Sandbox Account
If you don't already have a Zendesk account, you can sign up for a free trial or a sandbox account on the Zendesk website. This will give you access to the necessary tools and permissions to test API integrations.
- Visit the Zendesk registration page and follow the instructions to create your account.
- Once your account is set up, log in to the Zendesk Admin Center.
Registering Your Application for OAuth Authentication
Since the Zendesk API uses OAuth for authentication, you'll need to register your application to generate the necessary credentials.
- In the Admin Center, navigate to Apps and integrations > APIs > Zendesk API.
- Select the OAuth Clients tab and click on Add OAuth client.
- Fill in the required fields, such as Client Name, Description, and Redirect URLs.
- Click Save to generate your Client ID and Client Secret. Make sure to store these securely as they will be used for API authentication.
Configuring OAuth Scopes for Zendesk Support API
To access organization data, you'll need to configure the appropriate OAuth scopes:
- In the OAuth client settings, specify the scopes required for your application, such as
organizations:read
to read organization data. - Ensure that the scopes align with the API endpoints you plan to use.
Generating an OAuth Access Token
With your OAuth client set up, you can now generate an access token to authenticate your API requests:
- Direct users to the Zendesk authorization page using the URL format:
https://{subdomain}.zendesk.com/oauth/authorizations/new
. - After users grant access, handle the authorization code returned to your redirect URL.
- Exchange the authorization code for an access token by making a POST request to
https://{subdomain}.zendesk.com/oauth/tokens
.
For detailed steps on implementing the OAuth flow, refer to the Zendesk OAuth documentation.
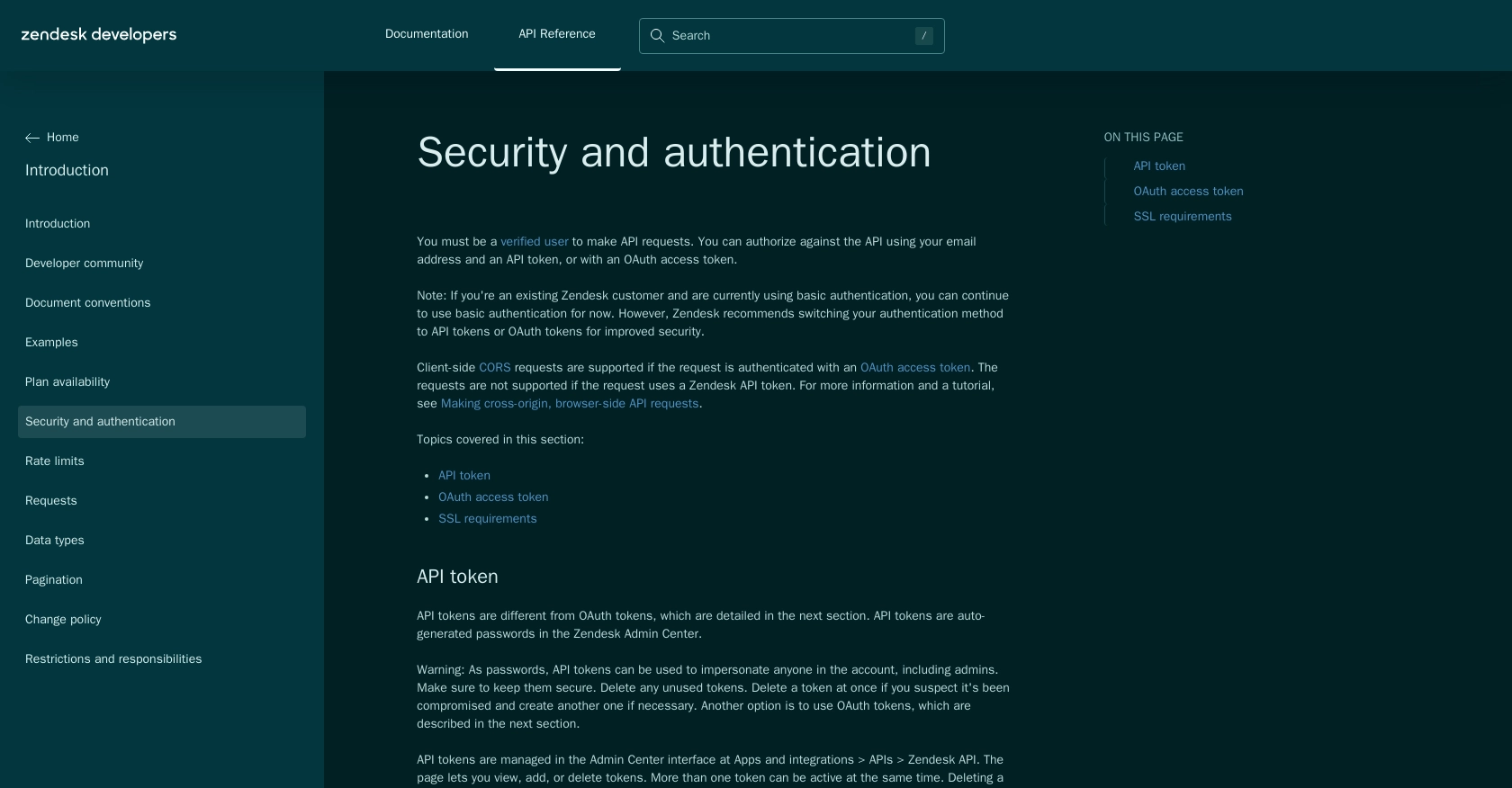
sbb-itb-96038d7
Making API Calls to Retrieve Organizations with Zendesk Support API in JavaScript
To interact with the Zendesk Support API and retrieve organization data using JavaScript, you need to ensure you have the right setup and dependencies. This section will guide you through the process of making API calls, handling responses, and managing potential errors.
Setting Up Your JavaScript Environment for Zendesk Support API
Before making API calls, ensure you have Node.js installed on your machine. Node.js provides the runtime environment necessary for executing JavaScript code outside a web browser.
- Download and install Node.js from the official website.
- Initialize a new Node.js project by running
npm init -y
in your project directory. - Install the Axios library for making HTTP requests by executing
npm install axios
.
Writing JavaScript Code to Fetch Organizations from Zendesk Support API
With your environment set up, you can now write the JavaScript code to make a GET request to the Zendesk Support API to retrieve organization data.
const axios = require('axios');
// Define the API endpoint and headers
const endpoint = 'https://{subdomain}.zendesk.com/api/v2/organizations.json';
const headers = {
'Content-Type': 'application/json',
'Authorization': 'Bearer {access_token}' // Replace with your actual access token
};
// Function to fetch organizations
async function fetchOrganizations() {
try {
const response = await axios.get(endpoint, { headers });
console.log('Organizations:', response.data.organizations);
} catch (error) {
console.error('Error fetching organizations:', error.response ? error.response.data : error.message);
}
}
// Call the function
fetchOrganizations();
Replace {subdomain}
with your Zendesk subdomain and {access_token}
with the OAuth access token you generated earlier.
Understanding the API Response and Handling Errors
When you make the API call, the response will contain the organization data in JSON format. You can access this data through the response.data.organizations
property. If the request is successful, you should see a list of organizations printed in the console.
In case of errors, the catch block will handle them by logging the error message. Common error codes include:
- 401 Unauthorized: Check if your access token is valid and not expired.
- 403 Forbidden: Ensure your OAuth scopes are correctly configured to access organization data.
- 429 Too Many Requests: You have exceeded the rate limit. Refer to the Zendesk rate limits documentation for more details.
Verifying API Call Success in Zendesk Support Sandbox
After executing the code, verify the success of your API call by checking the organizations listed in your Zendesk Support sandbox account. The data returned by the API should match the organizations present in your account.
For further information on handling API responses and errors, refer to the Zendesk API documentation.
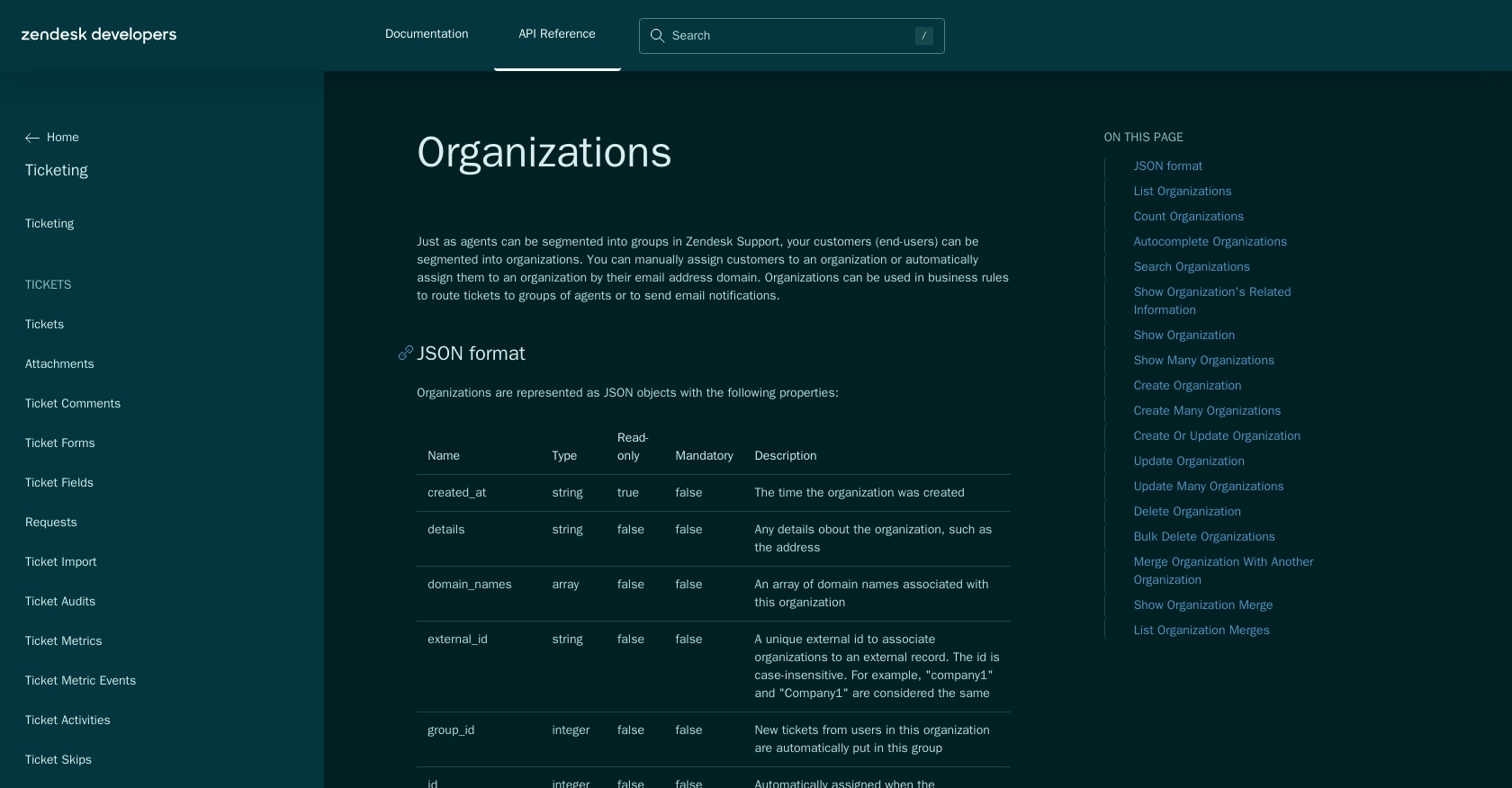
Conclusion and Best Practices for Using Zendesk Support API with JavaScript
Integrating with the Zendesk Support API using JavaScript provides a powerful way to manage and automate customer support processes. By retrieving organization data, developers can enhance customer segmentation, streamline ticket routing, and improve overall support efficiency.
Best Practices for Managing Zendesk Support API Integrations
- Securely Store Credentials: Ensure that your OAuth credentials, such as the Client ID and Client Secret, are stored securely and not exposed in your codebase.
- Handle Rate Limiting: Be mindful of the rate limits imposed by Zendesk. Implement logic to handle
429 Too Many Requests
errors by respecting theRetry-After
header. For more details, refer to the Zendesk rate limits documentation. - Optimize Data Handling: Consider transforming and standardizing data fields to match your application's requirements, ensuring seamless integration with other systems.
- Monitor API Usage: Regularly monitor your API usage to ensure compliance with Zendesk's limits and to optimize performance.
Enhance Your Integration Experience with Endgrate
While building integrations with the Zendesk Support API can be rewarding, it can also be time-consuming and complex. Endgrate offers a solution to streamline this process by providing a unified API endpoint that connects to multiple platforms, including Zendesk Support. By leveraging Endgrate, you can focus on your core product while outsourcing integration complexities.
Explore how Endgrate can simplify your integration needs by visiting Endgrate's website. Save time and resources, and provide an intuitive integration experience for your customers.
Read More
- https://endgrate.com/provider/zendesksupport
- https://developer.zendesk.com/api-reference/introduction/security-and-auth/
- https://support.zendesk.com/hc/en-us/articles/4408845965210-Using-OAuth-authentication-with-your-application
- https://developer.zendesk.com/api-reference/introduction/rate-limits/
- https://developer.zendesk.com/api-reference/introduction/pagination/
- https://developer.zendesk.com/api-reference/ticketing/organizations/organizations/
Ready to get started?