Using the Monday.com API to Create Board Items (with PHP examples)
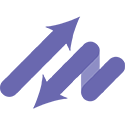
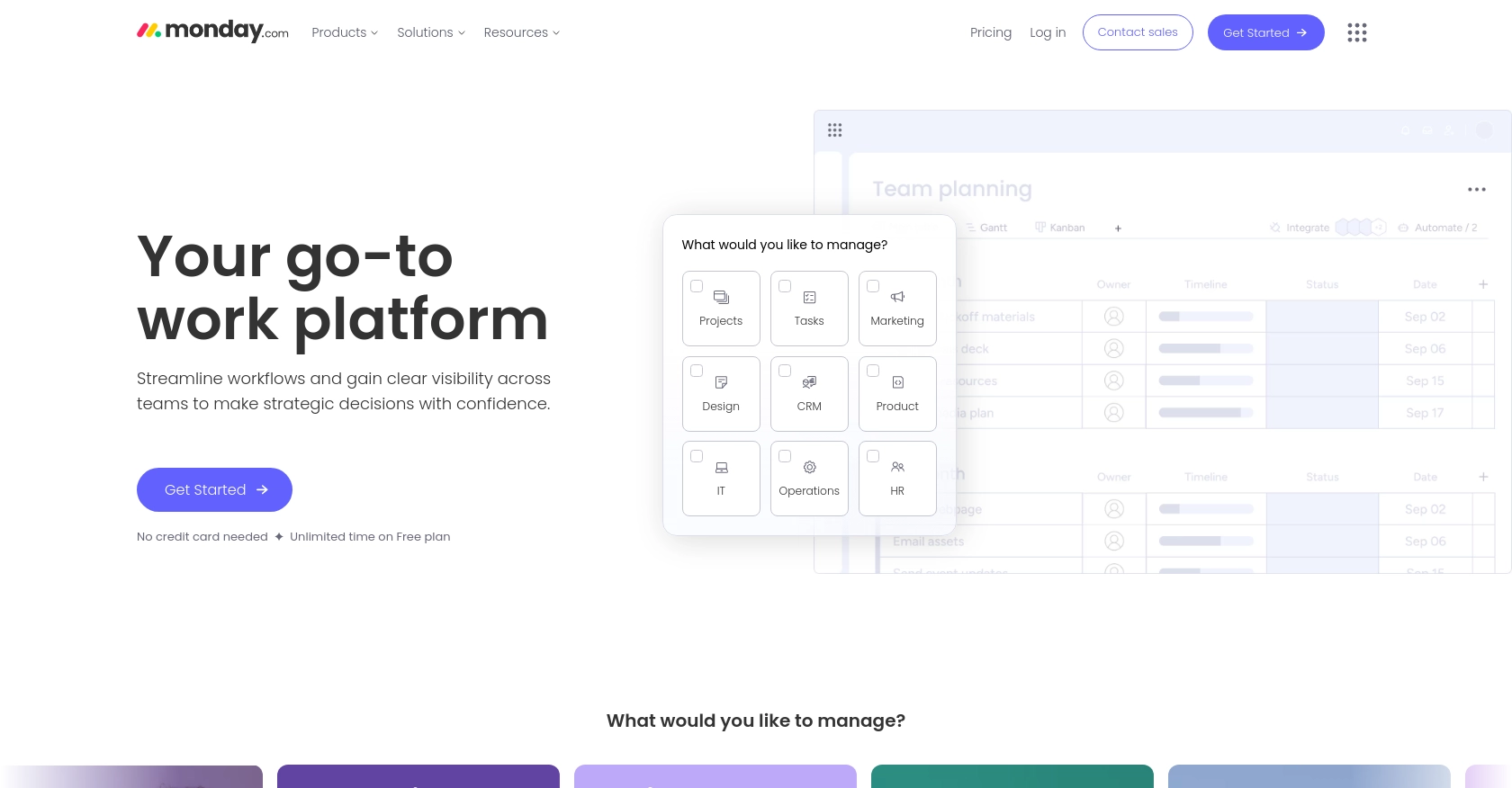
Introduction to Monday.com API Integration
Monday.com is a versatile work operating system that empowers teams to run projects and workflows with confidence. Its intuitive interface and customizable features make it a popular choice for businesses looking to enhance productivity and collaboration.
Developers often seek to integrate with Monday.com's API to automate and streamline tasks, such as managing board items. For example, you might want to create new board items programmatically to keep track of tasks or projects, ensuring that your team stays organized and efficient.
This article will guide you through using PHP to interact with the Monday.com API, specifically focusing on creating board items. By following this tutorial, you'll learn how to leverage Monday.com's API to enhance your workflow automation.
Setting Up Your Monday.com Account for API Integration
Before you can start creating board items using the Monday.com API, you'll need to set up your account and obtain the necessary API token. This process involves creating a Monday.com account and accessing your API token, which will be used to authenticate your requests.
Create a Monday.com Account
If you don't already have a Monday.com account, you can sign up for a free trial or use the free version available on the Monday.com website. Follow these steps to get started:
- Visit the Monday.com website and click on the "Get Started" button.
- Fill in the required information to create your account.
- Once your account is set up, log in to access the Monday.com dashboard.
Accessing Your Monday.com API Token
To interact with the Monday.com API, you'll need a personal API token. This token is used to authenticate your API requests. Follow these steps to obtain your token:
- Log into your Monday.com account.
- Click on your avatar/profile picture in the top right corner of the dashboard.
- Select Administration > Connections > API if you're an admin, or Developers > My Access Tokens if you're a member user.
- Copy your personal API token. Keep it secure, as it grants access to your account's API capabilities.
For more details on accessing API tokens, refer to the Monday.com authentication documentation.
Using the API Token for Authentication
Once you have your API token, you can authenticate your requests by including it in the Authorization header. Here's an example of how to set this up in PHP:
$apiToken = 'YOUR_API_TOKEN';
$headers = [
'Content-Type: application/json',
'Authorization: ' . $apiToken
];
Replace YOUR_API_TOKEN
with the token you copied earlier. This setup will allow you to make authenticated requests to the Monday.com API.
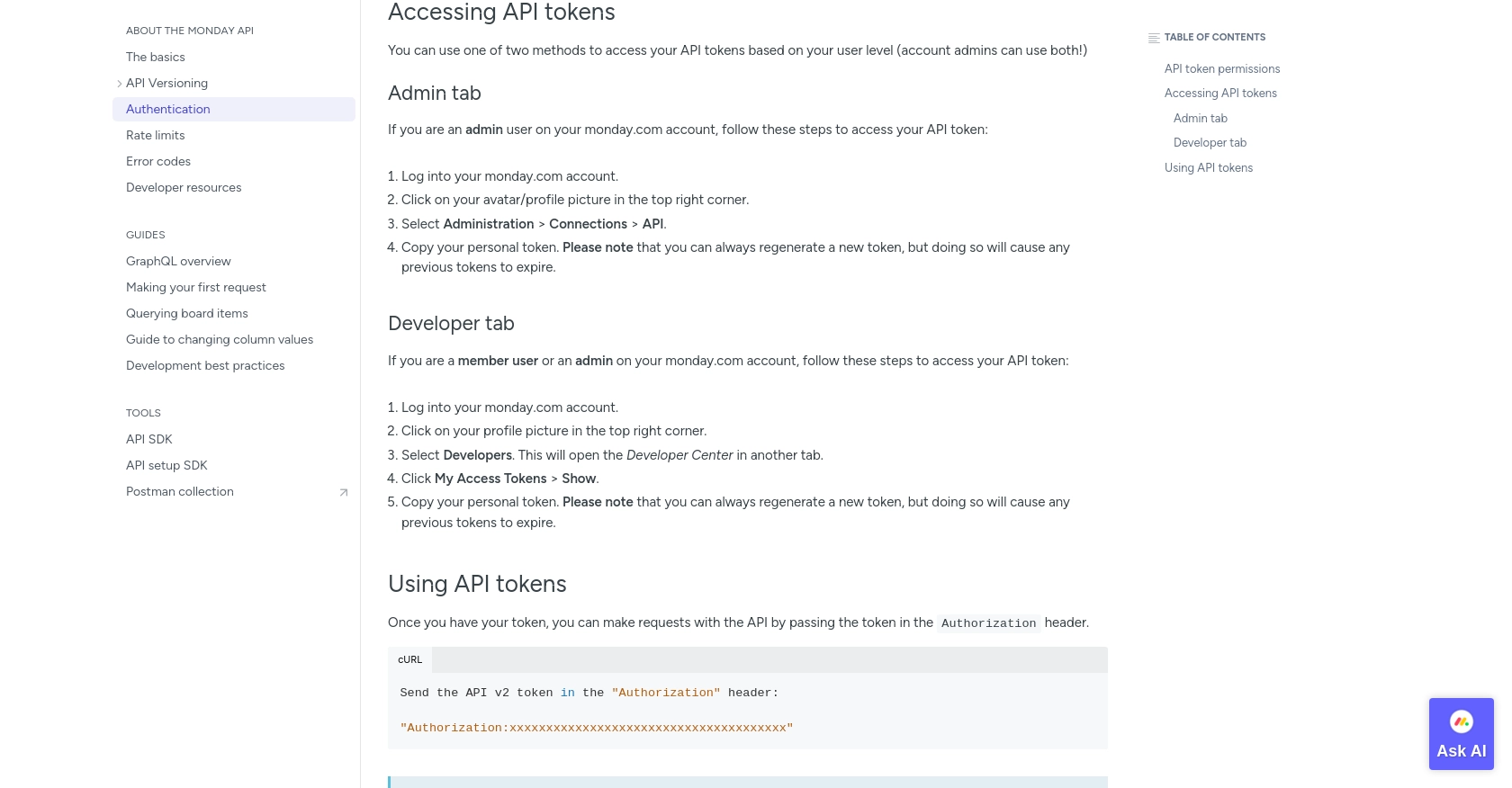
sbb-itb-96038d7
Making API Calls to Create Board Items on Monday.com Using PHP
To interact with the Monday.com API and create board items, you'll need to set up your PHP environment and make the necessary API calls. This section will guide you through the process, including setting up PHP, installing dependencies, and executing the API call to create board items.
Setting Up PHP Environment for Monday.com API Integration
Before making API calls, ensure you have the following prerequisites installed on your machine:
- PHP 7.4 or later
- Composer, the PHP package manager
Once you have these installed, open your terminal and install the Guzzle HTTP client, which will be used to make HTTP requests:
composer require guzzlehttp/guzzle
Creating a New Board Item on Monday.com
Now that your environment is set up, you can proceed to create a new board item using the Monday.com API. Follow these steps to write the PHP code for this task:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$apiToken = 'YOUR_API_TOKEN';
$boardId = 'YOUR_BOARD_ID';
$groupId = 'YOUR_GROUP_ID';
$itemName = 'New Task';
$query = 'mutation {
create_item (board_id: ' . $boardId . ', group_id: "' . $groupId . '", item_name: "' . $itemName . '") {
id
}
}';
$response = $client->post('https://api.monday.com/v2', [
'headers' => [
'Content-Type' => 'application/json',
'Authorization' => $apiToken
],
'body' => json_encode(['query' => $query])
]);
$result = json_decode($response->getBody(), true);
echo "Created Item ID: " . $result['data']['create_item']['id'];
Replace YOUR_API_TOKEN
, YOUR_BOARD_ID
, and YOUR_GROUP_ID
with your actual API token, board ID, and group ID, respectively. This script will create a new item on the specified board and group, and output the ID of the created item.
Verifying the API Request and Handling Errors
After running the script, you should verify that the item was successfully created by checking your Monday.com board. If the item appears, the request was successful.
In case of errors, the Monday.com API provides detailed error messages. Here are some common error codes and their meanings:
- 401 Unauthorized: Ensure your API token is valid and included in the request header.
- 429 Rate Limit Exceeded: Reduce the number of requests sent per minute. The limit is 5,000 requests per minute.
- 500 Internal Server Error: Check for invalid arguments or malformed JSON.
For more details on error handling, refer to the Monday.com error codes documentation.
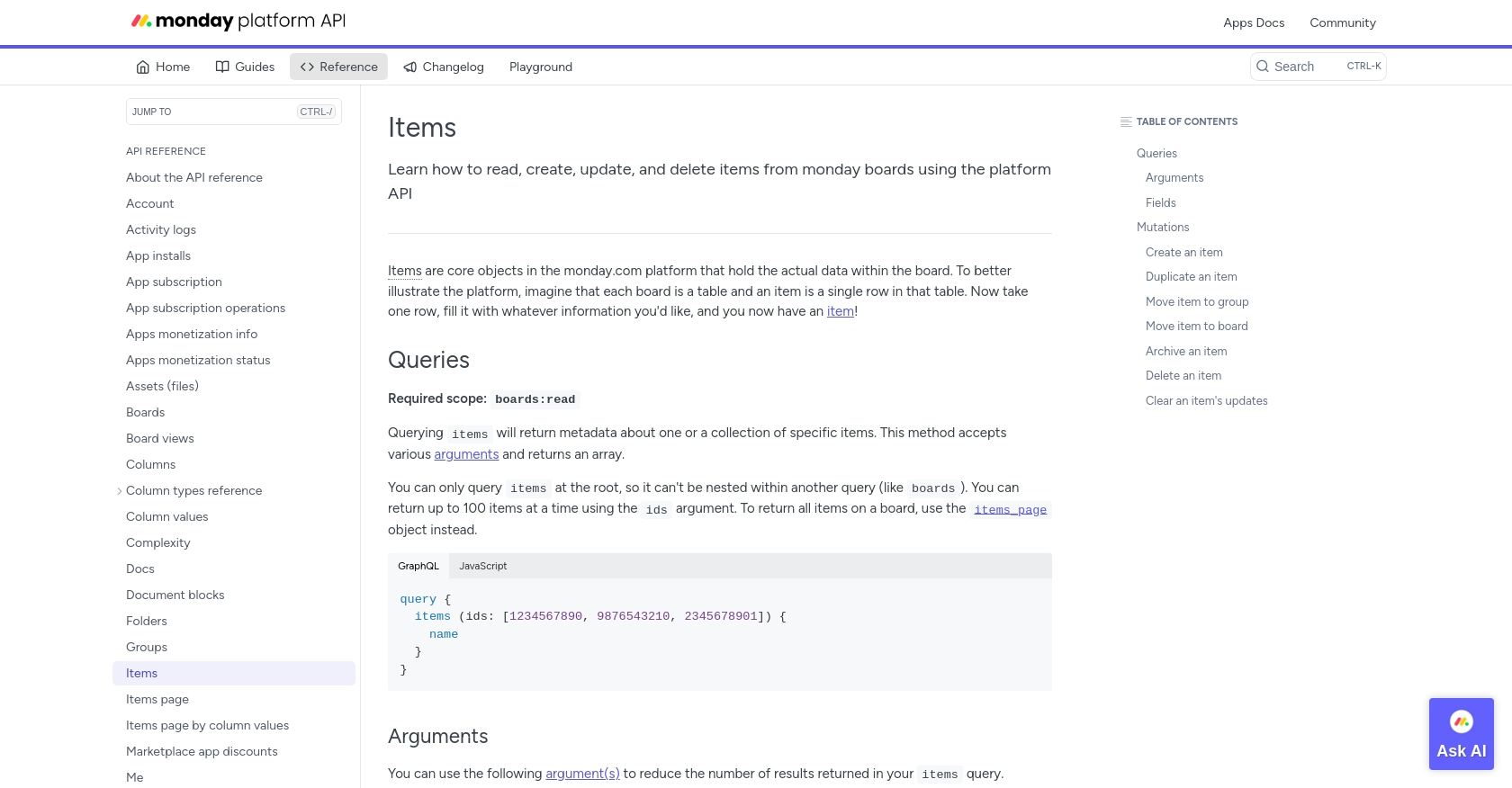
Conclusion and Best Practices for Monday.com API Integration
Integrating with the Monday.com API to create board items using PHP can significantly enhance your team's workflow automation and productivity. By following the steps outlined in this article, you can seamlessly create new tasks or projects programmatically, ensuring that your team remains organized and efficient.
Best Practices for Secure and Efficient Monday.com API Usage
- Secure Storage of API Tokens: Always store your API tokens securely, such as in environment variables or a secure vault, to prevent unauthorized access.
- Handling Rate Limits: Be mindful of the rate limits imposed by Monday.com. The API allows up to 5,000 requests per minute. Implementing retry mechanisms and optimizing your queries can help you stay within these limits. For more details, refer to the Monday.com rate limits documentation.
- Error Handling: Implement robust error handling in your code to gracefully manage API errors. Understanding common error codes, such as 401 Unauthorized and 429 Rate Limit Exceeded, will help you troubleshoot issues effectively.
- Data Standardization: Ensure that the data you send to Monday.com is standardized and formatted correctly to avoid errors and maintain data integrity.
Streamlining Integrations with Endgrate
If managing multiple integrations is becoming a challenge, consider using Endgrate to simplify the process. Endgrate provides a unified API endpoint that connects to various platforms, including Monday.com, allowing you to build once for each use case instead of multiple times for different integrations.
By leveraging Endgrate, you can save time and resources, focus on your core product, and offer an intuitive integration experience for your customers. Visit Endgrate to learn more about how it can streamline your integration efforts.
Read More
Ready to get started?