How to Create Sales Invoices with the Sage Accounting API in PHP
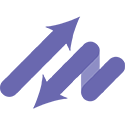
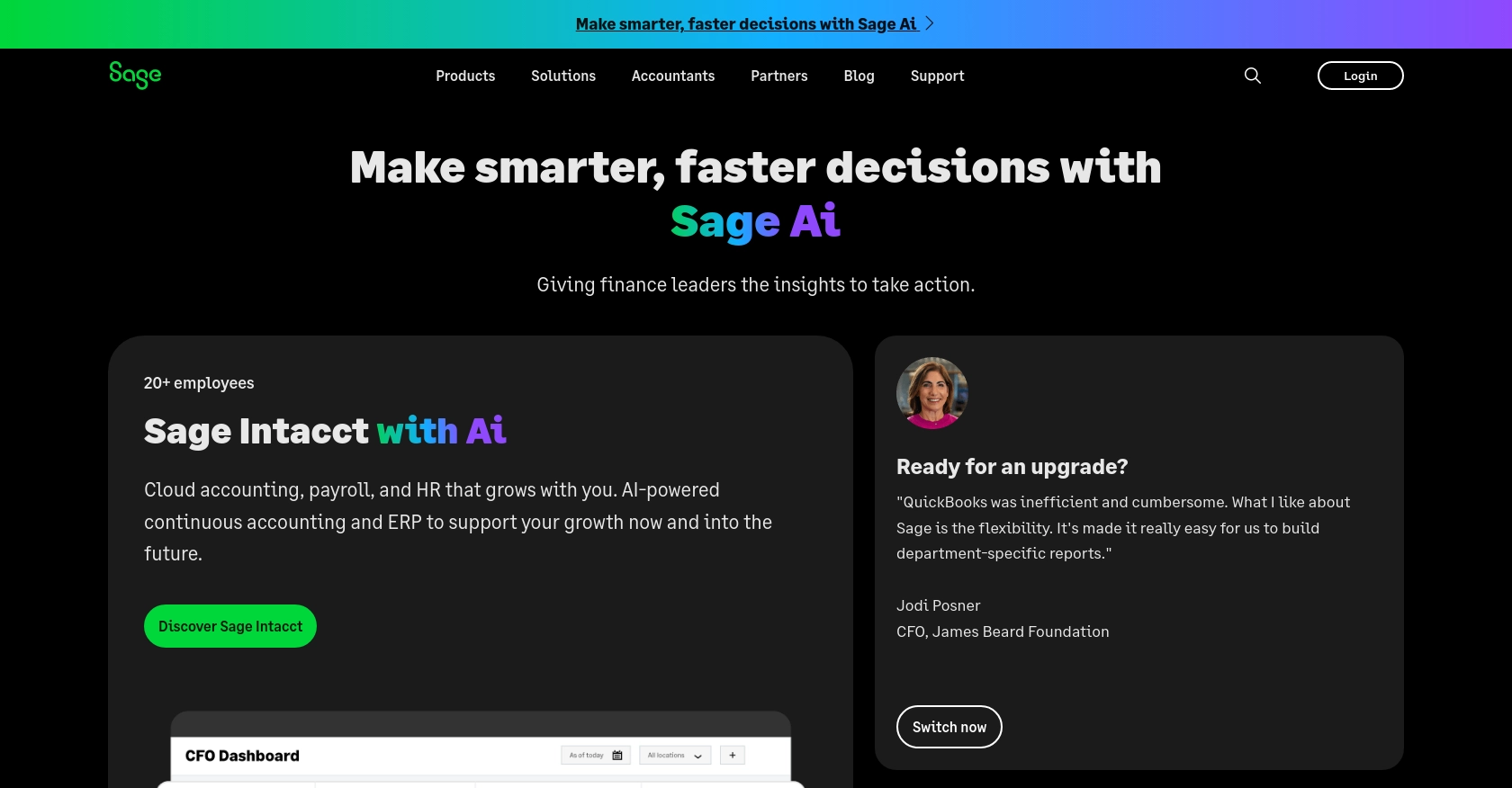
Introduction to Sage Accounting API
Sage Accounting is a robust cloud-based accounting solution tailored for small to medium-sized businesses. It offers a comprehensive suite of tools that streamline financial management, including invoicing, expense tracking, and reporting. Sage Accounting's flexibility and user-friendly interface make it a preferred choice for businesses looking to enhance their financial operations.
Integrating with the Sage Accounting API allows developers to automate and manage financial transactions seamlessly. For example, creating sales invoices programmatically can save time and reduce errors, enabling businesses to maintain accurate financial records effortlessly. By leveraging the Sage Accounting API, developers can build custom solutions that cater to specific business needs, enhancing operational efficiency.
Setting Up a Test or Sandbox Account for Sage Accounting API
Before you begin integrating with the Sage Accounting API, it's essential to set up a test or sandbox account. This environment allows you to experiment and test your integration without affecting live data, ensuring a smooth development process.
Step 1: Sign Up for a Sage Developer Account
To access the Sage Accounting API, you need a Sage Developer account. Follow these steps to create one:
- Visit the Sage Developer Portal.
- Sign up using your GitHub account or an email address.
- Once registered, you can manage your applications and obtain client credentials.
Step 2: Create a Trial Business Account
Next, set up a trial business account to use as your sandbox environment:
- Navigate to the trial business setup page.
- Select the appropriate region and subscription tier that matches your integration needs.
- Complete the sign-up process to create your trial business account.
Step 3: Register Your Application
With your developer account ready, you can now register your application:
- Log in to your Sage Developer account.
- Click on "Create App" and provide the necessary details, including a name and callback URL.
- Save your application to generate the Client ID and Client Secret, which are crucial for OAuth authentication.
- For more details, refer to the Create an App guide.
Step 4: Upgrade to a Developer Account
To fully utilize the sandbox environment, upgrade your account to a developer account:
- Submit a request through the upgrade form.
- Provide your trial account details, including your name, email, app name, and Client ID.
- Wait for confirmation, which typically takes 3-5 working days.
Once these steps are completed, you'll have a fully functional sandbox environment to test your Sage Accounting API integration.
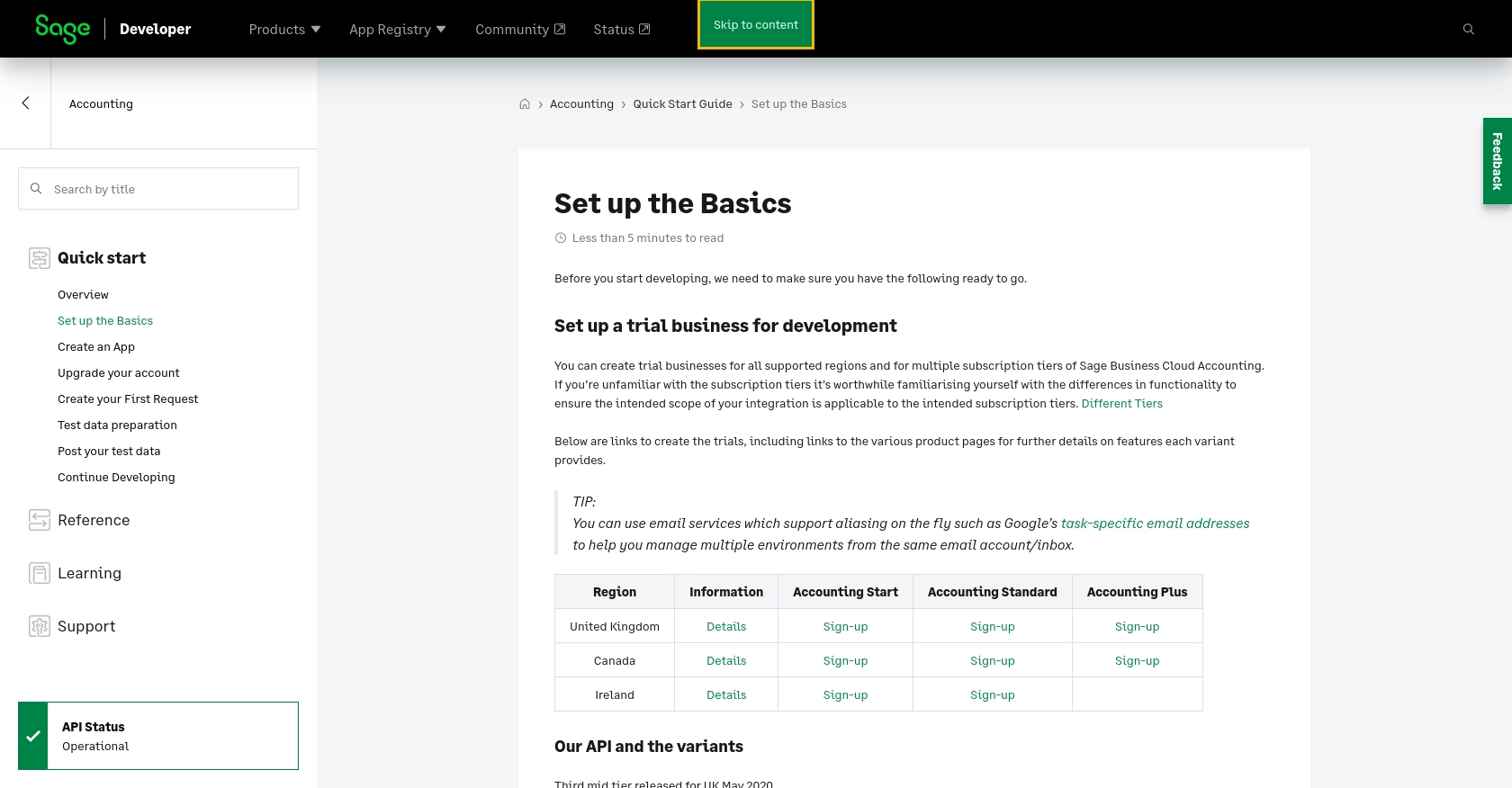
sbb-itb-96038d7
How to Make API Calls to Create Sales Invoices with Sage Accounting API in PHP
Prerequisites for Using PHP with Sage Accounting API
Before diving into the code, ensure you have the following prerequisites set up on your development environment:
- PHP 7.4 or higher installed on your machine.
- Composer for managing PHP dependencies.
- The
guzzlehttp/guzzle
package for making HTTP requests. Install it using Composer:
composer require guzzlehttp/guzzle
Setting Up OAuth Authentication for Sage Accounting API
To interact with the Sage Accounting API, you need to authenticate using OAuth. Follow these steps to set up authentication:
- Use the Client ID and Client Secret obtained during app registration.
- Implement the OAuth 2.0 flow to obtain an access token.
- Store the access token securely for use in API requests.
Creating a Sales Invoice with Sage Accounting API
Once authentication is set up, you can proceed to create a sales invoice. Below is a step-by-step guide to making the API call using PHP:
Step 1: Define the API Endpoint and Headers
Set the API endpoint for creating sales invoices and prepare the necessary headers:
$endpoint = "https://api.sage.com/accounting/v3.1/sales_invoices";
$headers = [
'Authorization' => 'Bearer YOUR_ACCESS_TOKEN',
'Content-Type' => 'application/json'
];
Step 2: Prepare the Invoice Data
Create an array with the invoice details you want to send to the API:
$invoiceData = [
'contact_id' => 'CONTACT_ID',
'date' => '2023-10-01',
'due_date' => '2023-10-15',
'line_items' => [
[
'description' => 'Product Description',
'quantity' => 1,
'unit_price' => 100.00
]
]
];
Step 3: Make the API Call
Use Guzzle to send a POST request to the Sage Accounting API:
use GuzzleHttp\Client;
$client = new Client();
$response = $client->post($endpoint, [
'headers' => $headers,
'json' => $invoiceData
]);
if ($response->getStatusCode() == 201) {
echo "Invoice Created Successfully";
} else {
echo "Failed to Create Invoice: " . $response->getBody();
}
Step 4: Verify the Invoice Creation
Check your Sage Accounting sandbox account to ensure the invoice appears as expected. If the request was successful, the invoice should be listed in your sales invoices.
Handling Errors and Troubleshooting
When making API calls, it's crucial to handle potential errors. The Sage Accounting API may return various error codes, such as:
- 400 Bad Request: The request was invalid. Check your data format and required fields.
- 401 Unauthorized: Authentication failed. Verify your access token.
- 500 Internal Server Error: An error occurred on the server. Try again later.
For more detailed error handling, refer to the Sage Accounting API documentation.
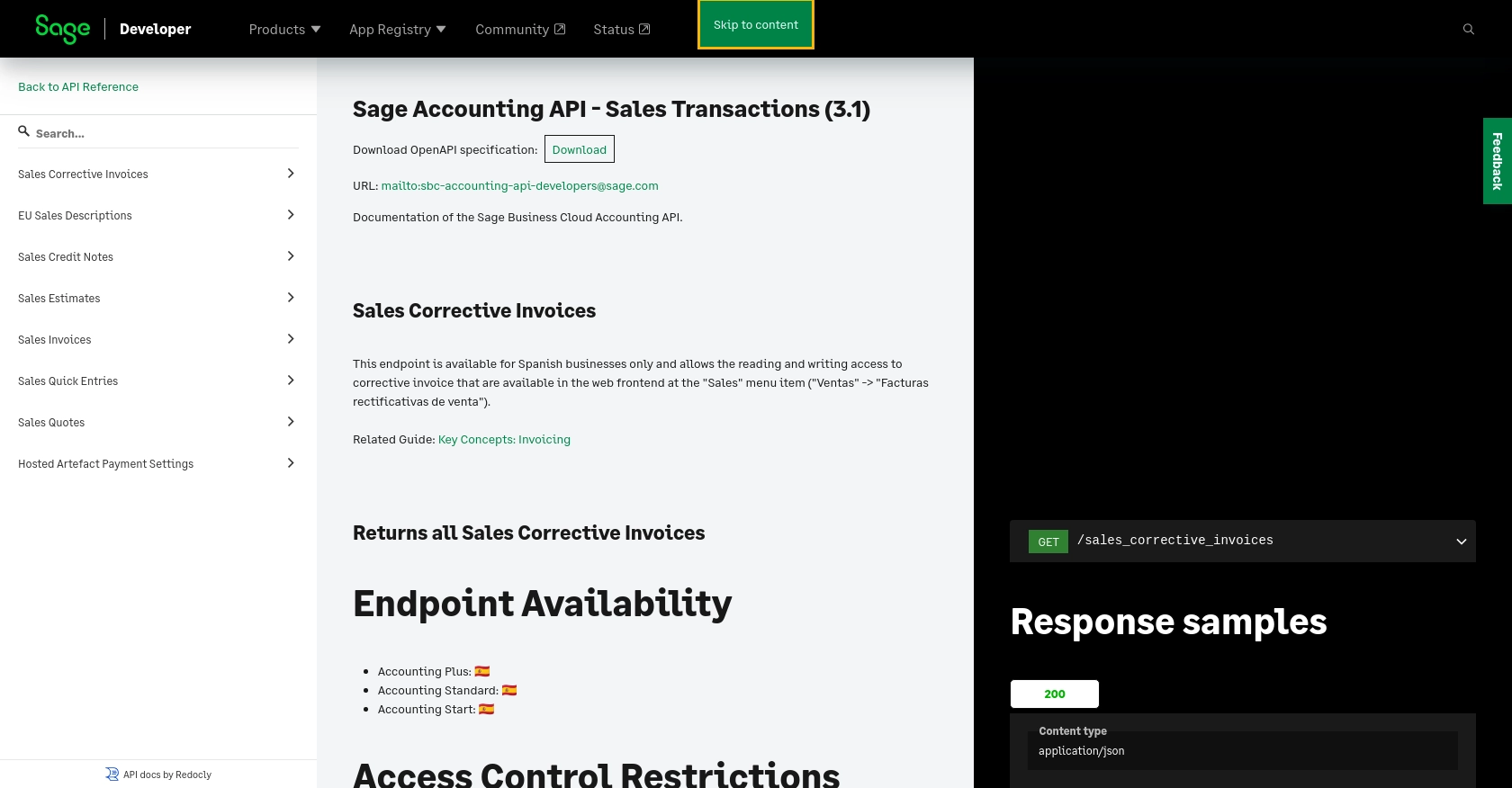
Conclusion and Best Practices for Integrating with Sage Accounting API
Integrating with the Sage Accounting API using PHP can significantly enhance your business's financial operations by automating tasks such as creating sales invoices. By following the steps outlined in this guide, you can streamline your invoicing process, reduce manual errors, and improve efficiency.
Best Practices for Secure and Efficient API Integration
- Securely Store Credentials: Always store your Client ID, Client Secret, and access tokens securely. Consider using environment variables or a secure vault to protect sensitive information.
- Handle Rate Limiting: Be aware of any rate limits imposed by the Sage Accounting API to avoid exceeding them. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Standardize Data Fields: Ensure that your data fields are consistent and standardized across your application to prevent discrepancies and errors during API interactions.
- Monitor API Usage: Regularly monitor your API usage and logs to detect any unusual activity or errors. This helps in maintaining the health and performance of your integration.
Leverage Endgrate for Simplified Integration Management
For businesses looking to streamline their integration processes further, consider using Endgrate. Endgrate offers a unified API endpoint that connects to multiple platforms, including Sage Accounting, allowing you to manage integrations more efficiently. By outsourcing integrations to Endgrate, you can focus on your core product development and provide a seamless integration experience for your customers.
Visit Endgrate to learn more about how you can save time and resources by simplifying your integration management.
Read More
- https://endgrate.com/provider/sage
- https://developer.sage.com/accounting/quick-start/set-up-the-basics/
- https://developer.sage.com/accounting/quick-start/create-an-app/
- https://developer.sage.com/accounting/quick-start/upgrade-your-account/
- https://developer.sage.com/accounting/reference/invoicing-sales/
Ready to get started?