Using the Sugar Market API to Create Or Update Accounts (with Python examples)
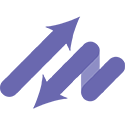
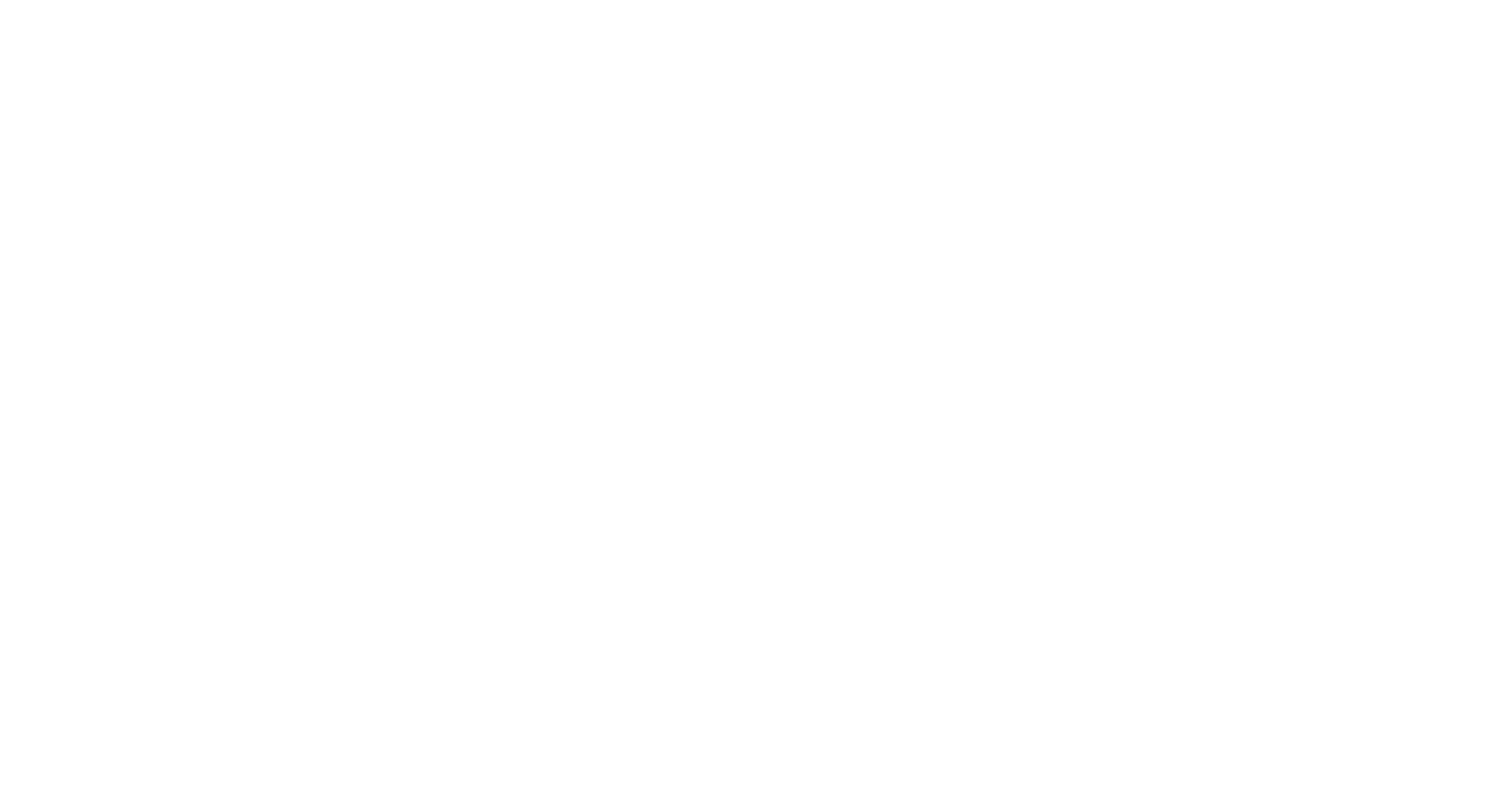
Introduction to Sugar Market API Integration
Sugar Market is a powerful marketing automation platform that helps businesses streamline their marketing efforts by providing tools for campaign management, lead nurturing, and analytics. Its robust features and user-friendly interface make it a preferred choice for companies looking to enhance their marketing strategies.
Developers may want to integrate with the Sugar Market API to automate and optimize marketing processes, such as creating or updating account information. For example, a developer could use the Sugar Market API to synchronize account data between Sugar Market and other CRM systems, ensuring that all platforms have the most up-to-date information.
Setting Up Your Sugar Market Test/Sandbox Account
Before you can start integrating with the Sugar Market API, you need to set up a test or sandbox account. This allows you to safely experiment with API calls without affecting live data.
Creating a Sugar Market Account
If you don't already have a Sugar Market account, you can sign up for a free trial or request access to a sandbox environment through the Sugar Market website. This will give you the necessary credentials to access the API.
Generating API Credentials for Sugar Market
Once your account is set up, you'll need to generate API credentials to authenticate your requests. Sugar Market uses a custom authentication method, so follow these steps to obtain your credentials:
- Log in to your Sugar Market account.
- Navigate to the API settings section, typically found under the developer or integrations tab.
- Follow the prompts to create a new API application. This process will provide you with a client ID and client secret.
- Store these credentials securely, as you'll need them to authenticate your API requests.
Configuring OAuth for Sugar Market API
If your integration requires OAuth-based authentication, you'll need to configure an OAuth app within your Sugar Market account:
- In the API settings, select the option to create an OAuth app.
- Provide the necessary details, such as the app name and redirect URI.
- Once created, note the OAuth client ID and client secret.
- Use these credentials to obtain an access token for API calls.
For more detailed instructions, refer to the Sugar Market API documentation.
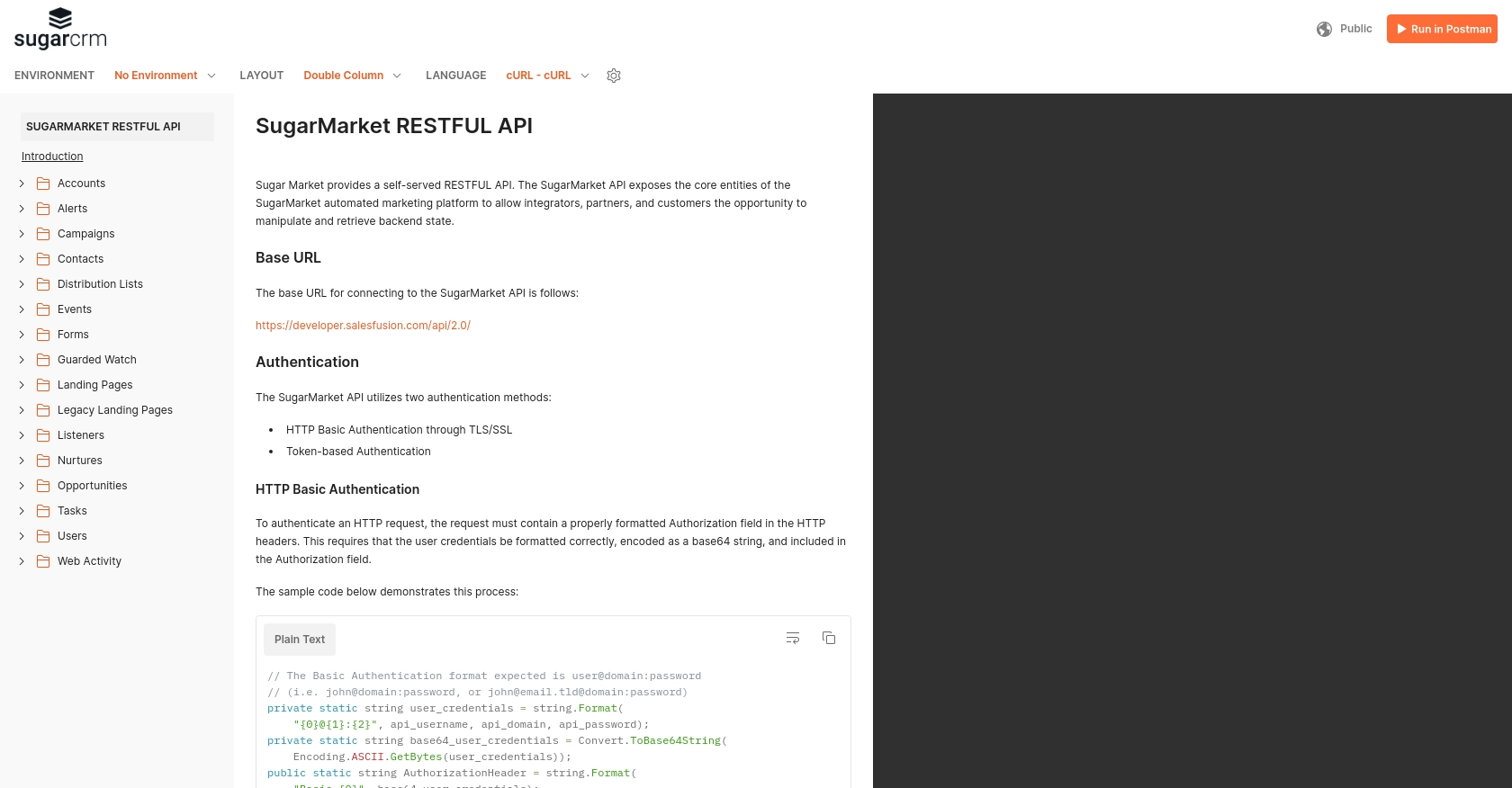
sbb-itb-96038d7
Making API Calls to Sugar Market with Python
To interact with the Sugar Market API using Python, you'll need to ensure you have the correct environment set up. This section will guide you through the necessary steps to make API calls for creating or updating accounts.
Setting Up Your Python Environment for Sugar Market API
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests.
- Install Python 3.11.1 if you haven't already.
- Use the following command to install the
requests
library:
pip install requests
Creating or Updating Accounts with Sugar Market API
Once your environment is ready, you can proceed to create or update accounts using the Sugar Market API. Below is an example of how to perform these actions using Python.
Example Code to Create an Account
import requests
# Define the API endpoint and headers
url = "https://api.sugarmarket.com/v1/accounts"
headers = {
"Content-Type": "application/json",
"Authorization": "Bearer Your_Access_Token"
}
# Define the account data
account_data = {
"name": "New Account",
"industry": "Technology",
"website": "https://example.com"
}
# Make a POST request to create the account
response = requests.post(url, json=account_data, headers=headers)
# Check if the request was successful
if response.status_code == 201:
print("Account created successfully.")
else:
print("Failed to create account:", response.json())
Replace Your_Access_Token
with the token obtained from your Sugar Market account. This code sends a POST request to create a new account with the specified details.
Example Code to Update an Account
import requests
# Define the API endpoint and headers
url = "https://api.sugarmarket.com/v1/accounts/{account_id}"
headers = {
"Content-Type": "application/json",
"Authorization": "Bearer Your_Access_Token"
}
# Define the updated account data
updated_data = {
"industry": "Healthcare"
}
# Make a PUT request to update the account
response = requests.put(url, json=updated_data, headers=headers)
# Check if the request was successful
if response.status_code == 200:
print("Account updated successfully.")
else:
print("Failed to update account:", response.json())
Replace {account_id}
with the actual account ID you wish to update. This code sends a PUT request to update the specified account's industry.
Verifying API Call Success in Sugar Market
To verify that your API calls were successful, log into your Sugar Market sandbox account and check the accounts section. You should see the newly created or updated account details reflected there.
Handling Errors and Troubleshooting
When making API calls, it's essential to handle potential errors. The Sugar Market API will return error codes and messages if something goes wrong. Common error codes include:
- 400 Bad Request: The request was invalid or cannot be served.
- 401 Unauthorized: Authentication failed or user does not have permissions.
- 404 Not Found: The requested resource could not be found.
- 500 Internal Server Error: An error occurred on the server.
Always check the response and handle errors appropriately in your code to ensure a smooth integration process.
Conclusion: Best Practices for Sugar Market API Integration
Integrating with the Sugar Market API can significantly enhance your marketing automation efforts by allowing seamless data synchronization and process automation. To ensure a successful integration, consider the following best practices:
Securely Storing Sugar Market API Credentials
Always store your API credentials securely. Use environment variables or secure vaults to keep your client ID, client secret, and access tokens safe. Avoid hardcoding these credentials in your source code.
Handling Sugar Market API Rate Limits
Be mindful of the API rate limits imposed by Sugar Market. Implement logic to handle rate limiting gracefully, such as retrying requests after a delay or queuing requests to avoid exceeding the limit.
Transforming and Standardizing Data Fields
Ensure that data fields are transformed and standardized to match the requirements of both Sugar Market and any other systems you are integrating with. This will help maintain data consistency across platforms.
Utilizing Endgrate for Efficient Integration Management
Consider using Endgrate to streamline your integration processes. Endgrate allows you to build once for each use case and manage multiple integrations efficiently. By outsourcing integrations, you can focus on your core product and provide an intuitive integration experience for your customers.
For more information on how Endgrate can simplify your integration efforts, visit Endgrate.
Read More
Ready to get started?