Using the Sap Business One API to Get Customers (with Javascript examples)
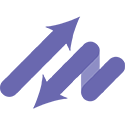
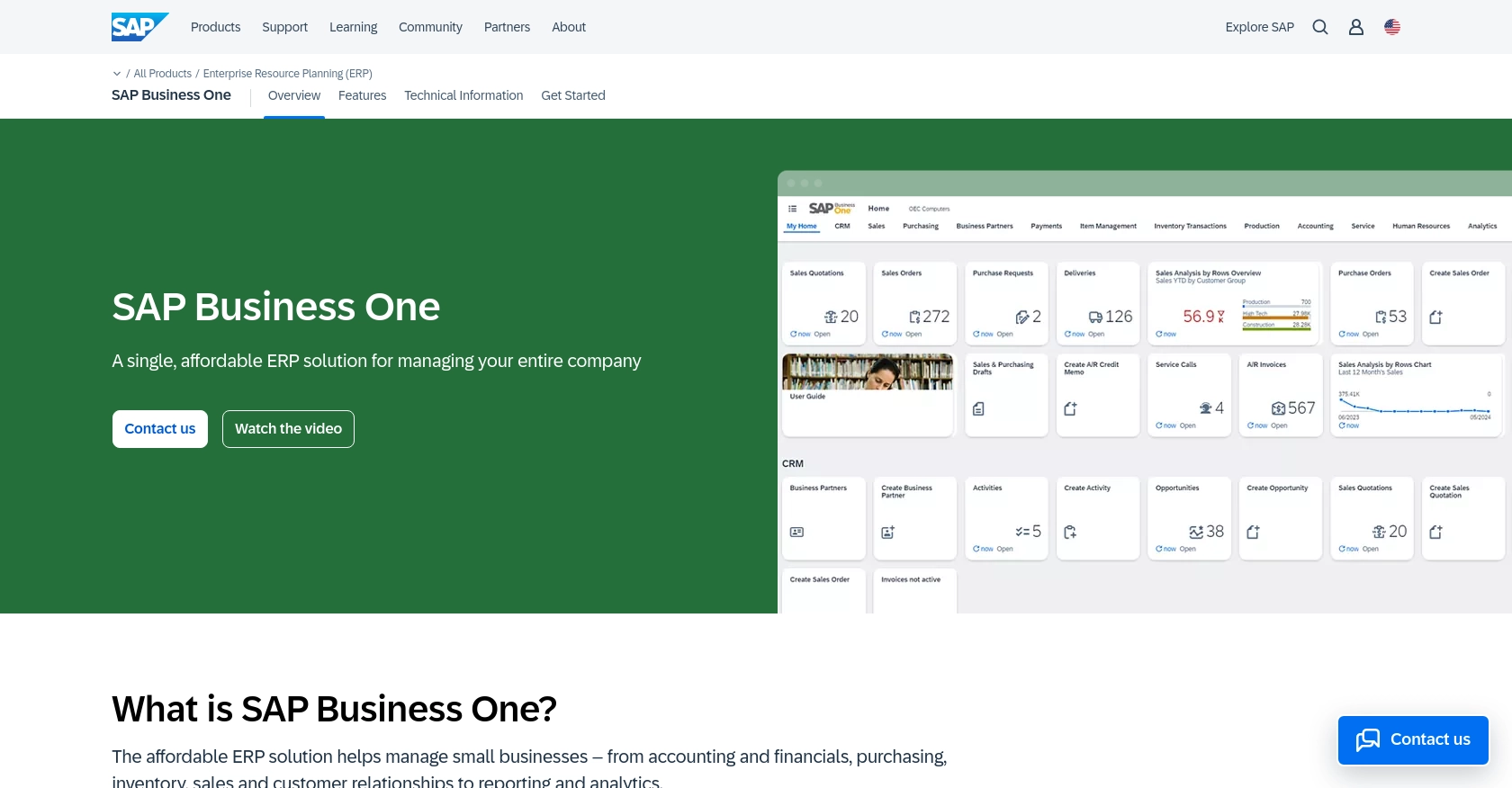
Introduction to SAP Business One
SAP Business One is a comprehensive enterprise resource planning (ERP) solution tailored for small to medium-sized businesses. It offers a wide array of functionalities, including financial management, sales, customer relationship management, and inventory control, all designed to streamline business operations and enhance efficiency.
Integrating with the SAP Business One API allows developers to access and manage critical business data programmatically. For example, you might want to retrieve customer information to synchronize with an external CRM system, ensuring that customer data is consistent and up-to-date across platforms.
Setting Up a Test/Sandbox Account for SAP Business One API
Before you can start interacting with the SAP Business One API, you need to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting live data.
Creating a SAP Business One Sandbox Account
To begin, you need access to a SAP Business One sandbox environment. If your organization already uses SAP Business One, you may request access to a test environment from your system administrator. If not, consider reaching out to SAP or a certified SAP partner to explore trial options.
Configuring OAuth Authentication for SAP Business One API
SAP Business One uses a custom authentication method. Follow these steps to configure your authentication:
- Log in to your SAP Business One sandbox account.
- Navigate to the Service Layer settings.
- Create a new application to generate your Client ID and Client Secret.
- Ensure that your application has the necessary permissions to access customer data.
- Save your Client ID and Client Secret securely, as you will need them for API requests.
For detailed instructions, refer to the SAP Business One Service Layer documentation.
Generating an API Key for SAP Business One
In addition to OAuth, you may need an API key for certain operations. Follow these steps to generate an API key:
- Access the API management section within your SAP Business One sandbox account.
- Locate the option to create a new API key.
- Generate the key and store it securely.
Ensure that the API key has the appropriate access rights for customer data retrieval.
With your sandbox account and authentication credentials set up, you are ready to start making API calls to retrieve customer data using JavaScript.
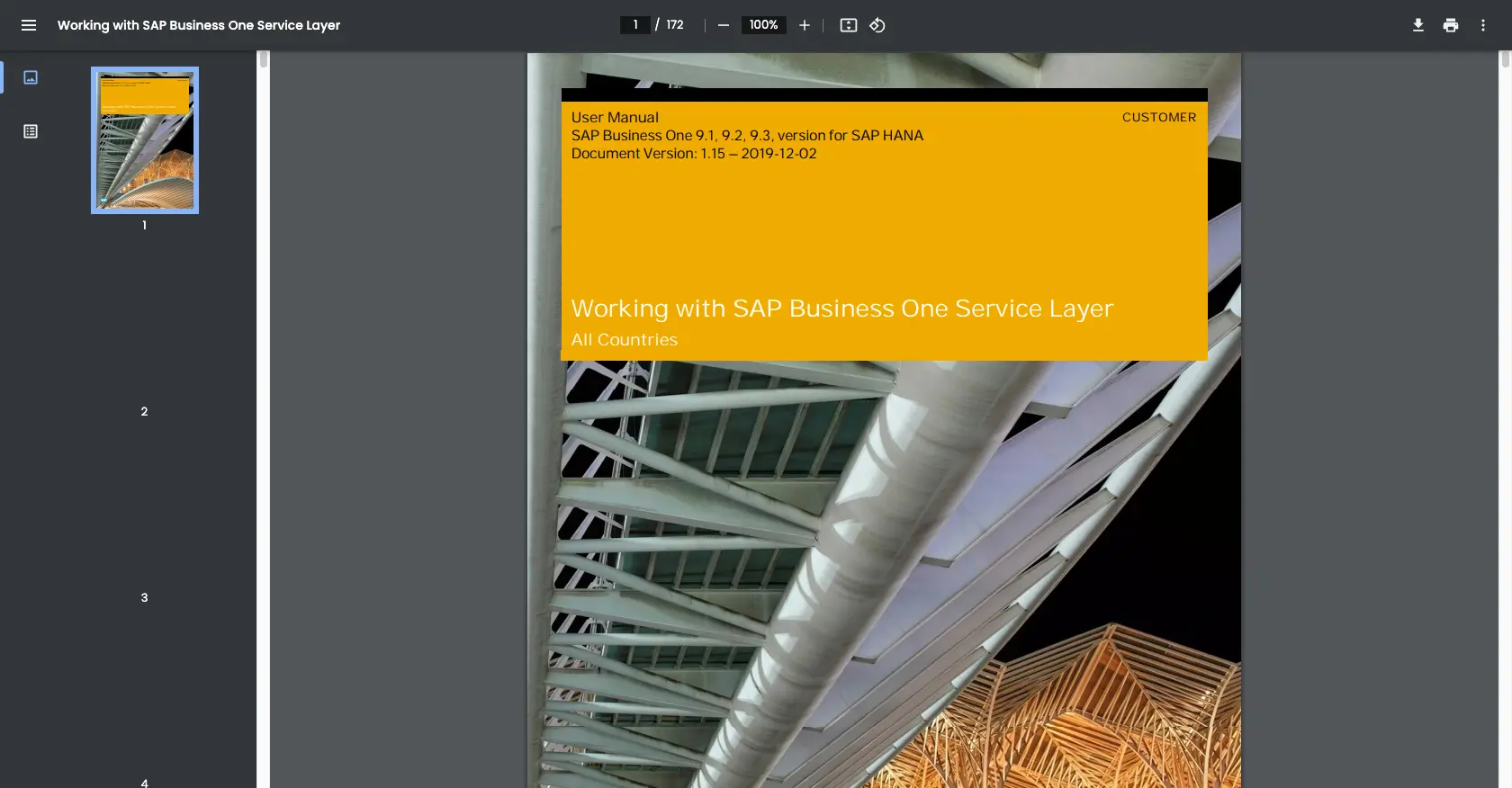
sbb-itb-96038d7
Making API Calls to Retrieve Customers from SAP Business One Using JavaScript
To interact with the SAP Business One API and retrieve customer data, you'll need to use JavaScript. This section will guide you through the process, including setting up your environment, writing the code, and handling potential errors.
Setting Up Your JavaScript Environment for SAP Business One API
Before making API calls, ensure you have the following prerequisites:
- Node.js installed on your machine. You can download it from the official Node.js website.
- A code editor like Visual Studio Code.
- The
axios
library for making HTTP requests. Install it using the command:
npm install axios
Writing JavaScript Code to Retrieve Customers
Now, let's write the JavaScript code to make an API call to SAP Business One and retrieve customer data. Create a file named getCustomers.js
and add the following code:
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://your-sap-business-one-url.com/b1s/v1/BusinessPartners';
const headers = {
'Content-Type': 'application/json',
'Authorization': 'Bearer Your_Access_Token'
};
// Function to get customers
async function getCustomers() {
try {
const response = await axios.get(endpoint, { headers });
const customers = response.data.value;
console.log('Retrieved Customers:', customers);
} catch (error) {
console.error('Error fetching customers:', error.response ? error.response.data : error.message);
}
}
// Call the function
getCustomers();
Replace Your_Access_Token
with the token you obtained during the authentication setup.
Running the JavaScript Code and Verifying Results
To execute the code, run the following command in your terminal:
node getCustomers.js
If successful, you should see a list of customers printed in the console. Verify the data by checking your SAP Business One sandbox account to ensure it matches the retrieved information.
Handling Errors and Common Error Codes
When interacting with the SAP Business One API, you might encounter errors. Here are some common error codes and how to handle them:
- 401 Unauthorized: Check if your access token is valid and has the necessary permissions.
- 404 Not Found: Ensure the endpoint URL is correct.
- 500 Internal Server Error: This might be an issue with the SAP Business One server. Try again later or contact support.
For more detailed error handling, refer to the SAP Business One API Reference.
Conclusion and Best Practices for Using SAP Business One API with JavaScript
Integrating with the SAP Business One API using JavaScript can significantly enhance your ability to manage and synchronize customer data across platforms. By following the steps outlined in this guide, you can efficiently retrieve customer information and ensure data consistency.
Best Practices for Secure and Efficient SAP Business One API Integration
- Secure Storage of Credentials: Always store your API keys and tokens securely. Consider using environment variables or a secure vault to manage sensitive information.
- Handle Rate Limiting: Be mindful of the API's rate limits to avoid throttling. Implement exponential backoff strategies to manage retries gracefully.
- Data Transformation and Standardization: Ensure that the data retrieved from SAP Business One is transformed and standardized to match your application's data structures.
Streamlining Integration Development with Endgrate
While building integrations with SAP Business One can be rewarding, it can also be time-consuming and complex. Endgrate offers a solution by providing a unified API endpoint that simplifies the integration process across multiple platforms, including SAP Business One.
By leveraging Endgrate, you can save time and resources, allowing your team to focus on core product development. With Endgrate, you build once for each use case, reducing the need for multiple integrations and enhancing the overall integration experience for your customers.
Explore how Endgrate can transform your integration strategy by visiting Endgrate today.
Read More
Ready to get started?