How to Get Opportunities with the Copper API in Javascript
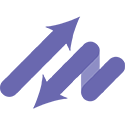
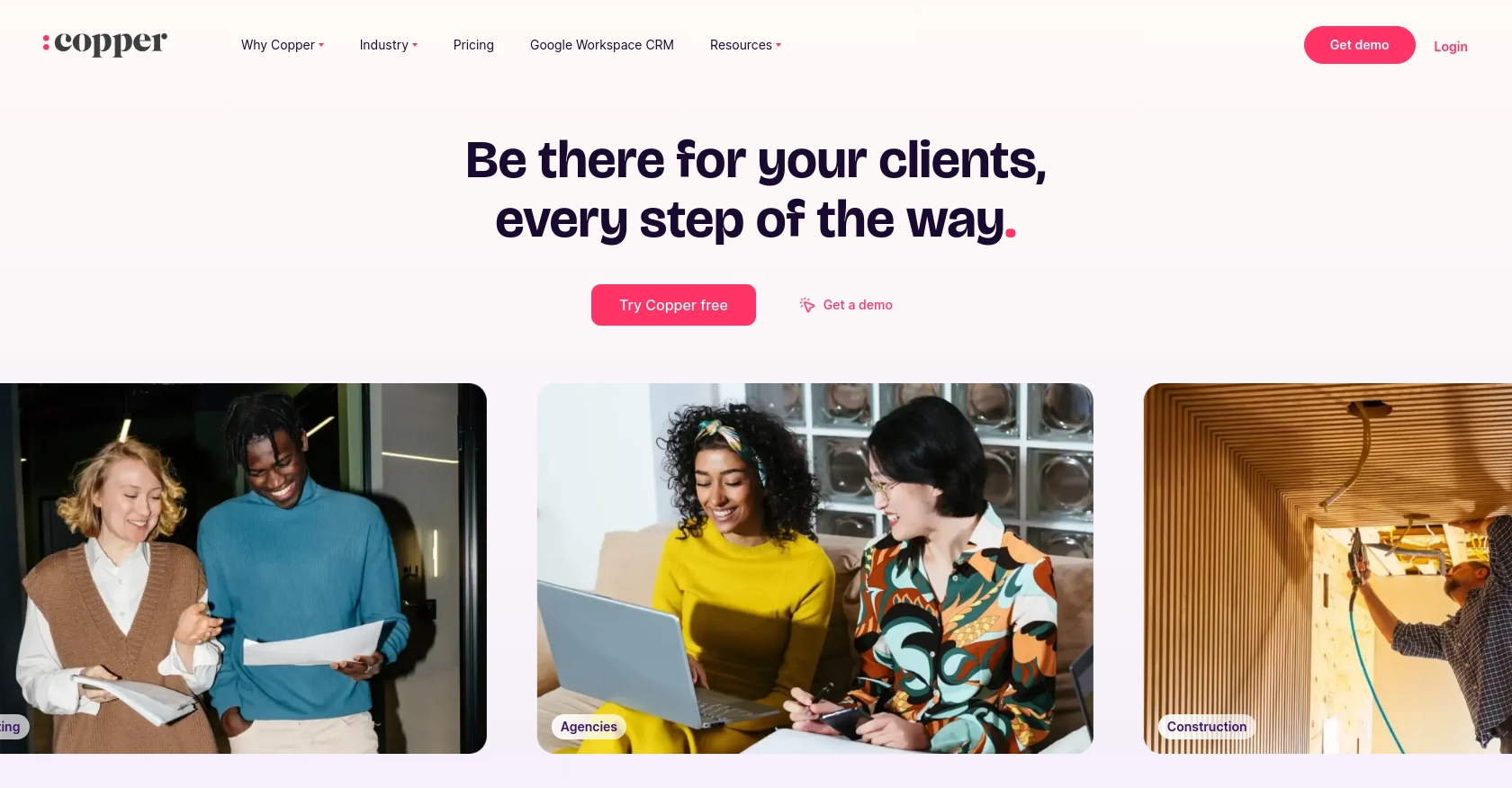
Introduction to Copper API for B2B SaaS Integration
Copper is a powerful platform that offers a comprehensive API for seamless integration with various applications. It is designed to facilitate efficient data exchange and management, making it an ideal choice for B2B SaaS products looking to enhance their integration capabilities.
Developers might want to connect with Copper to access and manage opportunities, streamline workflows, and automate business processes. For example, using the Copper API, a developer can retrieve and manage sales opportunities, enabling more effective sales pipeline management and customer relationship strategies.
Setting Up Your Copper API Test or Sandbox Account
Before you can start integrating with the Copper API, you'll need to set up a test or sandbox account. This environment allows developers to safely experiment with API calls without affecting live data, ensuring a smooth integration process.
Creating a Copper Sandbox Account
To begin, visit the Copper website and sign up for a sandbox account. This account will provide you with access to Copper's API features in a controlled environment. Follow these steps:
- Navigate to the Copper website and click on the "Sign Up" button.
- Choose the option for a sandbox or developer account.
- Fill in the required details, such as your name, email, and company information.
- Verify your email address to activate the account.
Generating Copper API Keys for Authentication
Once your sandbox account is set up, you'll need to generate API keys for authentication. Copper uses a custom authentication method involving an API key and a secret. Follow these steps to obtain them:
- Log in to your Copper sandbox account.
- Navigate to the "API Settings" section in your account dashboard.
- Click on "Generate API Key" to create a new key.
- Copy the API key and secret to a secure location, as you'll need them for API requests.
Configuring OAuth for Copper API Access
For enhanced security, Copper supports OAuth-based authentication. To set this up:
- Go to the "OAuth Settings" in your Copper account.
- Create a new OAuth app by providing necessary details such as the app name and redirect URI.
- Once created, note down the client ID and client secret.
- Use these credentials to authenticate API requests via OAuth.
With your Copper sandbox account and API keys ready, you are now set to explore the Copper API and integrate it into your B2B SaaS product. This setup ensures you can test and refine your integration without impacting live data.
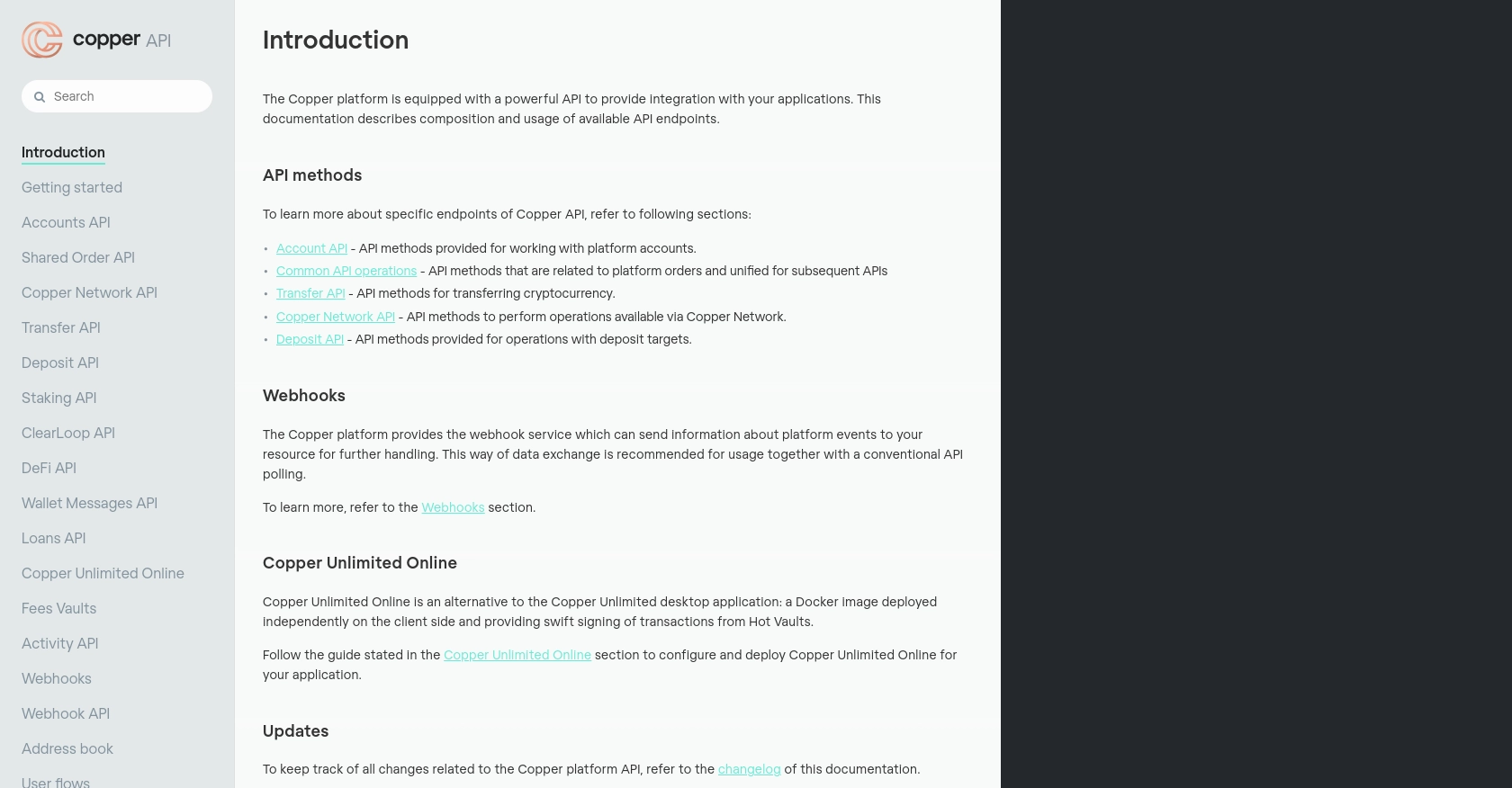
sbb-itb-96038d7
Making API Calls to Retrieve Opportunities with Copper API in JavaScript
To interact with the Copper API and retrieve opportunities, you'll need to use JavaScript to make HTTP requests. This section will guide you through the process of setting up your environment, writing the code, and handling responses effectively.
Setting Up Your JavaScript Environment for Copper API Integration
Before making API calls, ensure you have Node.js installed on your machine. You will also need the axios
library to handle HTTP requests. Install it using npm:
npm install axios
Writing JavaScript Code to Retrieve Opportunities from Copper API
Below is a sample code snippet to retrieve opportunities using the Copper API:
const axios = require('axios');
// Copper API credentials
const apiKey = 'Your_API_Key';
const apiSecret = 'Your_API_Secret';
// Set up the request headers
const headers = {
'Authorization': `ApiKey ${apiKey}`,
'X-Signature': 'Generated_Signature',
'X-Timestamp': Date.now().toString(),
'Content-Type': 'application/json'
};
// Define the API endpoint
const endpoint = 'https://api.copper.co/platform/opportunities';
// Make the GET request to retrieve opportunities
axios.get(endpoint, { headers })
.then(response => {
console.log('Opportunities:', response.data);
})
.catch(error => {
console.error('Error fetching opportunities:', error);
});
Understanding the Sample Code for Copper API Integration
- API Credentials: Replace
Your_API_Key
andYour_API_Secret
with your actual Copper API credentials. - Headers: The headers include authorization and signature for secure API access. Ensure the signature is generated correctly as per Copper's requirements.
- Endpoint: The endpoint
https://api.copper.co/platform/opportunities
is used to fetch opportunities data. - Handling Responses: The response data is logged to the console. You can modify this to suit your application's needs.
Verifying Successful API Requests in Copper Sandbox
After running the code, verify the retrieved opportunities by checking your Copper sandbox account. Ensure the data matches the expected output. If there are discrepancies, review the API documentation and adjust your request parameters accordingly.
Handling Errors and Common Copper API Error Codes
When making API calls, handle potential errors gracefully. Copper API may return various error codes, such as:
- 400 Bad Request: Check for validation errors or missing parameters.
- 401 Unauthorized: Verify your API key and signature.
- 429 Request Limit Exceeded: Copper API limits requests to 30,000 per 5 minutes. Implement rate limiting in your application.
For more details on error codes, refer to the Copper API documentation.
Conclusion and Best Practices for Copper API Integration in JavaScript
Integrating with the Copper API using JavaScript can significantly enhance your B2B SaaS product by streamlining data management and automating workflows. By following the steps outlined in this guide, you can efficiently retrieve and manage opportunities, improving your sales pipeline and customer relationship strategies.
Best Practices for Secure and Efficient Copper API Integration
- Secure API Credentials: Always store your API key and secret securely. Avoid hardcoding them in your source code and consider using environment variables.
- Implement Rate Limiting: Copper API has a request limit of 30,000 requests per 5 minutes. Ensure your application handles rate limiting to avoid disruptions.
- Handle Errors Gracefully: Implement robust error handling to manage API errors effectively. Refer to the Copper API documentation for detailed error code explanations.
- Regularly Review API Documentation: Stay updated with Copper's API documentation to leverage new features and ensure compliance with any changes.
Transforming Data for Seamless Copper API Integration
When integrating with the Copper API, consider transforming and standardizing data fields to match your application's requirements. This ensures consistency and improves data interoperability across different systems.
Leverage Endgrate for Simplified Integration Solutions
For developers looking to streamline their integration processes further, consider using Endgrate. With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Endgrate provides a unified API endpoint that connects to multiple platforms, including Copper, offering an intuitive integration experience for your customers.
Visit Endgrate to learn more about how you can enhance your integration capabilities and deliver a seamless experience to your users.
Read More
Ready to get started?