How to Get Page Parameters with the Pendo API in Python
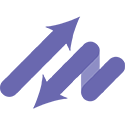
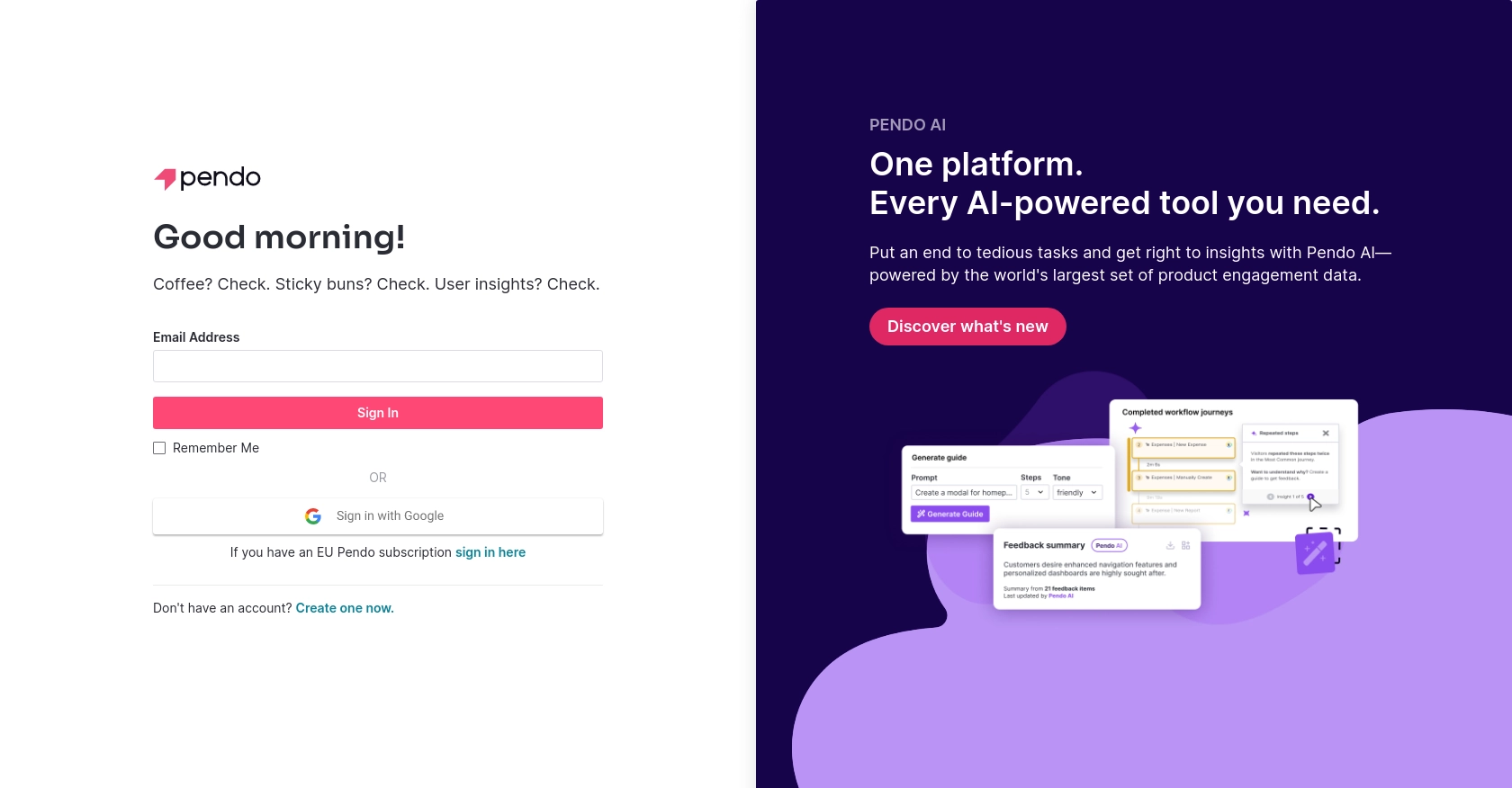
Introduction to Pendo API
Pendo is a powerful platform designed to help businesses understand and guide user behavior within their software applications. By providing insights into user interactions, Pendo enables companies to enhance their product experience and drive user engagement.
Developers might want to integrate with the Pendo API to access detailed analytics and user data, such as page parameters, which can be crucial for optimizing user journeys and personalizing content. For example, a developer could use the Pendo API to retrieve page parameters in order to analyze which features users interact with the most, allowing for data-driven decisions to improve the application.
Setting Up Your Pendo Test Account for API Access
Before you can start using the Pendo API to retrieve page parameters, you'll need to set up a Pendo account. This will allow you to generate the necessary API key for authentication and access the data you need.
Creating a Pendo Account
If you don't already have a Pendo account, you can sign up for a free trial on the Pendo website. This will give you access to the platform's features and allow you to explore its capabilities.
- Visit the Pendo website and click on the "Sign Up" button.
- Fill in the required information, such as your name, email, and company details.
- Follow the instructions to complete the registration process and log in to your new Pendo account.
Generating Your Pendo API Key
Once your account is set up, you'll need to generate an API key to authenticate your requests to the Pendo API. Follow these steps to obtain your API key:
- Log in to your Pendo account and navigate to the "Settings" section.
- Under "Integrations," find the "API Keys" option and click on it.
- Click on "Generate API Key" to create a new key.
- Copy the generated API key and store it securely, as you'll need it for making API calls.
With your Pendo account and API key ready, you can now proceed to interact with the Pendo API using Python to retrieve page parameters and gain valuable insights into user behavior.
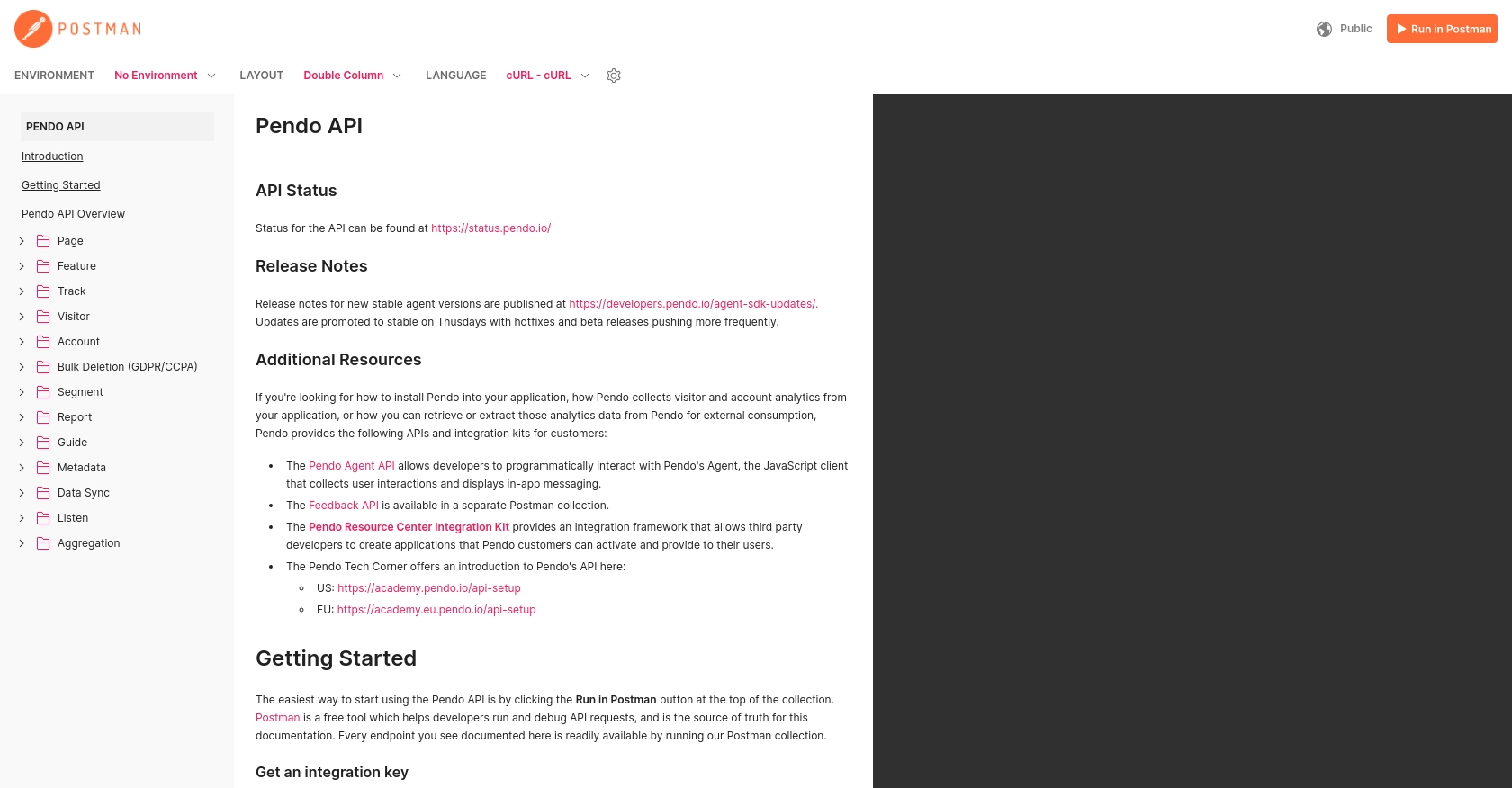
sbb-itb-96038d7
Using Python to Make API Calls to Retrieve Pendo Page Parameters
To interact with the Pendo API and retrieve page parameters using Python, you'll need to ensure your environment is set up correctly. This section will guide you through the necessary steps, including installing dependencies and writing the code to make the API call.
Setting Up Your Python Environment for Pendo API Integration
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests.
- Verify your Python installation by running
python --version
in your terminal. - Install the
requests
library using pip:
pip install requests
Writing Python Code to Retrieve Pendo Page Parameters
With your environment ready, you can now write the Python code to call the Pendo API and retrieve page parameters. Follow these steps:
import requests
# Set the API endpoint and headers
endpoint = "https://engageapi.pendo.io/api/v1/pageParameters"
headers = {
"Content-Type": "application/json",
"x-pendo-integration-key": "Your_API_Key"
}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
# Parse the JSON data from the response
data = response.json()
# Print the page parameters
for parameter in data:
print(parameter)
else:
print(f"Failed to retrieve page parameters. Status code: {response.status_code}")
Replace Your_API_Key
with the API key you generated from your Pendo account.
Verifying Successful API Calls and Handling Errors
After running the script, you should see the page parameters printed in your terminal. If the request fails, the script will output the status code, helping you diagnose the issue.
Common error codes include:
- 401 Unauthorized: Check if your API key is correct.
- 404 Not Found: Verify the endpoint URL.
For more details on error codes, refer to the Pendo API documentation.
Conclusion and Best Practices for Using the Pendo API in Python
Integrating with the Pendo API allows developers to access valuable insights into user behavior, enabling data-driven decisions to enhance product experiences. By following the steps outlined in this guide, you can efficiently retrieve page parameters using Python, providing a foundation for further analysis and optimization.
Best Practices for Secure and Efficient API Integration
- Securely Store API Keys: Always store your API keys securely, using environment variables or a secure vault, to prevent unauthorized access.
- Handle Rate Limiting: Be aware of any rate limits imposed by the Pendo API to avoid exceeding them. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Standardize Data Fields: Ensure that the data retrieved from the API is standardized and transformed as needed to fit your application's requirements.
Streamline Your Integrations with Endgrate
Building and maintaining multiple integrations can be time-consuming and complex. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Pendo. By leveraging Endgrate, you can save time and resources, allowing you to focus on your core product development.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate and discover a more efficient way to manage your integrations.
Read More
Ready to get started?