How to Get Applicants with the JazzHR API in Javascript
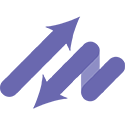
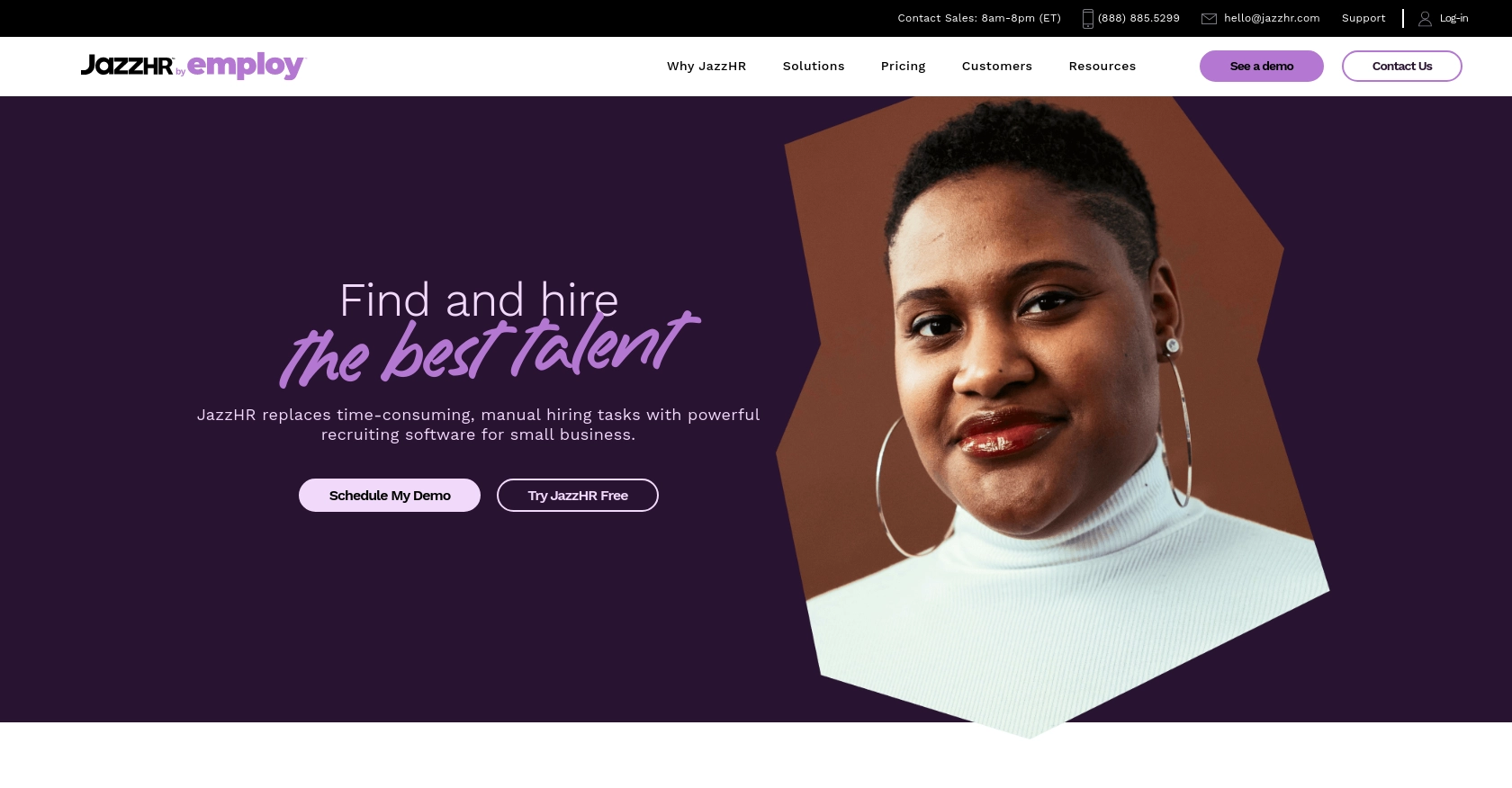
Introduction to JazzHR
JazzHR is a powerful recruiting software designed to streamline the hiring process for businesses of all sizes. It offers a comprehensive suite of tools to manage job postings, track applicants, and collaborate with hiring teams, making it a popular choice for companies looking to enhance their recruitment efforts.
Integrating with the JazzHR API allows developers to automate and optimize various aspects of the recruitment process. For example, you can use the API to retrieve applicant data directly into your own systems, enabling seamless integration with your existing HR tools and workflows.
This article will guide you through the process of using JavaScript to interact with the JazzHR API, specifically focusing on how to retrieve applicant information efficiently.
Setting Up Your JazzHR Account for API Access
Before you can start interacting with the JazzHR API using JavaScript, you'll need to set up your JazzHR account to obtain the necessary API credentials. This involves generating an API key, which will be used to authenticate your requests.
Creating a JazzHR Account
If you don't already have a JazzHR account, you'll need to sign up for one. Visit the JazzHR website and follow the instructions to create a free trial or demo account. This will give you access to the platform's features and allow you to explore its capabilities.
Generating Your JazzHR API Key
Once your account is set up, follow these steps to generate your API key:
- Log in to your JazzHR account.
- Navigate to the Integrations section in the account settings.
- Locate the API key section and generate a new API key.
- Copy the API key and store it securely, as you'll need it to authenticate your API requests.
For more detailed instructions, you can refer to the JazzHR API documentation.
Understanding API Rate Limits
JazzHR imposes a rate limit on API calls to ensure fair usage. Each API call is limited to 100 results per page. If you need to retrieve more results, you can paginate through the data by appending /page/#
to the request URI, where #
is the page number. Be mindful of these limits to avoid hitting rate restrictions during your integration process.
For more information on rate limits, visit the JazzHR API documentation.
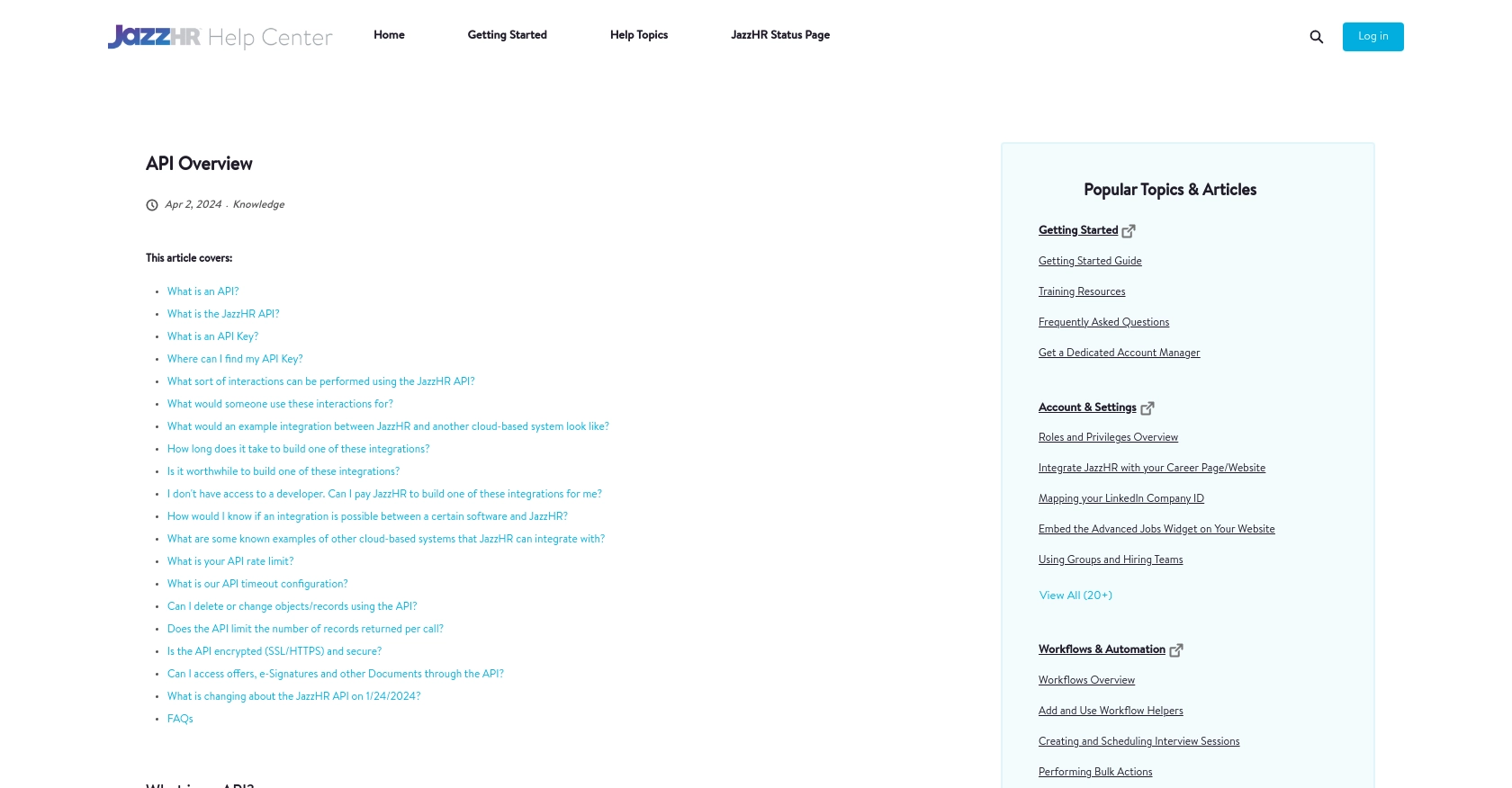
sbb-itb-96038d7
Making API Calls to Retrieve Applicants from JazzHR Using JavaScript
To interact with the JazzHR API and retrieve applicant data using JavaScript, you'll need to set up your development environment and write the necessary code to make API requests. This section will guide you through the process, including setting up JavaScript, making the API call, and handling the response.
Setting Up Your JavaScript Environment
Before you begin, ensure you have Node.js installed on your machine. Node.js allows you to run JavaScript code outside of a browser, making it ideal for server-side scripting and API interactions.
To install Node.js, visit the official Node.js website and download the appropriate version for your operating system. Once installed, you can use npm (Node Package Manager) to manage your project dependencies.
Installing Required Dependencies
For this tutorial, you'll need the axios
library to handle HTTP requests. Install it by running the following command in your terminal:
npm install axios
Writing JavaScript Code to Fetch Applicants from JazzHR
Create a new JavaScript file named getApplicants.js
and add the following code to it:
const axios = require('axios');
// Set your JazzHR API key
const apiKey = 'Your_API_Key';
// Define the API endpoint
const endpoint = 'https://api.resumatorapi.com/v1/applicants';
// Make a GET request to the JazzHR API
axios.get(endpoint, {
headers: {
'Authorization': `Bearer ${apiKey}`
}
})
.then(response => {
// Handle the successful response
const applicants = response.data;
console.log('Applicants:', applicants);
})
.catch(error => {
// Handle errors
console.error('Error fetching applicants:', error);
});
Replace Your_API_Key
with the API key you generated from your JazzHR account. This code uses the axios
library to send a GET request to the JazzHR API endpoint for applicants. If the request is successful, it logs the applicant data to the console. If there's an error, it logs the error message.
Running Your JavaScript Code
To execute your code, run the following command in your terminal:
node getApplicants.js
You should see the list of applicants retrieved from JazzHR displayed in your console. This confirms that your API call was successful.
Handling Errors and Verifying API Requests
When making API calls, it's crucial to handle potential errors gracefully. The code above includes a catch
block to log any errors that occur during the request. Common errors might include network issues or invalid API keys.
To verify that your request succeeded, you can cross-check the returned applicant data with the data available in your JazzHR account. Ensure that the data matches and that pagination is handled correctly if you have more than 100 applicants.
Conclusion and Best Practices for Using the JazzHR API with JavaScript
Integrating with the JazzHR API using JavaScript allows you to efficiently manage and retrieve applicant data, streamlining your recruitment processes. By following the steps outlined in this tutorial, you can seamlessly connect your systems with JazzHR and enhance your HR workflows.
Best Practices for Secure and Efficient API Integration with JazzHR
- Secure API Key Storage: Always store your API keys securely. Avoid hardcoding them directly into your codebase. Consider using environment variables or secure vaults to manage sensitive credentials.
- Handle Rate Limits: Be mindful of JazzHR's rate limits, which restrict API calls to 100 results per page. Implement pagination to handle large datasets and avoid exceeding these limits.
- Error Handling: Implement robust error handling to manage potential issues such as network errors or invalid API keys. Use try-catch blocks and log errors for troubleshooting.
- Data Standardization: Ensure that the data retrieved from JazzHR is standardized and transformed as needed to fit your existing systems and workflows.
Streamline Your Integrations with Endgrate
While integrating with the JazzHR API can greatly enhance your recruitment processes, managing multiple integrations can be complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process across various platforms, including JazzHR.
By using Endgrate, you can save time and resources, allowing you to focus on your core product. Build once for each use case instead of multiple times for different integrations, and provide an intuitive integration experience for your customers.
Explore how Endgrate can transform your integration strategy by visiting Endgrate today.
Read More
Ready to get started?