Using the Todoist API to Get Tasks in Python
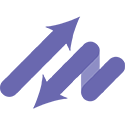
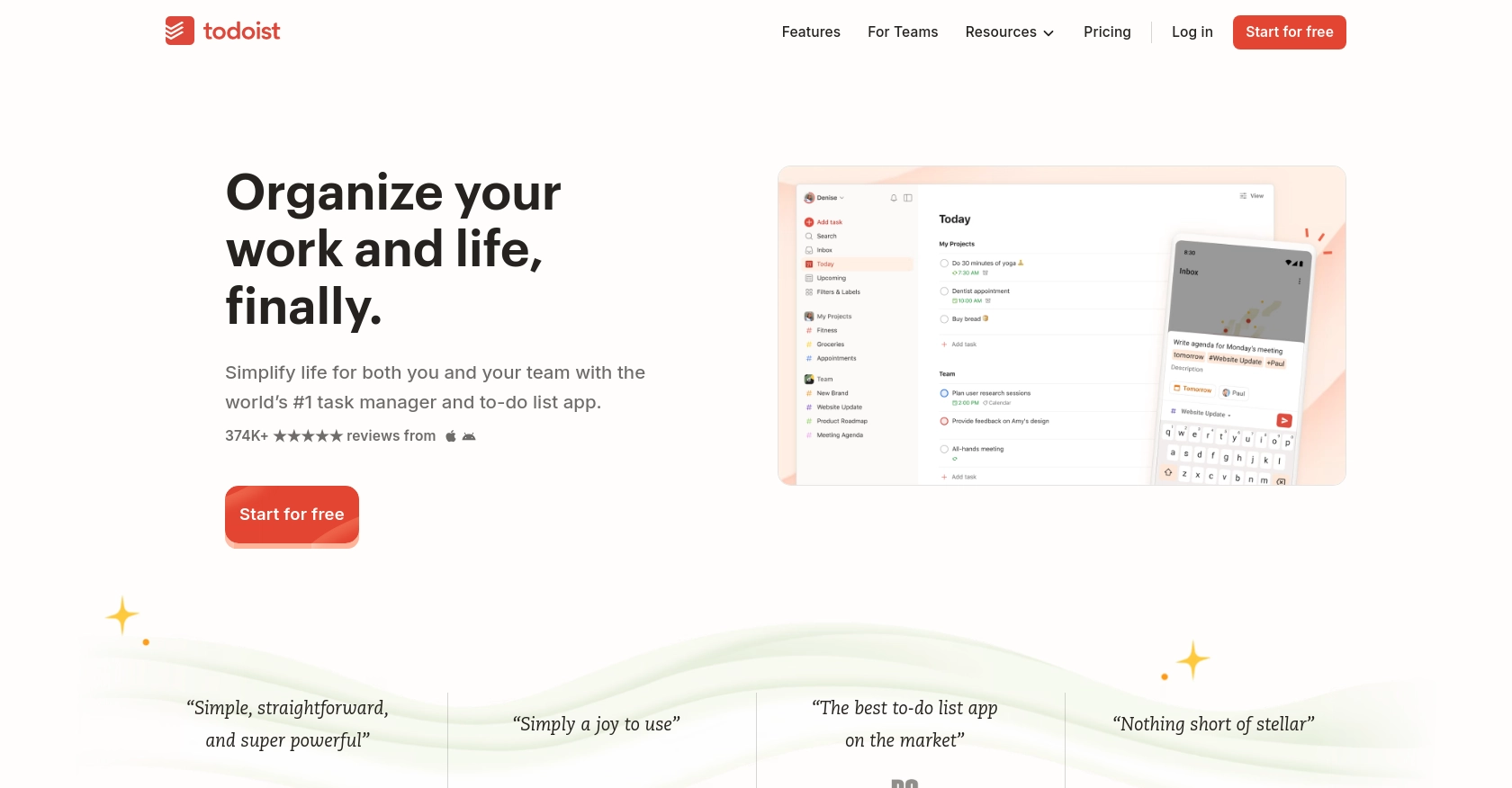
Introduction to Todoist API for Task Management
Todoist is a popular task management application that helps individuals and teams organize, plan, and collaborate on projects. With its intuitive interface and robust features, Todoist is a go-to solution for managing tasks efficiently.
Integrating with the Todoist API allows developers to automate and enhance task management processes. By accessing Todoist's API, developers can retrieve, create, update, and manage tasks programmatically, enabling seamless integration with other applications and systems.
For example, a developer might use the Todoist API to fetch tasks and display them in a custom dashboard, providing a unified view of tasks across multiple platforms. This integration can streamline workflows and improve productivity by ensuring that all tasks are easily accessible and manageable from a single interface.
Setting Up Your Todoist Developer Account for API Access
Before you can start interacting with the Todoist API, you'll need to set up a developer account and obtain the necessary credentials. This process involves creating an application in Todoist and generating an API token for authentication.
Creating a Todoist Developer Account
If you don't already have a Todoist account, you can sign up for a free account on the Todoist website. Once your account is set up, you can proceed to the developer section to create your application.
Generating an API Token for Todoist
Todoist uses OAuth for authentication, which requires you to create an application to obtain a client ID and client secret. Follow these steps to generate your API token:
- Log in to your Todoist account and navigate to the Integrations section.
- Click on Create New App to start the process of creating a new application.
- Fill in the required details for your application, such as the name and description.
- Once your app is created, you'll receive a client ID and client secret. Keep these credentials secure as they are essential for API authentication.
Obtaining Your Personal API Token
For testing purposes, you can use your personal API token to authenticate API requests. Here's how to find it:
- Go to your Todoist account settings.
- Navigate to the Integrations tab.
- Locate your personal API token and copy it for use in your API requests.
With your API token ready, you can now proceed to make authenticated requests to the Todoist API. This token will be used in the authorization header of your HTTP requests.
For more detailed information on setting up OAuth authentication, refer to the Todoist API documentation.
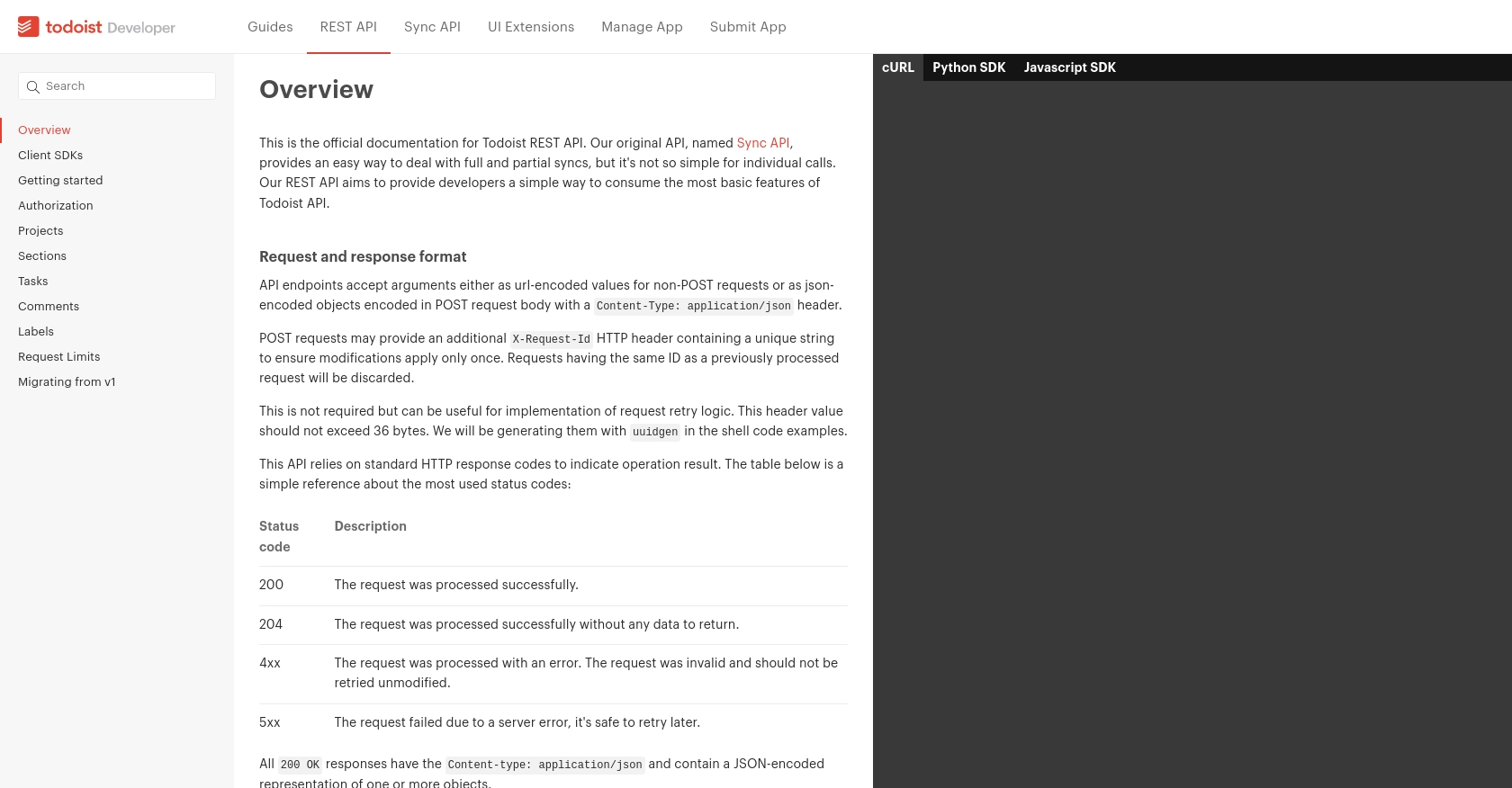
sbb-itb-96038d7
Making API Calls to Retrieve Tasks from Todoist Using Python
To interact with the Todoist API and retrieve tasks, you'll need to use Python. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling potential errors.
Setting Up Your Python Environment for Todoist API Integration
Before you begin, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests.
- Install Python from the official website if you haven't already.
- Open your terminal or command prompt and install the
requests
library using pip:
pip install requests
Writing Python Code to Fetch Tasks from Todoist
With your environment set up, you can now write the Python code to fetch tasks from Todoist. Create a new Python file named get_todoist_tasks.py
and add the following code:
import requests
# Set the API endpoint and headers
endpoint = "https://api.todoist.com/rest/v2/tasks"
headers = {"Authorization": "Bearer Your_Token"}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
tasks = response.json()
for task in tasks:
print(task)
else:
print(f"Failed to retrieve tasks: {response.status_code} - {response.text}")
Replace Your_Token
with your personal API token obtained from the Todoist integrations settings.
Running the Python Script to Retrieve Todoist Tasks
To execute the script and see the output, run the following command in your terminal:
python get_todoist_tasks.py
If successful, the script will print out the list of tasks from your Todoist account. If there are any issues, the error message will be displayed, providing insight into what went wrong.
Handling Errors and Verifying API Call Success
It's crucial to handle errors gracefully when making API calls. The Todoist API uses standard HTTP response codes to indicate success or failure:
- 200 OK: The request was successful, and tasks are returned.
- 4xx: Client error, such as invalid request or authentication failure.
- 5xx: Server error, indicating a problem on Todoist's end.
Refer to the Todoist API documentation for more details on error codes and handling.
Conclusion and Best Practices for Using Todoist API with Python
Integrating with the Todoist API using Python provides a powerful way to automate and enhance your task management workflows. By following the steps outlined in this guide, you can efficiently retrieve tasks and integrate them into your applications, improving productivity and streamlining processes.
Best Practices for Secure and Efficient Todoist API Integration
- Securely Store API Credentials: Always keep your API tokens and client secrets secure. Avoid hardcoding them in your scripts. Instead, use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Todoist API allows a maximum of 1000 requests per 15-minute period. Implement logic to handle rate limits gracefully, such as retrying requests after a delay.
- Standardize Data Fields: When integrating with multiple APIs, consider standardizing data fields to maintain consistency across different platforms.
Enhance Your Integrations with Endgrate
While integrating with Todoist API directly is powerful, managing multiple integrations can be complex. Endgrate simplifies this process by offering a unified API endpoint that connects to various platforms, including Todoist. This allows you to build once for each use case and streamline your integration efforts.
By leveraging Endgrate, you can save time and resources, allowing you to focus on your core product while providing an intuitive integration experience for your customers. Visit Endgrate to learn more about how it can enhance your integration strategy.
Read More
Ready to get started?