Using the Moneybird API to Get Recurring Invoices in Python
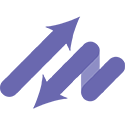
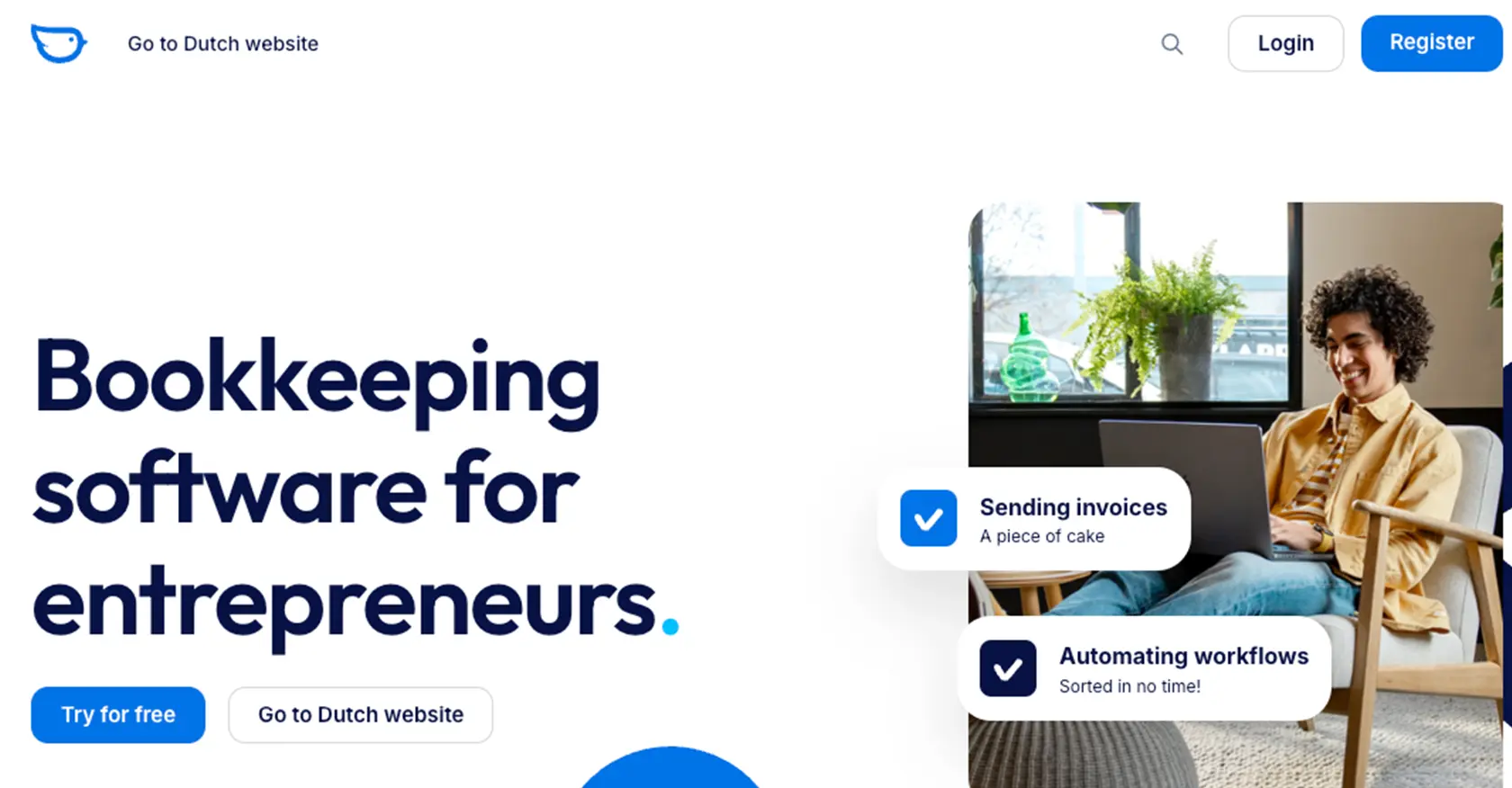
Introduction to Moneybird API for Recurring Invoices
Moneybird is a comprehensive financial management platform designed to simplify invoicing, bookkeeping, and financial administration for businesses. With its user-friendly interface and robust features, Moneybird helps businesses efficiently manage their financial tasks, from invoicing to tax calculations.
Integrating with the Moneybird API allows developers to automate and streamline financial processes, such as managing recurring invoices. For example, a developer might use the Moneybird API to automatically retrieve recurring invoice data, enabling seamless integration with other financial systems or custom applications.
This article will guide you through using Python to interact with the Moneybird API, specifically focusing on retrieving recurring invoices. By the end of this tutorial, you'll be equipped to efficiently access and manage recurring invoice data within the Moneybird platform using Python.
Setting Up Your Moneybird Sandbox Account for API Integration
Before diving into the Moneybird API, you'll need to set up a sandbox account. This allows you to test your integration without affecting live data. Moneybird provides a sandbox environment that mirrors the production environment, enabling you to experiment with all features safely.
Creating a Moneybird Sandbox Account
To begin, you'll need a Moneybird user account. If you don't have one, register on the Moneybird website. Once registered, follow these steps to create a sandbox account:
- Log in to your Moneybird account.
- Navigate to the sandbox creation page by visiting Moneybird Sandbox.
- Follow the prompts to create your sandbox environment. This will give you full access to Moneybird's features with some limitations, such as watermarked invoices.
Setting Up OAuth Authentication for Moneybird API
The Moneybird API uses OAuth2 for authentication, ensuring secure access to your data. Follow these steps to set up OAuth authentication:
- Log in to your Moneybird account and go to the application registration page.
- Register your application by providing the necessary details. You'll receive a Client ID and Client Secret upon registration.
- Use the following command to obtain a request token and authorization URL:
curl -vv 'https://moneybird.com/oauth/authorize?client_id=YOUR_CLIENT_ID&redirect_uri=urn:ietf:wg:oauth:2.0:oob&response_type=code'
Replace YOUR_CLIENT_ID
with your actual Client ID.
- Direct your user to the authorization URL. After authorization, Moneybird will provide a code.
- Exchange this code for an access token using the following command:
curl -vv -X POST -d "client_id=YOUR_CLIENT_ID&client_secret=YOUR_CLIENT_SECRET&code=YOUR_CODE&redirect_uri=urn:ietf:wg:oauth:2.0:oob&grant_type=authorization_code" https://moneybird.com/oauth/token
Replace YOUR_CLIENT_ID
, YOUR_CLIENT_SECRET
, and YOUR_CODE
with your actual credentials and code.
Storing Your Moneybird API Credentials Securely
Once you have your access token, store it securely. Treat it like a password, as it provides access to your Moneybird account. Consider using environment variables or a secure vault to manage your credentials.
With your sandbox account and OAuth authentication set up, you're ready to start interacting with the Moneybird API using Python.
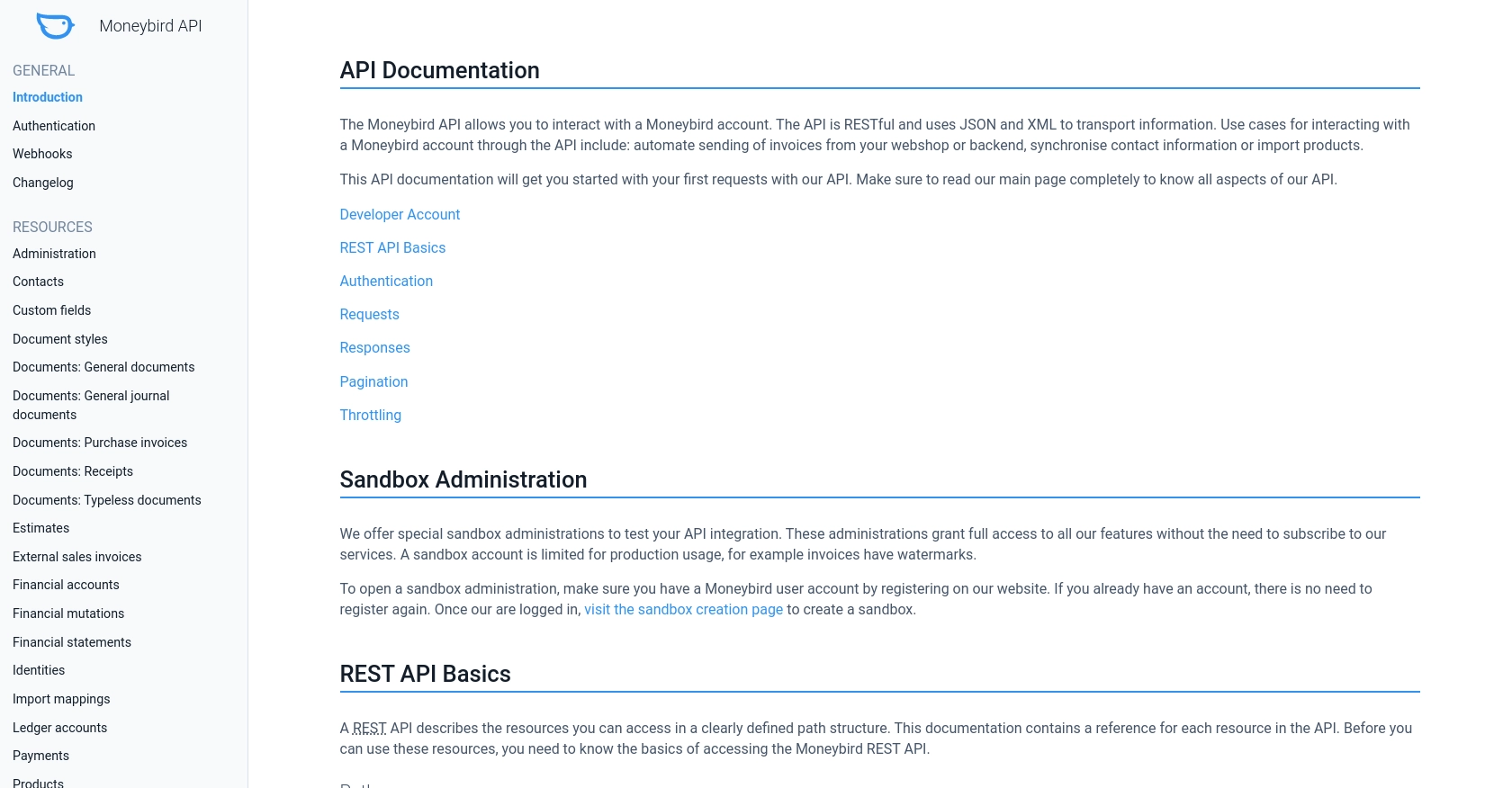
sbb-itb-96038d7
How to Make API Calls to Retrieve Recurring Invoices Using Moneybird API in Python
To interact with the Moneybird API and retrieve recurring invoices, you'll need to use Python. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling potential errors.
Setting Up Your Python Environment for Moneybird API Integration
Before making API calls, ensure you have the following prerequisites installed on your machine:
- Python 3.11.1 or later
- The Python package installer
pip
Next, install the requests
library, which will be used to make HTTP requests to the Moneybird API:
pip install requests
Writing Python Code to Retrieve Recurring Invoices from Moneybird
Create a new Python file named get_recurring_invoices.py
and add the following code:
import requests
# Set the API endpoint and headers
administration_id = "YOUR_ADMINISTRATION_ID"
endpoint = f"https://moneybird.com/api/v2/{administration_id}/recurring_sales_invoices.json"
headers = {
"Authorization": "Bearer YOUR_ACCESS_TOKEN",
"Content-Type": "application/json"
}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
invoices = response.json()
for invoice in invoices:
print(invoice)
else:
print(f"Failed to retrieve invoices: {response.status_code} - {response.text}")
Replace YOUR_ADMINISTRATION_ID
and YOUR_ACCESS_TOKEN
with your actual Moneybird administration ID and access token.
Understanding the Sample Output and Verifying API Call Success
Run the script using the following command:
python get_recurring_invoices.py
If successful, the script will print out the details of the recurring invoices. You can verify the retrieved data by checking your Moneybird sandbox account to ensure it matches the expected results.
Handling Errors and Understanding Moneybird API Error Codes
When making API calls, it's crucial to handle potential errors gracefully. The Moneybird API may return various HTTP status codes, such as:
- 200 OK: Request was successful.
- 400 Bad Request: Parameters are missing or malformed.
- 401 Authorization Required: Invalid or missing authorization information.
- 429 Too Many Requests: Rate limit exceeded. Retry after the specified time.
For more details on error codes, refer to the Moneybird Authentication Documentation.
By following these steps, you can efficiently retrieve recurring invoices from Moneybird using Python, enabling seamless integration with your financial systems.
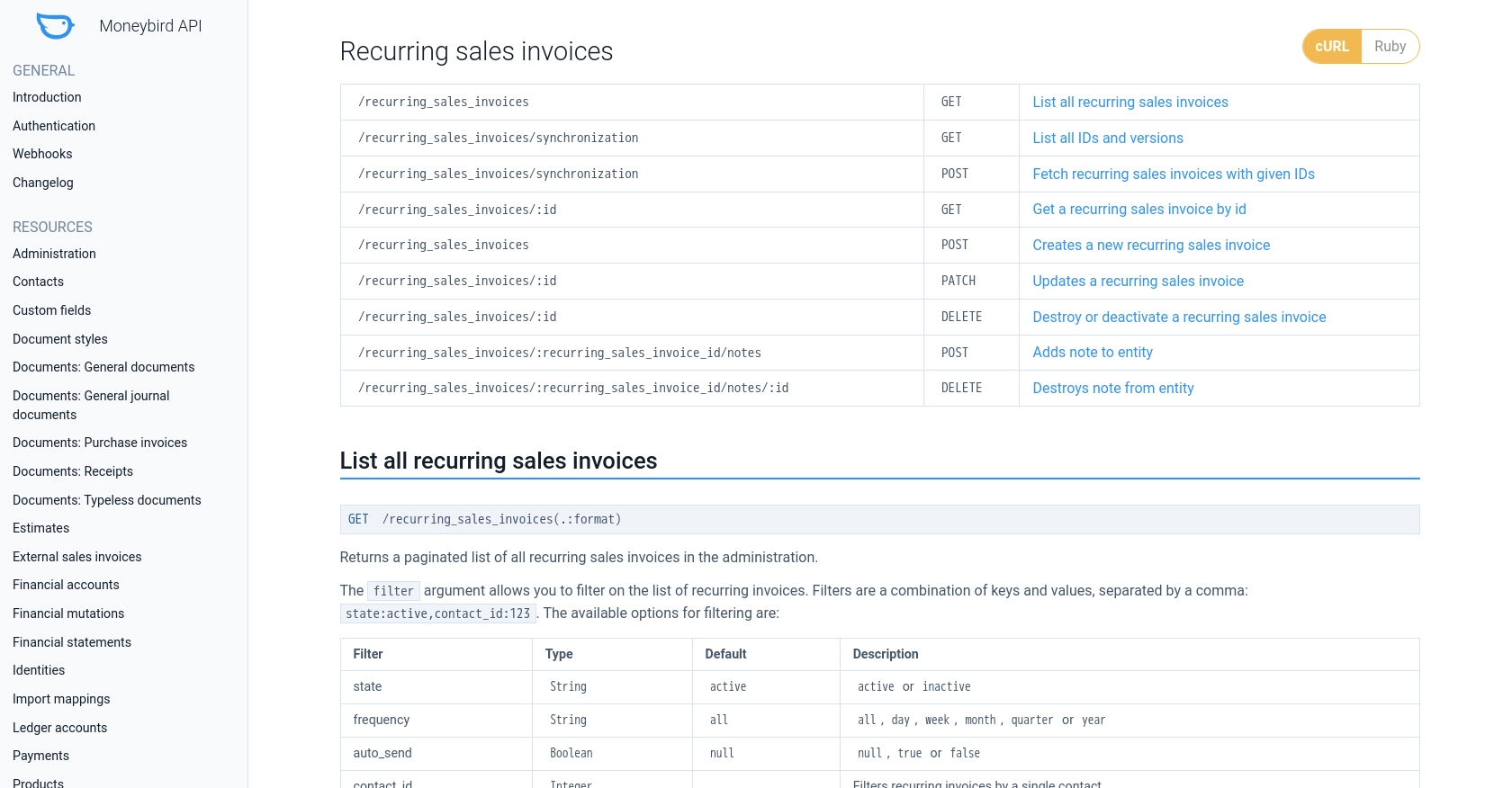
Conclusion and Best Practices for Using Moneybird API with Python
Integrating with the Moneybird API to manage recurring invoices using Python can significantly streamline your financial processes. By automating the retrieval and management of recurring invoices, you can enhance efficiency and accuracy in your financial operations.
Best Practices for Secure and Efficient API Integration with Moneybird
- Secure Credential Storage: Always store your API credentials securely. Use environment variables or secure vaults to protect your access tokens and client secrets.
- Handle Rate Limiting: Be mindful of Moneybird's rate limits, which allow 150 requests every 5 minutes. Implement retry logic with exponential backoff to handle
429 Too Many Requests
errors gracefully. - Data Standardization: Ensure that the data retrieved from Moneybird is standardized and transformed as needed to fit your application's requirements.
- Error Handling: Implement robust error handling to manage various HTTP status codes returned by the API. This will help maintain the reliability of your integration.
Leverage Endgrate for Simplified Integration Management
While integrating with Moneybird's API can be straightforward, managing multiple integrations across different platforms can become complex. Endgrate offers a unified API solution that simplifies integration management, allowing you to focus on your core product development. By using Endgrate, you can build once for each use case and leverage a single API endpoint to connect with multiple platforms, including Moneybird.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate and discover how you can save time and resources while providing an intuitive integration experience for your customers.
Read More
Ready to get started?